Song Genius API#
In this lesson, we’re going to use the Genius API to access data about Missy Elliott songs.
API Keys#
To use the Genius API, you need a special API key, specifically a “Client Access Token”, which is kind of like a password. Many APIs require authentication keys to gain access to them. To get your necessary Genius API keys, you need to navigate to the following URL: https://genius.com/api-clients.
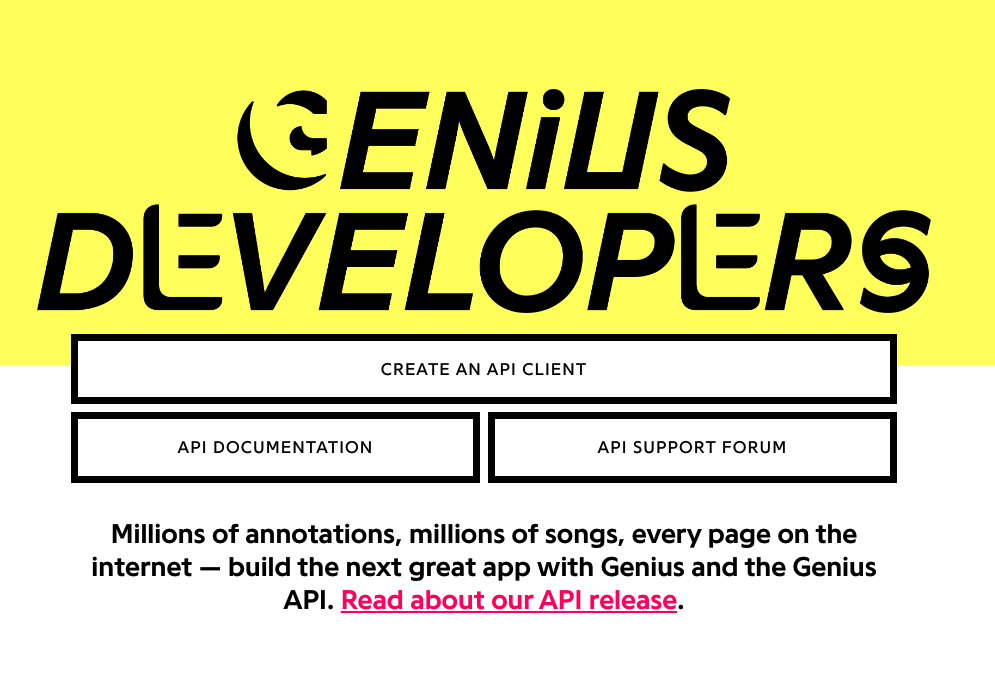
You’ll be prompted to sign up for a Genius account, which is required to gain API access. Signing up for a Genius account is free and easy. You just need a Genius nickname (which must be one word), an email address, and a password.
Once you’re signed in, you should be taken to https://genius.com/api-clients, where you need to click the button that says “New API Client.”
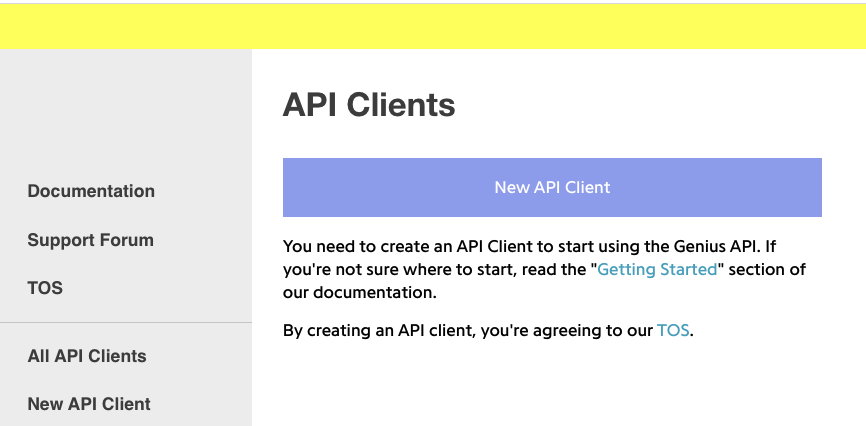
After clicking “New API Client,” you’ll be prompted to fill out a short form about the “App” that you need the Genius API for. You only need to fill out “App Name” and “App Website URL.”
It doesn’t really matter what you type in. You can simply put “Song Lyrics Project” for the “App Name” and the URL for our course website “https://melaniewalsh.github.io/Intro-Cultural-Analytics/” for the “App Website URL.”
When you click “Save,” you’ll be given a series of API Keys: a “Client ID” and a “Client Secret.” To generate your “Client Access Token,” which is the API key that we’ll be using in this notebook, you need to click “Generate Access Token”.
