Topic Modeling — CSV Files#
In these lessons, we’re learning about a text analysis method called topic modeling. This method will help us identify the main topics or discourses within a collection of texts a single text that has been separated into smaller text chunks.
Dataset#
Am I the Asshole?#
AITA for lying about my biggest fear on a quiz show and subsequently winning a car and making other contestants lose?
—Reddit user iwonacar, r/AmItheAsshole
In this particular lesson, we’re going to use Little MALLET Wrapper, a Python wrapper for MALLET, to topic model a CSV file with 2,932 Reddit posts from the subreddit r/AmITheAsshole that have at least an upvote score of 2,000. This is an online forum where people share their personal conflicts and ask the community to judge who’s the a**hole in the story. This data was collected with PSAW, a wrapper for the Pushshift API.
Attention
If you're working in this Jupyter notebook on your own computer, you'll need to have both the Java Development Kit and MALLET pre-installed. For set up instructions, please see the previous lesson.
If you’re working in this Jupyter notebook in the cloud via Binder, then the Java Development Kit and Mallet will already be installed. You’re good to go!
Set MALLET Path#
Since Little MALLET Wrapper is a Python package built around MALLET, we first need to tell it where the bigger, Java-based MALLET lives.
We’re going to make a variable called path_to_mallet
and assign it the file path of our MALLET program. We need to point it, specifically, to the “mallet” file inside the “bin” folder inside the “mallet-2.0.8” folder.
path_to_mallet = 'mallet-2.0.8/bin/mallet'
If MALLET is located in another directory, then set your path_to_mallet
to that file path.
Install Packages#
#!pip install little_mallet_wrapper
#!pip install seaborn
#To install the most updated version of little_mallet_wrapper:
#!!pip install git+https://github.com/maria-antoniak/little-mallet-wrapper.git
Import Packages#
Now let’s import
the little_mallet_wrapper
and the data viz library seaborn
.
import little_mallet_wrapper
import seaborn
import glob
from pathlib import Path
import pandas as pd
import random
pd.options.display.max_colwidth = 100
We’re also going to import glob
and pathlib
for working with files and the file system, as well as pandas for working with the CSV data.
Get Training Data From CSV File#
Note
We're calling these text files our *training data*, because we're *training* our topic model with these texts. The topic model will be learning and extracting topics based on these texts.Pandas
Do you need a refresher or introduction to the Python data analysis library Pandas? Be sure to check out Pandas Basics (1-3) in this textbook!reddit_df = pd.read_csv("../data/top-reddit-aita-posts.csv")
reddit_df.head()
author | full_date | date | title | selftext | url | subreddit | upvote_score | num_comments | num_crossposts | |
---|---|---|---|---|---|---|---|---|---|---|
0 | Additional-Pizza-805 | 2020-07-24 19:13:49+00:00 | 2020-07-24 | AITA for kicking my cousin off of my sister’s wedding Zoom call? | My [27M] older sister [30F] and her fiancé [31M] were planning for over a year for their wedding... | https://www.reddit.com/r/AmItheAsshole/comments/hx80wd/aita_for_kicking_my_cousin_off_of_my_sist... | AmItheAsshole | 11159 | 2209 | 4 |
1 | decadel8ter | 2020-07-24 14:37:13+00:00 | 2020-07-24 | AITA for resenting my family for something that happened over a decade ago? | when i was 15 i was in a car accident. i was riding my bike on new bike lanes that my city had i... | https://www.reddit.com/r/AmItheAsshole/comments/hx2vvl/aita_for_resenting_my_family_for_somethin... | AmItheAsshole | 2541 | 1143 | 0 |
2 | Snoo_66130 | 2020-07-24 12:35:35+00:00 | 2020-07-24 | AITA for telling my step dad to stop trying to be my dad? | I'm 35, and my mom who is 52 is dating a man who is is 27. This is fucking weird as hell and... | https://www.reddit.com/r/AmItheAsshole/comments/hx0zk7/aita_for_telling_my_step_dad_to_stop_tryi... | AmItheAsshole | 2809 | 1253 | 1 |
3 | ohnoihaveabluechair | 2020-07-24 10:56:56+00:00 | 2020-07-24 | AITA for confronting my SIL for wearing clothes that belonged to me? | Some info: A few years ago, my family didn’t have a lot of spare money to buy a lot of things (l... | https://www.reddit.com/r/AmItheAsshole/comments/hwzpbu/aita_for_confronting_my_sil_for_wearing_c... | AmItheAsshole | 7581 | 1550 | 1 |
4 | FormalLettuce3 | 2020-07-24 10:52:08+00:00 | 2020-07-24 | AITA for saying we'd only help with my ex's kid's party if we could tell people we're engaged? | This guy, "Jack", and I were together for about a year, and within a couple weeks of ending it I... | https://www.reddit.com/r/AmItheAsshole/comments/hwzncq/aita_for_saying_wed_only_help_with_my_exs... | AmItheAsshole | 2915 | 1214 | 0 |
reddit_df['selftext'] = reddit_df['selftext'].astype(str)
Process Reddit Posts#
little_mallet_wrapper.process_string(text, numbers='remove')
Next we’re going to process our texts with the function little_mallet_wrapper.process_string()
. This function will take every individual post, transform all the text to lowercase as well as remove stopwords, punctuation, and numbers, and then add the processed text to our master list training_data
.
training_data = [little_mallet_wrapper.process_string(text, numbers='remove') for text in reddit_df['selftext']]
original_texts = [text for text in reddit_df['selftext']]
Process Reddit Post Titles#
We’re also going to extract the file name for each Reddit post.
reddit_titles = [title for title in reddit_df['title']]
Get Dataset Statistics#
We can get training data summary statisitcs by using the funciton little_mallet_wrapper.print_dataset_stats()
.
little_mallet_wrapper.print_dataset_stats(training_data)
Number of Documents: 2932
Mean Number of Words per Document: 147.3
Vocabulary Size: 19331
Training the Topic Model#
Set Number of Topics#
We need to make a variable num_topics
and assign it the number of topics we want returned.
num_topics = 15
Set Training Data#
We already made a variable called training_data
, which includes all of our processed Reddit post texts, so we can just set it equal to itself.
training_data = training_data
Set Other MALLET File Paths#
Then we’re going to set a file path where we want all our MALLET topic modeling data to be dumped. I’m going to output everything onto my Desktop inside a folder called “topic-model-output” and a subfolder specific to the Reddit posts called “Reddit.”
All the other necessary variables below output_directory_path
will be automatically created inside this directory.
#Change to your desired output directory
output_directory_path = 'topic-model-output/reddit'
#No need to change anything below here
Path(f"{output_directory_path}").mkdir(parents=True, exist_ok=True)
path_to_training_data = f"{output_directory_path}/training.txt"
path_to_formatted_training_data = f"{output_directory_path}/mallet.training"
path_to_model = f"{output_directory_path}/mallet.model.{str(num_topics)}"
path_to_topic_keys = f"{output_directory_path}/mallet.topic_keys.{str(num_topics)}"
path_to_topic_distributions = f"{output_directory_path}/mallet.topic_distributions.{str(num_topics)}"
Train Topic Model#
Then we’re going to train our topic model with little_mallet_wrapper.quick_train_topic_model()
. The topic model should take about 1-1.5 minutes to train and complete. If you want it, you can look at your Terminal or PowerShell and see what the model looks like as it’s training.
little_mallet_wrapper.quick_train_topic_model(path_to_mallet,
output_directory_path,
num_topics,
training_data)
Importing data...
Complete
Training topic model...
Complete
([['baby',
'pregnant',
'child',
'husband',
'pregnancy',
'pain',
'birth',
'hospital',
'doctor',
'could',
'sil',
'think',
'abortion',
'would',
'medical',
'surgery',
'help',
'weeks',
'control',
'children'],
['table',
'store',
'manager',
'drink',
'order',
'restaurant',
'bar',
'drinking',
'drinks',
'pay',
'tip',
'place',
'wine',
'people',
'coffee',
'bottle',
'food',
'alcohol',
'beer',
'ordered'],
['money',
'pay',
'buy',
'would',
'paying',
'college',
'job',
'bought',
'house',
'gift',
'paid',
'car',
'give',
'afford',
'since',
'year',
'got',
'rent',
'working',
'much'],
['time',
'get',
'like',
'want',
'work',
'one',
'doesn',
'would',
'going',
'take',
'even',
'home',
'really',
'think',
'day',
'every',
'make',
'feel',
'something',
'week'],
['sister',
'mom',
'dad',
'family',
'parents',
'brother',
'mother',
'cousin',
'aunt',
'year',
'father',
'also',
'house',
'siblings',
'older',
'nephew',
'always',
'close',
'birthday',
'years'],
['school',
'work',
'office',
'class',
'name',
'people',
'company',
'high',
'teacher',
'boss',
'job',
'coworkers',
'students',
'team',
'college',
'year',
'one',
'student',
'english',
'coworker'],
['years',
'told',
'would',
'never',
'wife',
'life',
'family',
'ago',
'relationship',
'time',
'know',
'want',
'together',
'love',
'months',
'married',
'feel',
'even',
'things',
'much'],
['kids',
'daughter',
'son',
'husband',
'wife',
'old',
'child',
'year',
'kid',
'name',
'mother',
'children',
'mom',
'two',
'told',
'school',
'think',
'house',
'baby',
'parents'],
['room',
'house',
'dog',
'door',
'sleep',
'bed',
'bathroom',
'apartment',
'roommate',
'living',
'live',
'cat',
'use',
'stuff',
'home',
'dogs',
'girlfriend',
'bedroom',
'moved',
'place'],
['said',
'told',
'didn',
'got',
'asked',
'like',
'really',
'went',
'back',
'one',
'friend',
'could',
'friends',
'get',
'know',
'started',
'wasn',
'would',
'left',
'aita'],
['wedding',
'dress',
'hair',
'wear',
'ring',
'married',
'fiancé',
'party',
'wearing',
'one',
'would',
'think',
'tattoo',
'family',
'husband',
'getting',
'makeup',
'engaged',
'fiance',
'ceremony'],
['people',
'like',
'girls',
'gay',
'think',
'girl',
'women',
'said',
'always',
'group',
'girlfriend',
'friends',
'woman',
'one',
'white',
'doesn',
'really',
'look',
'know',
'thing'],
['car',
'seat',
'back',
'get',
'minutes',
'around',
'front',
'woman',
'guy',
'next',
'move',
'people',
'away',
'flight',
'door',
'phone',
'lady',
'sit',
'park',
'drive'],
['food',
'eat',
'eating',
'vegan',
'dinner',
'make',
'meat',
'cook',
'weight',
'cooking',
'meal',
'ate',
'chicken',
'lunch',
'fridge',
'eats',
'diet',
'cake',
'family',
'hungry'],
['phone',
'facebook',
'post',
'pictures',
'social',
'account',
'game',
'sent',
'picture',
'video',
'posted',
'media',
'call',
'also',
'message',
'messages',
'number',
'instagram',
'photos',
'sarah']],
[[0.011076196974682066,
0.000564392649472897,
0.0013542299952905628,
0.1530219806345308,
0.1521519084626053,
0.000814322382504041,
0.08234356736699737,
0.006933273680468054,
0.000988281118714402,
0.33177055578329395,
0.2551412694103212,
0.0016105767704565042,
0.0009311983390601434,
0.0004891183939972939,
0.000809128037605374],
[0.030659743069425077,
0.0003286990259242552,
0.0007886957435486457,
0.1799029812385095,
0.08861239449323562,
0.0004742566618601059,
0.07519157752888074,
0.0010117699042429768,
0.01873234157261896,
0.3747889828354061,
0.0003124971959932035,
0.0009379906278363181,
0.19421456289706449,
0.0002848597334120084,
0.033758647472042005],
[0.0012253645477922296,
0.0010108426229672714,
0.002425462843676183,
0.14377969582205702,
0.24458960704166374,
0.0014584735889811961,
0.11025476175579381,
0.0589486977385588,
0.0017700384283942886,
0.3336364238362757,
0.000961017406058512,
0.08664041489008946,
0.0016678016137124127,
0.0008760243791118095,
0.010755373484867579],
[0.004361723196766212,
0.0004186476183287587,
0.08579757833138776,
0.12121505436523619,
0.004942769683897052,
0.0006040371473751598,
0.05722547843376467,
0.0783732371932396,
0.008441533541657158,
0.35016052290363936,
0.2740483284306971,
0.001194672059809699,
0.008399191442106147,
0.0042170414962901135,
0.0006001841558051314],
[0.03221035460555476,
0.004391354449543325,
0.004993474374362835,
0.11665387052572497,
0.04072970956899532,
0.0006207857026874088,
0.13011198540142804,
0.12411843178271961,
0.03244218997114491,
0.30045318515488134,
0.004370146809909712,
0.04479988325145667,
0.0007098842272727663,
0.00037287161993664124,
0.16302187255438164],
[0.015572862094219599,
0.012846554761838653,
0.14909490922441876,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.1472027094161871,
0.0004425259734907111,
0.005135880480814765,
0.21775817055604668,
0.18448346453001507,
0.0006384895433869057,
0.0034525584934437924,
0.0013621411276311538,
0.049663642908323286,
0.33754008314152567,
0.0004207135250284459,
0.05015156915850283,
0.0007301290190266942,
0.00038350533738880626,
0.0006344167891880765],
[0.0005134300219136186,
0.012121494110239269,
0.004915589828456542,
0.1070357563457792,
0.001101273561318268,
0.020107685098806897,
0.12028396142976884,
0.0831893597295936,
0.0007416493897394194,
0.41664574516094505,
0.0004026680784432595,
0.17667788487309052,
0.0006988119744599671,
0.00036705584225903674,
0.05519763455518659],
[0.0005094569542093847,
0.0004202674255671795,
0.02809240581821036,
0.11394576792686122,
0.0010927515929228624,
0.058643509811888546,
0.04970860721587741,
0.02837762358836332,
0.016212479777928317,
0.3012164930016918,
0.042960118194644334,
0.02054500626902629,
0.3334396482388346,
0.00036421545963572546,
0.0044716487243387166],
[0.0005408461134097952,
0.00044616135246105626,
0.005178072065066604,
0.322235361439273,
0.0011600792708225355,
0.0006437347755266249,
0.11027674691369722,
0.001373331203483586,
0.20615783929029227,
0.3279904011143188,
0.00042416971334969546,
0.001273186514424549,
0.0007361270752774289,
0.000386655858086068,
0.021177287300510782],
[0.0011210384341754434,
0.0009247806567365862,
0.010732845546902545,
0.1570801109779233,
0.002404553563458652,
0.0013343008424909545,
0.2456038239149933,
0.01136045516104057,
0.0697304097426151,
0.2626615874702891,
0.000879197500894119,
0.2325138588906044,
0.001525806922454279,
0.0008014406814922261,
0.0013257896939294137],
[0.0017450326536059197,
0.001439533555881558,
0.02995987483220643,
0.1252382358959597,
0.0037429800421304197,
0.0020770015272430957,
0.18351878752973905,
0.004431034512175474,
0.26757860881481654,
0.36910601697109996,
0.001368577830391183,
0.004107919030413992,
0.0023751040299872317,
0.0012475398848932268,
0.002063752889456199],
[0.0006536033703561519,
0.0005391784399682883,
0.0012937298472986084,
0.25539114883689656,
0.05104076182938633,
0.0007779425763957847,
0.24743688046881915,
0.16546777222768536,
0.01583577741794099,
0.18788777832897693,
0.0005126019164684316,
0.0015386243448686217,
0.0008895971062422918,
0.00046726705757339917,
0.0702673362311231],
[0.0004134704029152257,
0.0003410850324951858,
0.0008184153042762446,
0.1835419098606515,
0.18929640853608853,
0.016192922705433332,
0.0466233493673081,
0.004190054354550954,
0.0005972577740087449,
0.4799763587942305,
0.0003242726866935049,
0.07319699358323313,
0.003702919398606435,
0.00029559379178018783,
0.0004889884077284531],
[0.0013511007255191903,
0.0011145664396260758,
0.3925970608803931,
0.24062210225758257,
0.023420270347666323,
0.0016081293748651795,
0.02921802226973235,
0.0034307518153484633,
0.032735036765312024,
0.26526002989354697,
0.001059628595344661,
0.003180577951305109,
0.00183893680812691,
0.0009659143283710803,
0.0015978715472600468],
[0.0007138416111568596,
0.0005888709020552902,
0.001412964253984992,
0.09460224711788633,
0.06116621700807879,
0.0008496403282303828,
0.17807818302613482,
0.0018126060900504517,
0.028137995547406383,
0.3461597642030865,
0.0005598449985570444,
0.2185352428363556,
0.0009715853075458372,
0.06556677606987824,
0.0008442206995925524],
[0.2452885300944724,
0.005318266262956612,
0.0012502859392524138,
0.23242344241657217,
0.0013548586417752339,
0.14466762778678352,
0.03284853614236874,
0.0016039159528641873,
0.04888436177076141,
0.1911728272131794,
0.0004953885619417059,
0.04466169943213384,
0.024845692252359563,
0.0244375441968153,
0.0007470233357634649],
[0.0007177327059646266,
0.0005920807912990945,
0.0014206662116550293,
0.24229280546375553,
0.07240147289621228,
0.0063051934621045766,
0.053677671627847666,
0.007273408274998879,
0.001036764506661912,
0.25538098322690944,
0.15318870737444434,
0.18157000868183423,
0.000976881343089867,
0.0005131137090947655,
0.02265250972412772],
[0.0006806319884541681,
0.005730630418778736,
0.006516384972771135,
0.064355347824632,
0.001459910760727126,
0.000810113023724003,
0.30419159439511245,
0.0017282793102461444,
0.07852050057781984,
0.3403938926938406,
0.0005337996673750508,
0.1721843731318664,
0.021603005640345698,
0.0004865900651063213,
0.0008049455292002927],
[0.000597272362830001,
0.05492553503638454,
0.0057182981637939985,
0.10637025711704953,
0.010353247499954355,
0.34545212577823786,
0.16714256213520073,
0.028733023203173672,
0.000862759605973368,
0.257879919963898,
0.00046842316261841974,
0.00594208635376004,
0.0008129269059958175,
0.0004269955024825086,
0.014314567208647192],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.1294034699905887,
0.01841715630801397],
[0.0004241249615360593,
0.0003498743205501134,
0.004060581302103782,
0.15283968794548608,
0.000909720092124594,
0.0037258855732349937,
0.0027296964006984455,
0.007519102812782336,
0.345267841367953,
0.32806977850798813,
0.0003326287439231188,
0.11051502083223826,
0.007019415064890016,
0.0003032108336779176,
0.03593343124081319],
[0.0006702382912044729,
0.0005529011211519951,
0.0115070943060832,
0.21098894489084802,
0.016708273393238816,
0.0007977420660707404,
0.07048654264645433,
0.08314538830084492,
0.0009681588501566421,
0.3351958545082709,
0.0005256481960531033,
0.0066680028271576,
0.260513397604484,
0.0004791595154007293,
0.000792653482580496],
[0.11610564569407086,
0.000501814235903712,
0.0012040764367843796,
0.07599668005127136,
0.010544571100772969,
0.0007240323993184447,
0.11017267072428444,
0.0015446365865564183,
0.019358276794557327,
0.36428308663923753,
0.00047707942300236154,
0.02915136077532713,
0.07012635104425038,
0.0004348861973653139,
0.19937483189729735],
[0.024482109309326927,
0.000433691540074929,
0.00902607945701877,
0.20941819287617428,
0.14087320237288886,
0.04454577179417828,
0.07126004102116607,
0.1450732239978234,
0.04867217534993833,
0.27091060283230595,
0.00041231454768782933,
0.029186711342299162,
0.0007155529792235594,
0.0043685790651022734,
0.0006217515147913545],
[0.0002230004944772756,
0.01881365450957335,
0.13762369673362992,
0.2531095923749623,
0.0004783213646397213,
0.0002654233241126874,
0.1030517599644691,
0.0005662489382185235,
0.00032212409399870614,
0.20128702370707743,
0.20340792069859726,
0.08012456011679099,
0.0003035183164209908,
0.0001594251033849934,
0.00026373025964680297],
[0.0008155314678010957,
0.0006727581351277902,
0.044969924861548015,
0.4363431672717756,
0.0017492612538803401,
0.0009706753054950015,
0.11673484296057644,
0.22504287636528397,
0.050727380680390594,
0.06720731847396133,
0.0006395973648473705,
0.0019198134943543225,
0.0011099918799532165,
0.05013237685792831,
0.0009644836270766143],
[0.004953582842680283,
0.000475455586182045,
0.0011408302656625978,
0.16830378888306716,
0.07564908420239413,
0.05321270912613859,
0.126271791280899,
0.0014635019119711315,
0.0008325476581770538,
0.32326236716813356,
0.0004520200115695875,
0.0013567818843915594,
0.0007844599899787468,
0.1273515868227549,
0.11448949236599966],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.3367685494340417,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.023291561755824756,
0.0008078549438343119,
0.0019384047574076964,
0.19671887360698123,
0.18803605699870082,
0.0011655969708237956,
0.32611193568543556,
0.039673767013164524,
0.008852017398117142,
0.16995229728601635,
0.01564287722296252,
0.024617595062533033,
0.0013328897578708844,
0.0007001096011438889,
0.0011581619391835224],
[0.09009517562247414,
0.0009658983899677301,
0.0023176215588895186,
0.27966581135376223,
0.0025114651767607576,
0.001393626722300377,
0.043105592974436543,
0.002973136043765749,
0.0016913387201149014,
0.32769466155433685,
0.0009182885091628505,
0.06500333736994042,
0.001593647573686215,
0.0008370744546492807,
0.1792333239757524],
[0.00048327871601241864,
0.0003986721549912839,
0.030319217046357698,
0.24756317749683746,
0.14050906746582834,
0.007915872088878784,
0.03614336647509688,
0.15905126129126568,
0.10713760972860366,
0.26738696580634724,
0.0003790212952564482,
0.0011376691607455655,
0.000657774067228066,
0.0003455003965109951,
0.000571546810039548],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.00035257723122304404,
0.0002908522968533528,
0.07835110586872526,
0.1993542343791459,
0.030210925084832873,
0.005775044857843351,
0.13079868024529195,
0.0008952737225451313,
0.0005092976203416843,
0.21381673016664735,
0.31088940127616177,
0.027606962336523804,
0.00047988076385228694,
0.00025206070359030724,
0.0004169734464220202],
[0.0009441870585218019,
0.0007788902694529082,
0.00186890556940843,
0.3976178277225915,
0.1741234875145481,
0.0011238058832312363,
0.041930656509194,
0.07410511740679226,
0.0013638776967942196,
0.178200382975354,
0.0007404981639541379,
0.11695485602247725,
0.0012851005871568951,
0.007845769194750647,
0.0011166374257725283],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.1294034699905887,
0.01841715630801397],
[0.0008990585208941215,
0.028053766975273068,
0.00860760518838118,
0.289848872948239,
0.029240525966061182,
0.014726144595268168,
0.1764870546080013,
0.002282913919298463,
0.0012986895485307885,
0.2789315035750307,
0.0007051051780477748,
0.0021164415461252823,
0.0012236776840579484,
0.16451537332799282,
0.001063266418798185],
[0.013188473804799861,
0.0004574349232795624,
0.013731552281540533,
0.20824928403736248,
0.0011893920648208464,
0.0006600006164386703,
0.13411981401496453,
0.0014080324309528297,
0.14398589104336693,
0.42892708937936924,
0.00043488760111862906,
0.0013053572935750918,
0.0007547274777300018,
0.029875669659666013,
0.021712393371014722],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.15781332392994726,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.15492980606133822,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.15781332392994726,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0003116822819780025,
0.0002571167380472395,
0.05979480919926624,
0.17386429543310794,
0.09772024693474243,
0.002738090476092073,
0.08012079800724563,
0.0007914321519567535,
0.00045022488821361956,
0.2837010151597289,
0.22748746915935986,
0.029139098363579908,
0.024095368584287992,
0.00022282452845716563,
0.01930552809393635],
[0.000547593891289293,
0.00045172781144112184,
0.026036568902264016,
0.1515869729592654,
0.07603256930117315,
0.0006517662269337108,
0.14908160491595582,
0.022184358814987298,
0.09228413034799854,
0.35703519175409165,
0.00458824049122067,
0.06782953032081673,
0.0007453112440675867,
0.02118537337637686,
0.02975905964211812],
[0.0011813876153939375,
0.0009745646371420428,
0.0023384157557682643,
0.27320281877425817,
0.09225613979415592,
0.0014061306396581708,
0.13321448679850423,
0.020944239899776652,
0.001706513773263599,
0.2588570952529878,
0.0009265275902922873,
0.011753276502987091,
0.0016079461209513887,
0.19823329553684776,
0.0013971613080127504],
[0.00221463864662537,
0.001826926641963742,
0.004383612827086564,
0.24303803667660873,
0.004750253949529512,
0.00263594371247293,
0.014253561310436988,
0.005623470858792709,
0.003199044330600865,
0.350700502180031,
0.0017368760107932535,
0.3584204689737789,
0.0030142686233957717,
0.0015832655260530385,
0.0026191297318305783],
[0.20098435213005011,
0.00044433622730088746,
0.0174290768209666,
0.08365490491096247,
0.02569970739135385,
0.0006411014310452877,
0.25709187677508366,
0.04636573171014975,
0.0007780560292774427,
0.28165066285330786,
0.00042243455002426226,
0.074901099324654,
0.000733115778940892,
0.0003850741537743277,
0.008818469913108593],
[0.03945330002914502,
0.0004692929823470593,
0.022728495244201098,
0.014905161469763336,
0.10059150296495752,
0.004997600137666823,
0.15055806528915527,
0.023046984591294367,
0.005142246979183504,
0.3536363342487985,
0.13870185279635017,
0.07910802253658594,
0.0007742922344971972,
0.0004067023729918666,
0.06548014612306231],
[0.0005386336585570413,
0.053623812556472514,
0.08288073999533167,
0.34955228655682063,
0.0052460626493647635,
0.004731830379443106,
0.015738868819612412,
0.0013677132777737578,
0.03350388761645998,
0.2611970181113188,
0.00042243455002426226,
0.02581235194388019,
0.000733115778940892,
0.16401423208968705,
0.0006370120163129578],
[0.0008929613573754144,
0.0007366325411683359,
0.0017675104090900736,
0.09121330711323868,
0.0019153432642898065,
0.0010628351848919876,
0.03965576059759938,
0.002267431835383333,
0.0012898821991164777,
0.6229076088887164,
0.0007003233518723565,
0.09704617413665258,
0.13684978719463858,
0.0006383862827393256,
0.0010560556432271852],
[0.0008580471424749677,
0.0007078306824623708,
0.0016984018886014283,
0.2049449964592443,
0.008357014074447035,
0.05967031451461502,
0.07720460285820024,
0.0021787767085754152,
0.0012394486345235138,
0.15542627589441416,
0.37863339217233694,
0.04763581462687174,
0.007684418005224955,
0.0201631042902493,
0.033597562047758635],
[0.15841655425111667,
0.0005041433243405023,
0.01977500862822523,
0.14132810901076814,
0.3633350416936834,
0.0007273928768985143,
0.2174347451903057,
0.0015518057638244029,
0.0008827813917083186,
0.05036300445335801,
0.0004792937088636312,
0.04321066978429568,
0.0008317922402295195,
0.0004369046502929779,
0.000722753032089354],
[0.06851269875405684,
0.00046132045994059205,
0.0011069137857770575,
0.16754726910613832,
0.052164602320902746,
0.0006656067834822551,
0.28390728221067635,
0.03539673074519854,
0.0008077963110641547,
0.33488735283555654,
0.00043858161666396377,
0.05228155257971219,
0.0007611382722158748,
0.00039979316296016165,
0.0006613610556543307],
[0.00048685253304347586,
0.06325860895498761,
0.012056076231425248,
0.1310748548547357,
0.0010442665991818717,
0.044949108688756886,
0.0955701632190042,
0.004933699151668733,
0.0007032582214008764,
0.40986813330017896,
0.00038182413493309986,
0.16753222856473204,
0.0006626382668837922,
0.000348055351158028,
0.06713023192790954],
[0.0022525247252984113,
0.0018581800866703001,
0.004458603797144915,
0.26430283169085317,
0.004831517091542784,
0.002681037105483402,
0.01449739862719342,
0.14257659461370623,
0.03746800134844864,
0.5106640137955503,
0.0017665889489696467,
0.005302587986769581,
0.003065834064277816,
0.0016103506319558732,
0.002663935486135569],
[0.0006601572565511275,
0.020599212856469783,
0.01634767341140283,
0.10252866980316654,
0.0014159938101057778,
0.005799400227098203,
0.024303443821373623,
0.02674457419378117,
0.3468959281624166,
0.45048195083521764,
0.0005177419368175157,
0.001554052614229585,
0.000898517375717822,
0.0004719524910593503,
0.0007807312045924258],
[0.07160693987631493,
0.0006987241277568265,
0.07886920416794369,
0.17014468305653435,
0.02754766063587903,
0.0010081398064971086,
0.06334588934373789,
0.00858346686373179,
0.0012235025798035693,
0.34640828055799433,
0.0006642834735601595,
0.1885428225884081,
0.020450996731599804,
0.019903697038201405,
0.0010017091520369934],
[0.00045177371860279385,
0.00037268266943329135,
0.045497998346775384,
0.13535485400923863,
0.1553666770901779,
0.0005377175616555535,
0.12642576742515443,
0.0011471561490189182,
0.0006525868928608183,
0.25681809534079475,
0.038095961176011946,
0.12801268509939567,
0.0006148936969623784,
0.000322977184260068,
0.11032817363965755],