Finally, copy and paste your “Client Access Token” into the quotation marks below, and run the cell to save your variable
client_access_token = "INSERT YOUR CLIENT ACCESS TOKEN IN THESE QUOTATION MARKS"
Protecting Your API Key#
For this lesson, if you just copy and paste your Genius API key into your Jupyter notebook, everything should be fine. But that’s actually not the best way of storing your API keys. If you published this notebook to GitHub, for example, other people might be able to read and use/steal your API key.
For this reason, it’s best practice to keep your API keys away from your code, such as in another file. For example, I made a new Python file called “api_key.py” that contains just one variable your_client_access_token = "MY API KEY"
, and I can import this variable into my notebook with import api_key
.
import api_key
By importing this Python file/module, I get access to the variable your_client_access_token
without ever explicitly typing my secret API token in this notebook. If I wanted to publish this notebook to GitHub, then I could ignore or leave out the “api_key.py” file that actually contains my Client Access Token.
api_key.your_client_access_token
'INSERT YOUR API KEY HERE'
Attention
You should only un-comment and run the cell below if you are importing your API key from the Python file “api_key.py”. If you have already set your client_acces_token
in this notebook, you can skip the cell below.
#client_access_token = api_key.your_client_access_token
Making an API Request#
Making an API request looks a lot like typing a specially-formatted URL. But instead of getting a rendered HTML web page in return, you get some data in return.
There are a few different ways that we can query the Genius API, all of which are discussed in the Genius API documentation. The way we’re going to cover in this lesson is the basic search, which allows you to get a bunch of Genius data about any artist or songs that you search for:
http://api.genius.com/search?q={search_term}&access_token={client_access_token}
Sticking with our Missy Elliott theme/obsession, we’re going to search for Genius data about Missy Elliott.
First we’re going to assign the string “Missy Elliott” to the variable search_term
. Then we’re going to make an f-string URL that contains the variables search_term
and client_access_token
.
search_term = "Missy Elliott"
genius_search_url = f"http://api.genius.com/search?q={search_term}&access_token={client_access_token}"
This URL is basically all we need to make a Genius API request. Want proof? Run the cell below and print this URL, then copy and paste it into a new tab in your web browser.
print(genius_search_url)
http://api.genius.com/search?q=Missy Elliott&access_token=YOUR-API-KEY
It doesn’t look pretty, but that’s a bunch of Genius data about Missy Elliott!
We can programmatically do the same thing by again using the Python library requests
with this URL. Instead of getting the .text
of the response, as we did before, we’re going to use .json()
.
JSON is a data format that is commonly used by APIs. JSON data can be nested and contains key/value pairs, much like a Python dictionary.
import requests
response = requests.get(genius_search_url)
json_data = response.json()
The JSON data that we get from our Missy Elliott API query looks something like this:
json_data
{'meta': {'status': 200},
'response': {'hits': [{'highlights': [],
'index': 'song',
'type': 'song',
'result': {'annotation_count': 32,
'api_path': '/songs/4176',
'full_title': 'Work It by\xa0Missy\xa0Elliott',
'header_image_thumbnail_url': 'https://images.genius.com/27c1fbfef17041b435302af288cfa0c6.300x311x1.jpg',
'header_image_url': 'https://images.genius.com/27c1fbfef17041b435302af288cfa0c6.482x500x1.jpg',
'id': 4176,
'lyrics_owner_id': 6654,
'lyrics_state': 'complete',
'path': '/Missy-elliott-work-it-lyrics',
'pyongs_count': 39,
'song_art_image_thumbnail_url': 'https://images.genius.com/27c1fbfef17041b435302af288cfa0c6.300x311x1.jpg',
'song_art_image_url': 'https://images.genius.com/27c1fbfef17041b435302af288cfa0c6.482x500x1.jpg',
'stats': {'unreviewed_annotations': 2,
'hot': False,
'pageviews': 1205121},
'title': 'Work It',
'title_with_featured': 'Work It',
'url': 'https://genius.com/Missy-elliott-work-it-lyrics',
'song_art_primary_color': '#d63220',
'song_art_secondary_color': '#512913',
'song_art_text_color': '#fff',
'primary_artist': {'api_path': '/artists/1529',
'header_image_url': 'https://images.genius.com/d91c82fa4ae2f1016fadc1c24fbbc59e.1000x333x1.jpg',
'id': 1529,
'image_url': 'https://images.genius.com/085828b7d79bf8cf068b1557ca7a5e4c.1000x1000x1.jpg',
'is_meme_verified': False,
'is_verified': False,
'name': 'Missy Elliott',
'url': 'https://genius.com/artists/Missy-elliott'}}},
{'highlights': [],
'index': 'song',
'type': 'song',
'result': {'annotation_count': 27,
'api_path': '/songs/2368201',
'full_title': 'WTF (Where They From) by\xa0Missy\xa0Elliott (Ft.\xa0Pharrell\xa0Williams)',
'header_image_thumbnail_url': 'https://images.rapgenius.com/a439d6d6020559ffe7c10f0d73722de1.300x300x1.jpg',
'header_image_url': 'https://images.rapgenius.com/a439d6d6020559ffe7c10f0d73722de1.1000x1000x1.jpg',
'id': 2368201,
'lyrics_owner_id': 282633,
'lyrics_state': 'complete',
'path': '/Missy-elliott-wtf-where-they-from-lyrics',
'pyongs_count': 88,
'song_art_image_thumbnail_url': 'https://images.rapgenius.com/a439d6d6020559ffe7c10f0d73722de1.300x300x1.jpg',
'song_art_image_url': 'https://images.rapgenius.com/a439d6d6020559ffe7c10f0d73722de1.1000x1000x1.jpg',
'stats': {'unreviewed_annotations': 0, 'hot': False, 'pageviews': 289808},
'title': 'WTF (Where They From)',
'title_with_featured': 'WTF (Where They From) (Ft.\xa0Pharrell\xa0Williams)',
'url': 'https://genius.com/Missy-elliott-wtf-where-they-from-lyrics',
'song_art_primary_color': '#c920a0',
'song_art_secondary_color': '#500863',
'song_art_text_color': '#fff',
'primary_artist': {'api_path': '/artists/1529',
'header_image_url': 'https://images.genius.com/d91c82fa4ae2f1016fadc1c24fbbc59e.1000x333x1.jpg',
'id': 1529,
'image_url': 'https://images.genius.com/085828b7d79bf8cf068b1557ca7a5e4c.1000x1000x1.jpg',
'is_meme_verified': False,
'is_verified': False,
'name': 'Missy Elliott',
'url': 'https://genius.com/artists/Missy-elliott'}}},
{'highlights': [],
'index': 'song',
'type': 'song',
'result': {'annotation_count': 14,
'api_path': '/songs/4640',
'full_title': 'Get Ur Freak On by\xa0Missy\xa0Elliott',
'header_image_thumbnail_url': 'https://images.genius.com/338e122c1534b6f6363afb06f77912cf.300x259x1.jpg',
'header_image_url': 'https://images.genius.com/338e122c1534b6f6363afb06f77912cf.300x259x1.jpg',
'id': 4640,
'lyrics_owner_id': 1297,
'lyrics_state': 'complete',
'path': '/Missy-elliott-get-ur-freak-on-lyrics',
'pyongs_count': 18,
'song_art_image_thumbnail_url': 'https://images.genius.com/338e122c1534b6f6363afb06f77912cf.300x259x1.jpg',
'song_art_image_url': 'https://images.genius.com/338e122c1534b6f6363afb06f77912cf.300x259x1.jpg',
'stats': {'unreviewed_annotations': 0, 'hot': False, 'pageviews': 165394},
'title': 'Get Ur Freak On',
'title_with_featured': 'Get Ur Freak On',
'url': 'https://genius.com/Missy-elliott-get-ur-freak-on-lyrics',
'song_art_primary_color': '#d58f3a',
'song_art_secondary_color': '#eddb91',
'song_art_text_color': '#000',
'primary_artist': {'api_path': '/artists/1529',
'header_image_url': 'https://images.genius.com/d91c82fa4ae2f1016fadc1c24fbbc59e.1000x333x1.jpg',
'id': 1529,
'image_url': 'https://images.genius.com/085828b7d79bf8cf068b1557ca7a5e4c.1000x1000x1.jpg',
'is_meme_verified': False,
'is_verified': False,
'name': 'Missy Elliott',
'url': 'https://genius.com/artists/Missy-elliott'}}},
{'highlights': [],
'index': 'song',
'type': 'song',
'result': {'annotation_count': 41,
'api_path': '/songs/4173',
'full_title': 'Gossip Folks by\xa0Missy\xa0Elliott (Ft.\xa0Ludacris)',