[0.0008524918287494637,
0.0007032479255123578,
0.0016874058082509311,
0.08707946692766058,
0.0018285387923801554,
0.1952457335793645,
0.05080727621876472,
0.002164670504434877,
0.001231423986843242,
0.4716640644653713,
0.007142953179944515,
0.1314941983622714,
0.027057772918739908,
0.0006094542447173579,
0.02043130125699463],
[0.020407831560340615,
0.000536515241314821,
0.0012873396444791508,
0.4615829729915787,
0.001395011482945514,
0.060046469759836754,
0.11285186773209323,
0.10537809692148752,
0.0849086567594011,
0.14744481902357576,
0.0005100699889422053,
0.001531024518948863,
0.0008852030621933268,
0.00046495905542366135,
0.0007691622574387928],
[0.0006471783358377201,
0.0005338782223661319,
0.02585644596120861,
0.2577956962788421,
0.08985971621039063,
0.020430642228108784,
0.004165282721271847,
0.021303679845225878,
0.07466114986530646,
0.36298393153280056,
0.0005075629507028033,
0.0015234994006363214,
0.05494680635840222,
0.0299531941846314,
0.0548313359042685],
[0.12795459726059202,
0.050111554751108255,
0.0008107773944083448,
0.23782435351826178,
0.02887626979354873,
0.0004875347480051661,
0.0555207940871772,
0.03214863018596583,
0.016145950366018298,
0.23596124601083796,
0.0034320996996088293,
0.19694801054233077,
0.013000921603018615,
0.00029283514500595085,
0.00048442489411236716],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.1294034699905887,
0.01841715630801397],
[0.0015230060179176567,
0.0012563766437674521,
0.0030146086026481365,
0.2596706147079238,
0.003266747540414607,
0.08277951864275233,
0.056068899991269044,
0.015433941468094844,
0.002199981371459674,
0.4725102411677391,
0.0011944488645340677,
0.04985198624035623,
0.04833964338067703,
0.001088811002108482,
0.0018011743583375669],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.15492980606133822,
0.021195687909900382,
0.011133182261280586,
0.13668744403732208],
[0.0009308374326541273,
0.0007678777337532055,
0.03718935947529413,
0.17991474082014794,
0.051482214005020344,
0.02231604340949995,
0.02719905823222267,
0.10133486590953023,
0.06496897438343549,
0.4513864996981169,
0.0007300284446805396,
0.023399377885033983,
0.022475057607743595,
0.0006654642371236012,
0.015239600725743319],
[0.0007177327059646266,
0.0005920807912990945,
0.3230250530534358,
0.3295075544377978,
0.05059778565270172,
0.017207037083859858,
0.13544149879101228,
0.06723354819465294,
0.001036764506661912,
0.053696876224436745,
0.0005628966698703897,
0.007140510733749713,
0.000976881343089867,
0.011414957330850047,
0.0008488224806171558],
[0.012604572938149376,
0.00043718264450247395,
0.02922308872610613,
0.20707908468596442,
0.06955952910632125,
0.016730261366688662,
0.03158496253096575,
0.03756952680976093,
0.0007655297307555983,
0.3817628619484679,
0.004440503906912164,
0.0012475644612665018,
0.0007213129951405835,
0.13319959568300097,
0.07307442246599724],
[0.001111574617060615,
0.0009169736496439852,
0.010642238762798576,
0.2908261912404295,
0.09524635883528139,
0.0013230366620984384,
0.05780622502775289,
0.0028225405873500233,
0.2886339920456536,
0.24356017684620432,
0.0008717753072363287,
0.0026167180961988138,
0.0015129260459147804,
0.0007946749116427698,
0.0013145973647339377],
[0.02183031083800161,
0.0007571722635348408,
0.09243741450848103,
0.19134806924309644,
0.0856185524934211,
0.0010924705029087703,
0.061673943587535376,
0.10689290924443123,
0.022238299129841894,
0.17323157398666425,
0.06345720305546139,
0.16946030792601335,
0.0012492677834208962,
0.007627003511719236,
0.0010855019254686186],
[0.0006736674057042582,
0.0005557299079825455,
0.001333444210113101,
0.17113832594148326,
0.006561233890632005,
0.0008018235232505978,
0.2550325914784584,
0.001710594651759105,
0.0009731122042021137,
0.2959807106135725,
0.23076011409157562,
0.027167164878358442,
0.0009169055758658381,
0.005597872721806652,
0.0007967089052355302],
[0.0007770301329568366,
0.0065422615918234615,
0.0015380383897177431,
0.17379151363137257,
0.0016666784279259633,
0.012727378504152325,
0.16433515754763073,
0.001973056108157838,
0.16635782409609345,
0.3059862954879679,
0.0006094018993359154,
0.025434236661434344,
0.06007023349506495,
0.030061828214752055,
0.048129065811613976],
[0.25870062616638073,
0.0006226257498162832,
0.06454739117271659,
0.09429284088614472,
0.018815301419403234,
0.0008983428194401181,
0.027786206526217234,
0.03630928919718887,
0.029750902133021683,
0.3602699627823618,
0.0005919360436911985,
0.099222969547406,
0.0010272778439380465,
0.0005395848211274864,
0.006624742891146038],
[0.04235740181557645,
0.0002685595410622216,
0.0006443942641130959,
0.32994970591779355,
0.0006982907749614885,
0.0003874856370404981,
0.19989221715934166,
0.015661424292152695,
0.02519487850014814,
0.20484585142773554,
0.017562553697744353,
0.14416915048426324,
0.007860484672449866,
0.002705202846740329,
0.007802398968876941],
[0.0010841182084065403,
0.0008943239751522991,
0.035079834386779724,
0.22600823374630793,
0.05172628982809162,
0.0012903570428435876,
0.1881141916978735,
0.09332119000458397,
0.018032984072604456,
0.13050878411651687,
0.1325860492949485,
0.002552083945437319,
0.0014755560707979734,
0.0007750460727089507,
0.11655095753694679],
[0.0005074933900312224,
0.0004186476183287587,
0.4596578695846818,
0.25996732740769585,
0.0010885398771620619,
0.11237670154268986,
0.022537410173149763,
0.08608169680670959,
0.0007330739281871779,
0.026405219137900234,
0.0003980121525127894,
0.02817428070695463,
0.0006907318286361662,
0.00036281168955512377,
0.0006001841558051314],
[0.0011920832414871007,
0.010036831358598926,
0.002359586470822459,
0.2032486966290543,
0.0025569399931996894,
0.001418860964036008,
0.2340084157462689,
0.0030269702824759995,
0.22805805248746933,
0.24309375624276078,
0.0009349158555336334,
0.03902001479496928,
0.0016225035703977446,
0.0008522312673031561,
0.028570141095622736],
[0.0009441870585218019,
0.007949651456833642,
0.00186890556940843,
0.19683651447593098,
0.03070826376693339,
0.0011238058832312363,
0.013247611759671063,
0.01673902790774639,
0.0013638776967942196,
0.4004939797841568,
0.0007404981639541379,
0.1456379007720002,
0.18055413027167527,
0.0006750080073699129,
0.0011166374257725283],
[0.03086753095048423,
0.0010706232473256631,
0.18984366410162548,
0.07343020961868142,
0.042210029272775465,
0.0015447268392681534,
0.2941933564953255,
0.249709649090339,
0.001874717435772456,
0.07738362305921427,
0.0010178513970756477,
0.032624878721547915,
0.0017664343974003334,
0.0009278319336673835,
0.0015348734394970294],
[0.02103812735010055,
0.00037268266943329135,
0.09696388242551977,
0.23142450428956152,
0.02155537848544251,
0.0005377175616555535,
0.07839094228499299,
0.19328645670966463,
0.062411647787354085,
0.25681809534079475,
0.0003543128515993926,
0.001063504371826167,
0.024632306267043092,
0.000322977184260068,
0.010827464420751734],
[0.1338431498235277,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.15167305065014264,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0004564722683726394,
0.0003765586542429141,
0.004370275098098064,
0.23036462921154743,
0.0009791029335607122,
0.0005433099470058398,
0.06187251230122935,
0.0011590868347538463,
0.02839331582166226,
0.3322906595641525,
0.08702656614921471,
0.2506800419457259,
0.0006212887317317253,
0.0003263362206809199,
0.0005398443180212272],
[0.011494267619584591,
0.0005856956291402618,
0.006797482926644837,
0.13183710177906197,
0.06622853792161569,
0.0008450589506952904,
0.23643147423828068,
0.3307232240569062,
0.001025583752966063,
0.18252909602422368,
0.005948963807606029,
0.023239918212250302,
0.0009663463859062685,
0.0005075801496774745,
0.0008396685454406551],
[0.000547593891289293,
0.00045172781144112184,
0.001083896738435293,
0.1432694155713225,
0.02196844627954425,
0.2959250534989069,
0.04927091626064091,
0.09704237530647346,
0.000790999080626543,
0.2988122900384913,
0.00042946179724921555,
0.07198830901478818,
0.0007453112440675867,
0.00039147990651959075,
0.017282723560203758],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.15167305065014264,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.15492980606133822,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.024673295196390863,
0.0006486528902976949,
0.06724561561694596,
0.02657353742447808,
0.17486722281155198,
0.006907641698874547,
0.208100116304779,
0.013940113593613123,
0.21611868670810397,
0.23200814047100102,
0.0006166802862312924,
0.001851025661263696,
0.018985458799037902,
0.0005621406661841139,
0.006901671871246795],
[0.07759208952473103,
0.0004186476183287587,
0.0010045225832179903,
0.20215388030667095,
0.1012985148522718,
0.0006040371473751598,
0.17670660244254938,
0.06681054777303463,
0.0007330739281871779,
0.3347436036766994,
0.0003980121525127894,
0.00890313167327968,
0.0006907318286361662,
0.00036281168955512377,
0.02757979280295006],
[0.0005521867361561326,
0.00045551659687680514,
0.18980767418989716,
0.1654393604156616,
0.08925125784213557,
0.0006572327984520742,
0.12097638205851302,
0.0014021276220919975,
0.059508869231148565,
0.2761565659600929,
0.004626723529697071,
0.026461841264409557,
0.004945222106707706,
0.059105999162122606,
0.0006530404860372068],
[0.20008920746731781,
0.0024340929325763747,
0.0005722376462479015,
0.15468014389232726,
0.029162971636204522,
0.00034409658999697023,
0.24118167121256553,
0.016103328034008574,
0.00041760385102312855,
0.2455806179009258,
0.00022673212244005267,
0.10606962646477323,
0.002589088779049786,
0.000206679780754311,
0.00034190168978877095],
[0.013982282518914185,
0.007271777151168214,
0.0017095422222106157,
0.1275776498023462,
0.10680138415240074,
0.007587281484027785,
0.005558671771031964,
0.08746401466724549,
0.09307782884755063,
0.464733032865513,
0.0006773551844835802,
0.00859245084267365,
0.007734822435851882,
0.06621048535922669,
0.0010214206953553845],
[0.0007462052513234392,
0.0006155687096372843,
0.0014770242162644412,
0.3085761966156826,
0.1432795749476936,
0.000888160713458837,
0.004802626521539199,
0.09823651415236515,
0.0010778930830762535,
0.3221835840120728,
0.0005852268504961548,
0.10943266565794901,
0.006682794901365848,
0.0005334689934994158,
0.000882495373575921],
[0.1573823557092318,
0.0005673405590777166,
0.08493176394931616,
0.12770546903399657,
0.0014751614375661895,
0.006041729501196612,
0.17679042866058783,
0.001746333447497024,
0.000993443062982884,
0.3230571368430542,
0.0005393759028840499,
0.10608206481373586,
0.0009360621311918643,
0.000491673134589513,
0.011259661813091735],
[0.0005453260011345062,
0.00044985695591751723,
0.3365453533052421,
0.287630478355203,
0.038443682288610385,
0.000649066901333483,
0.10704862642942765,
0.05108336526172067,
0.03392016218460877,
0.1236294374213945,
0.0004276831576454293,
0.001283732458078056,
0.009025334264166709,
0.00038985857100085026,
0.008928036444516394],
[0.0010016476683084978,
0.0008262913743883605,
0.024804103556093072,
0.07949382411952029,
0.0630056990893669,
0.001192197597298404,
0.15098259414080376,
0.13947218049472565,
0.01666118722931281,
0.1662237032507576,
0.2822502555161069,
0.04800086613118307,
0.024184769819264873,
0.0007160871255004884,
0.001184592887369288],
[0.01929897035253372,
0.03719716202516886,
0.002359586470822459,
0.022179825518588075,
0.0025569399931996894,
0.001418860964036008,
0.3788635126346419,
0.06640107517113918,
0.001721963599386564,
0.4603764015753202,
0.0009349158555336334,
0.002806240572876033,
0.0016225035703977446,
0.0008522312673031561,
0.0014098104290528048],
[0.0015590726154938214,
0.0012861291400030272,
0.003085998127076945,
0.1474139654849911,
0.027025299784719785,
0.0018556651056197576,
0.010034294825580943,
0.015799435595757545,
0.073295654860222,
0.43633746326313155,
0.0012227348371537676,
0.13391671187928164,
0.025803191976297685,
0.001114595344249979,
0.12024978716042042],
[0.08637395430793865,
0.0007733447985867396,
0.04457384430100683,
0.15983655874723812,
0.002010800050453078,
0.0011158047140947096,
0.14842773448234817,
0.037978973322054244,
0.001354167286574431,
0.2766075528942538,
0.007854933499070283,
0.0022068522141817496,
0.008395658512334878,
0.0006702021478206216,
0.2218196187220437],
[0.05110619600678134,
0.00019732814501991064,
0.0004734783366696588,
0.10436794188662474,
0.01867986200039496,
0.0002847108751997186,
0.09782348788270537,
0.10960809099420551,
0.05302920087327067,
0.3612482331030669,
0.00018760168770160017,
0.18041425036427547,
0.01849235651642022,
0.00017101006803108386,
0.003916251259632855],
[0.058773997476929164,
0.0004218998160214908,
0.004896496838076778,
0.14546171899180033,
0.01274950836771113,
0.000608729514251293,
0.1741951489060188,
0.28484311862629974,
0.05900133040175495,
0.19751385823353362,
0.00428527482718576,
0.020624806582058074,
0.000696097669407827,
0.03532316715762443,
0.0006048465913267184],
[0.030105690720706284,
0.018237168131939426,
0.0015290152662893917,
0.06717235179190943,
0.0016569006192112493,
0.0009194238488733797,
0.22790414383258273,
0.25422716555305985,
0.04804898540675816,
0.29245789521696197,
0.006472470584800415,
0.013551735395156467,
0.024517959854262084,
0.012285534687686287,
0.0009135590898028718],
[0.008939506134525987,
0.12626530757242466,
0.24432525027930121,
0.28705549169202454,
0.001184404159252077,
0.0006572327984520742,
0.1042017432617733,
0.0014021276220919975,
0.0007976334425595747,
0.09163553919595609,
0.00043306383051214264,
0.0012998830692999889,
0.000751562407522778,
0.1303982140482664,
0.0006530404860372068],
[0.0006055122992142174,
0.005098154743949864,
0.0011985393128756002,
0.41594716301954576,
0.0058974321529561245,
0.0007207028290465576,
0.24302682245292956,
0.0015375333463363554,
0.005473310364802582,
0.10508357907830371,
0.04186271907908163,
0.17157539735345134,
0.0008241420004942215,
0.0004328863087473949,
0.0007161056582651267],
[0.0013651082709869133,
0.001126121714362429,
0.023437081734066938,
0.32605680494071454,
0.06513310589587927,
0.001624801666509275,
0.15393101753760632,
0.17971392957162413,
0.022706911033502666,
0.20580507634375447,
0.011438120737788555,
0.0032135526136859785,
0.0018580019973208604,
0.0009759284513872484,
0.001614437490810425],
[0.0006316551368978868,
0.024507040769433046,
0.0012502859392524138,
0.22282905516333396,
0.02054363314825167,
0.10629007877383063,
0.14798118318122733,
0.01599549683272151,
0.02489839363766587,
0.26313073161246603,
0.0004953885619417059,
0.011081344045800086,
0.1543699201710755,
0.00045157606371975796,
0.005544216962382573],
[0.009473607832964262,
0.0009092973527507742,
0.0021818103954462215,
0.4055903268920126,
0.0023642948994154634,
0.04316865399116057,
0.09917900246273709,
0.08651229797826554,
0.00159222733651802,
0.2415212521403959,
0.00086447738097082,
0.061194182746724564,
0.043356953746651584,
0.0007880224188936876,
0.001303592425092926],
[0.0008105114289062284,
0.0006686169435999072,
0.03238202486387125,
0.2489909527346699,
0.12484935058396918,
0.03174241451773229,
0.0790830195379382,
0.002058072728080424,
0.04425958326585352,
0.30070424921243316,
0.0006356602958618589,
0.038841253094934906,
0.001103159277373973,
0.0005794420709738006,
0.09329168944380142],
[0.00046782507257221506,
0.00038592394753119254,
0.0009260038842387151,
0.15792877816187115,
0.2745816203536836,
0.0005568224687413416,
0.15934133401748227,
0.008293840584925752,
0.0006757730650124646,
0.34410793816024027,
0.0003669014568297405,
0.04728981189134158,
0.000636740643734833,
0.003887415644803945,
0.0005532706469909978],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.2897476887972528,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.019517420614099282,
0.0006769509446935538,
0.0016243076083653299,
0.21470089677961152,
0.05785058272511256,
0.0009767248149600291,
0.24210774278507868,
0.12672910255276365,
0.013649914284713799,
0.2795233132803534,
0.00064358350757764,
0.0019317782881833588,
0.03851052271764345,
0.0005866645485066018,
0.000970494548337137],
[0.04444649599401836,
0.0006769509446935538,
0.0078565764533451,
0.10875232641495544,
0.0079924319652744,
0.0009767248149600291,
0.26703681816499775,
0.08933548948288504,
0.19438571078912714,
0.27329104443537366,
0.00064358350757764,
0.0019317782881833588,
0.0011169096477648295,
0.0005866645485066018,
0.000970494548337137],
[0.0011813876153939375,
0.08172449172980577,
0.029255058119989512,
0.23731396228862983,
0.0025339985800851123,
0.0014061306396581708,
0.13321448679850423,
0.07477752462821914,
0.001706513773263599,
0.15119052579610276,
0.0009265275902922873,
0.002781062381580009,
0.10927451557783636,
0.0008445848658919542,
0.1718692296147473],
[0.0005763571955909842,
0.000475455586182045,
0.16747540485505594,
0.3784106199433535,
0.0012362482018760514,
0.0006860013610670012,
0.04748172963329161,
0.0014635019119711315,
0.11901764012958811,
0.2532267568147048,
0.0004520200115695875,
0.010111233178570158,
0.0007844599899787468,
0.00916649435134384,
0.0094360768358565],
[0.0006055122992142174,
0.005098154743949864,
0.0011985393128756002,
0.2595931250638894,
0.01509472850328885,
0.10189096268270656,
0.0038971173442786445,
0.0015375333463363554,
0.1158408665687953,
0.3028254506104573,
0.16142757163340707,
0.010622711222628603,
0.019218734701159673,
0.0004328863087473949,
0.0007161056582651267],
[0.000568886758462687,
0.0004692929823470593,
0.005446533790564504,
0.2438911507304482,
0.19132180059654963,
0.0006771097742576745,
0.17216051710620103,
0.023046984591294367,
0.0008217566157743553,
0.3190724113415253,
0.00044616116725742404,
0.03158262853908531,
0.009415272961315495,
0.0004067023729918666,
0.0006727906719250853],
[0.13507866725361325,
0.000561475216272618,
0.0013472297702650166,
0.08503196863465648,
0.17204203244342903,
0.000810113023724003,
0.12327116230739829,
0.0999422281578624,
0.000983172540228061,
0.3662396687063712,
0.0005337996673750508,
0.0016022514491644635,
0.0009263848303212256,
0.0004865900651063213,
0.01114325593421253],
[0.000503611322490915,
0.00041544517596819695,
0.4484922257619704,
0.08968984536065529,
0.02785344139620817,
0.01207365726814178,
0.1485816580847557,
0.04717574640347322,
0.004552213183445671,
0.18684260236635314,
0.004219714460746738,
0.005010280323022506,
0.02363392947815614,
0.00036003636378550264,
0.0005955930508267699],
[0.001202974301423786,
0.0009923721886718289,
0.0023811440238398645,
0.07719940732849633,
0.002580300599029284,
0.0014318239009042941,
0.007742422442754645,
0.0030546251589357054,
0.3215031991087537,
0.5559440451634701,
0.0009434573937954013,
0.02110419330681072,
0.001637327018138394,
0.0008600173861655749,
0.0014226906788103839],
[0.0004850590418268004,
0.10354791278436418,
0.012011663467064216,
0.4695061028205747,
0.0010404196787154377,
0.10740895594837187,
0.025224966181516553,
0.008599373148319902,
0.008068365522973815,
0.17995960302875053,
0.00038041755247570497,
0.07481884014882614,
0.0043440462069014715,
0.00034677316780095166,
0.004257501301517845],
[0.08899224360458421,
0.0004574349232795624,
0.005308911192675605,
0.07769834715995612,
0.157008252208822,
0.0006600006164386703,
0.2773047125256683,
0.10669104604176442,
0.0008009925326631522,
0.23520634433547594,
0.00043488760111862906,
0.04762988328233219,
0.0007547274777300018,
0.00039642584863876614,
0.0006557906488524014],
[0.0007420002169625285,
0.028788223994081933,
0.0014687008527256916,
0.22794415274334945,
0.06921423953268684,
0.0008831557281528752,
0.42741742492914453,
0.05823635549502064,
0.03488316789623637,
0.1456760373338302,
0.000581928965616761,
0.0017467162035811558,
0.0010099110190960863,
0.0005304627757809437,
0.0008775223137339408],
[0.06134666436726693,
0.0005986067080208719,
0.07307934939211684,
0.5150024503755753,
0.018089463287396555,
0.00086368743659881,
0.1038683241529153,
0.0073535758085241315,
0.006559193620676989,
0.15348675744794205,
0.0005691009190953646,
0.04579622671197359,
0.0009876485329493368,
0.0005187692503377225,
0.01188018198861026],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.2897476887972528,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.1295344810686041,
0.0005185848842359863,
0.0012443167110566373,
0.06421362031478715,
0.20186858825478732,
0.01507110087898478,
0.004045965104640582,
0.006370548823270239,
0.03432810233715834,
0.500588990628025,
0.0004930234330708048,
0.015802728921951618,
0.0008556195466281263,
0.00044942010843987575,
0.02461490898435943],
[0.0015968888465021569,
0.001317324965124682,
0.18507779925348544,
0.11460618896993324,
0.051936407581813865,
0.0019006753633914887,
0.07091666540195313,
0.004054863716353747,
0.0023067050775018106,
0.21649295258120052,
0.34083069669725935,
0.0037591789863913212,
0.002173470580582876,
0.0011416305154152563,
0.0018885514630910994],
[0.0007138416111568596,
0.0005888709020552902,
0.001412964253984992,
0.21929376505332632,
0.07743032804313618,
0.0008496403282303828,
0.03170118371061825,
0.0018126060900504517,
0.4076339196987456,
0.243153727647723,
0.0005598449985570444,
0.007101799380609472,
0.0009715853075458372,
0.005931702274667787,
0.0008442206995925524],
[0.0005430768186838288,
0.004572474684816696,
0.0423196872508015,
0.06784707712226769,
0.038285122353153296,
0.03364217505701341,
0.13135394615479473,
0.0838684585078815,
0.13689208818227314,
0.4035837054508232,
0.0004259191899442283,
0.034274222952708794,
0.0007391632116357502,
0.012761670065299827,
0.008891212997902373],
[0.0007138416111568596,
0.2445505364279162,
0.06646940839421457,
0.21387239470830718,
0.0015311432128683495,
0.02795649205332604,
0.05880803543571391,
0.0018126060900504517,
0.0010311438223107267,
0.2973674310979143,
0.0005598449985570444,
0.07757961386585817,
0.0009715853075458372,
0.0005103319296486564,
0.006265591044611684],
[0.0006503749853043746,
0.000536515241314821,
0.0012873396444791508,
0.16522112436603506,
0.001395011482945514,
0.18846993749757232,
0.0041858565693939165,
0.0016514499025472706,
0.07502992847188299,
0.3203225640551428,
0.0005100699889422053,
0.1447725846879616,
0.005824567205952387,
0.08937351364308675,
0.0007691622574387928],
[0.0012605575618978842,
0.0010398744721037425,
0.012068604610136568,
0.023453854400430643,
0.05057121987907101,
0.0015003615982941954,
0.03683347631752244,
0.04149476772950075,
0.18371702124659925,
0.47724734967355253,
0.000988618252833566,
0.002967433524382627,
0.1165974783715992,
0.04876859116953434,
0.0014907911925412668],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.15781332392994726,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.00042549551517101345,
0.00035100493432033424,
0.004073703011430839,
0.2987504316533972,
0.0913942519619433,
0.0005064402852399924,
0.07059971146679839,
0.09479350737363387,
0.0006146280421765722,
0.17401863043663984,
0.07465786858670789,
0.004233129224838091,
0.0005791273365205406,
0.00030419065507006034,
0.18469787951611216],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.13668744403732208],
[0.0008006545027086768,
0.0006604856481826538,
0.007665483048602984,
0.2155594674126072,
0.032120766339846674,
0.0009529681988951073,
0.10852467718244103,
0.0810819232609834,
0.0011565449973354157,
0.4369029846565914,
0.0006279297983024605,
0.0018847921622940497,
0.0010897433535591867,
0.018814444381234814,
0.09215713505641494],
[0.000702417416305115,
0.0005794467163221277,
0.0013903514240465487,
0.4078301033890565,
0.0068412467609491765,
0.0008360428347361696,
0.20723589744967827,
0.1244795744057585,
0.15571826468090877,
0.08989332300059538,
0.0005508853382481907,
0.0016535357466070865,
0.0009560362281772253,
0.0005021646677347359,
0.0008307099408762157],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.020805889955187475,
0.0010601735761192402,
0.1391889075959477,
0.023911690651642302,
0.022277318682316357,
0.001529649745048118,
0.15467684486574335,
0.2960742058343141,
0.011616782211283148,
0.23279413399352966,
0.04004936758882557,
0.003025359979340845,
0.00174919335700012,
0.0009187759574723377,
0.05032170600622966],
[0.1453549213204616,
0.0004252029376739353,
0.0010202517216010602,
0.06830895064552542,
0.0011055845854139384,
0.008442656514388261,
0.23819224006920503,
0.024796302491589355,
0.004659133215371097,
0.4456788025587517,
0.0004042443550830512,
0.0012133786199857147,
0.0007015475302437939,
0.004283073291264603,
0.055413710143441486],
[0.01578024492033858,
0.010625169860963308,
0.021468968431001732,
0.07281178124062565,
0.20297994467584415,
0.0007897025485379661,
0.014348066150822661,
0.0016847360009259655,
0.0009584018994060287,
0.39732376918364526,
0.14664904224181832,
0.11241813204374924,
0.0009030449332472661,
0.00047433062209185103,
0.0007846652469819794],
[0.003923215002896769,
0.0003765586542429141,
0.13263975627549082,
0.14716280358296832,
0.007912588402608969,
0.03521073729224713,
0.04107205589408458,
0.07742742699428468,
0.02839331582166226,
0.23868860573200104,
0.00035799778611148475,
0.001074565059988656,
0.20515911006865534,
0.0003263362206809199,
0.08027492721207619],
[0.06652594095688368,
0.0004775459123974538,
0.0011458458916873034,
0.3141272410530006,
0.018827563283108265,
0.0006890173454628505,
0.09165518151540245,
0.15534638574395765,
0.005232677917248692,
0.23235771070987654,
0.018039887257099155,
0.05851685678284938,
0.031563198762732246,
0.0004138545920999484,
0.0050810922761937855],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.2897476887972528,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0008206140791599731,
0.0006769509446935538,
0.0016243076083653299,
0.02773283143021843,
0.03292150734519348,
0.05083487557479819,
0.042675139745726016,
0.1142645648628041,
0.02611445197467334,
0.5849044866843621,
0.013108121197537181,
0.0019317782881833588,
0.044742791562623216,
0.0005866645485066018,
0.05706091415315507],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.031053482128685188,
0.0005501009867621174,
0.0013199379145138396,
0.14408268796048193,
0.00649477594379728,
0.005858141652627337,
0.014420731066700294,
0.09285318290149815,
0.15796088646565212,
0.30311163709547506,
0.010651865488756877,
0.05221419045723892,
0.17816300796007162,
0.0004767328409267923,
0.0007886391368124761],
[0.00047456958566715447,
0.0003914877132854811,
0.07662724622902223,
0.2863520838277435,
0.0010179204865213968,
0.00416903538600858,
0.0210752881081355,
0.04445526425716712,
0.15206130028302584,
0.23373492206553223,
0.000372190980254671,
0.0011171673081242185,
0.15202170514142416,
0.0003392741591703931,
0.025790544468917584],
[0.0010579854763849024,
0.0008727662440839018,
0.026199213702967645,
0.148245118969367,
0.0826195016247951,
0.0012592529118075194,
0.29606995774411066,
0.23570202383115976,
0.025633317200179407,
0.1755729778388019,
0.0008297469189841413,
0.0024905658145493005,
0.0014399877064977917,
0.000756363542367268,
0.0012512204739437028],
[0.007097114046903529,
0.006952549950120626,
0.0016344942157434073,
0.027906753822631736,
0.1209269202525194,
0.032339618235043686,
0.11192766031074965,
0.03345356146695121,
0.001192810510537043,
0.6387434583999207,
0.0006476196473290857,
0.008215246759596784,
0.0011239141831126734,
0.0005903437293387891,
0.007247934469501637],
[0.0009374647228724892,
0.022132467194853282,
0.008975306973895925,
0.18831538860892683,
0.10168670456636361,
0.15774936895338268,
0.14130802701692596,
0.0522183882528986,
0.022713289682840972,
0.2552484304979873,
0.0007352260336481023,
0.044925097006714834,
0.0012759510469126957,
0.0006702021478206216,
0.001108687293956086],
[0.0008580471424749677,
0.0007078306824623708,
0.0016984018886014283,
0.11371316345605426,
0.08003916857695348,
0.0010212790125642818,
0.03810524585683309,