'header_image_thumbnail_url': 'https://images.genius.com/206153c53d05804677e236aa2ae4eb45.300x300x1.jpg',
'header_image_url': 'https://images.genius.com/206153c53d05804677e236aa2ae4eb45.600x600x1.jpg',
'id': 4173,
'lyrics_owner_id': 6654,
'lyrics_state': 'complete',
'path': '/Missy-elliott-gossip-folks-lyrics',
'pyongs_count': 7,
'song_art_image_thumbnail_url': 'https://images.genius.com/206153c53d05804677e236aa2ae4eb45.300x300x1.jpg',
'song_art_image_url': 'https://images.genius.com/206153c53d05804677e236aa2ae4eb45.600x600x1.jpg',
'stats': {'unreviewed_annotations': 0, 'hot': False, 'pageviews': 111483},
'title': 'Gossip Folks',
'title_with_featured': 'Gossip Folks (Ft.\xa0Ludacris)',
'url': 'https://genius.com/Missy-elliott-gossip-folks-lyrics',
'song_art_primary_color': '#ad3920',
'song_art_secondary_color': '#72281f',
'song_art_text_color': '#fff',
'primary_artist': {'api_path': '/artists/1529',
'header_image_url': 'https://images.genius.com/d91c82fa4ae2f1016fadc1c24fbbc59e.1000x333x1.jpg',
'id': 1529,
'image_url': 'https://images.genius.com/085828b7d79bf8cf068b1557ca7a5e4c.1000x1000x1.jpg',
'is_meme_verified': False,
'is_verified': False,
'name': 'Missy Elliott',
'url': 'https://genius.com/artists/Missy-elliott'}}},
{'highlights': [],
'index': 'song',
'type': 'song',
'result': {'annotation_count': 9,
'api_path': '/songs/2967124',
'full_title': "I'm Better by\xa0Missy\xa0Elliott (Ft.\xa0Cainon\xa0Lamb)",
'header_image_thumbnail_url': 'https://images.genius.com/609afe10820cce09ce9e3c768518c63c.300x300x1.jpg',
'header_image_url': 'https://images.genius.com/609afe10820cce09ce9e3c768518c63c.600x600x1.jpg',
'id': 2967124,
'lyrics_owner_id': 3075478,
'lyrics_state': 'complete',
'path': '/Missy-elliott-im-better-lyrics',
'pyongs_count': 13,
'song_art_image_thumbnail_url': 'https://images.genius.com/609afe10820cce09ce9e3c768518c63c.300x300x1.jpg',
'song_art_image_url': 'https://images.genius.com/609afe10820cce09ce9e3c768518c63c.600x600x1.jpg',
'stats': {'unreviewed_annotations': 0, 'hot': False, 'pageviews': 107273},
'title': 'I’m Better',
'title_with_featured': "I'm Better (Ft.\xa0Cainon\xa0Lamb)",
'url': 'https://genius.com/Missy-elliott-im-better-lyrics',
'song_art_primary_color': '#ba704b',
'song_art_secondary_color': '#6b3621',
'song_art_text_color': '#fff',
'primary_artist': {'api_path': '/artists/1529',
'header_image_url': 'https://images.genius.com/d91c82fa4ae2f1016fadc1c24fbbc59e.1000x333x1.jpg',
'id': 1529,
'image_url': 'https://images.genius.com/085828b7d79bf8cf068b1557ca7a5e4c.1000x1000x1.jpg',
'is_meme_verified': False,
'is_verified': False,
'name': 'Missy Elliott',
'url': 'https://genius.com/artists/Missy-elliott'}}},
{'highlights': [],
'index': 'song',
'type': 'song',
'result': {'annotation_count': 16,
'api_path': '/songs/128',
'full_title': 'The Rain (Supa Dupa Fly) by\xa0Missy\xa0Elliott',
'header_image_thumbnail_url': 'https://images.genius.com/a1543159342cd360256c12b42185771f.300x308x1.jpg',
'header_image_url': 'https://images.genius.com/a1543159342cd360256c12b42185771f.975x1000x1.jpg',
'id': 128,
'lyrics_owner_id': 4619460,
'lyrics_state': 'complete',
'path': '/Missy-elliott-the-rain-supa-dupa-fly-lyrics',
'pyongs_count': 6,
'song_art_image_thumbnail_url': 'https://images.genius.com/a1543159342cd360256c12b42185771f.300x308x1.jpg',
'song_art_image_url': 'https://images.genius.com/a1543159342cd360256c12b42185771f.975x1000x1.jpg',
'stats': {'unreviewed_annotations': 0, 'hot': False, 'pageviews': 103348},
'title': 'The Rain (Supa Dupa Fly)',
'title_with_featured': 'The Rain (Supa Dupa Fly)',
'url': 'https://genius.com/Missy-elliott-the-rain-supa-dupa-fly-lyrics',
'song_art_primary_color': '#3a73ac',
'song_art_secondary_color': '#2d3c74',
'song_art_text_color': '#fff',
'primary_artist': {'api_path': '/artists/1529',
'header_image_url': 'https://images.genius.com/d91c82fa4ae2f1016fadc1c24fbbc59e.1000x333x1.jpg',
'id': 1529,
'image_url': 'https://images.genius.com/085828b7d79bf8cf068b1557ca7a5e4c.1000x1000x1.jpg',