0.0021787767085754152,
0.053371924636346386,
0.3509230609012499,
0.0006729411591211052,
0.13886764763006176,
0.0011678585049970957,
0.20914332979685724,
0.007531324046847197],
[0.08287308373965306,
0.000685495327033656,
0.0016448093970881346,
0.16061243694861588,
0.09013542333132517,
0.0009890529021360681,
0.18836520842009002,
0.10308493320808369,
0.00751126994708898,
0.30198421342530524,
0.0006517067306850642,
0.020888956002519988,
0.001131007128748731,
0.0384596594936873,
0.0009827439979390295],
[0.19868782907167767,
0.0008943239751522991,
0.0021458825762345353,
0.4894798482306694,
0.00232536211227383,
0.0012903570428435876,
0.023444432645147544,
0.0027528225255847028,
0.07566739974105854,
0.07287436844806279,
0.033784193863312904,
0.002552083945437319,
0.06734345969188835,
0.0007750460727089507,
0.025982590057947515],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.13048362584298057,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.001817285539683112,
0.0014991372852458651,
0.01739872997876698,
0.08901882077161301,
0.0038979577212096147,
0.002162999548208462,
0.09450595149823833,
0.004614501011298432,
0.0026250679819323237,
0.45339696217833925,
0.042830134223477825,
0.2803106108201848,
0.002473444952459679,
0.0012991941373186374,
0.0021492023520236943],
[0.13259375823488956,
0.0006226257498162832,
0.0072260875674933205,
0.09429284088614472,
0.018815301419403234,
0.0008983428194401181,
0.37744615851807917,
0.07643420172084515,
0.024018771772499357,
0.171109660885125,
0.012056196764735853,
0.08202657846583902,
0.0010272778439380465,
0.0005395848211274864,
0.0008926125306237111],
[0.16546239926956102,
0.004810567355658863,
0.0011309295975834089,
0.02364831451153961,
0.00556475745089755,
0.031054713910309638,
0.3768517485344078,
0.040503942940083665,
0.000825322413307757,
0.3378138944708489,
0.004787335166118197,
0.005684245084742307,
0.0007776520727837444,
0.0004084671513831869,
0.0006757100707743243],
[0.04303014508387033,
0.00046132045994059205,
0.005354006064141475,
0.12932343860085857,
0.07764715599108926,
0.0006656067834822551,
0.16498869841647273,
0.026902546188469705,
0.005054888589428572,
0.24569841498990383,
0.00043858161666396377,
0.0013164452393391907,
0.0007611382722158748,
0.29769625264846933,
0.0006613610556543307],
[0.0008869463353128437,
0.0007316705560269427,
0.0017556043909643302,
0.11754304467848056,
0.03558263382793114,
0.0010556758861908749,
0.07980486873582462,
0.06961254310643016,
0.0012811935029912532,
0.34253410167259934,
0.14888845246092638,
0.15701681367475617,
0.0012071922041291914,
0.04105031695565663,
0.001048942011779589],
[0.0009115060958508604,
0.0076744917200389905,
0.4379255622506505,
0.28693930494412456,
0.01580024256161188,
0.0010849078091814146,
0.005866513727575649,
0.0023145211411531533,
0.0013166700638422876,
0.22049029503776452,
0.0007148674452997505,
0.002145743937654536,
0.008163180678657175,
0.0006516440867438675,
0.008000548499850827],
[0.0008636753341790245,
0.03350899152063854,
0.02138745299931336,
0.12101834620997862,
0.0018525266745194407,
0.0010279778916602042,
0.1957784759496918,
0.015311675151202103,
0.2111452935101671,
0.31386905024105866,
0.0006773551844835802,
0.08074479035871704,
0.0011755188434843004,
0.0006174494355508743,
0.0010214206953553845],
[0.030502125642813124,
0.0008018909432627509,
0.0019240944568155803,
0.32076933465418334,
0.0759101655671233,
0.0011569919346681604,
0.0431688711728307,
0.10582350241153657,
0.016169181425959647,
0.38279053302302707,
0.0007623651167122712,
0.0022883128029123297,
0.0013230496803440454,
0.0006949410320916781,
0.01591464013571939],
[0.0009178600379786612,
0.014698806130216808,
0.0018167943750482397,
0.26802705550984723,
0.0856185524934211,
0.0010924705029087703,
0.13835292985428618,
0.0023306552443164794,
0.14074218699663862,
0.3126479126534839,
0.0007198506553925402,
0.030043969259193688,
0.0012492677834208962,
0.0006561865783782531,
0.0010855019254686186],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.15167305065014264,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0010171224827424186,
0.0008390570464820244,
0.0020132725349401117,
0.15796874782122133,
0.009906340203169994,
0.0012106162859955127,
0.2537360120381104,
0.048930782195635784,
0.09416538696585763,
0.39280745863197114,
0.0007976992738773531,
0.0023943717010026125,
0.0013843704888617462,
0.0007271502125881397,
0.03210161211754381],
[0.08501928740042529,
0.03954946126628582,
0.0016874058082509311,
0.041758884696758224,
0.027726014352895796,
0.001014666875497194,
0.05080727621876472,
0.18344699942804435,
0.001231423986843242,
0.43281785112459786,
0.0006685842898156042,
0.0667505094609823,
0.05942961736938446,
0.0006094542447173579,
0.007482563476736809],
[0.00817355888471583,
0.0007845158452267454,
0.07410792769480692,
0.3860445385414031,
0.0020398462678562173,
0.001131922630094234,
0.056678607643802434,
0.3852100980844953,
0.0013737283749070063,
0.07837171117671836,
0.0007458464507348127,
0.0022387304256306097,
0.0012943822934685322,
0.0006798832880639103,
0.0011247023980760057],
[0.0005134300219136186,
0.00042354493688951246,
0.03221080456627264,
0.23961251364374309,
0.001101273561318268,
0.008409735925457141,
0.003304469696271277,
0.001303715516145306,
0.06703002803872138,
0.2996662534274475,
0.0004026680784432595,
0.009007280055077345,
0.0006988119744599671,
0.33570826547828536,
0.0006072050795543896],
[0.1904644719635068,
0.00047965469989025815,
0.0011509058145603534,
0.28460320056490174,
0.001247166459905023,
0.0006920599663349312,
0.2951905975792998,
0.0058923115132129816,
0.0008399005264276192,
0.20247258543725002,
0.00045601214770623553,
0.001368764668010853,
0.0007913881590721335,
0.00041568212609197563,
0.013935298373829334],
[0.0006111330711793428,
0.0005041433243405023,
0.010492336794111268,
0.2620028428542496,
0.015234847914409927,
0.0007273928768985143,
0.33810947903378713,
0.13615054735847681,
0.0008827813917083186,
0.05500434037041498,
0.0004792937088636312,
0.07105868528663756,
0.0008317922402295195,
0.0004369046502929779,
0.10747347912439988],
[0.06329882902210358,
0.0005235843719496182,
0.0012563127145999331,
0.32030951445701483,
0.025462977608770317,
0.015216395865332867,
0.0667490998852546,
0.001611647337198554,
0.049119999921323515,
0.3849070459054019,
0.000497776492157364,
0.0014941243965945882,
0.000863868262587545,
0.014914705674582088,
0.053774118085128705],
[0.012149378840606946,
0.0006190771190665769,
0.0014854424571634779,
0.21914354942466613,
0.0016096834226186313,
0.15477865114813602,
0.22140949072011182,
0.007605044289313348,
0.0010840364919227855,
0.192932269408215,
0.0005885623277035096,
0.013165547505004765,
0.006720883225672969,
0.0005365094788550334,
0.16617187414094298],
[0.14769060502449993,
0.0010601735761192402,
0.0025438298289958204,
0.18983785651151244,
0.012516955984676939,
0.001529649745048118,
0.037552492494070305,
0.003263324905131534,
0.011616782211283148,
0.32063739827228444,
0.14741335726285917,
0.003025359979340845,
0.09935282033339432,
0.010679138655111758,
0.011280255215671987],
[0.0010752650267627977,
0.0008870207008977187,
0.0021283587600249817,
0.183331337623463,
0.01047262384266902,
0.001279819663066185,
0.0640842356263456,
0.00273034228527234,
0.0913819831886593,
0.4234280605758641,
0.0008432987625656629,
0.17402251864008897,
0.0014635063092323021,
0.0007687168517709064,
0.042102912143317205],
[0.12307327925852915,
0.00030994062156941506,
0.009303981031313933,
0.09544693987986418,
0.07214167942950946,
0.0004471914820770706,
0.3048818956768794,
0.0038074612701672478,
0.05761135612011201,
0.32486597068456624,
0.00029466340793827617,
0.006591324168393322,
0.0005113748243926546,
0.0002686031775894616,
0.0004443389670982836],
[0.000432483333125964,
0.0003567694100782845,
0.04683982469570806,
0.18869730445304042,
0.06333420080188967,
0.003799312838656426,
0.06190548859095569,
0.001098173476044648,
0.0006247219414436687,
0.23928305214955017,
0.04632295953490092,
0.03386364754004685,
0.0005886382156157396,
0.3123419490780198,
0.0005114739409236968],
[0.0007061846342879855,
0.005945772774676712,
0.1247518304413419,
0.3832012934915038,
0.01224115622026404,
0.022293400151366383,
0.009908269533305095,
0.02860925508096801,
0.07610514035057828,
0.21909268113897137,
0.0005538398566080356,
0.10892677120163055,
0.006324382018315632,
0.0005048578865000159,
0.0008351652196821995],
[0.09131864878969533,
0.0004927091802653558,
0.0011822293424507326,
0.3422458358268994,
0.04210572924935721,
0.0007108953561496817,
0.07188511688699213,
0.01058874791780061,
0.000862759605973368,
0.4347866039962853,
0.00046842316261841974,
0.0014060175324167748,
0.0008129269059958175,
0.0004269955024825086,
0.0007063607446173949],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.2897476887972528,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0896285075175286,
0.08952051531450847,
0.005905822691005355,
0.15670677432215416,
0.0950195942152898,
0.0007342082915266417,
0.008654970170569034,
0.0015663456363453935,
0.08990270108698183,
0.36940289264018383,
0.0004837845218180923,
0.006136949738323148,
0.08048158699092361,
0.00044099829272373686,
0.005414348570118246],
[0.08768239887263198,
0.00029640813567902434,
0.01981314070220099,
0.27956998495262464,
0.0007707008507295658,
0.00042766641178822645,
0.14148372623635386,
0.0009123751742480472,
0.000519026185402125,
0.19607026967351857,
0.08214719176518197,
0.047236233690561805,
0.08508328768727527,
0.0002568755483021549,
0.05773071411350189],
[0.05577142191896359,
0.05992061235704243,
0.0011069137857770575,
0.11658216176576533,
0.0011994949805297435,
0.004912699061846672,
0.0035991918386248586,
0.11184439175575804,
0.0008077963110641547,
0.27542806093845473,
0.00043858161666396377,
0.0013164452393391907,
0.3660110742115557,
0.00039979316296016165,
0.0006613610556543307],
[0.08665800235847933,
0.0008455889501166258,
0.00202894548857724,
0.19812257878219622,
0.009983459226065979,
0.009004855415432517,
0.1856479675223245,
0.0026028110326240124,
0.0014806705834568565,
0.42700465073642624,
0.0008039092149155755,
0.07247634379476663,
0.0013951475566019486,
0.0007328109422565454,
0.0012122583957598296],
[0.02227854276018309,
0.0005856956291402618,
0.012189620496944085,
0.09409213878696723,
0.0015228870780247333,
0.0008450589506952904,
0.41976415162845504,
0.08807703339344009,
0.001025583752966063,
0.3550774982737996,
0.0005568262373067808,
0.0016713679310533123,
0.0009663463859062685,
0.0005075801496774745,
0.0008396685454406551],
[0.008060615677477359,
0.0004107323343014856,
0.2165229750457903,
0.3306793524588029,
0.12585279552063397,
0.04975027958262624,
0.13933341909714195,
0.005045635668340588,
0.0007192138508797903,
0.11665858943645183,
0.004171845699036158,
0.0011720846419937558,
0.0006776723046571248,
0.0003559520838974881,
0.0005888365979691852],
[0.000625652385624295,
0.00526772566300195,
0.08676729221596154,
0.25397269813203915,
0.04885803202825611,
0.014999488962362006,
0.1228168625080023,
0.0015886736030970767,
0.005655359422830549,
0.146591627729092,
0.29509018391323355,
0.001472825930230625,
0.000851553980607041,
0.014702099309367445,
0.0007399242162942886],
[0.0020116321619637278,
0.0016594600641159762,
0.17203563766130955,
0.12909403840359618,
0.0043148184183138425,
0.002394317988271609,
0.318499456168567,
0.0051079912556618555,
0.002905801572997215,
0.10466656138759226,
0.12379864721706155,
0.00473551140905459,
0.0027379634672503703,
0.0014381343240757961,
0.1246000285001685],
[0.007622984813226445,
0.31058944054005255,
0.021963719824705134,
0.22531966032509815,
0.00863847991627674,
0.0010556758861908749,
0.005708445478775018,
0.06287650462851656,
0.014753270458818454,
0.3021178708051177,
0.0006956059468271629,
0.002087928682743373,
0.03488738459369719,
0.0006340860881750212,
0.001048942011779589],
[0.0008929613573754144,
0.0007366325411683359,
0.0017675104090900736,
0.1251219091508644,
0.029042224894390376,
0.0010628351848919876,
0.11425468508037594,
0.11077495835578559,
0.1504877311646696,
0.3787656742178114,
0.0074820437593975,
0.069919292506552,
0.0012153790441357395,
0.0006383862827393256,
0.007837776050752327],
[0.0008929613573754144,
0.0007366325411683359,
0.0017675104090900736,
0.13868534996591467,
0.0019153432642898065,
0.0010628351848919876,
0.25667081363840394,
0.002267431835383333,
0.0012898821991164777,
0.45336459870058793,
0.12955301109485007,
0.008883808838825733,
0.0012153790441357395,
0.0006383862827393256,
0.0010560556432271852],
[0.0007216664533755098,
0.0005953258660469093,
0.0014284525950612533,
0.15044721066081237,
0.0015479269837603761,
0.2255716392655985,
0.0484910708527139,
0.16625639138042383,
0.0010424468026752943,
0.38283901038887874,
0.006046779000286249,
0.001698849217123081,
0.011943829847466114,
0.0005159259812789171,
0.0008534747044989415],
[0.001025040592803845,
0.0008455889501166258,
0.00981376019454774,
0.3304644287836947,
0.0021986445200954784,
0.001220040709462018,
0.06887574693276699,
0.0882357727982995,
0.009265485289427356,
0.09225761837969475,
0.0008039092149155755,
0.11140041732461912,
0.0013951475566019486,
0.273201325651224,
0.00899707310173033],
[0.0013373777266104862,
0.001103245896497221,
0.002647179559501883,
0.24833502984705502,
0.02318239293530754,
0.0015917957609165602,
0.03907816057723181,
0.00339590600220602,
0.02224564725139189,
0.30319342121370174,
0.0010488660505669882,
0.0031482731298137015,
0.34715495829782295,
0.0009561036303055868,
0.001581642121070617],
[0.0005498807235265207,
0.00045361429289770573,
0.0010884232498568206,
0.15639616870747627,
0.017884043364077385,
0.009006780823064851,
0.007715215193492469,
0.0013962721319232558,
0.12607869328315993,
0.6257995901705621,
0.0004312552925716248,
0.03470362546724183,
0.017453009222855097,
0.0003931147839071892,
0.000650313293386886],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.13680567632871515,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.13668744403732208],
[0.051311240941280686,
0.0005501009867621174,
0.0013199379145138396,
0.20992040410141732,
0.0014303362406484033,
0.0007937019494784597,
0.09038732661393344,
0.047273225573158266,
0.041478773293227966,
0.47023814729938795,
0.0005229860824591233,
0.08260082867613218,
0.0009076183498609309,
0.0004767328409267923,
0.0007886391368124761],
[0.04664457080632652,
0.0008262913743883605,
0.0019826419870840606,
0.08710097797585663,
0.009755622095012545,
0.001192197597298404,
0.06730390172110405,
0.0025434110806715907,
0.0014468795166401338,
0.6682958577689558,
0.008392716687998746,
0.0023579429931650433,
0.0013633082502558604,
0.0007160871255004884,
0.10007759301974169],
[0.0004978983173538798,
0.007973449694424966,
0.0009855302822711434,
0.19455043997658025,
0.0615696979595844,
0.1480656052642315,
0.13933341909714195,
0.001264276988278848,
0.0007192138508797903,
0.43807407724169967,
0.0003904870189744179,
0.004953443322055495,
0.0006776723046571248,
0.0003559520838974881,
0.0005888365979691852],
[0.0010171224827424186,
0.02401309556851051,
0.0020132725349401117,
0.0729972732404502,
0.05625441724722696,
0.0012106162859955127,
0.08379306287656817,
0.0025827051515788157,
0.28728237464942835,
0.4623295741980566,
0.0007976992738773531,
0.0023943717010026125,
0.0013843704888617462,
0.0007271502125881397,
0.0012028940881724994],
[0.01988928676078358,
0.000689848916422238,
0.001655255602124119,
0.14893046354896794,
0.052601799549703246,
0.000995334389477665,
0.011733168298546981,
0.002123426956158633,
0.1282282115581741,
0.4944324938467256,
0.0006558457282759823,
0.001968584532332045,
0.007489202666093502,
0.006948854787079789,
0.12165822285913454],
[0.06894588210093017,
0.0006727581351277902,
0.001614247185920422,
0.19479010593613613,
0.0017492612538803401,
0.0009706753054950015,
0.13531584767870256,
0.26839855404091156,
0.01356537124413837,
0.3025667115702254,
0.0006395973648473705,
0.0019198134943543225,
0.0011099918799532165,
0.000583030942925349,
0.007158151866451985],
[0.0009720689558830165,
0.28871994364083126,
0.20863449126532632,
0.29862179213898576,
0.0020850238497980298,
0.015922020278133213,
0.05055138534456322,
0.0024683040072811927,
0.0014041530824945925,
0.0505773952950634,
0.0007623651167122712,
0.07611345452023759,
0.0013230496803440454,
0.0006949410320916781,
0.0011496117922543388],
[0.01769680050745749,
0.0009017485106510523,
0.002163697352317997,
0.09505506120539893,
0.010646507959935906,
0.0013010693819252128,
0.04024274672072623,
0.060888563447638246,
0.0015790089182372733,
0.339138273824234,
0.15859228078609078,
0.21842113852664125,
0.001487805902774327,
0.05059252674799982,
0.0012927702079714852],
[0.0627104944185276,
0.000561475216272618,
0.0013472297702650166,
0.16773845187475436,
0.001459910760727126,
0.000810113023724003,
0.18530102473747173,
0.28086266024557655,
0.000983172540228061,
0.2887023406687795,
0.0005337996673750508,
0.0016022514491644635,
0.006095540032827345,
0.0004865900651063213,
0.0008049455292002927],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.15781332392994726,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0003725275515044189,
0.00030730995765200806,
0.009225012204758116,
0.06351548001566747,
0.0007990470479411675,
0.023077098248541806,
0.11839533667135596,
0.0009459321199757217,
0.000538115847228068,
0.33342547698409325,
0.00029216241148573535,
0.4478925755304209,
0.0005070344598028558,
0.00026632337094841045,
0.0004405675786241801],
[0.0004548952635228521,
0.00037525773222614516,
0.06654096391632722,
0.2226592414349798,
0.05279720960860885,
0.003996198888069042,
0.12384454434833364,
0.004609848410792603,
0.20448828699914326,
0.26895688601223505,
0.00035676098754993437,
0.021799448387818325,
0.004073908274344652,
0.0003252088054175354,
0.024721340930631167],
[0.0005430768186838288,
0.03756825989950492,
0.001074955732441215,
0.15033654015898823,
0.0671564344160055,
0.0460155945125215,
0.02824211735889402,
0.1457355557854219,
0.14101656133410917,
0.2839739840475784,
0.004550392341780257,
0.07551895447106907,
0.01723705581897986,
0.0003882506097917441,
0.0006422666942303172],
[0.0007504582185372428,
0.0006190771190665769,
0.0014854424571634779,
0.12225272413707367,
0.0757026674660717,
0.000893222750195016,
0.17581380823183299,
0.18428831393139375,
0.006783496802957638,
0.4152112215385742,
0.011987482949773214,
0.0017666268829350602,
0.001021422914638116,
0.0005365094788550334,
0.0008875251209322889],
[0.0005430768186838288,
0.0004480015329806687,
0.09593783822466985,
0.11734075494430003,
0.04240959550498932,
0.004770862994161216,
0.1396028924584668,
0.03025030753401313,
0.0007844741716842007,
0.33346766186961074,
0.21489852308541768,
0.0012784377380205727,
0.008988109515307806,
0.0003882506097917441,
0.008891212997902373],
[0.0007504582185372428,
0.15450450551700756,
0.024283283701302887,
0.11085380351500397,
0.0016096834226186313,
0.2972651589240073,
0.027627840144926842,
0.007605044289313348,
0.0010840364919227855,
0.3240198565620166,
0.0005885623277035096,
0.013165547505004765,
0.001021422914638116,
0.0005365094788550334,
0.035084286987141396],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.2897476887972528,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.003275885770782308,
0.00031442659563354956,
0.0007544498099359059,
0.12577571891332107,
0.0008175512598250403,
0.006243126541985874,
0.13561077455914172,
0.0038625691563864846,
0.05265574052986785,
0.39904151989216674,
0.00029892826486140956,
0.1601074826769581,
0.0005187762878976989,
0.0002724908476925577,
0.11045055889354376],
[0.0012727421350499791,
0.0010499259183504872,
0.021851278817607563,
0.15900482366891142,
0.09939013648279178,
0.0015148641217818857,
0.027523490545996905,
0.09022595117567225,
0.15649477689311153,
0.40453228076784176,
0.0009981742554987761,
0.0029961167927590286,
0.03073034216456962,
0.0009098950517504658,
0.0015052012083065678],
[0.0007547599428760237,
0.006354756110338609,
0.0072260875674933205,
0.21466757845711357,
0.001618910337836253,
0.0008983428194401181,
0.17682159589979773,
0.18534467857076936,
0.07560794501720029,
0.20550244304825896,
0.0005919360436911985,
0.1221514909894953,
0.0010272778439380465,
0.0005395848211274864,
0.0008926125306237111],
[0.0007099924789860977,
0.0005856956291402618,
0.0014053453563455897,
0.2288955780444484,
0.0554442627810172,
0.0008450589506952904,
0.23643147423828068,
0.1473905466667318,
0.06033909702625778,
0.1933133711648222,
0.0005568262373067808,
0.060984881204345025,
0.0009663463859062685,
0.0005075801496774745,
0.011623943686039149],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.14076527862529675,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.13668744403732208],
[0.00028349731259945485,
0.01315222692538367,
0.0027142092334505167,
0.205509326659664,
0.022138684511028645,
0.0003374288441088135,
0.17622247933149238,
0.056699427900594505,
0.0004095117151476687,
0.3463218164778355,
0.051895782111371046,
0.08033059629178375,
0.024069520062605536,
0.010967975563931044,
0.008947517059003483],
[0.0011404578859955405,
0.009602167931697528,
0.2274529564027811,
0.28972168129761433,
0.011107574552475002,
0.001357414582514798,
0.050646900367122896,
0.12415502739646081,
0.010308758345851397,
0.16327519403261723,
0.03553989778480775,
0.00268471116777439,
0.0015522380715693093,
0.07010626418313648,
0.0013487559975814203],
[0.015572862094219599,
0.012846554761838653,
0.14909490922441876,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.15167305065014264,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0009792986419858353,
0.0008078549438343119,
0.37380945665472304,
0.070282715961894,
0.0021005310500431377,
0.0011655969708237956,
0.20711319907829465,
0.06942345116494976,
0.001414596360170835,
0.26663877077931836,
0.0007680351470699035,
0.002305331948694112,
0.0013328897578708844,
0.0007001096011438889,
0.0011581619391835224],
[0.0006536033703561519,
0.0005391784399682883,
0.0012937298472986084,
0.15114961492305182,
0.060968526964038205,
0.0007779425763957847,
0.0588093429104334,
0.001659647505929337,
0.1746800195723711,
0.21270719116560666,
0.0005126019164684316,
0.3192271086537288,
0.015781244808220112,
0.00046726705757339917,
0.0007729802885599384],
[0.0014241685786064394,
0.01199089069640532,
0.0028189716903142697,
0.15629056946533845,
0.02468684421644235,
0.0016950973992978402,
0.0524302364305625,
0.0036162877009326905,
0.012873258978601042,
0.3228695492238471,
0.02274903003517471,
0.0033525843739862908,
0.2398914481224367,
0.14162677826215242,
0.0016842848259018947],
[0.012916496274191912,
0.0004480015329806687,
0.005199428884277243,
0.113216281792464,
0.030036176049481243,
0.04189112136068547,
0.06123790257358224,
0.26946975034050275,
0.14101656133410917,
0.24272925252921818,
0.0004259191899442283,
0.05489658871188893,
0.0007391632116357502,
0.025135089520807914,
0.0006422666942303172],
[0.04869524172460453,
0.007569688459397179,
0.00177957901642255,
0.11232019247731467,
0.1521449970613165,
0.0010700922513509085,
0.10137876671645638,
0.002282913919298463,
0.0012986895485307885,
0.22430729419936168,
0.0007051051780477748,
0.2547534099085946,
0.014879730027975208,
0.000642745200985689,
0.07617155431034311],
[0.0005195071906247365,
0.0043740284909763215,
0.0010283024673377029,
0.09252079433087336,
0.0011143086877105277,
0.004563806713309845,
0.1374895730314223,
0.08417402317440413,
0.0007504278566649518,
0.4886503305401259,
0.00040743422327865644,
0.009113893922959426,
0.0007070834001360781,
0.00037140046603365684,
0.17421508550414236],
[0.004482663709340991,
0.00043025575008421457,
0.0010323756749037248,
0.22756463411058006,
0.009040919973322434,
0.016465180500523854,
0.11822868930305071,
0.03301316169516007,
0.09581976916249069,
0.3479863695483907,
0.012292344208827934,
0.0012277975574064453,
0.016554279025109208,
0.004333970319395752,
0.11152758946141321],
[0.0018688725143226695,
0.001541693039653441,
0.017892624758962975,
0.06315895342343418,
0.004008608382164729,
0.0022244002474393933,
0.1397689199676326,
0.047325736208555735,
0.14463373248613487,
0.5372345379810977,
0.02985253122340348,
0.004399446022479689,
0.0025436582124282407,
0.0013360741396904192,
0.0022102113925999245],
[0.10334074381716458,
0.0006190771190665769,
0.0014854424571634779,
0.2476408509798404,
0.0016096834226186313,
0.000893222750195016,
0.07322352263320565,
0.001905583978278495,
0.14927000457882894,
0.22142957096338928,
0.0005885623277035096,
0.018865007816039617,
0.17770469255671853,
0.0005365094788550334,
0.0008875251209322889],
[0.054775254158621646,
0.08830017076890374,
0.10953222003758192,
0.06365473685517174,
0.00863847991627674,
0.0010556758861908749,
0.443550946543159,
0.056140466150602954,
0.0012811935029912532,
0.1606610627689321,
0.0006956059468271629,
0.008823967160656972,
0.0012071922041291914,
0.0006340860881750212,
0.001048942011779589],
[0.0005257299462949511,
0.000433691540074929,
0.060931568076921384,
0.153519974362433,
0.12090955290369557,
0.028574852218823624,
0.12715825953490734,
0.005327677713470216,
0.000759416623874378,
0.3507652007090792,
0.004405044441526493,
0.005230331979267185,
0.0007155529792235594,
0.0003758491712636114,
0.14036729779914453],
[0.0003460901584737539,
0.0002855009019194234,
0.04536835929414154,
0.16151671947459587,
0.0007423405821479584,
0.003040359435166937,
0.015369610741005941,
0.0008788015704764004,
0.15032045428196625,
0.588376677097917,
0.0002714283410255974,
0.0008147184783594269,
0.03201221506022413,
0.00024742303565084467,
0.00040930154692902447],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.13048362584298057,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0020116321619637278,
0.0016594600641159762,
0.003981785724728106,
0.06798354679029384,
0.03487006422496501,
0.002394317988271609,
0.13516798132865995,
0.23427233480554566,
0.002905801572997215,
0.5018847568740575,
0.0015776639904568628,
0.00473551140905459,
0.0027379634672503703,
0.0014381343240757961,
0.002379045273563803],
[0.04383421576721623,
0.00024886930679078736,
0.0005971485994334129,
0.12246360259224634,
0.09916808517770129,
0.00035907598553466824,
0.3181253095547222,
0.0030572315501634748,
0.00043578320369441325,
0.3547923554592326,
0.0002366023456327941,
0.046533901923118744,
0.0004106125147857002,
0.0002156770747571106,
0.009521528944970279],
[0.0006702382912044729,
0.026003995168076197,
0.001326656687313518,
0.18044763203453898,
0.2050463693404779,
0.0007977420660707404,
0.004313698124451412,
0.006792106160072329,
0.0009681588501566421,
0.554075263311819,
0.015796304624207624,
0.00157778401777276,
0.0009122383258571359,
0.0004791595154007293,
0.000792653482580496],
[0.0007420002169625285,
0.0006120998415598547,
0.0014687008527256916,
0.047616958167208165,
0.04667334021066917,
0.0008831557281528752,
0.34288905247157836,
0.12585905346107362,
0.0010718189132098732,
0.39362592987602446,
0.000581928965616761,
0.0017467162035811558,
0.029186035171618163,
0.006165687606285359,
0.0008775223137339408],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.13668744403732208],
[0.03514754210600998,
0.0006226257498162832,
0.18492212874368544,
0.18600692665450191,
0.001618910337836253,
0.006630473179962445,
0.022054076165694908,
0.0019165070340549102,
0.0010902503304100503,
0.48637683071385296,
0.0005919360436911985,
0.0017767534185264473,
0.006759408204460374,
0.0005395848211274864,
0.06394604649636931],
[0.00055451217150247,
0.0004574349232795624,
0.0010975906482431407,
0.07348702661552366,
0.009612033153685773,
0.055407167694060705,
0.310995276881128,
0.0856344433196021,
0.0008009925326631522,
0.3152214346796927,
0.00043488760111862906,
0.08974308872665683,
0.038656612377622177,
0.00039642584863876614,
0.017501072826582257],
[0.0007099924789860977,
0.022154245910337253,
0.0014053453563455897,
0.28820909131774014,
0.0015228870780247333,
0.0008450589506952904,
0.1393729979728942,
0.2282726102212205,
0.011809858893564559,
0.2795875722896101,
0.0005568262373067808,
0.023239918212250302,
0.0009663463859062685,
0.0005075801496774745,
0.0008396685454406551],
[0.001111574617060615,
0.0009169736496439852,
0.09506233379539544,
0.1388700201817552,
0.002384254299424837,
0.0013230366620984384,
0.007154168008194773,
0.0028225405873500233,
0.01848968794134363,
0.45461041442769645,
0.0008717753072363287,
0.01950073710271819,
0.0015129260459147804,
0.237170941002914,
0.01819861637125331],
[0.0051101611415470555,
0.0004904843096808544,
0.1276132936575134,
0.2187795784261855,
0.0193376681490724,
0.0007076852471646933,
0.22057484531272425,
0.001509761891271431,
0.0008588637409363245,
0.2883245417490449,
0.01852865121093203,
0.0013996685395941068,
0.0008092560647918323,
0.00042506736764914485,
0.09553047319189206],
[0.0005738453450292592,
0.00047338347987303634,
0.009852156447958057,
0.22422623347530968,
0.0883938413542415,
0.0006830116648901827,
0.13879592713022093,
0.0014571237525106589,
0.018261515472652635,
0.06472274791772938,
0.03095709335818788,
0.4110168790862626,
0.0051391902417366455,
0.00041024731210902973,
0.00503680396128849],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.1294034699905887,
0.01841715630801397],
[0.004536573151370678,
0.0004354300948312387,
0.0010447912385524337,
0.242327580602055,
0.005140912256422167,
0.012654458478124922,
0.0595194966130432,
0.1777246697503174,
0.20119924555816537,
0.07957729858698934,
0.0004139674077317548,
0.12952210547069148,
0.056840721139445646,
0.0003773558512515183,
0.028685393801007812],
[0.0005299619360240709,
0.02056153431471132,
0.041297699728231434,
0.2755018803646745,
0.17420615779140722,
0.00868052069860512,
0.3173507562479313,
0.0013456938033850113,
0.012840140732880905,
0.11209654956766944,
0.0004156335728703945,
0.029421656799558882,
0.0007213129951405835,
0.0003788746596225976,
0.004651626787287182],
[0.000523639196421812,
0.00043196681326645385,
0.24760126810243357,
0.1568862996377742,
0.0408916855715314,
0.0006232544751784704,
0.09086090742908595,
0.09677407253938294,
0.036548059140131754,
0.2738100830224265,
0.008364377634739574,
0.04100119432953253,
0.0007127073313560959,
0.0003743544749605442,
0.004596130301778131],
[0.25197755079478895,
0.0008018909432627509,
0.02407163697201316,
0.19526659373473043,
0.03161508053672814,
0.0011569919346681604,
0.19081915460748122,
0.00985081817901372,
0.00878666725422712,
0.27943533461877174,
0.0007623651167122712,
0.0022883128029123297,
0.0013230496803440454,
0.0006949410320916781,
0.0011496117922543388],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.13668744403732208],
[0.0006440129562954902,
0.0005312669989837355,
0.006165793588698536,
0.17827805631930696,
0.001381365349987198,
0.0007665277156997818,
0.05794642516134168,
0.001635295264954916,
0.010712370010367425,
0.3758817005918544,
0.03963345500591567,
0.3243251380910337,
0.0008765439229464634,
0.00046041078240365203,
0.0007616382402103712],
[0.00890217344849986,
0.00045361429289770573,
0.00944071597483016,
0.2816805595820764,
0.017884043364077385,
0.0006544880980915116,
0.40027297326723943,
0.009748564856896597,
0.0007943024085598318,
0.16642349029702846,
0.0004312552925716248,
0.0806412354545952,
0.02162915558534177,
0.0003931147839071892,
0.000650313293386886],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.14076527862529675,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.13668744403732208],
[0.0005945753282217145,
0.0004904843096808544,
0.0011768908844038651,
0.25941985074611357,
0.0012753248957710344,
0.0007076852471646933,
0.07607609928631334,
0.001509761891271431,
0.0008588637409363245,
0.4373388735887811,
0.000466307957630667,
0.18653868688593309,
0.018871599318093193,
0.013971824807625165,
0.0007031711120599107],
[0.0006139827714690162,
0.005169472499782963,
0.0898118945560619,
0.1000203136313478,
0.10390247663517053,
0.0007307846941470948,
0.1764818334089017,
0.03886286872875086,
0.010212854514295154,
0.4236361153357868,
0.0004815286450590355,
0.0480751385687091,
0.0008356708694507394,
0.00043894192722531095,
0.0007261232138419676],
[0.001111574617060615,
0.0009169736496439852,
0.0022002292595388892,
0.32459422925346826,
0.002384254299424837,
0.0013230366620984384,
0.03248019651797383,
0.0028225405873500233,
0.2379819350260955,
0.14225606280708808,
0.0008717753072363287,
0.0110587275994585,
0.237889192137186,
0.0007946749116427698,
0.0013145973647339377],
[0.015572862094219599,
0.012846554761838653,
0.14909490922441876,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0005386336585570413,
0.004535065175698704,
0.1810582347568793,
0.2922820812792512,
0.07887918372052549,
0.01291328827623874,
0.023920326716408046,
0.021821358019762847,
0.09895555079082506,
0.25710628916292094,
0.00042243455002426226,
0.0053587072018911,
0.01709603157253216,
0.0003850741537743277,
0.004727740964710776],
[0.0011306647827424405,
0.0009327217412510619,
0.002238015963605295,
0.20995091138611188,
0.0024252014467463405,
0.0013457584737473736,
0.0072770335814958285,
0.4236336447569521,
0.19054707850159502,
0.09317721159914438,
0.009473739610136374,
0.028422634914082607,
0.0015389090149728866,
0.0008083226465708725,
0.027098151580845536],
[0.18625563331804706,
0.009771435927714987,
0.055181500016827936,
0.27720082262521795,
0.011303380010001463,
0.0013813432252735521,
0.23663478041841685,
0.011760981686432335,
0.001676431174351433,
0.17496747682756553,
0.000910194667396204,
0.020360139561027633,
0.010393652098103282,
0.000829696437702287,
0.0013725320059215257],
[0.0013373777266104862,
0.001103245896497221,
0.37845258772175233,
0.08582458307418993,
0.002868587088699405,
0.0015917957609165602,
0.1711178985801847,
0.00339590600220602,
0.001931841404783755,
0.2828796153670936,
0.0010488660505669882,
0.0640896906696381,
0.0018202589054846744,
0.0009561036303055868,
0.001581642121070617],
[0.07275979103242126,
0.004708412738305009,
0.009601098342505892,
0.14631180771431623,
0.009693679537258576,
0.004912699061846672,
0.27966018993231195,
0.052385099858656206,
0.0008077963110641547,
0.38160536789756516,
0.00043858161666396377,
0.0013164452393391907,
0.02199659966403796,
0.00039979316296016165,
0.013402637890747582],
[0.00864932744700937,
0.00044073041000757305,
0.05380543049235479,
0.18035681795997055,
0.11069933337251743,
0.0006358988513662144,
0.20225763809552816,
0.13525518398183103,
0.0007717420541541937,
0.25096232253898837,
0.0004190064662620541,
0.05400560995859787,
0.0007271664968628483,
0.0003819492520499683,
0.0006318426224995765],
[0.040615194385448423,
0.0004354300948312387,
0.0010447912385524337,
0.25034505198740564,
0.13342045442203193,
0.0006282514000990055,
0.11965053200317279,
0.04543639189203233,
0.064902232007205,
0.2920402902987805,
0.0004139674077317548,
0.009260034690432321,
0.000718421441991373,
0.0003773558512515183,
0.04071160087903373],
[0.0009052395200287691,
0.0007467611924044905,
0.1324162176835717,
0.15434220210447352,
0.14631602050675485,
0.001077449102018444,
0.17770039757339992,
0.0022986088808963196,
0.0013076179984471096,
0.1845995879857091,
0.17945913733549604,
0.015880929290875666,
0.0012320904297585596,
0.000647164054084529,
0.0010705763420809937],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0005664394622246906,
0.00046727412897574913,
0.001121199296399799,
0.1783133939789581,
0.0012149753173964976,
0.009278004970011376,
0.01655135405776372,
0.005740222561397433,
0.0008182214976785011,
0.35641692870637587,
0.004746145853244297,
0.0013334349025744675,
0.22446997078611677,
0.00040495277841508844,
0.19855748170246762],
[0.07527660759376961,
0.025886868764625846,
0.04002971273677138,
0.30016345874402045,
0.05257401453447059,
0.0019240093574597675,
0.13317071555183868,
0.01638132971125089,
0.1496552522603365,
0.1945973971029913,
0.0012677682310516828,
0.003805329245325354,
0.002200153595795113,
0.0011556459544470605,
0.0019117366158458418],
[0.02550026605511802,
0.00045172781144112184,
0.001083896738435293,
0.0892052925496936,
0.013650888891601341,
0.0006517662269337108,
0.29048008051098523,
0.24259962959547435,
0.000790999080626543,
0.31128862612040564,
0.00042946179724921555,
0.017924185993159286,
0.0007453112440675867,
0.004550258600491045,
0.0006476087843179422],
[0.03845017698467218,
0.05684806127828719,
0.002425462843676183,
0.19031071136815697,
0.002628326201944101,
0.11313291089962103,
0.14747957419267374,
0.15201072883075864,
0.057607257083714204,
0.16612476787031594,
0.000961017406058512,
0.0028845869071095873,
0.0016678016137124127,
0.0008760243791118095,
0.06659259214018749],
[0.0007177327059646266,
0.0005920807912990945,
0.006871588022532671,
0.07331422932654867,
0.023343176598313517,
0.04991256794912571,
0.16269610784540048,
0.21440843708834922,
0.006487686317539553,
0.413457715742361,
0.0005628966698703897,
0.0452969634098932,
0.000976881343089867,
0.0005131137090947655,
0.0008488224806171558],
[0.00029037444263737704,
0.00023953921611905067,
0.06232286685986151,
0.20828932029137348,
0.0006228340434149224,
0.011372061632726804,
0.052590527121078354,
0.005147905656123885,
0.0004194457257783165,
0.3878022970376952,
0.23178312707097717,
0.0006835601020938344,
0.002600508159520988,
0.00020759135824490761,
0.035628041282354264],
[0.18007577883012535,
0.0008455889501166258,
0.00202894548857724,
0.17476813466428473,
0.025553088638006975,
0.001220040709462018,
0.06887574693276699,
0.04152688456247651,
0.0014806705834568565,
0.48149835367821975,
0.0008039092149155755,
0.017982640852973138,
0.0013951475566019486,
0.0007328109422565454,
0.0012122583957598296],
[0.0004797569849598963,
0.00039576696578784974,
0.0009496216804304388,
0.07086721849666272,
0.0010290471161481676,
0.0005710242661695641,
0.054097892771729376,
0.00850537559024861,
0.34318969640268293,
0.21442832827446287,
0.0003762593050821539,
0.10314966873168208,
0.17190132460834032,
0.00034298267860880574,
0.029716036127004346],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.13680567632871515,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.15510062368538338,
0.0009833878030756174,
0.002359586470822459,
0.10366081751829787,
0.011610383548723,
0.001418860964036008,
0.10726020596894256,
0.16598895428189556,
0.001721963599386564,
0.17971965135409762,
0.0009349158555336334,
0.10239411968363243,
0.0016225035703977446,
0.0008522312673031561,
0.16437179442847238],
[0.012289465482125358,
0.00062621529753551,
0.3185873110337527,
0.1524882268863253,
0.0016282436426546412,
0.041259761675665405,
0.022181221820726977,
0.0019275560365335323,
0.024157244245666495,
0.25280861788642606,
0.0005953486598192447,
0.0017869967166601686,
0.0010332002826467057,
0.12161141487549024,
0.04701917545797169],
[0.0004997881957862085,
0.0004122913557984485,
0.0009892710710224881,
0.21806316710669132,
0.0010720127432885323,
0.125853349840696,
0.14745371183040265,
0.0012690758191432217,
0.0007219437792559965,
0.15885421969965668,
0.00039196919509259815,
0.1568007102512736,
0.0006802445533033231,
0.004153014802531204,
0.18278522975605782],
[0.020275598499136193,
0.0007032479255123578,
0.014636143588508751,
0.09355383581778949,
0.0018285387923801554,
0.001014666875497194,
0.3292051384943079,
0.06690835940572398,
0.007705792876972152,
0.14794561995892586,
0.039514797630589064,
0.26745594505497855,
0.0011602973582242701,
0.0006094542447173579,
0.007482563476736809],
[0.0012368751592449117,
0.0010203380965336888,
0.0024482467249601447,
0.17331117413733643,
0.0026530157049462814,
0.0014721739386665273,
0.01735423267769726,
0.003140707141807118,
0.001786665500429522,
0.45888756514163903,
0.0009700448403680278,
0.05927341601080333,
0.27409850817005676,
0.0008842534210481058,
0.0014627833344628845],
[0.0008006545027086768,
0.0006604856481826538,
0.001584800009467225,
0.3493344942735939,
0.0017173511441678775,
0.07392116466852422,
0.0233951146345404,
0.05675919110444037,
0.0011565449973354157,
0.07814268534758162,
0.0006279297983024605,
0.11741776990587346,
0.0010897433535591867,
0.29244518114234397,
0.0009468894693785477],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.1294034699905887,
0.01841715630801397],
[0.0006601572565511275,
0.04065384076147729,
0.0013067024826471962,
0.19277449537570032,
0.0014159938101057778,
0.06596328394212074,
0.07945367056014428,
0.0016762893125217802,
0.0009535968010370186,
0.17974447411761627,
0.17599573610563324,
0.1569774188780378,
0.000898517375717822,
0.0004719524910593503,
0.10105387072962997],
[0.0005215650098818798,
0.00043025575008421457,
0.0010323756749037248,
0.1998369432143663,
0.0011187225744042138,
0.024387377899442078,
0.01920122181657293,
0.05281865519245562,
0.0007534003754720252,
0.20142571766840361,
0.19054178568448793,
0.2903880026179215,
0.0007098842272727663,
0.00037287161993664124,
0.016461220674394546],
[0.004648377860833262,
0.00044616135246105626,
0.0010705403176431377,
0.14971902804748738,
0.03812786499763372,
0.21834291738897033,
0.06509389769203909,
0.013695926445753985,
0.0007812519191189741,
0.3074527423772015,
0.020961828450467023,
0.1778970516536336,
0.0007361270752774289,
0.000386655858086068,
0.0006396285633934535],
[0.0006771317891435346,
0.12398032652654636,
0.06819374335957902,
0.11545012025992166,
0.037450410195571535,
0.09337225101174706,
0.14320752121410088,
0.0017193915086838863,
0.0009781165042116581,
0.338643392440015,
0.041671634142996794,
0.01187915664977911,
0.0009216208292171398,
0.02105437752998614,
0.0008008060385001812],
[0.0012486040740239627,
0.001030013655534622,
0.0024714627116185547,
0.13702383601026905,
0.002678173454197059,
0.12476122052557133,
0.283034368359439,
0.00317048952213619,
0.0018036079115019551,
0.16927539019541799,
0.03891003930037245,
0.20207597228386276,
0.02066483004335432,
0.0008926385300391289,
0.010959353422661607],
[0.020407520354351612,
0.0010398744721037425,
0.0024951232139097035,
0.33937874047591715,
0.03142425708661727,
0.0015003615982941954,
0.14214177167601796,
0.022347804937047022,
0.0018208747182888068,
0.2474837961641078,
0.000988618252833566,
0.1752900986564662,
0.0017157016168768315,
0.010474665584626877,
0.0014907911925412668],
[0.007233199909932474,
0.0006942581586640128,
0.28289648457618916,
0.0667914947063696,
0.05293800950966202,
0.007393301838785374,
0.05015779606982096,
0.0021369990639195265,
0.0012156824337841061,
0.43367665473119316,
0.007051643299933761,
0.001981166948379721,
0.0011454650318898736,
0.0006016634623119188,
0.08408618025916433],
[0.06075173512622134,
0.0003639417307347653,
0.010925018289354686,
0.2863072068747563,
0.23883794014742588,
0.0005251058771608083,
0.0899546989226814,
0.014522596706658054,
0.01068904061029392,
0.2440934835632227,
0.00034600276054890276,
0.0010385608279423508,
0.0006004719152373758,
0.0003154020432616075,
0.04072879460450002],
[0.032912237751199724,
0.0003914877132854811,
0.0009393538427795728,
0.08451770413109641,
0.019038847245150602,
0.03660670355154115,
0.08595062443920064,
0.10212222988478058,
0.2890203436486078,
0.24094329276898394,
0.000372190980254671,
0.10563854250817362,
0.0006459203689388233,
0.0003392741591703931,
0.0005612470068366971],
[0.0009115060958508604,
0.01459705274774595,
0.09179751086530262,
0.2730941828887107,
0.02272280358931884,
0.0010849078091814146,
0.012789074755282607,
0.0023145211411531533,
0.1743806957565162,
0.22049029503776452,
0.021482550528420623,
0.1336744034640867,
0.0012406196509502168,
0.0006516440867438675,
0.028768231582971697],
[0.027710095287019437,
0.03785456848272811,
0.0008881376400199525,
0.3320775173784428,
0.000962420610269669,
0.0005340528281958398,
0.0710413304243048,
0.004547012948758497,
0.13354746703659418,
0.27210616545127925,
0.00035189808545624284,
0.0010562562171555561,
0.11647165538021531,
0.0003207759816617415,
0.0005306462478987007],
[0.0008206140791599731,
0.0006769509446935538,
0.0078565764533451,
0.27702358522940923,
0.02668923850021371,
0.007208993659939799,
0.13615917242042255,
0.03947733872304688,
0.0011853765947542586,
0.21096835598557595,
0.00064358350757764,
0.15773849941267762,
0.0011169096477648295,
0.13146431029308178,
0.000970494548337137],
[0.0006841685619354881,
0.000564392649472897,
0.042922343646276874,
0.2517462505556233,
0.0014674964777799016,
0.000814322382504041,
0.10832363839886382,
0.006933273680468054,
0.000988281118714402,
0.3421625841960405,
0.0005365732980300889,
0.04317869042144281,
0.0009311983390601434,
0.18234961561706242,
0.01639717065672524],
[0.0008206140791599731,
0.0006769509446935538,
0.0016243076083653299,
0.5138498013386406,
0.0017601631202946288,
0.0009767248149600291,
0.04890740859070578,
0.2015163286925209,
0.1382952911843092,
0.06762617255104124,
0.00064358350757764,
0.0019317782881833588,
0.0011169096477648295,
0.01928347108344591,
0.000970494548337137],
[0.0005321035839537637,
0.008521220118651411,
0.07379367236330063,
0.17154570088200088,
0.06579949320045698,
0.00871559985791663,
0.06000054930016257,
0.017515673454017926,
0.016933164859828435,
0.4237169711561219,
0.10548683308306443,
0.02145828293789154,
0.01688876943839874,
0.0003804057434141644,
0.008711560020819973],
[0.023414847468839067,
0.0006155687096372843,
0.02414566643378007,
0.2349031094087568,
0.0016005610882209294,
0.000888160713458837,
0.010469787075918105,
0.001894784727923739,
0.31277172357391614,
0.3051821023489361,
0.011919547959253969,
0.05842822066853885,
0.006682794901365848,
0.0005334689934994158,
0.006549655927954828],
[0.0007547599428760237,
0.0006226257498162832,
0.0072260875674933205,
0.11722136232823402,
0.08186873538514883,
0.0008983428194401181,
0.1710894655392754,
0.13948763568659073,
0.0010902503304100503,
0.37746635386392874,
0.012056196764735853,
0.0075088837790487735,
0.0010272778439380465,
0.0693251491473954,
0.012356873251668364],
[0.0008636753341790245,
0.007271777151168214,
0.04762466736878368,
0.25220441805733024,
0.16583511648370897,
0.0010279778916602042,
0.05803310050997261,
0.021870978743569684,
0.06028131088571273,
0.3663434789799993,
0.0006773551844835802,
0.00859245084267365,
0.007734822435851882,
0.0006174494355508743,
0.0010214206953553845],
[0.0005074933900312224,
0.0004186476183287587,
0.29007175808834224,
0.4064280600636255,
0.0010885398771620619,
0.01216672656758013,
0.0880593168876446,
0.07066477757976963,
0.0007330739281871779,
0.07265597681872012,
0.0003980121525127894,
0.032028510513689615,
0.019961880862311113,
0.00036281168955512377,
0.004454413962540121],
[0.02859185296763512,
0.0005064941337552794,
0.0012153056015359182,
0.15597605402367998,
0.06659864970694906,
0.0053937630601747786,
0.08788524445437568,
0.0015590418005293932,
0.047516681442516626,
0.2977356994530394,
0.0004815286450590355,
0.001445354908432265,
0.03347651943164452,
0.270891687156831,
0.0007261232138419676],
[0.007834067123557819,
0.0007519306923320339,
0.0018042175051121735,
0.01695942486355326,
0.09887097489409537,
0.0010849078091814146,
0.05432444092152435,
0.0023145211411531533,
0.015161792119256202,
0.5873860295062332,
0.15301121005485283,
0.057526232159310194,
0.0012406196509502168,
0.0006516440867438675,
0.0010779874721438688],
[0.0010093257646916717,
0.0008326252830209767,
0.0019978398622968745,
0.12609597876869713,
0.140143329522346,
0.24649625594403837,
0.1368090136438535,
0.01789383998720762,
0.0014579705227245022,
0.2518180140471108,
0.00079158453702586,
0.04836881514987688,
0.0013737586436193448,
0.0007215762671840382,
0.024190072056306484],
[0.05874958409744534,
0.0006298464740085478,
0.15807367476167528,
0.18236548172297065,
0.0016376851878082803,
0.006707368197733303,
0.2020666622408474,
0.0019387331766606874,
0.0011028941970325586,
0.3180592338801206,
0.0005988008527875387,
0.001797358808516547,
0.0010391914051457055,
0.0005458425018816853,
0.0646876424953659],
[0.0008636753341790245,
0.0007124735588006343,
0.09353979251535675,
0.1997299893183896,
0.028089741043989765,
0.0010279778916602042,
0.12362613643364842,
0.0021930679664669407,
0.0012475785544044991,
0.21547949635554492,
0.0006773551844835802,
0.32343902327631757,
0.0011755188434843004,
0.007176753027918455,
0.0010214206953553845],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.2897476887972528,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.13668744403732208],
[0.21009896829948166,
0.006824159792734478,
0.0016043106181983938,
0.16281335284678594,
0.0017384936012777488,
0.0009647002720594302,
0.011372048197457916,
0.008213615577214995,
0.22892586873989065,
0.3499485920055097,
0.0006356602958618589,
0.014219081698396622,
0.001103159277373973,
0.0005794420709738006,
0.0009585467067828556],
[0.0011813876153939375,
0.0009745646371420428,
0.011310629877175347,
0.47059152944521393,
0.0025339985800851123,
0.0014061306396581708,
0.01657570322021216,
0.002999811656962489,
0.019650942016077765,
0.15119052579610276,
0.0009265275902922873,
0.029697704745801256,
0.019552374363765553,
0.2520665802652903,
0.019341589550826915],
[0.0636552124380475,
0.0007571722635348408,
0.0018167943750482397,
0.058902547509617736,
0.05076446782671618,
0.0010924705029087703,
0.12441129598760423,
0.14174699391113613,
0.05709238379654681,
0.277793827986779,
0.16104864012223516,
0.050956420059216634,
0.00822008471676188,
0.0006561865783782531,
0.0010855019254686186],
[0.0007958154014945261,
0.0006564937179665155,
0.007619153391800887,
0.2807398905522109,
0.14071740311784048,
0.0009472085240881596,
0.23479132935017624,
0.03224041520200381,
0.0011495549182415094,
0.15019757795243607,
0.0006241346334228689,
0.1469277639393879,
0.0010831570190447603,
0.0005689357458871624,
0.0009411665339982622],
[0.07317306928467465,
0.0003162575569199347,
0.038609484642199064,
0.33323086756999565,
0.06778883164946858,
0.00045630574295478277,
0.24412920890979778,
0.027177764060178158,
0.047139188533132315,
0.13641071797303675,
0.00030066897664587136,
0.024195189566060163,
0.000521797213330299,
0.0031856654204094432,
0.003364982901196674],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.1294034699905887,
0.01841715630801397],
[0.009634922225309534,
0.0009247806567365862,
0.3938576161479365,
0.1570801109779233,
0.01943232114572683,
0.0013343008424909545,
0.12640945083911603,
0.06244375790784511,
0.0016193394135423829,
0.2030644009323505,
0.000879197500894119,
0.011152880321118096,
0.01003969071358837,
0.0008014406814922261,
0.0013257896939294137],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.15781332392994726,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.08755329330123447,
0.0013334973496961148,
0.16279656981142004,
0.1282898588395123,
0.015743957412075994,
0.0019240093574597675,
0.3664277439936711,
0.028658015418715755,
0.002335023770758114,
0.1945973971029913,
0.0012677682310516828,
0.003805329245325354,
0.002200153595795113,
0.0011556459544470605,
0.0019117366158458418],
[0.024656372108592672,
0.16318993927849257,
0.0030146086026481365,
0.2596706147079238,
0.003266747540414607,
0.0018127373253897644,
0.056068899991269044,
0.0038672584227573345,
0.002199981371459674,
0.13707643285295135,
0.0011944488645340677,
0.23491891496575637,
0.013639594244664514,
0.08205559231947104,
0.013367857403675076],
[0.0009243031862811307,
0.0007624874237799859,
0.0018295478179580185,
0.07335552934239385,
0.1072788243387334,
0.0011001393730796691,
0.279719139434858,
0.023406266845613127,
0.001335155454066794,
0.48331663080786824,
0.02178415481064808,
0.00217586911107396,
0.0012580373313530672,
0.0006607928443269177,
0.0010931218779657477],
[0.044230764863073105,
0.005369786069979035,
0.0061060635037190674,
0.08452137075356406,
0.059491971171078344,
0.15091273665027127,
0.18332038533588646,
0.006463119248416462,
0.0009212644726839059,
0.06224580969759358,
0.010187518815848827,
0.3744636149765659,
0.005711718182002625,
0.0052996162610408504,
0.0007542599982765569],
[0.0013373777266104862,
0.011260148819801287,
0.033117888329414084,
0.24833502984705502,
0.04349619878191568,
0.011748698684220628,
0.018764354730623677,
0.12527874108185483,
0.032402550174695954,
0.18131058613405293,
0.0010488660505669882,
0.013305176053117767,
0.0018202589054846744,
0.2751924825595154,
0.001581642121070617],
[0.0005174655456818493,
0.0004268739702276115,
0.06390369716280506,
0.28865542802087524,
0.07970922435374143,
0.0006159063708383759,
0.0858597021979533,
0.017033821600149282,
0.004677443444640419,
0.13303312509996185,
0.00040583302114608204,
0.0012181471551821615,
0.043933916760240714,
0.2793974376278934,
0.0006119776686632212],
[0.09866136863952958,
0.00046328808719839056,
0.0011116350022113088,
0.06589692474564751,
0.19313892634967983,
0.0006684457341984752,
0.2552617564971061,
0.027017291116954847,
0.0008112417295496119,
0.2723376116275419,
0.0004404522580481081,
0.0013220601507971746,
0.0050295916906128195,
0.0004014983635598125,
0.07743790800736461],
[0.0007816428139040031,
0.0006448022946753411,
0.14401827501977216,
0.1095239344255362,
0.17976545529802773,
0.006866635892403433,
0.19499218019790346,
0.013857360951666136,
0.0011290826231108828,
0.331547905136716,
0.012485611687683843,
0.0018400374248513744,
0.0010638671966841067,
0.0005588036327402911,
0.0009244054043250876],
[0.0008929613573754144,
0.034645234578794044,
0.008549230816615216,
0.34891868259919406,
0.008697063671814949,
0.0010628351848919876,
0.11425468508037594,
0.002267431835383333,
0.0012898821991164777,
0.3041667497350348,
0.12277129068732492,
0.049574131283976586,
0.0012153790441357395,
0.0006383862827393256,
0.0010560556432271852],
[0.00043534315051559254,
0.08632227030420407,
0.1000499502649124,
0.057694087403242444,
0.02077143046175978,
0.0699499296275763,
0.14497170874672702,
0.0738434782121111,
0.0006288529461205652,
0.24086532314398262,
0.0036477015221412718,
0.1332758130489027,
0.0038988052971354933,
0.00031123082010013034,
0.06333407505056857],
[0.026944244952912606,
0.0005703194251433278,
0.006619029342657553,
0.018113850922273807,
0.04348753381578008,
0.016574608807279314,
0.5452591662760985,
0.19077632388179208,
0.0009986592136371705,
0.1409831308489829,
0.005792786304266283,
0.0016274896895517783,
0.0009409769987670718,
0.0004942547029131004,
0.0008176248179444192],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.13680567632871515,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.13668744403732208],
[0.0009308374326541273,
0.007837253313087328,
0.07960561295129887,
0.1304291117648091,
0.0019965849496814815,
0.0011079166714975826,
0.11203156518423214,
0.3275548844482221,
0.1003158522801061,
0.12619522304874725,
0.0007300284446805396,
0.07995438251970696,
0.0012669308697412253,
0.0006654642371236012,
0.029378351884411565],
[0.0011210384341754434,
0.0009247806567365862,
0.036274496920304815,
0.24221894888926418,
0.002404553563458652,
0.0013343008424909545,
0.02424284534550697,
0.07947152549011328,
0.5039384830904536,
0.07535614406533918,
0.017906965083162297,
0.0026389965299840083,
0.001525806922454279,
0.009315324472626315,
0.0013257896939294137],
[0.001170882209499363,
0.0009658983899677301,
0.0023176215588895186,
0.0929247951865152,
0.0025114651767607576,
0.001393626722300377,
0.40769519596763315,
0.002973136043765749,
0.0016913387201149014,
0.30101737353044444,
0.0009182885091628505,
0.17171248946551015,
0.001593647573686215,
0.0008370744546492807,
0.010277166491100331],