'is_meme_verified': False,
'is_verified': False,
'name': 'Missy Elliott',
'url': 'https://genius.com/artists/Missy-elliott'}}},
{'highlights': [],
'index': 'song',
'type': 'song',
'result': {'annotation_count': 5,
'api_path': '/songs/33158',
'full_title': 'Lose Control by\xa0Missy\xa0Elliott (Ft.\xa0Ciara & Fatman\xa0Scoop)',
'header_image_thumbnail_url': 'https://images.genius.com/51639e476eac5cb5bed5794c023763ff.300x303x1.jpg',
'header_image_url': 'https://images.genius.com/51639e476eac5cb5bed5794c023763ff.700x707x1.jpg',
'id': 33158,
'lyrics_owner_id': 50,
'lyrics_state': 'complete',
'path': '/Missy-elliott-lose-control-lyrics',
'pyongs_count': 11,
'song_art_image_thumbnail_url': 'https://images.genius.com/51639e476eac5cb5bed5794c023763ff.300x303x1.jpg',
'song_art_image_url': 'https://images.genius.com/51639e476eac5cb5bed5794c023763ff.700x707x1.jpg',
'stats': {'unreviewed_annotations': 0, 'hot': False, 'pageviews': 93331},
'title': 'Lose Control',
'title_with_featured': 'Lose Control (Ft.\xa0Ciara & Fatman\xa0Scoop)',
'url': 'https://genius.com/Missy-elliott-lose-control-lyrics',
'song_art_primary_color': '#cf7129',
'song_art_secondary_color': '#77330b',
'song_art_text_color': '#fff',
'primary_artist': {'api_path': '/artists/1529',
'header_image_url': 'https://images.genius.com/d91c82fa4ae2f1016fadc1c24fbbc59e.1000x333x1.jpg',
'id': 1529,
'image_url': 'https://images.genius.com/085828b7d79bf8cf068b1557ca7a5e4c.1000x1000x1.jpg',
'is_meme_verified': False,
'is_verified': False,
'name': 'Missy Elliott',
'url': 'https://genius.com/artists/Missy-elliott'}}},
{'highlights': [],
'index': 'song',
'type': 'song',
'result': {'annotation_count': 17,
'api_path': '/songs/4671',
'full_title': 'One Minute Man (Amended Version) by\xa0Missy\xa0Elliott (Ft.\xa0Ludacris & Trina)',
'header_image_thumbnail_url': 'https://images.genius.com/a94eaf3c98a7134cb546ebf9e6d2b991.300x300x1.jpg',
'header_image_url': 'https://images.genius.com/a94eaf3c98a7134cb546ebf9e6d2b991.953x953x1.jpg',
'id': 4671,
'lyrics_owner_id': 7299,
'lyrics_state': 'complete',
'path': '/Missy-elliott-one-minute-man-amended-version-lyrics',
'pyongs_count': 6,
'song_art_image_thumbnail_url': 'https://images.genius.com/a94eaf3c98a7134cb546ebf9e6d2b991.300x300x1.jpg',
'song_art_image_url': 'https://images.genius.com/a94eaf3c98a7134cb546ebf9e6d2b991.953x953x1.jpg',
'stats': {'unreviewed_annotations': 5, 'hot': False, 'pageviews': 74646},
'title': 'One Minute Man (Amended Version)',
'title_with_featured': 'One Minute Man (Amended Version) (Ft.\xa0Ludacris & Trina)',
'url': 'https://genius.com/Missy-elliott-one-minute-man-amended-version-lyrics',
'song_art_primary_color': '#fbbd46',
'song_art_secondary_color': '#f5deae',
'song_art_text_color': '#000',
'primary_artist': {'api_path': '/artists/1529',
'header_image_url': 'https://images.genius.com/d91c82fa4ae2f1016fadc1c24fbbc59e.1000x333x1.jpg',
'id': 1529,
'image_url': 'https://images.genius.com/085828b7d79bf8cf068b1557ca7a5e4c.1000x1000x1.jpg',
'is_meme_verified': False,
'is_verified': False,
'name': 'Missy Elliott',
'url': 'https://genius.com/artists/Missy-elliott'}}},
{'highlights': [],
'index': 'song',
'type': 'song',
'result': {'annotation_count': 1,
'api_path': '/songs/4042648',
'full_title': 'This Is Me (The Reimagined Remix) by\xa0Keala\xa0Settle, Kesha & Missy Elliott',
'header_image_thumbnail_url': 'https://images.genius.com/29f15eb96f814e785598d0eea14126a7.300x300x1.png',
'header_image_url': 'https://images.genius.com/29f15eb96f814e785598d0eea14126a7.1000x1000x1.png',
'id': 4042648,
'lyrics_owner_id': 93685,
'lyrics_state': 'complete',
'path': '/Keala-settle-kesha-and-missy-elliott-this-is-me-the-reimagined-remix-lyrics',
'pyongs_count': 16,
'song_art_image_thumbnail_url': 'https://images.genius.com/29f15eb96f814e785598d0eea14126a7.300x300x1.png',
'song_art_image_url': 'https://images.genius.com/29f15eb96f814e785598d0eea14126a7.1000x1000x1.png',
'stats': {'unreviewed_annotations': 0, 'hot': False, 'pageviews': 49366},