[0.0007138416111568596,
0.00601024124707442,
0.19116092632965456,
0.13797320987803938,
0.2563355494287675,
0.0008496403282303828,
0.1618140719910774,
0.06144767988526089,
0.028137995547406383,
0.1347263207473404,
0.0005598449985570444,
0.017944540070647736,
0.0009715853075458372,
0.0005103319296486564,
0.0008442206995925524],
[0.015572862094219599,
0.13111684249114675,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.13668744403732208],
[0.0008693778474167097,
0.0007171777453621543,
0.29223576337893387,
0.16803566831940506,
0.061288267358079504,
0.0010347652309568767,
0.005595373524693122,
0.0022075479436238763,
0.19933417972659423,
0.2433126724112308,
0.0006818274980408546,
0.02185440772249202,
0.0011832803384591332,
0.0006215262146835991,
0.0010281647400281972],
[0.0019519886683892953,
0.0016102582281428877,
0.003863728549177803,
0.4514088343828958,
0.004186887055178187,
0.03197263216018276,
0.012563128619001109,
0.06425515093771873,
0.002819646578697238,
0.22016048682242575,
0.0015308873511404725,
0.004595106791531532,
0.12125400089157314,
0.0013954946422596866,
0.07643176832168562],
[0.0011022692495805055,
0.0009092973527507742,
0.0021818103954462215,
0.062365444973278575,
0.1530483894003231,
0.0013119610742417826,
0.3754331757144011,
0.0027989121444279732,
0.00159222733651802,
0.24989259072377967,
0.00086447738097082,
0.002594812663038266,
0.0015002608297328079,
0.0007880224188936876,
0.14361634834261677],
[0.0009940855069110713,
0.0008200531042539346,
0.00951739555115118,
0.1468411654882346,
0.1908752968962212,
0.0011831968369176412,
0.2932874347707628,
0.1308694823510458,
0.008985677938395609,
0.05927265307066708,
0.000779632050701391,
0.15333458037547876,
0.0013530156520209062,
0.0007106808668039562,
0.001175649540434075],
[0.001170882209499363,
0.0009658983899677301,
0.0023176215588895186,
0.1729566592581925,
0.21592976936790023,
0.001393626722300377,
0.2298466091416836,
0.100789858798038,
0.01058376806141238,
0.2387703681413621,
0.0009182885091628505,
0.0027563319808580683,
0.001593647573686215,
0.0008370744546492807,
0.019169595832397808],
[0.0002690170645914326,
0.0002219208281196888,
0.16806569243913722,
0.2317880679975343,
0.09864524159627021,
0.04118195130852498,
0.1059288930106547,
0.008855446944990741,
0.004474770743096802,
0.23669371481205753,
0.00021098217837698497,
0.019021074284146604,
0.00036614989004712316,
0.00019232277235684336,
0.0840847541300949],
[0.0010665552684985334,
0.0008798357412013391,
0.002111118833273848,
0.03604446745851535,
0.02658800198217294,
0.04176997168866393,
0.19316680650214058,
0.0027082262295172158,
0.05014126094727095,
0.45239866431708187,
0.000836467955105466,
0.16451281444656313,
0.0014516517563040677,
0.0007624901655252392,
0.025561666708165544],
[0.0004147728556430652,
0.0003421594676370154,
0.0008209933539698734,
0.27547154617928443,
0.14264194147407971,
0.025694083301620502,
0.17592229355544556,
0.007353303927777375,
0.0005991391662716405,
0.1853835438302209,
0.00032529416209384784,
0.1458787336845466,
0.022614887920115616,
0.0160467783752945,
0.0004905287459994331],
[0.0006986901783047888,
0.011188973319511122,
0.13934679098324187,
0.19872030846911878,
0.07048055297883699,
0.0008316065400897173,
0.025722019001484457,
0.0070804338193179005,
0.0222344602290932,
0.3653439563651032,
0.0005479621750137965,
0.038788866214671716,
0.0009509632125078602,
0.11723811456953659,
0.0008263019441679768],
[0.0052769611374967,
0.0005064941337552794,
0.005878283967563602,
0.12333520546148621,
0.05260971460886601,
0.0566865250864793,
0.11586311465054178,
0.0015590418005293932,
0.0008868977822397863,
0.2837467643549563,
0.0004815286450590355,
0.1926274679155673,
0.0008356708694507394,
0.15898020637216656,
0.0007261232138419676],
[0.0007462052513234392,
0.0006155687096372843,
0.0014770242162644412,
0.3312448388331982,
0.07527364829514671,
0.000888160713458837,
0.06714139261970717,
0.01889626639106046,
0.14275690694254892,
0.17483740959822125,
0.09125979572055867,
0.05842822066853885,
0.0010156343469869401,
0.006200629547878323,
0.029218298145470455],
[0.0010171224827424186,
0.0008390570464820244,
0.0020132725349401117,
0.0420985552110789,
0.23392204591611201,
0.0012106162859955127,
0.029720306325168366,
0.04120610268829296,
0.024643271399772185,
0.36963342010994266,
0.0007976992738773531,
0.24185943642863028,
0.009109049996204575,
0.0007271502125881397,
0.0012028940881724994],
[0.0015230060179176567,
0.0012563766437674521,
0.0030146086026481365,
0.07460368598252363,
0.22303372540182728,
0.0018127373253897644,
0.20643577958065665,
0.0038672584227573345,
0.002199981371459674,
0.4378101920317266,
0.0011944488645340677,
0.03828530319501872,
0.0020729111993270048,
0.001088811002108482,
0.0018011743583375669],
[0.0010093257646916717,
0.00849809152040565,
0.0019978398622968745,
0.11076504629392776,
0.009830403486806548,
0.08552146495896021,
0.014161553845698711,
0.11754490107320838,
0.0627817004218019,
0.3361381426583422,
0.00079158453702586,
0.010041483962953502,
0.23900321200254426,
0.0007215762671840382,
0.0011936733441524634],
[0.016963849603145172,
0.0003005083412965844,
0.01178743177799169,
0.11467500324282244,
0.08931238653766356,
0.0004335823095419734,
0.2347384858659505,
0.05072369737432205,
0.036491934605445406,
0.22921504404780488,
0.00028569605207589275,
0.21388532234763538,
0.0004958123897439882,
0.00026042890072195107,
0.0004308166038385441],
[0.0005408461134097952,
0.00044616135246105626,
0.16947934196200523,
0.15793409154233432,
0.17778394441003156,
0.008858798270373556,
0.08973908817657988,
0.14924447411072836,
0.0007812519191189741,
0.20476444869161484,
0.00042416971334969546,
0.001273186514424549,
0.033596381054665156,
0.004494187605509533,
0.0006396285633934535],
[0.015914370928144597,
0.003438059843351868,
0.013213086648887698,
0.1502530457325734,
0.1218228958509435,
0.03149808190768061,
0.126676348614627,
0.013441695200827086,
0.019197084359843804,
0.38718857121610867,
0.0003202501412031133,
0.0009612618444688731,
0.003656985336505711,
0.012696750645050464,
0.09972151172978368],
[0.0006377741894516056,
0.0005261204395766464,
0.04485538854693818,
0.2734243303392572,
0.0013679836062496745,
0.010446433368601989,
0.10097806961904583,
0.001619453618014072,
0.0009212644726839059,
0.19302478171845808,
0.18455948151033483,
0.16618599286926317,
0.010555383812405015,
0.00045595063063846153,
0.010441591259081335],
[0.0016571827731110384,
0.0013670633642256917,
0.0032801954721172573,
0.08117626704447334,
0.01614025509003329,
0.12782951191597042,
0.46375119938863346,
0.1174793289687154,
0.002393799621969243,
0.03588149456902407,
0.0012996797506908586,
0.14234389453869503,
0.0022555345739279824,
0.0011847351977866565,
0.0019598577306263313],
[0.0005640131318606071,
0.004748749492911471,
0.005399873586972222,
0.14756525421780287,
0.0012097709986637719,
0.08634084744374211,
0.06788217977723197,
0.0014321575286458288,
0.14645293200881124,
0.3163389327207431,
0.00044233892513064854,
0.001327723164942456,
0.0007676589086626726,
0.21886054118858686,
0.0006670269052921862],
[0.02861144601963772,
0.010128529428076474,
0.11201503089669561,
0.1137440362861149,
0.020852615077838574,
0.0014318239009042941,
0.2361463534278708,
0.2588670278622658,
0.001737695729591091,
0.1996339128266889,
0.0009434573937954013,
0.002831878828001425,
0.01077348425754304,
0.0008600173861655749,
0.0014226906788103839],
[0.020246858701506765,
0.00042855818879991667,
0.11939241153262986,
0.18326661128093066,
0.31280646255964656,
0.0006183364111334399,
0.0704165778944234,
0.03682837954828727,
0.0007504278566649518,
0.20852193908560115,
0.008298374827631468,
0.01305936422513583,
0.0007070834001360781,
0.00037140046603365684,
0.02428721402143896],
[0.003414252583392589,
0.0003277073413255236,
0.058109295296588474,
0.14315622737583533,
0.2753989826245671,
0.012540821418924456,
0.33140963088631203,
0.013076712984447814,
0.0005738327354166992,
0.1473833275958097,
0.0003115543922975069,
0.013003156300036713,
0.0005406883527365948,
0.0002840003119104413,
0.0004698098003991256],
[0.001111574617060615,
0.00935898315290367,
0.03596826727257764,
0.18108006769805363,
0.010826263802684524,
0.24614131225662936,
0.07469024403427227,
0.045032588103648456,
0.001605668934824255,
0.26044419585272366,
0.0008717753072363287,
0.0110587275994585,
0.03528096405895352,
0.08521476994423964,
0.0013145973647339377],
[0.0015408282903797686,
0.0012710788094823797,
0.0030498856634416853,
0.06377466426506292,
0.015007011936276625,
0.025238023636154922,
0.033320946730192744,
0.003912513223119854,
0.013927762430359916,
0.5833579045598123,
0.0012084263490977152,
0.0036272088339274824,
0.0020971684824562476,
0.24684432502328074,
0.0018222517669547384],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.13680567632871515,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.043300747604611764,
0.00031082754674207356,
0.0007458140844920929,
0.24452311788425235,
0.0008081932506863116,
0.17500589206025147,
0.045349012001800616,
0.08966626860639268,
0.0005442753301418766,
0.3486883827034256,
0.0002955066158812636,
0.009471782946155105,
0.009097629364712067,
0.0031309688755282628,
0.029061581124926537],
[0.0011813876153939375,
0.0727522776083987,
0.0023384157557682643,
0.16553624931737318,
0.0025339985800851123,
0.0014061306396581708,
0.00760348909880508,
0.002999811656962489,
0.001706513773263599,
0.4652180200453506,
0.018870955833106454,
0.011753276502987091,
0.0016079461209513887,
0.2430943661438832,
0.0013971613080127504],
[0.0009866366743444138,
0.0008139083227166442,
0.009446080271491322,
0.4529600434536872,
0.09203407970601234,
0.031146933984694317,
0.058802114402098406,
0.017491596265376986,
0.06886355293906432,
0.20869152924580178,
0.0007737901502120463,
0.0023226061485260227,
0.023822329566802834,
0.03067795864797884,
0.0011668402211925416],
[0.13607740735775373,
0.0014588677780994353,
0.016931368991808502,
0.3821072783103355,
0.0037932516093307496,
0.002104897514044179,
0.15912182139233494,
0.004490547265847284,
0.002554553963704199,
0.18603092836379292,
0.0013869590538000667,
0.07131756073239198,
0.029268791271027404,
0.001264295460518152,
0.0020914709352110256],
[0.0012253645477922296,
0.0010108426229672714,
0.002425462843676183,
0.2182293206958169,
0.021240732420384073,
0.09452050468118106,
0.28707262083097357,
0.04964249462933881,
0.0017700384283942886,
0.3150240176178357,
0.000961017406058512,
0.0028845869071095873,
0.0016678016137124127,
0.0008760243791118095,
0.0014491703756475908],
[0.1734452215327932,
0.02584334143172704,
0.026274625485927475,
0.18002252667769764,
0.0008013141361485798,
0.023142573513653376,
0.056311973721312816,
0.0009486159563777647,
0.051609961734979753,
0.32869700516552086,
0.00029299134635188005,
0.02074012158852658,
0.0005084730382289103,
0.00026707899425643865,
0.11109417567649772],
[0.0005114357720247591,
0.008190241376675412,
0.004896496838076778,
0.2581026716212822,
0.001096996026730249,
0.000608729514251293,
0.0071758053519595045,
0.1022870919509326,
0.02792796415913927,
0.20139802901386059,
0.0004011040468587998,
0.07500319750663552,
0.2958930769742568,
0.0003656301346817859,
0.01614152971263456],
[0.3085876538856348,
0.0006686169435999072,
0.0016043106181983938,
0.2059021527907279,
0.00789403645041232,
0.02558687166859772,
0.24528267646457164,
0.002058072728080424,
0.0011707833219115164,
0.08526024949272314,
0.0006356602958618589,
0.11270776728454976,
0.001103159277373973,
0.0005794420709738006,
0.0009585467067828556],
[0.0006913531232353914,
0.0005703194251433278,
0.0013684509767221097,
0.674436146664204,
0.027735798717973752,
0.0008228737094729863,
0.135714053733134,
0.007006081074051604,
0.02200097267737894,
0.08847734718962846,
0.0005422079383308397,
0.03838153825109988,
0.0009409769987670718,
0.0004942547029131004,
0.0008176248179444192],
[0.00055451217150247,
0.0004574349232795624,
0.0010975906482431407,
0.23772852784838974,
0.07278184132017274,
0.0006600006164386703,
0.1846556605481541,
0.0014080324309528297,
0.005012313077095616,
0.19730445943558375,
0.26574808190036386,
0.03078460110460234,
0.0007547274777300018,
0.00039642584863876614,
0.0006557906488524014],
[0.00909300472643767,
0.0008727662440839018,
0.1547595217038119,
0.42143577347116107,
0.002269309124267439,
0.0012592529118075194,
0.0630543994925804,
0.002686465579629547,
0.0015282594500211073,
0.14343290083859084,
0.0008297469189841413,
0.0024905658145493005,
0.009475006956550559,
0.1855618062935809,
0.0012512204739437028],
[0.0005640131318606071,
0.004748749492911471,
0.09535288894652326,
0.4217077772183394,
0.056894970983147754,
0.009238262849841212,
0.20066996435561688,
0.022849542138062746,
0.10790163971186079,
0.05933031740774011,
0.00044233892513064854,
0.014178153930592607,
0.0007676589086626726,
0.004686695094417678,
0.0006670269052921862],
[0.16158753683005275,
0.0012420106996655033,
0.014414562966910334,
0.23383258126409387,
0.014663818840984204,
0.0017920097169793,
0.2612474286042692,
0.060995161943940286,
0.002174825850171929,
0.15837789206472502,
0.0011807910289593172,
0.0035442587415124537,
0.024918058010884786,
0.02394521043324821,
0.03608385300360287],
[0.0005763571955909842,
0.000475455586182045,
0.0011408302656625978,
0.18581269147142435,
0.15443914585000149,
0.0006860013610670012,
0.0649906322216488,
0.0014635019119711315,
0.0008325476581770538,
0.26198120810888337,
0.241199430601481,
0.06701516659073103,
0.01829336257833594,
0.0004120430571652428,
0.0006816255416779041],
[0.2703284854518569,
0.0007316705560269427,
0.03543579678053233,
0.31288816053797497,
0.00863847991627674,
0.0010556758861908749,
0.05286071482417022,
0.008988196805207746,
0.021489308936732055,
0.2751737168934633,
0.0006956059468271629,
0.002087928682743373,
0.0012071922041291914,
0.007370124566088622,
0.001048942011779589],
[0.0004904796001797482,
0.2797808267085747,
0.04939605629809921,
0.050101010569447574,
0.00477706260999627,
0.0005837867139906133,
0.01433180783132864,
0.0012454391792746909,
0.0007084975179229891,
0.42782172892882186,
0.0003846687371024549,
0.1315301871841588,
0.03791773685178337,
0.0003506483747947691,
0.0005800628945244185],
[0.0005195071906247365,
0.028046850304034754,
0.01286471337386692,
0.3292490124614577,
0.0011143086877105277,
0.1071460345698964,
0.011234523361777328,
0.0013191468286996193,
0.0007504278566649518,
0.2755949342226,
0.004352904525455063,
0.009113893922959426,
0.05594366763060575,
0.15424474225091347,
0.008505332812733338],
[0.0011813876153939375,
0.0009745646371420428,
0.0023384157557682643,
0.37189717410973605,
0.14608942452259843,
0.0014061306396581708,
0.00760348909880508,
0.020944239899776652,
0.028623156137484847,
0.30371816586002315,
0.0009265275902922873,
0.11044763183846498,
0.0016079461209513887,
0.0008445848658919542,
0.0013971613080127504],
[0.06017641020195764,
0.008385669614004564,
0.35895310731276714,
0.22449277344404814,
0.08066019957522197,
0.004600105875547525,
0.15846738123535992,
0.0013296389305256324,
0.0007563965368102597,
0.04712955320139039,
0.00041067483400146444,
0.013163234526949152,
0.0007127073313560959,
0.008328057275698655,
0.03243409010436152],
[0.0007177327059646266,
0.0005920807912990945,
0.012322509833410312,
0.12237252562444745,
0.0015394893548029507,
0.000854271651226935,
0.059128593438725306,
0.13809553173606226,
0.08825151348070417,
0.44616324660762685,
0.1204831765091785,
0.007140510733749713,
0.000976881343089867,
0.0005131137090947655,
0.0008488224806171558],
[0.0017684700017416548,
0.0014588677780994353,
0.043793156463010914,
0.11348940359831128,
0.0037932516093307496,
0.002104897514044179,
0.011381990300721649,
0.004490547265847284,
0.04284723517050782,
0.1188764596857869,
0.0013869590538000667,
0.17876471061720162,
0.4724882845458673,
0.001264295460518152,
0.0020914709352110256],
[0.0010579854763849024,
0.15353813199508645,
0.0020941559528093435,
0.22859531146989467,
0.010304328374320207,
0.009294272161860287,
0.2076847459935302,
0.010721484829682314,
0.0015282594500211073,
0.23181811258917126,
0.1293900549198284,
0.0024905658145493005,
0.0014399877064977917,
0.000756363542367268,
0.00928623972399647],
[0.03158480201464044,
0.0004218998160214908,
0.001012326057749818,
0.3241335748868405,
0.00498116680705721,
0.008377071074905214,
0.08874339173882567,
0.1294762874132213,
0.012391281037831428,
0.3334598355449772,
0.0004011040468587998,
0.005088123460750231,
0.000696097669407827,
0.0003656301346817859,
0.058867408296231126],
[0.0005814474489630556,
0.00047965469989025815,
0.0011509058145603534,
0.08147252317725026,
0.001247166459905023,
0.19057508448087868,
0.2377841017958331,
0.0058923115132129816,
0.014087553399535322,
0.22896789118346544,
0.00045601214770623553,
0.21774709492877,
0.0007913881590721335,
0.00041568212609197563,
0.018351182664865236],
[0.0006668439097919263,
0.0005501009867621174,
0.2646708024782554,
0.2605648011329061,
0.026752534756392787,
0.06663141809041385,
0.19674056038005988,
0.006757707947967256,
0.0009632556680369539,
0.17143620481360428,
0.0005229860824591233,
0.001569793425750158,
0.0009076183498609309,
0.0004767328409267923,
0.0007886391368124761],
[0.00902052463733114,
0.0008658094484983401,
0.010048435827554788,
0.2905409632377724,
0.13775775020045983,
0.0012492154416861084,
0.13429054562386744,
0.10628769217762461,
0.03339996707185866,
0.2538832138289487,
0.0008231330292487732,
0.0024707135833457504,
0.0014285096043281298,
0.008721306923404858,
0.009212219364070455],
[0.0017684700017416548,
0.0014588677780994353,
0.016931368991808502,
0.19407476601191853,
0.0037932516093307496,
0.002104897514044179,
0.038243777771924066,
0.004490547265847284,
0.01598544769930541,
0.3069089719842038,
0.0013869590538000667,
0.017593985789987145,
0.029268791271027404,
0.28331306390814354,
0.08267683334881827],
[0.0009308374326541273,
0.007837253313087328,
0.01598123273729176,
0.2647472477721574,
0.009065960529015604,
0.0011079166714975826,
0.16858656981890513,
0.22858362633754442,
0.0013445941694283886,
0.18981960326275435,
0.10677066213469237,
0.0021912511470316134,
0.0012669308697412253,
0.0006654642371236012,
0.0011008495670750747],
[0.007521652531487256,
0.0007219444646414595,
0.015025259889281823,
0.10268755310481963,
0.028463137767608015,
0.12067857740689031,
0.19173446144074208,
0.0022222204030119585,
0.001264162607064205,
0.3911527692865942,
0.0006863592620297532,
0.008706670229298849,
0.0011911450069302773,
0.0006256571864117162,
0.12731842941318847],
[0.0013107513776124918,
0.011035966342196212,
0.2713709810669148,
0.39271111632576955,
0.0028114753248087115,
0.001560104108946917,
0.2274391611583235,
0.0033282956505604248,
0.0018933796580168829,
0.07815395268012323,
0.0010279837874943667,
0.003085592992835585,
0.0017840186960660692,
0.000937068208650041,
0.0015501526216811748],
[0.0017684700017416548,
0.0014588677780994353,
0.09751673140541575,
0.2612292346899246,
0.0037932516093307496,
0.002104897514044179,
0.011381990300721649,
0.2059539532998654,
0.002554553963704199,
0.320339865719805,
0.0013869590538000667,
0.08474845446799319,
0.0024070037998249884,
0.001264295460518152,
0.0020914709352110256],
[0.0008105114289062284,
0.0006686169435999072,
0.30322591022579237,
0.2059021527907279,
0.03867175069608518,
0.0009647002720594302,
0.22066050506803336,
0.10670230116336812,
0.0011707833219115164,
0.09141579234185772,
0.0006356602958618589,
0.02037462454753119,
0.007258702126508545,
0.0005794420709738006,
0.0009585467067828556],
[0.0008415942455465081,
0.0006942581586640128,
0.014449046671978593,
0.22658163631601871,
0.0018051641945743026,
0.1863582604415924,
0.10768224704929465,
0.0021369990639195265,
0.0012156824337841061,
0.21636206214207035,
0.0006600376355477944,
0.2320789708662745,
0.0011454650318898736,
0.0006016634623119188,
0.007386912286532732],
[0.0006083097015130162,
0.000501814235903712,
0.26915789593951855,
0.12219561444829449,
0.0013047842213683427,
0.185519769987411,
0.017774801930238168,
0.0015446365865564183,
0.0008787030357480741,
0.3873825538377491,
0.00047707942300236154,
0.010671787016517878,
0.0008279494487156878,
0.0004348861973653139,
0.0007194139900978795],
[0.0011504221147629714,
0.0009490201998268809,
0.028488250080977346,
0.07382693489975889,
0.12478617152874467,
0.010106316650172972,
0.007404193081631173,
0.002921183213134189,
0.08029516463584796,
0.46176117379406,
0.0358504113743471,
0.011445209881814829,
0.0015658000412277777,
0.0008224473448472961,
0.15862730115884593],
[0.001981361665601562,
0.0016344889581733542,
0.00392186889073911,
0.1722946543560622,
0.0042498901984809115,
0.002358288939162008,
0.012752175178481276,
0.005031127585630081,
0.017909805225810854,
0.13318702603486735,
0.0015539237296940676,
0.034759711451915,
0.6046059380732789,
0.0014164936679715778,
0.0023432460441318473],
[0.0007296647423405847,
0.0006019239118376687,
0.0014442842532667458,
0.30173624561967377,
0.007106624122981601,
0.13386546567195395,
0.20419165882155307,
0.001852784616349593,
0.023220165672725432,
0.2707096787252014,
0.0005722546153631652,
0.046050008395353184,
0.0009931216281205977,
0.006063185368071044,
0.0008629338352081978],
[0.0007547599428760237,
0.0006226257498162832,
0.1791899983831631,
0.22039970881763588,
0.11052938718776047,
0.0008983428194401181,
0.03925046724726189,
0.06496994099980051,
0.0010902503304100503,
0.3488057020613171,
0.0005919360436911985,
0.03043740522113808,
0.0010272778439380465,
0.0005395848211274864,
0.0008926125306237111],
[0.0005892536771319013,
0.0004860943085535691,
0.0011663573113470907,
0.27052346435050406,
0.014689419665650667,
0.005176521010812424,
0.02169315492119244,
0.001496249009668905,
0.24251034511255495,
0.39314801407902844,
0.04073866242535516,
0.005862310817795168,
0.0008020129482097539,
0.00042126287036692566,
0.000696877491828458],
[0.001093118385464064,
0.0009017485106510523,
0.31763365767019314,
0.32750661091330696,
0.002344666898939191,
0.0013010693819252128,
0.0236390645987328,
0.0027756760206612387,
0.0015790089182372733,
0.28932722745825373,
0.0008573006271531735,
0.002573270940726661,
0.001487805902774327,
0.025687003565009674,
0.0012927702079714852],
[0.0005453260011345062,
0.2075276011439653,
0.025928737023170466,
0.38288624068170496,
0.009452798102283696,
0.09176327434407451,
0.03250063852173045,
0.0013847066565892037,
0.0007877231145211238,
0.18989431556156977,
0.00871079292516734,
0.03855772641192665,
0.0007422244966447967,
0.008672968338522762,
0.0006449266769944838],
[0.0006536033703561519,
0.0005391784399682883,
0.006257612414624548,
0.1858967928943334,
0.006365818723452868,
0.06034453338430707,
0.06377322547775933,
0.001659647505929337,
0.005908012283289108,
0.45097355439725173,
0.0005126019164684316,
0.20505780960523218,
0.010817362240894173,
0.00046726705757339917,
0.0007729802885599384],
[0.0008470079865913857,
0.0071314452086407845,
0.2589853944326945,
0.26663549926979374,
0.021114939554995073,
0.0010081398064971086,
0.005451399615782269,
0.002150745782847831,
0.0012235025798035693,
0.2885137908300387,
0.0006642834735601595,
0.008426632323657273,
0.12337453402574315,
0.0006055337955495302,
0.013867151313804909],
[0.0010412525084852137,
0.016674839432525672,
0.47653733759410033,
0.21707193782175382,
0.1287604320251769,
0.01705521347474636,
0.014609508626173566,
0.0421836686506025,
0.009412027062810475,
0.06999293837371481,
0.0008166237439783096,
0.002451175332584164,
0.0014172130384077278,
0.000744400989801639,
0.0012314313251384913],
[0.0011813876153939375,
0.0009745646371420428,
0.0023384157557682643,
0.0937585363461165,
0.09225613979415592,
0.0014061306396581708,
0.24088105625538922,
0.011972025778369571,
0.001706513773263599,
0.19605159640313818,
0.0009265275902922873,
0.28989191426660665,
0.0016079461209513887,
0.05467786959433445,
0.010369375429419833],
[0.006037025109474075,
0.0005794467163221277,
0.0013903514240465487,
0.17310736488962225,
0.0015066390677802167,
0.3742585813565633,
0.07387070512045425,
0.007118205156041408,
0.0010146415790089393,
0.20725469225031248,
0.0005508853382481907,
0.09767647422364836,
0.0009560362281772253,
0.0005021646677347359,
0.054176786872565816],
[0.0006226935917094316,
0.0005136799695099392,
0.0012325476303420694,
0.3899165107211862,
0.010793904544748247,
0.0007411526301885763,
0.06548643815742612,
0.006310294466295883,
0.05291995360491706,
0.11279443889708812,
0.0004883602853959203,
0.015653262479413087,
0.0008475268677982508,
0.31729714157132866,
0.024382094582652433],
[0.0009374647228724892,
0.2784419359500518,
0.1940877010748726,
0.15271685128181595,
0.1444049493588967,
0.0011158047140947096,
0.11282919715523725,
0.009500143460365517,
0.001354167286574431,
0.09149515879327713,
0.0007352260336481023,
0.00932655967960393,
0.0012759510469126957,
0.0006702021478206216,
0.001108687293956086],
[0.0009178600379786612,
0.0007571722635348408,
0.02272924517507119,
0.35864767564328004,
0.0019687492933292946,
0.008063287436249754,
0.012878225054148495,
0.0023306552443164794,
0.41260404739693696,
0.17323157398666425,
0.0007198506553925402,
0.002160701525829752,
0.0012492677834208962,
0.0006561865783782531,
0.0010855019254686186],
[0.0010330829521623256,
0.0008522233509922014,
0.1275791609121495,
0.16829345330854917,
0.04144536251756903,
0.0012296130189747858,
0.030186671043578833,
0.002623232410873358,
0.11133479723383606,
0.4068172031643503,
0.0008102166010262662,
0.0024319436718931832,
0.0014060937357943334,
0.0007385604988895497,
0.10321838557936107],
[0.0008990585208941215,
0.0007416622874385497,
0.1793082594873469,
0.0781800616175215,
0.001928421278226662,
0.0898344324868131,
0.20379915929583584,
0.05007909712300887,
0.0012986895485307885,
0.12188690161998224,
0.0007051051780477748,
0.0021164415461252823,
0.13095617495127193,
0.000642745200985689,
0.13762378985797077],
[0.0009510064990138774,
0.0007845158452267454,
0.023550060994893266,
0.2271483860559602,
0.0020398462678562173,
0.001131922630094234,
0.013343293329590729,
0.009637374027993865,
0.20360519517456163,
0.3239384922905847,
0.1668645513218797,
0.023906387582736464,
0.0012943822934685322,
0.0006798832880639103,
0.0011247023980760057],
[0.0009940855069110713,
0.0008200531042539346,
0.03216656143059756,
0.2751864388050974,
0.009681969860650168,
0.0011831968369176412,
0.2555388249716855,
0.3422616972258787,
0.0014359559785801486,
0.04417320915103616,
0.000779632050701391,
0.03253902901843139,
0.0013530156520209062,
0.0007106808668039562,
0.001175649540434075],
[0.0005215650098818798,
0.008352453149002436,
0.004993474374362835,
0.30282550940030317,
0.0011187225744042138,
0.0006207857026874088,
0.16576187369656004,
0.001324372099487177,
0.012636696473849358,
0.288569889056504,
0.12716420649314214,
0.08441087024604778,
0.0007098842272727663,
0.00037287161993664124,
0.000616825876558101],
[0.02624029925771955,
0.000689848916422238,
0.12232449304390741,
0.2060895760213916,
0.027197749561959396,
0.007346346886413628,
0.32293278064840925,
0.2625149393305331,
0.0012079616194548377,
0.018106556576528352,
0.0006558457282759823,
0.001968584532332045,
0.0011381901691575377,
0.000597842290143826,
0.000988985417351229],
[0.0778157184999253,
0.0005585877892955247,
0.0013403015435681544,
0.1925887069707034,
0.0014524030638733694,
0.10879996835390342,
0.05578378960854821,
0.1919945720619456,
0.011263261398982562,
0.17408107412368057,
0.0005310545639131844,
0.07873259846578998,
0.09863049732954073,
0.005626660187829785,
0.0008008060385001812],
[0.0011504221147629714,
0.0009490201998268809,
0.028488250080977346,
0.14372327313224315,
0.3432122285052579,