'title': 'This Is Me (The Reimagined Remix)',
'title_with_featured': 'This Is Me (The Reimagined Remix)',
'url': 'https://genius.com/Keala-settle-kesha-and-missy-elliott-this-is-me-the-reimagined-remix-lyrics',
'song_art_primary_color': '#c9a45c',
'song_art_secondary_color': '#f5e093',
'song_art_text_color': '#000',
'primary_artist': {'api_path': '/artists/1600466',
'header_image_url': 'https://assets.genius.com/images/default_avatar_300.png?1630359615',
'id': 1600466,
'image_url': 'https://assets.genius.com/images/default_avatar_300.png?1630359615',
'is_meme_verified': False,
'is_verified': False,
'name': 'Keala Settle, Kesha & Missy Elliott',
'url': 'https://genius.com/artists/Keala-settle-kesha-and-missy-elliott'}}},
{'highlights': [],
'index': 'song',
'type': 'song',
'result': {'annotation_count': 18,
'api_path': '/songs/33191',
'full_title': 'Bomb Intro/Pass That Dutch by\xa0Missy\xa0Elliott',
'header_image_thumbnail_url': 'https://images.genius.com/8c2a5015c6ba9e5f0c154f9a20f14534.300x300x1.jpg',
'header_image_url': 'https://images.genius.com/8c2a5015c6ba9e5f0c154f9a20f14534.583x583x1.jpg',
'id': 33191,
'lyrics_owner_id': 50,
'lyrics_state': 'complete',
'path': '/Missy-elliott-bomb-intro-pass-that-dutch-lyrics',
'pyongs_count': 5,
'song_art_image_thumbnail_url': 'https://images.genius.com/8c2a5015c6ba9e5f0c154f9a20f14534.300x300x1.jpg',
'song_art_image_url': 'https://images.genius.com/8c2a5015c6ba9e5f0c154f9a20f14534.583x583x1.jpg',
'stats': {'unreviewed_annotations': 2, 'hot': False, 'pageviews': 48848},
'title': 'Bomb Intro/Pass That Dutch',
'title_with_featured': 'Bomb Intro/Pass That Dutch',
'url': 'https://genius.com/Missy-elliott-bomb-intro-pass-that-dutch-lyrics',
'song_art_primary_color': '#7f5833',
'song_art_secondary_color': '#5e4125',
'song_art_text_color': '#fff',
'primary_artist': {'api_path': '/artists/1529',
'header_image_url': 'https://images.genius.com/d91c82fa4ae2f1016fadc1c24fbbc59e.1000x333x1.jpg',
'id': 1529,
'image_url': 'https://images.genius.com/085828b7d79bf8cf068b1557ca7a5e4c.1000x1000x1.jpg',
'is_meme_verified': False,
'is_verified': False,
'name': 'Missy Elliott',
'url': 'https://genius.com/artists/Missy-elliott'}}}]}}
We can index this data (again, like a Python dictionary) and look at the first “hit” about Missy Elliott from Genius.com.
json_data['response']['hits'][0]
{'highlights': [],
'index': 'song',
'type': 'song',
'result': {'annotation_count': 32,
'api_path': '/songs/4176',
'full_title': 'Work It by\xa0Missy\xa0Elliott',
'header_image_thumbnail_url': 'https://images.genius.com/27c1fbfef17041b435302af288cfa0c6.300x311x1.jpg',
'header_image_url': 'https://images.genius.com/27c1fbfef17041b435302af288cfa0c6.482x500x1.jpg',
'id': 4176,
'lyrics_owner_id': 6654,
'lyrics_state': 'complete',
'path': '/Missy-elliott-work-it-lyrics',
'pyongs_count': 39,
'song_art_image_thumbnail_url': 'https://images.genius.com/27c1fbfef17041b435302af288cfa0c6.300x311x1.jpg',
'song_art_image_url': 'https://images.genius.com/27c1fbfef17041b435302af288cfa0c6.482x500x1.jpg',
'stats': {'unreviewed_annotations': 2, 'hot': False, 'pageviews': 1205121},
'title': 'Work It',
'title_with_featured': 'Work It',
'url': 'https://genius.com/Missy-elliott-work-it-lyrics',
'song_art_primary_color': '#d63220',
'song_art_secondary_color': '#512913',
'song_art_text_color': '#fff',
'primary_artist': {'api_path': '/artists/1529',
'header_image_url': 'https://images.genius.com/d91c82fa4ae2f1016fadc1c24fbbc59e.1000x333x1.jpg',
'id': 1529,
'image_url': 'https://images.genius.com/085828b7d79bf8cf068b1557ca7a5e4c.1000x1000x1.jpg',
'is_meme_verified': False,
'is_verified': False,
'name': 'Missy Elliott',
'url': 'https://genius.com/artists/Missy-elliott'}}}
We can tell that this data describes the song “Work It” and contains other information about the song, such as its number of Genius annotations, its number of web page views, and links to images of its album cover.