0.0013692743711124433,
0.09477461587223647,
0.15145090195716318,
0.0016617841243031894,
0.12101652491069936,
0.0009022422581049878,
0.011445209881814829,
0.0015658000412277777,
0.09692991241451311,
0.0013605401357564256],
[0.0009052395200287691,
0.0007467611924044905,
0.04304162539284028,
0.21621691984421065,
0.07069136549152055,
0.001077449102018444,
0.21895020939989138,
0.022923514794142036,
0.0013076179984471096,
0.39084864711816625,
0.0007099527540331673,
0.0021309920153785218,
0.008107059067507132,
0.021272069967330245,
0.0010705763420809937],
[0.02676499300057701,
0.00040764939712897445,
0.05351979670746567,
0.22311396158649804,
0.04609565483032587,
0.0005881685890247292,
0.16455842103009533,
0.008760739352811305,
0.14332690283696803,
0.288419850905842,
0.011646483992485268,
0.004916263014409488,
0.02694341761296666,
0.00035328032465324296,
0.0005844168187484878],
[0.015618915732273824,
0.0014991372852458651,
0.0035970997861762676,
0.28224164346788294,
0.0038979577212096147,
0.002162999548208462,
0.025497800535284786,
0.004614501011298432,
0.05783158875229517,
0.2601741394820693,
0.0014252436457056947,
0.004278006968370631,
0.319910939382046,
0.0012991941373186374,
0.015950832544614404],
[0.04659199405087785,
0.0004995065687835009,
0.01499448383837469,
0.12163367980889851,
0.10246904383144977,
0.0007207028290465576,
0.2522241188032623,
0.006136181521502719,
0.014670606715135308,
0.37180517323795276,
0.0004748855025843623,
0.006024063047462239,
0.0008241420004942215,
0.0004328863087473949,
0.060498531935427854],
[0.0011210384341754434,
0.1541746888971502,
0.0022189617557684563,
0.14005234339565512,
0.002404553563458652,
0.09498702254496594,
0.02424284534550697,
0.0028465713699064804,
0.0016193394135423829,
0.3392865415904959,
0.000879197500894119,
0.07075006685905671,
0.01003969071358837,
0.15405134892190583,
0.0013257896939294137],
[0.1576644747074071,
0.0005501009867621174,
0.0317065761334071,
0.07824497181954654,
0.0014303362406484033,
0.0007937019494784597,
0.23219163830210202,
0.03207990646371164,
0.016156574777483584,
0.4195937502678992,
0.020780744895054628,
0.001569793425750158,
0.005972058053009808,
0.0004767328409267923,
0.0007886391368124761],
[0.0011022692495805055,
0.21856410052072844,
0.010553148978829977,
0.30513426389140746,
0.01073563348279922,
0.0013119610742417826,
0.04057963237905079,
0.0027989121444279732,
0.00159222733651802,
0.2247785749736284,
0.00086447738097082,
0.027708828413189536,
0.009871599413116565,
0.14310077833641757,
0.001303592425092926],
[0.0008636753341790245,
0.11877993822141708,
0.014828149406945778,
0.06854391747103795,
0.05432695541346008,
0.0010279778916602042,
0.07771101128707536,
0.008752371558834522,
0.014366185739139661,
0.261394621502118,
0.1712192485860407,
0.1266599155052901,
0.0011755188434843004,
0.07932909254396185,
0.0010214206953553845],
[0.0015590726154938214,
0.0012861291400030272,
0.05044838166575416,
0.43158826671705436,
0.027025299784719785,
0.0018556651056197576,
0.03371548659491955,
0.003958839711088241,
0.0022520795522061833,
0.09296018260772174,
0.0012227348371537676,
0.0036701571479193203,
0.0021220002069590772,
0.3444918759996598,
0.0018438283137273742],
[0.0016571827731110384,
0.0013670633642256917,
0.028451609976410035,
0.1063476815487661,
0.0035545478378869,
0.0019724393945065254,
0.17427993258926644,
0.004207963699397883,
0.002393799621969243,
0.12398144533404881,
0.0012996797506908586,
0.21785813805157336,
0.0022555345739279824,
0.0011847351977866565,
0.32918824628643245],
[0.0007504582185372428,
0.0006190771190665769,
0.0014854424571634779,
0.2875370731570844,
0.12699781026538537,
0.01229214337226472,
0.05042568138906625,
0.12729371082104524,
0.0010840364919227855,
0.3582166184282257,
0.006288022638738362,
0.0017666268829350602,
0.001021422914638116,
0.02333435072299444,
0.0008875251209322889],
[0.0004850590418268004,
0.0004001408030105109,
0.030430908463734512,
0.24847516286053115,
0.11155588965873722,
0.011628881965686315,
0.19099817115154924,
0.0012316751496517834,
0.004384516523639755,
0.37520359999345565,
0.00038041755247570497,
0.019561105158815248,
0.0006601972075674119,
0.004030622167135011,
0.0005736523021837847],
[0.00683974720701079,
0.0006564937179665155,
0.0015752215862846236,
0.2988716859687597,
0.0017069715909664015,
0.13391370824544596,
0.023253716157107,
0.002020756174422491,
0.0192813503347903,
0.28920800947931014,
0.0006241346334228689,
0.001873400606997573,
0.01921495243559355,
0.20001868532792386,
0.0009411665339982622],
[0.0008362492699756928,
0.09595503637046168,
0.001655255602124119,
0.28865273848155915,
0.0017936995742155399,
0.000995334389477665,
0.0053821558016110185,
0.02752747694390249,
0.2806525114846372,
0.1832328814968634,
0.0006558457282759823,
0.014670609526203974,
0.09640337762319699,
0.000597842290143826,
0.000988985417351229],
[0.0020116321619637278,
0.0016594600641159762,
0.12620276895133278,
0.11381641550027059,
0.0043148184183138425,
0.002394317988271609,
0.028224621005380847,
0.0051079912556618555,
0.002905801572997215,
0.3338309049374761,
0.0015776639904568628,
0.2186222320556128,
0.01801558637057596,
0.0014381343240757961,
0.1398776514034941],
[0.0005114357720247591,
0.015958582937329335,
0.004896496838076778,
0.1493458897721273,
0.028286191489018973,
0.000608729514251293,
0.04213334237490215,
0.0012986516624316354,
0.0007387686968505462,
0.4616374712957669,
0.0004011040468587998,
0.0012039526804232708,
0.000696097669407827,
0.2916784386592038,
0.0006048465913267184],
[0.0007138416111568596,
0.016852981937112686,
0.1586327042595398,
0.2626647278134794,
0.0015311432128683495,
0.022535121708306908,
0.06965077612575217,
0.07771179092031828,
0.011873884512348989,
0.21604687592262736,
0.0005598449985570444,
0.017944540070647736,
0.0660280294477754,
0.07640951675991649,
0.0008442206995925524],
[0.0008751561641214331,
0.0007219444646414595,
0.13466219450186662,
0.1824455095132095,
0.04175613050233966,
0.020981131896402976,
0.12526949776708385,
0.0022222204030119585,
0.001264162607064205,
0.2715158346740094,
0.21337424301773605,
0.0020601738619330273,
0.0011911450069302773,
0.0006256571864117162,
0.0010349984332378482],
[0.001202974301423786,
0.0009923721886718289,
0.0023811440238398645,
0.15942482248313816,
0.002580300599029284,
0.0014318239009042941,
0.007742422442754645,
0.0030546251589357054,
0.5773156018120837,
0.12654465491145175,
0.0009434573937954013,
0.01196803606740607,
0.001637327018138394,
0.10135774701961668,
0.0014226906788103839],
[0.011494267619584591,
0.016762108340038002,
0.0014053453563455897,
0.3636990173019296,
0.0015228870780247333,
0.18956987391116895,
0.026138108996610024,
0.0018028322686521373,
0.006417721323265311,
0.24184260929751542,
0.0005568262373067808,
0.00706350550135256,
0.0009663463859062685,
0.0005075801496774745,
0.13025097023262258],
[0.0023323233750817186,
0.10820295265299981,
0.05775602774528284,
0.34451873637776526,
0.005002679936496245,
0.002776016369699105,
0.05043730273119972,
0.09448808587614485,
0.0033690398573836453,
0.24534444026968036,
0.0018291726850177448,
0.005490439137502385,
0.02088760261561953,
0.05480687146712441,
0.0027583089030024117],
[0.04295896216649929,
0.0009092973527507742,
0.060781180479132516,
0.07073678355666232,
0.08607768073325302,
0.0013119610742417826,
0.2833484512971798,
0.3041671011462432,
0.00159222733651802,
0.07409448047272078,
0.00086447738097082,
0.03608016699657329,
0.009871599413116565,
0.0007880224188936876,
0.026417608175244193],
[0.19504010823595305,
0.0014991372852458651,
0.0035970997861762676,
0.19943186231233867,
0.0038979577212096147,
0.002162999548208462,
0.24632388361673616,
0.03221776139647985,
0.0026250679819323237,
0.2877773998672507,
0.0014252436457056947,
0.01807963716096134,
0.002473444952459679,
0.0012991941373186374,
0.0021492023520236943],
[0.0009374647228724892,
0.0007733447985867396,
0.08729208909353992,
0.13847743635097157,
0.002010800050453078,
0.0011158047140947096,
0.15554744194777034,
0.002380435994943336,
0.001354167286574431,
0.31220609022136475,
0.20720674253089136,
0.07340392686840355,
0.0012759510469126957,
0.0006702021478206216,
0.015348102224800446],
[0.21539740344935204,
0.11236533898158489,
0.0011663573113470907,
0.2302469362682128,
0.11314315497791824,
0.0007013512238911706,
0.003792475773507429,
0.001496249009668905,
0.0008511766188072837,
0.21414122260217838,
0.013887643703827647,
0.010337480604716422,
0.08135506911279232,
0.00042126287036692566,
0.000696877491828458],
[0.0011605619939717737,
0.0009573849122828307,
0.02873934697053147,
0.2331305675480572,
0.1875844003186446,
0.0013813432252735521,
0.025097556048045092,
0.17922795097964328,
0.21321365554472319,
0.10445506870410827,
0.000910194667396204,
0.020360139561027633,
0.001579601082671125,
0.000829696437702287,
0.0013725320059215257],
[0.0009374647228724892,
0.09332954184907509,
0.0018555995084737437,
0.21679421847061556,
0.07320787470467488,
0.0011158047140947096,
0.013153292639326719,
0.13765487783796476,
0.001354167286574431,
0.31220609022136475,
0.0007352260336481023,
0.00932655967960393,
0.13655039288993415,
0.0006702021478206216,
0.001108687293956086],
[0.001093118385464064,
0.009203589571647767,
0.018767379474311428,
0.16146978969337267,
0.0687593953869129,
0.0013010693819252128,
0.0236390645987328,
0.0027756760206612387,
0.05969189634521428,
0.3806474791292176,
0.0008573006271531735,
0.04408247624571024,
0.22563751454968564,
0.00078148038201953,
0.0012927702079714852],
[0.0009649452340179333,
0.19866314013261108,
0.0019099938997825133,
0.21582083587055845,
0.002069743935859688,
0.0011485130210141044,
0.013538863633258904,
0.0024502152584121696,
0.0013938628701026083,
0.35800005006474556,
0.0007567781909924117,
0.031585191366958976,
0.13322477101151123,
0.037331908546849375,
0.0011411869633249955],
[0.0009308374326541273,
0.04318413120975794,
0.04425873505462825,
0.03852722923346549,
0.009065960529015604,
0.0011079166714975826,
0.020129682652888548,
0.06598798801285961,
0.0013445941694283886,
0.5079415043327898,
0.0007300284446805396,
0.0021912511470316134,
0.05075255992508008,
0.2127467316171473,
0.0011008495670750747],
[0.0011210384341754434,
0.0009247806567365862,
0.0022189617557684563,
0.19964952993359372,
0.002404553563458652,
0.0013343008424909545,
0.10938168325684786,
0.0028465713699064804,
0.3677163424323082,
0.16900886576781415,
0.000879197500894119,
0.12183336960586125,
0.018553574504722458,
0.0008014406814922261,
0.0013257896939294137],
[0.0008693778474167097,
0.007319789875191937,
0.21960702995080628,
0.06239387424212851,
0.001864758189611456,
0.014239989490616443,
0.005595373524693122,
0.0022075479436238763,
0.15311589481778573,
0.4017753635271456,
0.0006818274980408546,
0.028457019852321803,
0.06060678950692718,
0.0006215262146835991,
0.040643837519006895],
[0.0015408282903797686,
0.0012710788094823797,
0.0030498856634416853,
0.13398688504070913,
0.003304975140335589,
0.0018339500442728512,
0.05672502032207481,
0.003912513223119854,
0.09584202000194716,
0.1971906902937581,
0.0012084263490977152,
0.2025618343649251,
0.1191175364418666,
0.001101552308518981,
0.1773528037060703],
[0.0008470079865913857,
0.0006987241277568265,
0.0016765511973361894,
0.028624819277087266,
0.12403847684913842,
0.07820079277710461,
0.23059663744672085,
0.002150745782847831,
0.026954386903339404,
0.41716821244771785,
0.03926060995886391,
0.04702295880896103,
0.0011528334889479269,
0.0006055337955495302,
0.0010017091520369934],
[0.0045548322700658395,
0.044710756318961924,
0.0332479590601479,
0.37612363871571874,
0.04138543676802887,
0.0006307800305215841,
0.03158496253096575,
0.0013456938033850113,
0.23018313877113641,
0.16441986391021243,
0.0004156335728703945,
0.0012475644612665018,
0.04097001633555827,
0.02855296699791498,
0.0006267564532454131],
[0.0007296647423405847,
0.006143465248326144,
0.0014442842532667458,
0.17428079488043885,
0.007106624122981601,
0.000868473596230528,
0.049028501399875704,
0.001852784616349593,
0.0010540003267715252,
0.1986696413508512,
0.0005722546153631652,
0.2566285791819153,
0.30023635379849833,
0.0005216440315825682,
0.0008629338352081978],
[0.07013711637292043,
0.0007519306923320339,
0.01564933956052609,
0.03080454691896718,
0.08502585283868146,
0.0010849078091814146,
0.08893724606005914,
0.06461757039051577,
0.029006914174670117,
0.5250829802568706,
0.0007148674452997505,
0.07829391524243107,
0.0012406196509502168,
0.0006516440867438675,
0.008000548499850827],
[0.02477891586364398,
0.0004389493587546723,
0.07379367236330063,
0.28065635614060686,
0.001141327121283073,
0.0006333290980198898,
0.03171260164052398,
0.03772135035375977,
0.03713884175957028,
0.14083749455973607,
0.0004173132044068154,
0.0012526060381496922,
0.0007242279186052627,
0.36812372531871573,
0.0006292892609232341],
[0.0011504221147629714,
0.035897189316069,
0.0022771232437957586,
0.10003806173694048,
0.011204621900957792,
0.0013692743711124433,
0.02487827763975223,
0.07281752144561843,
0.08029516463584796,
0.4093389201196968,
0.009639284537165518,
0.002708167602754301,
0.23746594157586207,
0.0008224473448472961,
0.010097582414816956],
[0.03350986892472823,
0.0011622712792675454,
0.00278880781051779,
0.4970282316550538,
0.013722374121131432,
0.08727946438197771,
0.0946704690109938,
0.014277905422112663,
0.002035197944546067,
0.19101098636291644,
0.0011049820264397076,
0.0033167106713833374,
0.0019176456068342547,
0.05450882233123588,
0.0016662624508613439],
[0.0011022692495805055,
0.07625134460320458,
0.07752385764590003,
0.2130495394741862,
0.027478310649566733,
0.034797315407776815,
0.015465616628899517,
0.1116263137284168,
0.00159222733651802,
0.2415212521403959,
0.009235815964354577,
0.061194182746724564,
0.08521364666357037,
0.0007880224188936876,
0.04316028534201171],
[0.0005788911327039284,
0.031252835830348005,
0.0011458458916873034,
0.23059431127570623,
0.0012416833299936628,
0.0006890173454628505,
0.12243047143335299,
0.0806063959432206,
0.062386787764871145,
0.2103753607684833,
0.0004540073039845546,
0.07610273673596397,
0.18104317836420636,
0.0004138545920999484,
0.0006846222879151352],
[0.0008751561641214331,
0.0007219444646414595,
0.06155073446084258,
0.13592003494164875,
0.0018771522981447242,
0.020981131896402976,
0.09203701593025473,
0.015515213137743603,
0.007910658974430027,
0.4376782438581549,
0.17349526481354113,
0.048585648433493785,
0.0011911450069302773,
0.0006256571864117162,
0.0010349984332378482],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.2897476887972528,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.04090441397296385,
0.0008658094484983401,
0.21729371650916737,
0.32242485257340514,
0.042106082193561704,
0.08095893878076788,
0.13429054562386744,
0.07440380284199191,
0.009487050070134131,
0.05460890548124428,
0.0008231330292487732,
0.018412658251162103,
0.0014285096043281298,
0.0007503345894966817,
0.001241247030162278],
[0.0011920832414871007,
0.0009833878030756174,
0.0385733606929157,
0.11271426107382117,
0.0025569399931996894,
0.001418860964036008,
0.08009987530237263,
0.0030269702824759995,
0.001721963599386564,
0.5871246113526465,
0.0009349158555336334,
0.002806240572876033,
0.0016225035703977446,
0.16381421526672277,
0.0014098104290528048],
[0.001111574617060615,
0.0009169736496439852,
0.0022002292595388892,
0.3499202577632473,
0.010826263802684524,
0.0013230366620984384,
0.024038187014714146,
0.3151768922079584,
0.001605668934824255,
0.1844661103233865,
0.10217588934635256,
0.0026167180961988138,
0.0015129260459147804,
0.0007946749116427698,
0.0013145973647339377],
[0.0006771317891435346,
0.06741202960530639,
0.24304120657068437,
0.08459468557560895,
0.006594975511258821,
0.0676593887748198,
0.024928354924235504,
0.006861963956069339,
0.0009781165042116581,
0.23064937104492053,
0.08281221372208042,
0.18158404741349904,
0.0009216208292171398,
0.000484087740444334,
0.0008008060385001812],
[0.00061976265113767,
0.01463188556351203,
0.0012267461833631772,
0.08213438185866509,
0.08134621609666605,
0.0007376641177924166,
0.15931569118754346,
0.08629745875546752,
0.00089524681530382,
0.5358822322660136,
0.0004860616348346152,
0.0014589611166801754,
0.03379165898120764,
0.00044307401633070914,
0.0007329587554819737],
[0.04810844415214381,
0.0008522233509922014,
0.002044864378865548,
0.22321470804186092,
0.017907681917578282,
0.0012296130189747858,
0.13218328697687204,
0.026160913010864102,
0.001492287767212593,
0.28128290663106637,
0.0008102166010262662,
0.2064251755384796,
0.0014060937357943334,
0.05565981523220128,
0.001221769646067847],
[0.0005763571955909842,
0.000475455586182045,
0.0011408302656625978,
0.08075927594128118,
0.04938573031985834,
0.07072161171449579,
0.11751733998672038,
0.12840304567756078,
0.009586998952355652,
0.19632282340254392,
0.2805944614252847,
0.05826071529655244,
0.005161685637068046,
0.0004120430571652428,
0.0006816255416779041],
[0.0009720689558830165,
0.08200954683232053,
0.03883666531547821,
0.3133868204824508,
0.0020850238497980298,
0.0011569919346681604,
0.05055138534456322,
0.16488361578539676,
0.0014041530824945925,
0.3384954479926319,
0.0007623651167122712,
0.0022883128029123297,
0.0013230496803440454,
0.0006949410320916781,
0.0011496117922543388],
[0.00427925699741562,
0.0004107323343014856,
0.004766888962332883,
0.22101995073701244,
0.01997475247890526,
0.061094355622811465,
0.037236734735474966,
0.1789881349511806,
0.0007192138508797903,
0.3359773928800327,
0.004171845699036158,
0.05789246484291985,
0.06117941118564497,
0.011700028124082707,
0.0005888365979691852],
[0.0009579251636902121,
0.0007902232742212089,
0.009171195664616579,
0.33065227281766446,
0.0020546863476382207,
0.05934093472538043,
0.03526565855301199,
0.25706079016513783,
0.0013837223823004396,
0.12259245592594707,
0.008026369712149777,
0.009530114563005608,
0.16135593648290322,
0.0006848295050393313,
0.0011328847172936201],
[0.0012368751592449117,
0.0010203380965336888,
0.011841868789039705,
0.17331117413733643,
0.012046637769025844,
0.2644935917328942,
0.02674785474177682,
0.003140707141807118,
0.001786665500429522,
0.4870684313338777,
0.0009700448403680278,
0.002911683626325967,
0.011077090375829051,
0.0008842534210481058,
0.0014627833344628845],
[0.019356153560883663,
0.0005088669694248937,
0.17924429578406284,
0.1426523035308252,
0.04817135824419317,
0.0007342082915266417,
0.28974438599714875,
0.01562081642767438,
0.0008910527418982475,
0.2944457150864293,
0.0004837845218180923,
0.0014521261412134868,
0.0008395858400593594,
0.005125821889833399,
0.0007295249730085836],
[0.001111574617060615,
0.08533706868224085,
0.019084248266058264,
0.05444992514915833,
0.0868043493320217,
0.0013230366620984384,
0.10001627254405132,
0.07035861661342752,
0.08602576396742112,
0.47993644293747556,
0.0008717753072363287,
0.0026167180961988138,
0.0015129260459147804,
0.009236684414902456,
0.0013145973647339377],
[0.0015055913203197073,
0.0012420106996655033,
0.002980138287643687,
0.16522603318849396,
0.003229394161717557,
0.013226434396245946,
0.0439933596982029,
0.17533940873660675,
0.002174825850171929,
0.34132868693299134,
0.0011807910289593172,
0.026413108100045747,
0.024918058010884786,
0.19546158062224792,
0.0017805789658029295],
[0.00047628620944647045,
0.00039290381144733684,
0.044349421599174925,
0.15716787331236756,
0.037193827451856405,
0.0005668932225313793,
0.18030931176431322,
0.35207998066280444,
0.000687995172663873,
0.15861872094984653,
0.00037353727783978467,
0.0011212083508385434,
0.02958603674299026,
0.0003405013892899141,
0.03673550208258947],
[0.006413365805702347,
0.0006155687096372843,
0.007144184770643348,
0.20656730663686226,
0.05260500607763109,
0.000888160713458837,
0.06714139261970717,
0.013229105836681554,
0.0010778930830762535,
0.2088403729244947,
0.14226424070996885,
0.035759578451023226,
0.0010156343469869401,
0.2555556939405502,
0.000882495373575921],
[0.03955448314680535,
0.0010398744721037425,
0.0024951232139097035,
0.023453854400430643,
0.0027038128979366832,
0.03979428718320166,
0.209156141449606,
0.022347804937047022,
0.040114800303196266,
0.5921291264282749,
0.000988618252833566,
0.012540914920609492,
0.0017157016168768315,
0.0009011841884000138,
0.011064272588768131],
[0.0016365851910038658,
0.001350071756331117,
0.003239425017185679,
0.5524797936800527,
0.1402324036321185,
0.014377199423601736,
0.022962446928672556,
0.1160191459619937,
0.0023640464257245904,
0.11001116970552252,
0.0012835256723283202,
0.0038526267328730128,
0.0022274999121297354,
0.0260285618425349,
0.0019354981179270216],
[0.0011306647827424405,
0.0009327217412510619,
0.002238015963605295,
0.2700598585147108,
0.011012193893689046,
0.10438966783705984,
0.033038010922323946,
0.0028710148567595507,
0.027394222009683625,
0.34219999256048284,
0.0008867471631936678,
0.04559661980796802,
0.1561047730599416,
0.0008083226465708725,
0.00133717424001742],
[0.0008751561641214331,
0.0007219444646414595,
0.16789467633869573,
0.11598054583955128,
0.0018771522981447242,
0.22037602291737765,
0.08539051956288891,
0.028808205872475248,
0.04114314081125914,
0.31139481287820436,
0.0006863592620297532,
0.021999662964030494,
0.0011911450069302773,
0.0006256571864117162,
0.0010349984332378482],
[0.0005999939768011625,
0.0004949543271567606,
0.15611672547905753,
0.26178406758963835,
0.014957163042229224,
0.12374607424154857,
0.16334744858241815,
0.028863952076247486,
0.0008666909758868278,
0.1679202413081028,
0.00047055764447818195,
0.05609328639604373,
0.0008166312013266916,
0.00042894120932833213,
0.023493271949736258],
[0.036719261834824435,
0.0004904843096808544,
0.19083149504406816,
0.23684192167948687,
0.0644935262823258,
0.0007076852471646933,
0.08962285672628936,
0.09182147815777823,
0.08665499419411779,
0.1980128254825381,
0.000466307957630667,
0.0013996685395941068,
0.0008092560647918323,
0.00042506736764914485,
0.0007031711120599107],
[0.0006226935917094316,
0.0005136799695099392,
0.11000262763883084,
0.28587556462610997,
0.0013356367179231357,
0.0007411526301885763,
0.1553399825122647,
0.06778903534065911,
0.1758774353536435,
0.14589837629097602,
0.024134029852458695,
0.006194994652587973,
0.024493196434861027,
0.0004451693726874906,
0.0007364250155896561],
[0.0017925454897304558,
0.015092466831928357,
0.2758228980011302,
0.2783992795654371,
0.003844891944461684,
0.0021335530381792335,
0.01153694173925541,
0.018165418811163927,
0.0025893309931190804,
0.1613360279395709,
0.16477070166582874,
0.017833505710112905,
0.04328098734847792,
0.0012815072481899945,
0.0021199436734141105],
[0.000702417416305115,
0.0005794467163221277,
0.0600710360489051,
0.35448402645736693,
0.012175854454118135,
0.0008360428347361696,
0.07387070512045425,
0.023122028235548285,
0.06502993389703646,
0.2339277307161573,
0.0005508853382481907,
0.0016535357466070865,
0.02762907469402202,
0.14453657238329665,
0.0008307099408762157],
[0.0009792986419858353,
0.0008078549438343119,
0.0019384047574076964,
0.10746982115162554,
0.04672505727772098,
0.0011655969708237956,
0.27404998841981143,
0.2702338191895,
0.001414596360170835,
0.2740761918172646,
0.0007680351470699035,
0.017180174024586728,
0.0013328897578708844,
0.0007001096011438889,
0.0011581619391835224],
[0.0017450326536059197,
0.001439533555881558,
0.01670697932781375,
0.03246796736521093,
0.0037429800421304197,
0.1611117475799553,
0.0642427279902049,
0.08394840753853157,
0.02902648973574826,
0.23657706192717315,
0.21341490590067413,
0.004107919030413992,
0.14815695457830674,
0.0012475398848932268,
0.002063752889456199],
[0.0005616074990990596,
0.00046328808719839056,
0.06082453308769075,
0.10854899480670427,
0.0012046110749244876,
0.00493365274030415,
0.0846534762528791,
0.12938225926349103,
0.0008112417295496119,
0.3917634077985007,
0.0004404522580481081,
0.0013220601507971746,
0.022090419715035517,
0.0004014983635598125,
0.1925984971722178],
[0.001678305433982833,
0.014130614392407892,
0.00332200525778179,
0.12044932879180366,
0.0035998545534468886,
0.19318947434500464,
0.010801685158599373,
0.004261598936032851,
0.0024243112942061943,
0.329499748745761,
0.02680849816475495,
0.22063498538723875,
0.002284283902404435,
0.001199835982209335,
0.06571546965436544],
[0.0009940855069110713,
0.0008200531042539346,
0.00951739555115118,
0.15439088744805005,
0.017231691820465626,
0.0087329187967331,
0.13474327361463811,
0.34981141918569414,
0.05428400969728837,
0.24046598010623813,
0.000779632050701391,
0.024989307058615934,
0.0013530156520209062,
0.0007106808668039562,
0.001175649540434075],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.13048362584298057,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.13668744403732208],
[0.03974067871158025,
0.0006052780853678051,
0.4138114966677123,
0.19754164542016653,
0.001573804086896194,
0.012018155374000847,
0.12174318365890745,
0.024152793666799746,
0.0010598736604724888,
0.16634218542847407,
0.0061478645976506575,
0.007299670465561507,
0.0009986557200743103,
0.0005245508518109689,
0.006440163604524898],
[0.0011813876153939375,
0.054807849365584534,
0.0023384157557682643,
0.12067517871033775,
0.0025339985800851123,
0.037294987125286505,
0.03452013146302633,
0.020944239899776652,
0.037595370258891925,
0.12427388343188152,
0.0009265275902922873,
0.5321416955445979,
0.028524588485172635,
0.0008445848658919542,
0.0013971613080127504],
[0.0010841182084065403,
0.0008943239751522991,
0.0021458825762345353,
0.13543986626730867,
0.05995977778072791,
0.03422430885338878,
0.06461187240832902,
0.010986310478221,
0.0015660081673318615,
0.38574691064824207,
0.0008502420527677204,
0.002552083945437319,
0.2896476344130684,
0.0007750460727089507,
0.009515614152674923],
[0.0007910344432636261,
0.0006525497517671285,
0.03160386898493055,
0.14087800198842143,
0.0016967167506323026,
0.2953150032535746,
0.11923597096807168,
0.002008616234875451,
0.03118075956138959,
0.31150104562427505,
0.0006203850683764411,
0.013877390217914487,
0.013091894114842142,
0.000565517794899981,
0.03698124524276549],
[0.0004941611164994315,
0.004160625380568628,
0.0835436045749829,
0.31318538518904976,
0.012318870979368984,
0.214507799645085,
0.12702866119569878,
0.008760739352811305,
0.0007138154662611723,
0.1345478355848162,
0.00038755604216630604,
0.09874066260040083,
0.000672585728889084,
0.00035328032465324296,
0.0005844168187484878],
[0.001214066201136415,
0.0010015222534581858,
0.011623495344382275,
0.14245399005686898,
0.057926469367926094,
0.27805691275951805,
0.07235658424959772,
0.003082790013232665,
0.0017537179727436468,
0.2844581826662045,
0.0009521564447915292,
0.06740076347701589,
0.02931361250949405,
0.0008679470872299913,
0.04753778959640006],
[0.00221463864662537,
0.018646310796791053,
0.004383612827086564,
0.22621865252178142,
0.05520840641401145,
0.13719101695109145,
0.19926678701353742,
0.005623470858792709,
0.003199044330600865,
0.23296481309623984,
0.0017368760107932535,
0.022032785877232643,
0.0030142686233957717,
0.0015832655260530385,
0.08671605050596715],
[0.014997928157998603,
0.001439533555881558,
0.01670697932781375,
0.15174402690474506,
0.0169958755465231,
0.3333993891370602,
0.03773693698141954,
0.004431034512175474,
0.0025206987269628953,
0.3956118079798854,
0.001368577830391183,
0.004107919030413992,
0.015627999534379915,
0.0012475398848932268,
0.002063752889456199],
[0.0006736674057042582,
0.041485823515411684,
0.026914752714756313,
0.11997570893219686,
0.006561233890632005,
0.11847584264460938,
0.09642847874967048,
0.022175641455473676,
0.0009731122042021137,
0.3471433276228589,
0.05169095455907315,
0.10902735209321672,
0.0571957842860809,
0.0004816110208780096,
0.0007967089052355302],
[0.0012253645477922296,
0.0010108426229672714,
0.002425462843676183,
0.19961691447737695,
0.1515275759494639,
0.0014584735889811961,
0.06372374620969387,
0.0031114790832388836,
0.02968864775605425,
0.3522488300547157,
0.000961017406058512,
0.1890086490915093,
0.0016678016137124127,
0.0008760243791118095,
0.0014491703756475908],
[0.0007138416111568596,
0.00601024124707442,
0.09357626011931021,
0.10544498780792458,
0.0015311432128683495,
0.109277047228613,
0.10760036854088609,
0.023498087470126976,
0.006452514167329858,
0.42748031937837344,
0.03308806706867183,
0.07757961386585817,
0.0009715853075458372,
0.0005103319296486564,
0.006265591044611684],
[0.0006408783900829178,
0.0005286811945079246,
0.025604746857658216,
0.07033103387388247,
0.0013746419120330999,
0.0007627968406371838,
0.09173507237540829,
0.09897215424795958,
0.0009257484888216081,
0.37405219379842447,
0.0005026220883786094,
0.3130120876722936,
0.0008722775724983166,
0.019927133524010706,
0.0007579311634029426],
[0.0006316551368978868,
0.010115459889575722,
0.03962783495220528,
0.22762624878995308,
0.04932679490796631,
0.0007518189882102542,
0.05203731064884517,
0.08315620760538903,
0.010506812757808542,
0.306305474252038,
0.0004953885619417059,
0.0014869567925618686,
0.2167334373171239,
0.00045157606371975796,
0.0007470233357634649],
[0.0007177327059646266,
0.0005920807912990945,
0.0014206662116550293,
0.3513112416813084,
0.0015394893548029507,
0.000854271651226935,
0.07548135887135823,
0.0018224864641212372,
0.12095704434597002,
0.14636254700935664,
0.006013818480748031,
0.0016895889228720723,
0.26262112826521666,
0.0005131137090947655,
0.02810343153500536],
[0.0005999939768011625,
0.0004949543271567606,
0.001187616452412234,
0.07040105055672356,
0.00128694753987817,
0.07362195073292804,
0.12233680207536496,
0.001523521071545376,
0.0008666909758868278,
0.32740608883553174,
0.00047055764447818195,
0.3978486739548201,
0.0008166312013266916,
0.00042894120932833213,
0.0007095794458178352],
[0.0027746543819977022,
0.02336140391988709,
0.00549209712301863,
0.30449507168333256,
0.027023953083973366,
0.003302494871412546,
0.017857859750321146,
0.007045478089241082,
0.004007986758305725,
0.3550921386430897,
0.002176079895326334,
0.0486767193347054,
0.02484898910076085,
0.14949114193779037,
0.024353931426837516],
[0.11916977316209376,
0.00044433622730088746,
0.001066161027375328,
0.27182843653726213,
0.0011553337009669454,
0.0006411014310452877,
0.3184528110010509,
0.0013677132777737578,
0.0007780560292774427,
0.1998360838853515,
0.00042243455002426226,
0.029903080892278007,
0.000733115778940892,
0.0003850741537743277,
0.05381648834548459],
[0.0012605575618978842,
0.12549513262305298,
0.0024951232139097035,
0.3872461474570515,
0.0027038128979366832,
0.06851473137188226,
0.0751274019024299,
0.08936217471063508,
0.0018208747182888068,
0.21876335197542718,
0.000988618252833566,
0.012540914920609492,
0.0017157016168768315,
0.010474665584626877,
0.0014907911925412668],
[0.0006316551368978868,
0.010115459889575722,
0.14516609473782566,
0.14607395713742824,
0.0013548586417752339,
0.0007518189882102542,
0.17196715131432289,
0.02079269045934062,
0.0009124255045703262,
0.33508863601175265,
0.0004953885619417059,
0.0014869567925618686,
0.0056569177458831305,
0.15875896574215034,
0.0007470233357634649],
[0.15931496287994695,
0.0004107323343014856,
0.0009855302822711434,
0.1416114184557159,
0.13341551288075745,
0.0005926167418236258,
0.23764874477874717,
0.06176601586926667,
0.0007192138508797903,
0.2603502192787979,
0.0003904870189744179,
0.0011720846419937558,
0.0006776723046571248,
0.0003559520838974881,
0.0005888365979691852],
[0.07014795931291931,
0.0005391784399682883,
0.02611314268392831,
0.21071620573096309,
0.14039064804125326,
0.015669590278373606,
0.1084481685836928,
0.0711540034484925,
0.060510720523874455,
0.2722737819735179,
0.0005126019164684316,
0.0015386243448686217,
0.02074512737554605,
0.00046726705757339917,
0.0007729802885599384],
[0.0005278374586953719,
0.052548994099610204,
0.0010447912385524337,
0.21025769506065256,
0.03721079779782461,
0.0006282514000990055,
0.015423403993614838,
0.009357770657954581,
0.0047711966170754195,
0.3882499469229878,
0.22490316619754885,
0.0012425633050817096,
0.048823249754095036,
0.004386091543926824,
0.0006242439522806736],
[0.0006503749853043746,
0.000536515241314821,
0.0012873396444791508,
0.23931158652242096,
0.006334375626704574,
0.28231785622899447,
0.13754868845088852,
0.0016514499025472706,
0.02069692289053332,
0.1820203680298892,
0.02026752656397844,
0.09043957910661193,
0.015703295493470507,
0.00046495905542366135,
0.0007691622574387928],
[0.0009441870585218019,
0.0007788902694529082,
0.1381133681296424,
0.2183487980380732,
0.1310989203902637,
0.0011238058832312363,
0.04910141769657474,
0.009568266720365655,
0.0013638776967942196,
0.2570787560365421,
0.015082020538715608,
0.17432094552152314,
0.0012851005871568951,
0.0006750080073699129,
0.0011166374257725283],
[0.001408929528862659,
0.0011622712792675454,
0.00278880781051779,
0.30442259527986043,
0.0030220609891762422,
0.0016769593263361905,
0.08397015587903861,
0.0035775922901574724,
0.03413613734041164,
0.45851881466179617,
0.0011049820264397076,
0.0033167106713833374,
0.0019176456068342547,
0.0010072566714599313,
0.09796908063845806],
[0.0009115060958508604,
0.028442174803159866,
0.0018042175051121735,
0.15541064541769242,
0.0019551205061979675,
0.0010849078091814146,
0.1443177342817148,
0.19614622991694794,
0.0013166700638422876,
0.1928000509269367,
0.08378559977778324,
0.14059696449179368,
0.04969854684489892,
0.0006516440867438675,
0.0010779874721438688],
[0.0012727421350499791,
0.03004798246187727,
0.16684156153524146,
0.48764946449554825,
0.002729948004369178,
0.0015148641217818857,
0.037189509393839165,
0.01289780039293416,
0.001838475327635346,
0.18221384726746978,
0.05899428734255234,
0.012662135640601289,
0.0017322856210428364,
0.0009098950517504658,
0.0015052012083065678],
[0.0011022692495805055,
0.19345008477057718,
0.0021818103954462215,
0.17956418514065117,
0.0023642948994154634,
0.0013119610742417826,
0.007094278045515762,
0.01117025072781173,
0.009963565919901777,
0.5512607797255948,
0.00086447738097082,
0.002594812663038266,
0.0015002608297328079,
0.0007880224188936876,
0.03478894675862795],
[0.024673295196390863,
0.0006486528902976949,
0.08516085407446118,
0.42668052964231806,
0.01363007669391497,
0.0009358955463694727,
0.26781757782982973,
0.0019966212886029745,
0.013079317522931453,
0.02299702513332344,
0.0006166802862312924,
0.001851025661263696,
0.0010702203415226814,
0.13791230217380082,
0.0009299257187417208],
[0.08982608430062979,
0.0006448022946753411,
0.0015471686410109139,
0.33510318619190815,
0.0016765723245761393,
0.11965626177558944,
0.022839593323566915,
0.03166624924901129,
0.013001674821340988,
0.337484201235831,
0.0006130194894537365,
0.043394110118656744,
0.0010638671966841067,
0.0005588036327402911,
0.0009244054043250876],
[0.001170882209499363,
0.0009658983899677301,
0.0023176215588895186,
0.386374963449332,
0.0025114651767607576,
0.001393626722300377,
0.1320298863874113,
0.020757994726360706,
0.028368626744007336,
0.05202935197411505,
0.24990631006549224,
0.0027563319808580683,
0.046055794280173604,
0.018621933137244237,
0.05473931319758772],
[0.00817355888471583,
0.0007845158452267454,
0.05244027053770107,
0.14047775742753676,
0.24038407499602057,
0.001131922630094234,
0.049456055258100484,
0.07464034549931142,
0.0013737283749070063,
0.3239384922905847,
0.0007458464507348127,
0.0022387304256306097,
0.0012943822934685322,
0.0006798832880639103,
0.10224043579790332],
[0.0008929613573754144,
0.0007366325411683359,
0.008549230816615216,
0.1251219091508644,
0.008697063671814949,
0.0010628351848919876,
0.07356436263522509,
0.19893732365361244,
0.0012898821991164777,
0.5144000823683141,
0.0007003233518723565,
0.06313757209902686,
0.0012153790441357395,
0.0006383862827393256,
0.0010560556432271852],
[0.0007061846342879855,
0.0005825544155892195,
0.02285068161867955,
0.2813001446688414,
0.001514719502089054,
0.15101064076946621,
0.025997924610567574,
0.3074966097535176,
0.0010200833233533736,
0.2030030260617089,
0.0005538398566080356,
0.001662404019880697,
0.0009611636592281382,
0.0005048578865000159,
0.0008351652196821995],
[0.23235025534892464,
0.0005136799695099392,
0.0012325476303420694,
0.05887713678230729,
0.03916870802522358,
0.0007411526301885763,
0.32558880339511664,
0.07251816925407166,
0.0008994805573789479,
0.25939759021287734,
0.0004883602853959203,
0.001465860739175418,
0.0008475268677982508,
0.0004451693726874906,
0.005465558929002212],
[0.0004997881957862085,
0.0004122913557984485,
0.08829063849593595,
0.3623002089391571,
0.0010720127432885323,
0.0005948661440810214,
0.0108080932522772,
0.11514042463424774,
0.0007219437792559965,
0.18542420108984775,
0.00039196919509259815,
0.00497224516446755,
0.04243307245217498,
0.0003573031753610532,
0.18658094138322798],
[0.0005866284153712822,
0.00048392864569930984,
0.02343732025844636,
0.2381315965160155,
0.001258279291418444,
0.0006982265415679193,
0.1552534629222455,
0.0014895828733987556,
0.11668341299429294,
0.4314935353287646,
0.00046007542741143107,
0.005836192863820354,
0.0007984397945644435,
0.022695545385964223,
0.0006937727410188608],
[0.0027746543819977022,
0.065506408495515,
0.00549209712301863,
0.2202050625320767,
0.005951450796159407,
0.003302494871412546,
0.017857859750321146,
0.02811798037705504,
0.04615299133393364,
0.3972371432187176,
0.002176079895326334,
0.006531714759077486,
0.1934290074032725,
0.001983625923092674,
0.003281429139023555],
[0.05552326789656315,
0.007569688459397179,
0.00860760518838118,
0.05086795692968699,
0.08386473534173021,
0.0010700922513509085,
0.019442452652952826,
0.002282913919298463,
0.0012986895485307885,
0.39500794849832743,
0.0007051051780477748,
0.29572156694034635,
0.0012236776840579484,
0.000642745200985689,
0.07617155431034311],
[0.0006771317891435346,
0.18054862344778633,
0.05276602601742267,
0.3622935977344234,
0.0014524030638733694,
0.0008059469588089319,
0.004358065134693697,
0.06857283332469476,
0.00612068895159711,
0.15351078433413878,
0.0622419239325386,
0.08901774336056088,
0.016349338171373497,
0.000484087740444334,
0.0008008060385001812],
[0.0010412525084852137,
0.10366216156737794,
0.0020610350403606806,
0.3910465820914583,
0.00223341801084631,
0.0012393367229550374,
0.030425385377964888,
0.08963129890597647,
0.04104378056639312,
0.26769139777110634,
0.0008166237439783096,
0.018267052084375488,
0.0014172130384077278,
0.048192031245175605,
0.0012314313251384913],
[0.15799643437619731,
0.001541693039653441,
0.10305311304725824,
0.2618667594294565,
0.10336251138517588,
0.0022244002474393933,
0.012028187535189713,
0.004745492064408101,
0.1872139766302825,
0.08304526711018956,
0.015659116508687603,
0.03278627545191145,
0.0025436582124282407,
0.0013360741396904192,
0.030597040822031678],
[0.0009052395200287691,
0.0007467611924044905,
0.04304162539284028,
0.18871704529321637,
0.0019416791140348373,
0.03545229229076131,
0.012701150267434217,
0.0022986088808963196,
0.0013076179984471096,
0.5145980825976405,
0.12445938823350747,
0.0021309920153785218,
0.0012320904297585596,
0.000647164054084529,
0.06982026271956672],
[0.0006841685619354881,
0.000564392649472897,
0.1572346561864892,
0.21537415111101024,
0.02744756750964635,
0.000814322382504041,
0.014795382684144612,
0.16800971407804,
0.09971255103980689,
0.2746143995131878,
0.0005365732980300889,
0.037982676215069525,
0.0009311983390601434,
0.0004891183939972939,
0.000809128037605374],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.13048362584298057,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.002417983623875596,
0.11217696926815435,
0.004786109935116351,
0.2286260119836499,
0.005186415525040604,
0.0028779723229021936,
0.03392601921540396,
0.07959467451481778,
0.021856492176386272,
0.29108274385454874,
0.0018963535009198682,
0.005692088869066567,
0.0032910344901815177,
0.2037295162135146,
0.0028596145064216953],
[0.0710467603472472,
0.0009490201998268809,
0.1420697997087642,
0.07382693489975889,
0.0024675796218972634,
0.0013692743711124433,
0.016141235360691703,
0.002921183213134189,
0.01913586868242425,
0.5666056811427863,
0.0009022422581049878,
0.07260450583523853,
0.0015658000412277777,
0.027033574182028883,
0.0013605401357564256],
[0.012289465482125358,
0.00062621529753551,
0.16869270624518515,
0.1755489353153357,
0.018923774964412433,
0.018199053246655012,
0.31044007718335687,
0.053814150001806914,
0.0010965358166561048,
0.22398273235016306,
0.006360525767071843,
0.0017869967166601686,
0.0010332002826467057,
0.0005426956231856716,
0.006662935707203508],
[0.000695002287208886,
0.0005733297378614908,
0.0013756740611863534,
0.20822798947265708,
0.0014907341045351712,
0.0008272170775643782,
0.1892133167399099,
0.012321353673021654,
0.0010039304290287332,
0.34230239430065257,
0.005823362324504329,
0.017470957418411398,
0.0009459437505591494,
0.1641239296010072,
0.053604865021891684],
[0.13441327301877654,
0.0008522233509922014,
0.002044864378865548,
0.18398524037520966,
0.049291256050899276,
0.056150867752286525,
0.03803256457690908,
0.002623232410873358,
0.001492287767212593,
0.3283582678310479,
0.0008102166010262662,
0.18288749493848888,
0.017097880802454828,
0.0007385604988895497,
0.001221769646067847],
[0.0006316551368978868,
0.0005210726363375042,
0.006047479565871522,
0.48187751100076587,
0.12608189293387206,
0.05831814250763956,
0.004065374382654087,
0.14551972475143743,
0.0009124255045703262,
0.07124298654770171,
0.0004953885619417059,
0.015878537672419195,
0.01045411137250224,
0.043626318703291735,
0.03432737872209722],
[0.0009792986419858353,
0.008245275981780618,
0.0019384047574076964,
0.10003240011367924,
0.0021005310500431377,
0.23172564914715932,
0.05092735728142221,
0.002486661823432993,
0.008852017398117142,
0.549260770221278,
0.0007680351470699035,
0.002305331948694112,
0.03108257390965611,
0.008137530639090194,
0.0011581619391835224],
[0.0010579854763849024,
0.0008727662440839018,
0.0020941559528093435,
0.14021009971931425,
0.002269309124267439,
0.0012592529118075194,
0.24785984224379404,
0.002686465579629547,
0.009563278700073875,
0.4407286130905432,
0.0008297469189841413,
0.0024905658145493005,
0.0014399877064977917,
0.000756363542367268,
0.1458815669748935],
[0.0006841685619354881,
0.005760406855846186,
0.03772632943990359,
0.11145386698354448,
0.0014674964777799016,
0.000814322382504041,
0.16547979466897,
0.0017372594740947644,
0.12049660786530005,
0.20187020062396174,
0.0005365732980300889,
0.17827505978714833,
0.026911269370926586,
0.0004891183939972939,
0.14629752581605746],
[0.0009115060958508604,
0.0007519306923320339,
0.008726778532819132,
0.16233320644539936,
0.0019551205061979675,
0.04262027397542316,
0.10970492914318002,
0.07846269244592968,
0.0013166700638422876,
0.42124456484126627,
0.0007148674452997505,
0.002145743937654536,
0.15353696226050328,
0.014496766142157782,
0.0010779874721438688],
[0.0006440129562954902,
0.0005312669989837355,
0.05018521497923916,
0.18806014996164933,
0.001381365349987198,
0.035003855463898045,
0.0041449101284586915,
0.001635295264954916,
0.2797199451747824,
0.282951810989602,
0.0005050804365462241,
0.011298141536078145,
0.044895965313487086,
0.08849925356348491,
0.010543731882552731],
[0.0019234738380761104,
0.04541101183086165,
0.003807286846549004,
0.06500421704488098,
0.004125724623319712,
0.002289388734956259,
0.026987697413063335,
0.06331650577270914,
0.002778456921689112,
0.36302528349081614,
0.016116616142092817,
0.26747363946780744,
0.061050342737180435,
0.07441556980007862,
0.0022747853359192365],
[0.0014556575021193287,
0.0233112092588403,
0.0028813002553976005,
0.049194262140533435,
0.0031222894123793853,
0.0017325766648533384,
0.09781027057914825,
0.0921378075211018,
0.02421308704838342,
0.33000832106088557,
0.0011416294027085945,
0.003426711323919607,
0.1014780030193692,
0.0010406629290777812,
0.2670462118812824],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.15167305065014264,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0008693778474167097,
0.0007171777453621543,
0.22620964208063607,
0.27367746239668156,
0.1405196129160369,
0.02744521375027601,
0.13764761612128878,
0.02861799646294301,
0.0012558158317007256,
0.1574787147234436,
0.0006818274980408546,
0.00204657133300267,
0.0011832803384591332,
0.0006215262146835991,
0.0010281647400281972],
[0.0009940855069110713,
0.05366810682296216,
0.00951739555115118,
0.15439088744805005,
0.03988085769991201,
0.0011831968369176412,
0.03659688813703713,
0.0025242090341830066,
0.0014359559785801486,
0.15741903854826808,
0.000779632050701391,
0.5308206783662517,
0.008902737611836367,
0.0007106808668039562,
0.001175649540434075],
[0.0007138416111568596,
0.0005888709020552902,
0.001412964253984992,
0.27892883884853675,
0.0015311432128683495,
0.0008496403282303828,
0.22144914578628785,
0.16445371644062437,
0.0010311438223107267,
0.23231098695768473,
0.0005598449985570444,
0.0830009842108773,
0.0009715853075458372,
0.011353072619686919,
0.0008442206995925524],
[0.0021082597544981705,
0.0017391712727228455,
0.004173048509169224,
0.3914785917555021,
0.02053355295853743,
0.0345322771183444,
0.013568899700570345,
0.1334451484512144,
0.0030453800782978178,
0.3658777549471976,
0.0016534461717664481,
0.02097445369483923,
0.0028694799657882518,
0.0015072142782066437,
0.002493321343345123],
[0.0019519886683892953,
0.0016102582281428877,
0.04833768460002667,
0.27351301017950036,
0.10795945117382555,
0.00232332812628351,
0.02738778063595073,
0.004956542869920239,
0.2844880349007401,
0.16086187875462726,
0.04600484340198934,
0.004595106791531532,
0.032306088789875405,
0.0013954946422596866,
0.002308508236937499],
[0.0019234738380761104,
0.00158673542758596,
0.018415378980974235,
0.021179940641605284,
0.39854421225280096,
0.002289388734956259,
0.1584605266228904,
0.20939742711696144,
0.002778456921689112,
0.17312008574328816,
0.0015085240076675864,
0.004527981048153317,
0.0026179741994795187,
0.0013751091279524605,
0.0022747853359192365],
[0.006486890303398351,
0.0006226257498162832,
0.07027952153323891,
0.21466757845711357,
0.05894021394305952,
0.0008983428194401181,
0.010589815444650255,
0.0019165070340549102,
0.0010902503304100503,
0.2857522680955715,
0.012056196764735853,
0.0017767534185264473,
0.0010272778439380465,
0.3330031457314224,
0.0008926125306237111],
[0.0005945753282217145,
0.4023776216956362,
0.0011768908844038651,
0.3226380521326684,
0.11416497022890455,
0.05489471500706878,
0.00834231208643323,
0.006025347704596772,
0.0008588637409363245,
0.06254525108277788,
0.000466307957630667,
0.0013996685395941068,
0.023387185131418534,
0.00042506736764914485,
0.0007031711120599107],
[0.0014556575021193287,
0.0012008186871698063,
0.0028813002553976005,
0.4914020735739432,
0.0031222894123793853,
0.012787771950688585,
0.19730702815166545,
0.0036962452344198407,
0.13476503990673588,
0.10890441534418062,
0.0011416294027085945,
0.003426711323919607,
0.0019812454468519846,
0.0010406629290777812,
0.03488711087874226],
[0.0009579251636902121,
0.0007902232742212089,
0.0018960985085941854,
0.11967445529301507,
0.19848230956024288,
0.00114015747720127,
0.0207154642409672,
0.08973355557662278,
0.0013837223823004396,
0.3408453706066189,
0.0007512725561273831,
0.1332067662153863,
0.0013037990504105508,
0.0006848295050393313,
0.08843405058956236],
[0.0013940131516301526,
0.0011499662799805638,
0.0027592826224381393,
0.24826452150047437,
0.0029900663430206015,
0.001659205309969316,
0.2207125333751944,
0.003539716218224213,
0.0020136512456787525,
0.08311846147849823,
0.03285436938216321,
0.0032815965606120415,
0.001897343437929548,
0.39271665141156537,
0.0016486216826211665],
[0.0007296647423405847,
0.0006019239118376687,
0.12335819365601322,
0.04682534414120389,
0.11239590951626266,
0.000868473596230528,
0.054570042736364184,
0.05172665664474588,
0.02876170700921391,
0.32612509209008617,
0.2222339080749022,
0.01834230171291081,
0.0009931216281205977,
0.011604726704559521,
0.0008629338352081978],
[0.0010093257646916717,
0.0008326252830209767,
0.03265970481183557,
0.4940383581631615,
0.002164937249421873,
0.0012013363477287862,
0.0754852837447761,
0.002562907512438269,
0.0014579705227245022,
0.2134906828601874,
0.00079158453702586,
0.010041483962953502,
0.16234854962869752,
0.0007215762671840382,
0.0011936733441524634],
[0.001408929528862659,
0.0011622712792675454,
0.00278880781051779,
0.10111664577271179,
0.0565236266489522,
0.0016769593263361905,
0.1909732871985905,
0.46369105696423063,
0.002035197944546067,
0.14820973383509567,
0.0011049820264397076,
0.02471733693529372,
0.0019176456068342547,
0.0010072566714599313,
0.0016662624508613439],
[0.0011210384341754434,
0.0009247806567365862,
0.09587168345824344,
0.08045515685771647,
0.17268222938614045,
0.043903719798161406,
0.058298380510043324,
0.0028465713699064804,
0.010133223204676472,
0.34780042538163003,
0.000879197500894119,
0.0026389965299840083,
0.001525806922454279,
0.0008014406814922261,
0.18011734930774528],
[0.004573238963902134,
0.0004389493587546723,
0.005094370904178352,
0.369561334499471,
0.07388176396035373,
0.0006333290980198898,
0.007465789360833768,
0.13066746409257227,
0.10583814321869256,
0.23378360829854858,
0.01658185472420029,
0.0012526060381496922,
0.0007242279186052627,
0.02866835340305275,
0.020834966160665082],
[0.0015590726154938214,
0.013126725024672329,
0.003085998127076945,
0.13557336960032182,
0.003344108015381181,
0.025536856874958366,
0.03371548659491955,
0.015799435595757545,
0.0022520795522061833,
0.17584435380040686,
0.0012227348371537676,
0.11023552010994304,
0.47574583559373124,
0.001114595344249979,
0.0018438283137273742],
[0.001214066201136415,
0.0010015222534581858,
0.06694587271936568,
0.1977763674318524,
0.08558765805541779,
0.13053057309289565,
0.053915791791269915,
0.03996437492988827,
0.03863530288939925,
0.24757659774954885,
0.0009521564447915292,
0.0028579898728685786,
0.010872820051166247,
0.0561903244622134,
0.06597858205472786],
[0.0013651082709869133,
0.001126121714362429,
0.002702068861743506,
0.025399118292024757,
0.0029280672789089683,
0.001624801666509275,
0.2679735883353852,
0.003466320156874962,
0.022706911033502666,
0.3613176728861802,
0.0010706143016268376,
0.0032135526136859785,
0.0018580019973208604,
0.0009759284513872484,
0.3022721241395002],
[0.0011306647827424405,
0.0009327217412510619,
0.002238015963605295,
0.34734279053719513,
0.011012193893689046,
0.009932750920690079,
0.32499575411837595,
0.0028710148567595507,
0.001633244668855508,
0.17046014362162873,
0.0008867471631936678,
0.11429255938350966,
0.0015389090149728866,
0.0008083226465708725,
0.009924166686960126],
[0.0007061846342879855,
0.011308991133764205,
0.09793573864590444,
0.23303117943705395,
0.001514719502089054,
0.0008405267150164102,
0.3531542445149046,
0.21095867928994275,
0.02783617511879084,
0.0581961303663466,
0.0005538398566080356,
0.001662404019880697,
0.0009611636592281382,
0.0005048578865000159,
0.0008351652196821995],
[0.0007504582185372428,
0.0006190771190665769,
0.07557842650061655,
0.32173383502329345,
0.035806445288827746,
0.000893222750195016,
0.03902676076699654,
0.12729371082104524,
0.30315543297676995,
0.07894306318751798,
0.0005885623277035096,
0.013165547505004765,
0.001021422914638116,
0.0005365094788550334,
0.0008875251209322889],
[0.002374381162214451,
0.0019587033803624408,
0.004699804067329931,
0.2425358682815922,
0.005092891118234702,
0.4356077819286921,
0.015281674742532062,
0.006029093411570907,
0.0034297923082133113,
0.21370348910019793,
0.0018621573715485262,
0.005589445871721737,
0.0032316886766835568,
0.0016974669188457494,
0.056905761660260366],
[0.06687487805967851,
0.0006525497517671285,
0.10970295689523904,
0.11684751340063422,
0.03774244963231315,
0.06702536166959595,
0.1492740817028057,
0.05006959341044991,
0.0011426488266555502,
0.3896001335345835,
0.0006203850683764411,
0.0018621459240208706,
0.0010766498209485262,
0.0065731399418467885,
0.000935512361084649],
[0.07207118623288288,
0.0004091850588275365,
0.0009818176774741884,
0.17498197695884388,
0.05757064402502189,
0.04202863687165696,
0.037096459715530085,
0.005026628184224727,
0.0007165044904056915,
0.39875266359539807,
0.00038901600990866677,
0.01623612475098302,
0.17772947138822226,
0.004121725043190332,
0.011887959997429896],
[0.0007296647423405847,
0.0006019239118376687,
0.0014442842532667458,
0.14657308819799647,
0.0015650827864931243,
0.000868473596230528,
0.004696170707967897,
0.06835128065421131,
0.0065955416632600015,
0.4591220841658095,
0.0005722546153631652,
0.007259219039933854,
0.0009931216281205977,
0.2997648762019603,
0.0008629338352081978],
[0.0013651082709869133,
0.13590370538446472,
0.002702068861743506,
0.19127922127061223,
0.0029280672789089683,
0.001624801666509275,
0.019153433867504004,
0.06567135877384526,
0.0019718981611792337,
0.38205268575850365,
0.0010706143016268376,
0.09652111053914142,
0.022593014869644296,
0.05281346063219583,
0.022349450363133856],
[0.0012253645477922296,
0.07546046749672715,
0.011731665952896169,
0.19031071136815697,
0.12360896662180391,
0.029377082916641154,
0.09164235553735384,
0.0031114790832388836,
0.04830105397449422,
0.41739225181925554,
0.000961017406058512,
0.0028845869071095873,
0.0016678016137124127,
0.0008760243791118095,
0.0014491703756475908],
[0.0009308374326541273,
0.0007678777337532055,
0.11495249084796946,
0.43441226167617636,
0.0019965849496814815,
0.029385418988834077,
0.18979469655690748,
0.016502358957520762,
0.1356627301767767,
0.04136271609673778,
0.021938155182682908,
0.0021912511470316134,
0.008336306449075348,
0.0006654642371236012,
0.0011008495670750747],
[0.014963803841868718,
0.00778223775157378,
0.0018295478179580185,
0.17865178425930078,
0.0019825694218264865,
0.31698890412380043,
0.01998837730648755,
0.11466302110693244,
0.001335155454066794,
0.2867636216296421,
0.0007249038272666946,
0.00217586911107396,
0.03635678897032204,
0.0006607928443269177,
0.015132622533553335],
[0.0469602838156126,
0.005701160236680975,
0.04248088112265177,
0.14630555494423436,
0.10944642445896786,
0.011091091853579834,
0.29748469463566446,
0.037717398640382045,
0.016405833846368012,
0.24093451593969145,
0.0005310545639131844,
0.006736584202393659,
0.0369196279609153,
0.000484087740444334,
0.0008008060385001812],
[0.000523639196421812,
0.00043196681326645385,
0.04875869808398083,
0.16881685383888137,
0.16019722758260305,
0.008576957275916581,
0.17039793543646706,
0.033144450133478075,
0.0007563965368102597,
0.2618795288213193,
0.00041067483400146444,
0.11258451953617553,
0.004689558731725151,
0.004351205875329599,
0.024480387303623403],
[0.0016365851910038658,
0.20021848812061452,
0.01566870103995339,
0.08016730481487967,
0.09051529954104766,
0.001947923400834023,
0.010533170905904844,
0.016584937779851993,
0.0023640464257245904,
0.42074307027471536,
0.0012835256723283202,
0.016281902755640724,
0.01465677593489745,
0.1254627700246766,
0.0019354981179270216],
[0.0007547599428760237,
0.0006226257498162832,
0.21358278054629706,
0.24906036062024753,
0.1391900389903721,
0.006630473179962445,
0.016321945805172582,
0.0019165070340549102,
0.0010902503304100503,
0.2341630948508706,
0.0005919360436911985,
0.0017767534185264473,
0.13286627613595156,
0.0005395848211274864,
0.0008926125306237111],
[0.14972771940091198,
0.0008078549438343119,