Looping Through JSON Data#
Get Song Titles#
for song in json_data['response']['hits']:
print(song['result']['full_title'])
Work It by Missy Elliott
WTF (Where They From) by Missy Elliott (Ft. Pharrell Williams)
Get Ur Freak On by Missy Elliott
Gossip Folks by Missy Elliott (Ft. Ludacris)
I'm Better by Missy Elliott (Ft. Cainon Lamb)
The Rain (Supa Dupa Fly) by Missy Elliott
Lose Control by Missy Elliott (Ft. Ciara & Fatman Scoop)
One Minute Man (Amended Version) by Missy Elliott (Ft. Ludacris & Trina)
This Is Me (The Reimagined Remix) by Keala Settle, Kesha & Missy Elliott
Bomb Intro/Pass That Dutch by Missy Elliott
Get Song Tiles and Page View Counts#
for song in json_data['response']['hits']:
print(song['result']['full_title'], song['result']['stats']['pageviews'])
Work It by Missy Elliott 1205121
WTF (Where They From) by Missy Elliott (Ft. Pharrell Williams) 289808
Get Ur Freak On by Missy Elliott 165394
Gossip Folks by Missy Elliott (Ft. Ludacris) 111483
I'm Better by Missy Elliott (Ft. Cainon Lamb) 107273
The Rain (Supa Dupa Fly) by Missy Elliott 103348
Lose Control by Missy Elliott (Ft. Ciara & Fatman Scoop) 93331
One Minute Man (Amended Version) by Missy Elliott (Ft. Ludacris & Trina) 74646
This Is Me (The Reimagined Remix) by Keala Settle, Kesha & Missy Elliott 49366
Bomb Intro/Pass That Dutch by Missy Elliott 48848
Transform Song Titles and Page View Counts into a DataFrame#
We can loop through this data, append it into a list, and then transform that list into a Pandas dataframe by calling pd.DataFrame()
import pandas as pd
Pandas Review
Do you need a refresher or introduction to the Python data analysis library Pandas? Be sure to check out Pandas Basics (1-3) in this textbook!
missy_songs = []
for song in json_data['response']['hits']:
missy_songs.append([song['result']['full_title'], song['result']['stats']['pageviews']])
#Make a Pandas dataframe from a list
missy_df = pd.DataFrame(missy_songs)
missy_df.columns = ['song_title', 'page_views']
missy_df
song_title | page_views | |
---|---|---|
0 | Work It by Missy Elliott | 1205121 |
1 | WTF (Where They From) by Missy Elliott (Ft. Ph... | 289808 |
2 | Get Ur Freak On by Missy Elliott | 165394 |
3 | Gossip Folks by Missy Elliott (Ft. Ludacris) | 111483 |
4 | I'm Better by Missy Elliott (Ft. Cainon Lamb) | 107273 |
5 | The Rain (Supa Dupa Fly) by Missy Elliott | 103348 |
6 | Lose Control by Missy Elliott (Ft. Ciara & Fat... | 93331 |
7 | One Minute Man (Amended Version) by Missy Elli... | 74646 |
8 | This Is Me (The Reimagined Remix) by Keala Set... | 49366 |
9 | Bomb Intro/Pass That Dutch by Missy Elliott | 48848 |
Transform Song Titles, Page View Counts, & Album Covers into a DataFrame#
Just for fun, we can do the same thing but also add links to images of Missy Elliott’s album art—and we can actually display those images, too!
To display images in a Pandas dataframe, you need to run from IPython.core.display import HTML
and make the function get_image_html()
. We’re going to take the image URLs and make them into HTML objects.
from IPython.core.display import HTML
def get_image_html(link):
image_html = f"<img src='{link}' width='500px'>"
return image_html
missy_songs = []
for song in json_data['response']['hits']:
missy_songs.append([song['result']['full_title'], song['result']['stats']['pageviews'], song['result']['song_art_image_url']])
missy_df = pd.DataFrame(missy_songs)
missy_df.columns = ['song_title', 'page_views','album_cover_url']
#Use the function get_image_html()
missy_df['album_cover'] = missy_df['album_cover_url'].apply(get_image_html)
missy_df
song_title | page_views | album_cover_url | album_cover | |
---|---|---|---|---|
0 | Work It by Missy Elliott | 1205121 | https://images.genius.com/27c1fbfef17041b43530... | <img src='https://images.genius.com/27c1fbfef1... |
1 | WTF (Where They From) by Missy Elliott (Ft. Ph... | 289808 | https://images.rapgenius.com/a439d6d6020559ffe... | <img src='https://images.rapgenius.com/a439d6d... |
2 | Get Ur Freak On by Missy Elliott | 165394 | https://images.genius.com/338e122c1534b6f6363a... | <img src='https://images.genius.com/338e122c15... |
3 | Gossip Folks by Missy Elliott (Ft. Ludacris) | 111483 | https://images.genius.com/206153c53d05804677e2... | <img src='https://images.genius.com/206153c53d... |
4 | I'm Better by Missy Elliott (Ft. Cainon Lamb) | 107273 | https://images.genius.com/609afe10820cce09ce9e... | <img src='https://images.genius.com/609afe1082... |
5 | The Rain (Supa Dupa Fly) by Missy Elliott | 103348 | https://images.genius.com/a1543159342cd360256c... | <img src='https://images.genius.com/a154315934... |
6 | Lose Control by Missy Elliott (Ft. Ciara & Fat... | 93331 | https://images.genius.com/51639e476eac5cb5bed5... | <img src='https://images.genius.com/51639e476e... |
7 | One Minute Man (Amended Version) by Missy Elli... | 74646 | https://images.genius.com/a94eaf3c98a7134cb546... | <img src='https://images.genius.com/a94eaf3c98... |
8 | This Is Me (The Reimagined Remix) by Keala Set... | 49366 | https://images.genius.com/29f15eb96f814e785598... | <img src='https://images.genius.com/29f15eb96f... |
9 | Bomb Intro/Pass That Dutch by Missy Elliott | 48848 | https://images.genius.com/8c2a5015c6ba9e5f0c15... | <img src='https://images.genius.com/8c2a5015c6... |
If we call HTML()
on our dataframe and add the method .to_html(escape=False)
to the dataframe, then it should display the dataframe with viewable images.
HTML(missy_df[['album_cover', 'page_views', 'song_title']].to_html(escape=False))
album_cover | page_views | song_title | |
---|---|---|---|
0 | ![]() |
1205121 | Work It by Missy Elliott |
1 | ![]() |
289808 | WTF (Where They From) by Missy Elliott (Ft. Pharrell Williams) |
2 | ![]() |
165394 | Get Ur Freak On by Missy Elliott |
3 | ![]() |
111483 | Gossip Folks by Missy Elliott (Ft. Ludacris) |
4 | ![]() |
107273 | I'm Better by Missy Elliott (Ft. Cainon Lamb) |
5 | ![]() |
103348 | The Rain (Supa Dupa Fly) by Missy Elliott |
6 | ![]() |
93331 | Lose Control by Missy Elliott (Ft. Ciara & Fatman Scoop) |
7 | ![]() |
74646 | One Minute Man (Amended Version) by Missy Elliott (Ft. Ludacris & Trina) |
8 | ![]() |
49366 | This Is Me (The Reimagined Remix) by Keala Settle, Kesha & Missy Elliott |
9 | ![]() |
48848 | Bomb Intro/Pass That Dutch by Missy Elliott |
Your Turn!#
Replace “Phoebe Bridgers” with any artist/musician of your choosing and run the following cells.
search_term = "Phoebe Bridgers"
genius_search_url = f"http://api.genius.com/search?q={search_term}&access_token={client_access_token}"
response = requests.get(genius_search_url)
json_data = response.json()
songs = []
for song in json_data['response']['hits']:
songs.append((song['result']['full_title'], song['result']['stats']['pageviews'], song['result']['song_art_image_url']))
artist_df = pd.DataFrame(songs)
artist_df.columns = ['song_title', 'page_views', 'album_cover_url']
artist_df['album_cover'] = artist_df['album_cover_url'].apply(get_image_html)
HTML(artist_df[['album_cover', 'page_views', 'song_title']].to_html(escape=False))
album_cover | page_views | song_title | |
---|---|---|---|
0 | ![]() |
302599 | Kyoto by Phoebe Bridgers |
1 | ![]() |
328494 | Motion Sickness by Phoebe Bridgers |
2 | ![]() |
277719 | I Know the End by Phoebe Bridgers |
3 | ![]() |
217543 | Punisher by Phoebe Bridgers |
4 | ![]() |
229410 | Garden Song by Phoebe Bridgers |
5 | ![]() |
198241 | Smoke Signals by Phoebe Bridgers |
6 | ![]() |
188749 | Savior Complex by Phoebe Bridgers |
7 | ![]() |
162229 | Chinese Satellite by Phoebe Bridgers |
8 | ![]() |
170431 | Graceland Too by Phoebe Bridgers |
9 | ![]() |
147483 | Funeral by Phoebe Bridgers |