0.0019384047574076964,
0.3157176102141221,
0.0021005310500431377,
0.0011655969708237956,
0.2666125673818651,
0.047111188051110836,
0.023726859474009755,
0.18482713936190895,
0.0007680351470699035,
0.002305331948694112,
0.0013328897578708844,
0.0007001096011438889,
0.0011581619391835224],
[0.0009649452340179333,
0.000796014355998688,
0.0019099938997825133,
0.3257470168575653,
0.009398156001660147,
0.0011485130210141044,
0.07949457222546304,
0.0024502152584121696,
0.0013938628701026083,
0.21876022081453686,
0.3012216728888112,
0.0462420154985599,
0.0013133538271029586,
0.008018260283647535,
0.0011411869633249955],
[0.0009866366743444138,
0.00830705907772878,
0.009446080271491322,
0.30309702835344443,
0.0021162706458666947,
0.031146933984694317,
0.021336360627037722,
0.0025052947553527096,
0.23371286954933135,
0.09629426792061974,
0.0007737901502120463,
0.0023226061485260227,
0.2860826059922276,
0.0007053556279302915,
0.0011668402211925416],
[0.23342745750562174,
0.041073789433789684,
0.0009636664708262828,
0.11258750525373744,
0.012136676359780836,
0.0005794696463610283,
0.08447775345840525,
0.008631169071868387,
0.015493137902199496,
0.4172630731405783,
0.00038182413493309986,
0.0011460821557475564,
0.052427217149678955,
0.000348055351158028,
0.019063122965314035],
[0.0005215650098818798,
0.00043025575008421457,
0.048565560068413054,
0.12061496922518408,
0.13183497965655486,
0.09568715448970608,
0.25290604508466047,
0.001324372099487177,
0.0007534003754720252,
0.33214197475055424,
0.00040904811045060067,
0.0012277975574064453,
0.0007098842272727663,
0.00037287161993664124,
0.012500121974935434],
[0.005617485937682093,
0.0005391784399682883,
0.0012937298472986084,
0.26531891397154844,
0.016293583858104747,
0.005741825143721725,
0.019098282371825877,
0.12575671168907784,
0.07043848565852633,
0.10350177468443596,
0.0005126019164684316,
0.03132191974882426,
0.010817362240894173,
0.34297516420306323,
0.0007729802885599384],
[0.0006668439097919263,
0.056258937721399754,
0.08235097316489585,
0.15927600706992856,
0.0014303362406484033,
0.0007937019494784597,
0.009356291363551417,
0.0016932682448183793,
0.06173653210582347,
0.24233836065768855,
0.20816501391156306,
0.17376074333281197,
0.0009076183498609309,
0.0004767328409267923,
0.0007886391368124761],
[0.0006668439097919263,
0.0005501009867621174,
0.04689989524285373,
0.11369604974158869,
0.026752534756392787,
0.0007937019494784597,
0.47022030435009915,
0.22452861518336895,
0.0009632556680369539,
0.10559848867266887,
0.0005229860824591233,
0.001569793425750158,
0.0009076183498609309,
0.0004767328409267923,
0.005853078839961353],
[0.0013373777266104862,
0.001103245896497221,
0.002647179559501883,
0.18739361230723062,
0.053653101705219745,
0.0015917957609165602,
0.27268692781322534,
0.03386661477211822,
0.06287325894460816,
0.36413483875352615,
0.0010488660505669882,
0.013305176053117767,
0.0018202589054846744,
0.0009561036303055868,
0.001581642121070617],
[0.0009243031862811307,
0.0007624874237799859,
0.07202705109589597,
0.18567153458709457,
0.00900231974962028,
0.0011001393730796691,
0.2165413864847138,
0.07254451914016968,
0.001335155454066794,
0.2797438713018483,
0.07092240710520463,
0.00217586911107396,
0.0012580373313530672,
0.0006607928443269177,
0.08533012581149128],
[0.0013940131516301526,
0.0011499662799805638,
0.0027592826224381393,
0.2694385787218422,
0.0029900663430206015,
0.001659205309969316,
0.19953847615382658,
0.003539716218224213,
0.0020136512456787525,
0.220749833417389,
0.08578951243558272,
0.1938481115529223,
0.001897343437929548,
0.0009965928162608828,
0.012235650293305072],
[0.013982282518914185,
0.0007124735588006343,
0.08698048892298917,
0.3046788467962709,
0.087123473375298,
0.0010279778916602042,
0.018677278955767124,
0.21209078292222952,
0.0668406144780803,
0.19580158557844218,
0.0006773551844835802,
0.00859245084267365,
0.0011755188434843004,
0.0006174494355508743,
0.0010214206953553845],
[0.001049552303422962,
0.0008658094484983401,
0.05787426983100385,
0.21083123989869068,
0.11384483319873528,
0.0012492154416861084,
0.030667905283061143,
0.12222963684544097,
0.1210806627448486,
0.29373807549848957,
0.0008231330292487732,
0.04232557525288663,
0.0014285096043281298,
0.0007503345894966817,
0.001241247030162278],
[0.0005892536771319013,
0.022861943243159835,
0.0011663573113470907,
0.15864421967747275,
0.028114929026414427,
0.0007013512238911706,
0.044069003855798705,
0.02387209794427517,
0.018751855766492295,
0.3618218255705797,
0.0004621343430638876,
0.005862310817795168,
0.33196457718038247,
0.00042126287036692566,
0.000696877491828458],
[0.061010435740487645,
0.0005953258660469093,
0.006909249802980539,
0.3203519241063102,
0.02347111581543752,
0.17624446439432492,
0.07589505689231033,
0.04567885280619955,
0.017484838426433152,
0.2567806746067352,
0.0005659817923669624,
0.001698849217123081,
0.0009822354316275424,
0.0005159259812789171,
0.011815069120337512],
[0.0029618982019054777,
0.002443365080841099,
0.005862723912175019,
0.23506530506860854,
0.0063530764502575336,
0.0035253592969644973,
0.04155752517085104,
0.03001548447271867,
0.13924577096700114,
0.44653870943503327,
0.024817481489538537,
0.006972498746435619,
0.026525889715937,
0.024612040019780405,
0.0035028719719522017],
[0.0013239306911037624,
0.001092153004453591,
0.0026205627579648084,
0.07490685846120572,
0.002839744083714094,
0.001575790608676776,
0.008520905794347802,
0.0033617609228611617,
0.4543774057526939,
0.3001448788166343,
0.0010383199357759801,
0.0031166179439083962,
0.13251406442014962,
0.011001267745089503,
0.0015657390614205432],
[0.0005299619360240709,
0.00043718264450247395,
0.03727282939418967,
0.33587493537530105,
0.0011367334276111832,
0.09320279771348226,
0.011460610860756911,
0.0013456938033850113,
0.10138728808179981,
0.4099369542867603,
0.0004156335728703945,
0.0012475644612665018,
0.0007213129951405835,
0.004403744993664366,
0.0006267564532454131],
[0.001170882209499363,
0.0009658983899677301,
0.055672197606674384,
0.030677789797432853,
0.10032818793103301,
0.001393626722300377,
0.1320298863874113,
0.002973136043765749,
0.0016913387201149014,
0.3365870908956344,
0.0009182885091628505,
0.09168062539383284,
0.07273308230406604,
0.0008370744546492807,
0.17034089463445493],
[0.007374606642702827,
0.0007078306824623708,
0.20371174639566506,
0.0746138064546871,
0.008357014074447035,
0.3789817300257801,
0.012039007855921656,
0.09341060971176544,
0.0012394486345235138,
0.18800907339555345,
0.0006729411591211052,
0.0020198981252767248,
0.0011678585049970957,
0.0006134257895657249,
0.027081002547530774],
[0.0007420002169625285,
0.0006120998415598547,
0.0014687008527256916,
0.2448498272348627,
0.007226766397138264,
0.0008831557281528752,
0.004775562641313382,
0.0018841071899764823,
0.0461536175572452,
0.44434295335056423,
0.000581928965616761,
0.0017467162035811558,
0.24332457873078597,
0.0005304627757809437,
0.0008775223137339408],
[0.15019850883788552,
0.0016102582281428877,
0.03351303258307705,
0.2438637061456011,
0.004186887055178187,
0.00232332812628351,
0.3238808209749432,
0.06425515093771873,
0.002819646578697238,
0.14603722673767763,
0.0015308873511404725,
0.004595106791531532,
0.002656784755976155,
0.01622014665920931,
0.002308508236937499],
[0.02457076355215307,
0.0008522233509922014,
0.017736651445526042,
0.050605050308595446,
0.07282893665089,
0.0012296130189747858,
0.014494883976918338,
0.03400680654419435,
0.001492287767212593,
0.25774522603107564,
0.0008102166010262662,
0.0024319436718931832,
0.49569738633559995,
0.0007385604988895497,
0.02475945024605859],
[0.04225228221292179,
0.000564392649472897,
0.0013542299952905628,
0.2517462505556233,
0.011859524890526479,
0.000814322382504041,
0.24861602197094262,
0.11085355780793382,
0.01138030953146098,
0.18628215800484188,
0.016124615917149955,
0.11592288931066885,
0.0009311983390601434,
0.0004891183939972939,
0.000809128037605374],
[0.06695202182949692,
0.06676222759624267,
0.0021458825762345353,
0.28364264941476197,
0.00232536211227383,
0.0012903570428435876,
0.05637838445569273,
0.07685421409931138,
0.0015660081673318615,
0.17990971183233467,
0.0008502420527677204,
0.22485625866661735,
0.03440950788134316,
0.0007750460727089507,
0.001282126200038625],
[0.005455017538887643,
0.0005235843719496182,
0.0012563127145999331,
0.12267649188936033,
0.1411506005752022,
0.024857031112535528,
0.17279608760448384,
0.0064319649607998824,
0.010557458932512887,
0.38008672828180057,
0.000497776492157364,
0.0014941243965945882,
0.010504503509790201,
0.0004537528037781028,
0.1212585648155473],
[0.000625652385624295,
0.000516120773764566,
0.0012384042096886207,
0.4060240545876355,
0.006093588025119649,
0.0007446742946498515,
0.018281554944779843,
0.0015886736030970767,
0.48081584834656904,
0.06581434461205646,
0.0004906807805157169,
0.001472825930230625,
0.005603158869844426,
0.00044728464165529123,
0.010243133994769057],
[0.15793804234491737,
0.0006564937179665155,
0.007619153391800887,
0.14172945902533685,
0.0017069715909664015,
0.0009472085240881596,
0.07160517060123711,
0.11685546047923148,
0.0011495549182415094,
0.4040427137841191,
0.0006241346334228689,
0.026049127829062626,
0.06756640687972365,
0.0005689357458871624,
0.0009411665339982622],
[0.0004886593362404497,
0.011536686623963326,
0.01581201058514134,
0.2428970570227721,
0.0010481420729687694,
0.0005816201694425235,
0.006856235860070897,
0.2313347174430914,
0.09348566666686278,
0.3334542018571171,
0.04120635250604154,
0.00486152742737616,
0.008087481326216965,
0.0003493470513721871,
0.008000294051322588],
[0.0006083097015130162,
0.000501814235903712,
0.010443863316189007,
0.20997358980263844,
0.07522307925660536,
0.11622136839187629,
0.017774801930238168,
0.024644103785067987,
0.0008787030357480741,
0.48902020951119995,
0.00047707942300236154,
0.01529168045622019,
0.014687629767822629,
0.02353435339587688,
0.0007194139900978795],
[0.14568833197733472,
0.0008727662440839018,
0.0020941559528093435,
0.284840446220264,
0.03440938612447851,
0.009294272161860287,
0.07108941874263316,
0.002686465579629547,
0.0015282594500211073,
0.28806324733954064,
0.0008297469189841413,
0.15515593156555185,
0.0014399877064977917,
0.000756363542367268,
0.0012512204739437028],
[0.0015968888465021569,
0.001317324965124682,
0.01528864740278541,
0.272267544259869,
0.015553017899520998,
0.0019006753633914887,
0.02240547915889598,
0.04043825339864661,
0.0023067050775018106,
0.27713193538502195,
0.001252392995859288,
0.0037591789863913212,
0.34175177428198295,
0.0011416305154152563,
0.0018885514630910994],
[0.0010171224827424186,
0.0008390570464820244,
0.032911990564311426,
0.32018701747542067,
0.002181660695827165,
0.0012106162859955127,
0.07606838336922533,
0.24204776987920648,
0.04781730992180067,
0.26921258651448593,
0.0007976992738773531,
0.0023943717010026125,
0.0013843704888617462,
0.0007271502125881397,
0.0012028940881724994],
[0.0013794093058433455,
0.0011379190979340919,
0.01320649370640551,
0.057093555403620294,
0.002958742056219769,
0.0016418232799309998,
0.05078244045424251,
0.0244548690413804,
0.1486582031453408,
0.4070073499510093,
0.032510183143088754,
0.013723335822311113,
0.24282817267209975,
0.0009861523926652416,
0.0016313505279081486],
[0.0015055913203197073,
0.0012420106996655033,
0.02584898764617698,
0.30243912933969375,
0.003229394161717557,
0.0017920097169793,
0.03255893501893625,
0.0038230385476070564,
0.05934694924650516,
0.31845983757445806,
0.02404964038749261,
0.014978683420779099,
0.20786885287915113,
0.0010763610747149157,
0.0017805789658029295],
[0.001214066201136415,
0.0010015222534581858,
0.002403099115218374,
0.050250027765229986,
0.057926469367926094,
0.04754700703042053,
0.28442569752036745,
0.003082790013232665,
0.0017537179727436468,
0.4780865034786464,
0.0009521564447915292,
0.0028579898728685786,
0.02931361250949405,
0.0008679470872299913,
0.038317393367236156],
[0.0005498807235265207,
0.00045361429289770573,
0.05120217959969686,
0.31508973048196975,
0.0011794579141307024,
0.0006544880980915116,
0.03277209336841249,
0.0013962721319232558,
0.05508420512088654,
0.1998326611969218,
0.0004312552925716248,
0.0012944545673484735,
0.00074842377290842,
0.3010756528829474,
0.03823563055576692],
[0.0006806319884541681,
0.005730630418778736,
0.0013472297702650166,
0.16256929667224826,
0.006629065963233245,
0.13520814828888308,
0.16462440392744723,
0.03274321052528286,
0.000983172540228061,
0.4489461519464691,
0.00570295486988117,
0.006771406651670582,
0.0009263848303212256,
0.0004865900651063213,
0.026650721541730885],
[0.0005134300219136186,
0.004322861328006098,
0.020512855392922885,
0.2903036267282587,
0.001101273561318268,
0.0006111031432239696,
0.14367985977646835,
0.001303715516145306,
0.0007416493897394194,
0.3269614681652636,
0.0004026680784432595,
0.18057720126420712,
0.0006988119744599671,
0.027662270580075133,
0.0006072050795543896],
[0.09269392562732516,
0.0007124735588006343,
0.0017095422222106157,
0.042306703101567644,
0.0018525266745194407,
0.007587281484027785,
0.3400831549817786,
0.19241287214512678,
0.0012475785544044991,
0.14332715683950153,
0.0006773551844835802,
0.15289712987476042,
0.0011755188434843004,
0.0006174494355508743,
0.020699331472458126],
[0.0011404578859955405,
0.026924903020954783,
0.09753244323335171,
0.19444663830669948,
0.0024462070078463756,
0.001357414582514798,
0.02466279773323702,
0.011557249316288662,
0.14022927151528078,
0.37114801510370427,
0.0008944276062932449,
0.020007446257031644,
0.018874973160826564,
0.0008153238261074697,
0.08796243144386769],
[0.0050871895008075116,
0.03645057511640041,
0.014657461300073909,
0.2222913883996291,
0.04622246153744896,
0.018685651836122127,
0.1701351415346834,
0.13186629689817714,
0.1671806203962497,
0.07574995310913424,
0.0004642117695497097,
0.04634624622091148,
0.03227262694169257,
0.0004231565678545255,
0.03216701887126524],
[0.0005299619360240709,
0.00043718264450247395,
0.2908396604388211,
0.12658167800512907,
0.06955952910632125,
0.04490435370498104,
0.19660464622667828,
0.0053705641374267804,
0.0007655297307555983,
0.17649447491233775,
0.0004156335728703945,
0.00527243479530827,
0.08121871967597596,
0.0003788746596225976,
0.0006267564532454131],
[0.0015590726154938214,
0.0012861291400030272,
0.003085998127076945,
0.1000515819463139,
0.027025299784719785,
0.0018556651056197576,
0.2231650207496284,
0.05132122324976546,
0.0022520795522061833,
0.30609090853176923,
0.21435346076120126,
0.03919194480192723,
0.0021220002069590772,
0.024795787113588586,
0.0018438283137273742],
[0.15867685098129689,
0.0014207050996532951,
0.003408905949093428,
0.2413161576150994,
0.003694023534240365,
0.06744760301443445,
0.050322908241411654,
0.00437307855900054,
0.054805943304894196,
0.3642782750572459,
0.001350677443374007,
0.017133742831462167,
0.0023440387296449973,
0.0012312226201655284,
0.028195867018983228],
[0.0006055122992142174,
0.0004995065687835009,
0.0011985393128756002,
0.08944314258273398,
0.028890673028787943,
0.023713943704878376,
0.0038971173442786445,
0.015333477871835446,
0.000874662189636218,
0.4085943586392837,
0.0004748855025843623,
0.1761740455286177,
0.24915114345947784,
0.0004328863087473949,
0.0007161056582651267],
[0.1466531842750005,
0.0008326252830209767,
0.04799063728660492,
0.14142691124346649,
0.002164937249421873,
0.1161833299084989,
0.02949248632046806,
0.10221396859843904,
0.0014579705227245022,
0.2441525478097261,
0.00079158453702586,
0.1326889437611083,
0.00903922488100402,
0.02371797497933806,
0.0011936733441524634],
[0.08631155942076194,
0.001826926641963742,
0.004383612827086564,
0.10848296343799024,
0.021569638104356827,
0.019455327867300243,
0.014253561310436988,
0.05608162332327465,
0.07047658094991012,
0.48525557541864955,
0.0017368760107932535,
0.12294909080619652,
0.0030142686233957717,
0.0015832655260530385,
0.0026191297318305783],
[0.013893179056445286,
0.0013334973496961148,
0.0031996556143767917,
0.2878867730365556,
0.12623412877925977,
0.014200695064924633,
0.17000077267423327,
0.09004144395604008,
0.002335023770758114,
0.1209372828582021,
0.0012677682310516828,
0.1634022434423686,
0.002200153595795113,
0.0011556459544470605,
0.0019117366158458418],
[0.0008105114289062284,
0.006824159792734478,
0.020070939165602108,
0.27976866698034275,
0.0017384936012777488,
0.3333640141253263,
0.023683133895727057,
0.002058072728080424,
0.0011707833219115164,
0.14681567798406886,
0.0006356602958618589,
0.0019079960001274778,
0.17961390190227655,
0.0005794420709738006,
0.0009585467067828556],
[0.02496246930514749,
0.12840136679102915,
0.002077463493646611,
0.09126665489006802,
0.002251220524020818,
0.0012492154416861084,
0.07052276695260203,
0.0106360241707265,
0.0015160777362259554,
0.5806930795191839,
0.016765077697065128,
0.010441685917253926,
0.05722531594168536,
0.0007503345894966817,
0.001241247030162278],
[0.0021082597544981705,
0.03376212071695787,
0.21232221989669683,
0.3594556423112671,
0.00452207823641992,
0.0025093276741093755,
0.029580374422687853,
0.1014221990069794,
0.0030453800782978178,
0.23778595717025752,
0.0016534461717664481,
0.004962978972721721,
0.0028694799657882518,
0.0015072142782066437,
0.002493321343345123],
[0.031957183489636415,
0.0004268739702276115,
0.004954226017890253,
0.09608715561415351,
0.24083777881650859,
0.0006159063708383759,
0.29021786883365797,
0.0013139626281719972,
0.0007474787016460977,
0.23521220841781418,
0.00040583302114608204,
0.0012181471551821615,
0.0007043045873031868,
0.0003699408752966129,
0.09493113150052693],
[0.0011813876153939375,
0.03686342112277037,
0.0023384157557682643,
0.46161931532380684,
0.0025339985800851123,
0.0014061306396581708,
0.03452013146302633,
0.011972025778369571,
0.04656758438029901,
0.37549587883127983,
0.0009265275902922873,
0.020725490624394174,
0.0016079461209513887,
0.0008445848658919542,
0.0013971613080127504],
[0.04488295900078164,
0.0005338782223661319,
0.0012810122584619877,
0.26762586975994074,
0.08002954272929198,
0.040090989190306084,
0.2695799667109354,
0.006558419623577904,
0.0009348487570665894,
0.0680787270998411,
0.0005075629507028033,
0.21778731598480663,
0.0008808522123596467,
0.00046267374133545015,
0.0007653817582259245],
[0.0014719299754449471,
0.0012142423737357916,
0.0029135096738087257,
0.15035320374227243,
0.003157192795286308,
0.00175194475626383,
0.5013397092596261,
0.003737564742542096,
0.002126202055655343,
0.07658549711332546,
0.0123331702946446,
0.22704059515790745,
0.002003393351588136,
0.012231075139131853,
0.0017407695687668807],
[0.004518459840133614,
0.000433691540074929,
0.12481524637833998,
0.2573309516022383,
0.06900406428379295,
0.028574852218823624,
0.04331093176429542,
0.02529132718266353,
0.2842432390864194,
0.13915051633563008,
0.00041231454768782933,
0.017208521660783176,
0.004708282873062223,
0.0003758491712636114,
0.0006217515147913545],
[0.0011504221147629714,
0.0009490201998268809,
0.0022771232437957586,
0.09130101945787994,
0.16847138292404734,
0.0013692743711124433,
0.007404193081631173,
0.011658225492194719,
0.22008784110081642,
0.1909128631431836,
0.009639284537165518,
0.13376380178866223,
0.019039884599348838,
0.14061512380981578,
0.0013605401357564256],
[0.02261866365611973,
0.0007845158452267454,
0.0018824038377874125,
0.16936796697034456,
0.08871047489627962,
0.10224765602992154,
0.10001392195801413,
0.19742373605624458,
0.0013737283749070063,
0.19393254934794957,
0.04408116076494652,
0.07446425428265012,
0.0012943822934685322,
0.0006798832880639103,
0.0011247023980760057],
[0.007571979666836654,
0.0007267749718517647,
0.12887224705641553,
0.15021140724899776,
0.0018897122638417147,
0.007739580253712568,
0.06588896120207052,
0.0022370892083084537,
0.18861972118497675,
0.28002350625849937,
0.0006909516697930582,
0.002073958419031186,
0.001199114919889376,
0.16121307210128455,
0.001041923574490774],
[0.0008470079865913857,
0.03286232953217662,
0.2139563468665068,
0.08651930900504289,
0.0018167763123431975,
0.13609528250506023,
0.005451399615782269,
0.07934339875345534,
0.0012235025798035693,
0.2627829065065029,
0.0006642834735601595,
0.008426632323657273,
0.08477820754043938,
0.08423090784704099,
0.0010017091520369934],
[0.000695002287208886,
0.0005733297378614908,
0.006653966515290395,
0.16072335738572074,
0.08594341337019984,
0.00610550953166842,
0.16282185446938968,
0.2604010990159116,
0.0010039304290287332,
0.17867532822342733,
0.0005450698704002867,
0.10192363668407606,
0.006224236204663191,
0.0268883257943021,
0.0008219404808512634],
[0.0011605619939717737,
0.0009573849122828307,
0.002297193924235001,
0.08329170028571051,
0.13470009422605164,
0.0013813432252735521,
0.36003149463446704,
0.07345933879445743,
0.045746686251512216,
0.2807360890127514,
0.000910194667396204,
0.002732037530163318,
0.010393652098103282,
0.000829696437702287,
0.0013725320059215257],
[0.000702417416305115,
0.0005794467163221277,
0.03339799758306031,
0.18911118796912912,
0.09219496985165254,
0.006170650527905129,
0.031193843575102572,
0.0017835974628724474,
0.04369150312436062,
0.3832967461248881,
0.21393519306500658,
0.0016535357466070865,
0.0009560362281772253,
0.0005021646677347359,
0.0008307099408762157],
[0.0005840264421545153,
0.00048178219431382934,
0.02776883552535252,
0.3790104369111502,
0.0012526982303417977,
0.1648075498048908,
0.003758832942272742,
0.01035391750591393,
0.06294021731785855,
0.23885441321670547,
0.00045803477627955654,
0.0013748357837510734,
0.10724619794471113,
0.0004175258720313801,
0.0006906955322724833],
[0.0006913531232353914,
0.0005703194251433278,
0.23239389907788158,
0.3594014447080776,
0.006733485254231979,
0.016574608807279314,
0.135714053733134,
0.05426128636747059,
0.03775270777518527,
0.0937279255555639,
0.0005422079383308397,
0.02262980315329355,
0.0009409769987670718,
0.0004942547029131004,
0.037571673379492514],
[0.010128862164099063,
0.000516120773764566,
0.0012384042096886207,
0.13043097101186718,
0.0013419831358822644,
0.0007446742946498515,
0.17983612117885095,
0.21541089361877938,
0.0009037545335931637,
0.2748849597385014,
0.052758334562126946,
0.1012565286042157,
0.000851553980607041,
0.005198889530892676,
0.02449794866248121],
[0.0006771317891435346,
0.0005585877892955247,
0.03219573622788086,
0.22344414165501614,
0.006594975511258821,
0.0008059469588089319,
0.2100609630301118,
0.16628170982501833,
0.00612068895159711,
0.22550679859753509,
0.11366764840639312,
0.006736584202393659,
0.0009216208292171398,
0.000484087740444334,
0.005943378485885633],
[0.16175073951580224,
0.01963716590815507,
0.004616555698375992,
0.4153713657736411,
0.022715837285465192,
0.002776016369699105,
0.015010988033261827,
0.0767749285271759,
0.0033690398573836453,
0.24534444026968036,
0.0018291726850177448,
0.005490439137502385,
0.02088760261561953,
0.0016673994202175651,
0.0027583089030024117],
[0.00221463864662537,
0.018646310796791053,
0.021202996981913875,
0.34395434160557264,
0.004750253949529512,
0.00263594371247293,
0.1488086345490555,
0.005623470858792709,
0.22185103834335593,
0.21614542894141253,
0.0017368760107932535,
0.005213401722405331,
0.0030142686233957717,
0.0015832655260530385,
0.0026191297318305783],
[0.16843181875253083,
0.0004927091802653558,
0.0011822293424507326,
0.2696687346854071,
0.03303359160667069,
0.0007108953561496817,
0.16260649331385746,
0.06955764259526306,
0.000862759605973368,
0.28509633289195757,
0.00046842316261841974,
0.00594208635376004,
0.0008129269059958175,
0.0004269955024825086,
0.0007063607446173949],
[0.0017450326536059197,
0.014692429060274239,
0.01670697932781375,
0.31077877295745726,
0.030248771050915783,
0.0020770015272430957,
0.17026589202534637,
0.150212885060495,
0.0025206987269628953,
0.24982995743156583,
0.001368577830391183,
0.04386660554359204,
0.0023751040299872317,
0.0012475398848932268,
0.002063752889456199],
[0.0020116321619637278,
0.01693708296744156,
0.003981785724728106,
0.3429807590501544,
0.11125817874159295,
0.002394317988271609,
0.13516798132865995,
0.03566323706231303,
0.0181834244763228,
0.15049943009756903,
0.0015776639904568628,
0.15751174044231045,
0.0027379634672503703,
0.0014381343240757961,
0.01765666817688939],
[0.008057172188294671,
0.0007733447985867396,
0.016095014439318104,
0.24527304833230426,
0.002010800050453078,
0.0011158047140947096,
0.18402627180945905,
0.04509868078747642,
0.001354167286574431,
0.20541047824003203,
0.0007352260336481023,
0.030685682075870475,
0.015515365977757057,
0.24274025597217477,
0.001108687293956086],
[0.0008415942455465081,
0.01986907515182191,
0.0016658353432066622,
0.7187352724737381,
0.0018051641945743026,
0.0010016961743994086,
0.056549401734206924,
0.0021369990639195265,
0.020390499426942002,
0.10770476584750893,
0.013443248964319726,
0.001981166948379721,
0.04588670468259163,
0.006993269126697884,
0.0009953066221467665],
[0.10996171799560961,
0.11897015534753257,
0.001187616452412234,
0.33469188360217733,
0.055967809549282384,
0.0007141347203890772,
0.09499637107066285,
0.001523521071545376,
0.18313623100723422,
0.06767199429086172,
0.014140773146829237,
0.01508263988899057,
0.0008166312013266916,
0.00042894120932833213,
0.0007095794458178352],
[0.0006736674057042582,
0.0056719916089111875,
0.05249606121939953,
0.20183589614705513,
0.006561233890632005,
0.0008018235232505978,
0.24480006807660112,
0.001710594651759105,
0.006089373905130756,
0.19877173829592829,
0.16936497368043194,
0.09879482869135943,
0.006033167276794481,
0.005597872721806652,
0.0007967089052355302],
[0.33589156511175494,
0.0008870207008977187,
0.04295961486795085,
0.16699883518029263,
0.002306372621083846,
0.02577857332782171,
0.2600742649443898,
0.10072535694429444,
0.0015532197512223753,
0.0559467556045312,
0.0008432987625656629,
0.0025312429868003033,
0.0014635063092323021,
0.0007687168517709064,
0.001271656035391333],
[0.0004997881957862085,
0.0004122913557984485,
0.004784982698192639,
0.3926659019565183,
0.0010720127432885323,
0.03855198241578253,
0.04876520952397871,
0.06579617348103578,
0.0007219437792559965,
0.048778582511722325,
0.0155748157037732,
0.008767956791637702,
0.012067379434813777,
0.36094990775652536,
0.0005910716518906001],
[0.0011813876153939375,
0.0009745646371420428,
0.0023384157557682643,
0.46161931532380684,
0.011506212701492194,
0.010378344761065254,
0.00760348909880508,
0.20936073644932537,
0.1004008691087415,
0.11530166931047445,
0.018870955833106454,
0.011753276502987091,
0.010580160242358472,
0.036733441351520284,
0.0013971613080127504],
[0.004997331739998957,
0.00047965469989025815,
0.0011509058145603534,
0.21836493619936317,
0.14697134806408974,
0.0006920599663349312,
0.2112887960496177,
0.0986258816249669,
0.05383051201885843,
0.19805670114621413,
0.04019897076702934,
0.001368764668010853,
0.022870809614251635,
0.00041568212609197563,
0.0006876455007216326],
[0.0010841182084065403,
0.0008943239751522991,
0.0021458825762345353,
0.09427242650412718,
0.0846602416386368,
0.0012903570428435876,
0.12224628807678312,
0.0027528225255847028,
0.04273344793051335,
0.4845487660798777,
0.0008502420527677204,
0.14252137914025437,
0.0014755560707979734,
0.0007750460727089507,
0.017749102105311217],
[0.0015055913203197073,
0.0012420106996655033,
0.002980138287643687,
0.06231621107509416,
0.003229394161717557,
0.058964133113312535,
0.00969008566040296,
0.0038230385476070564,
0.002174825850171929,
0.547148331159791,
0.012615215708225965,
0.2093639029683121,
0.08209018140721801,
0.0010763610747149157,
0.0017805789658029295],
[0.027848170233391797,
0.01875075707256269,
0.011210050900186997,
0.24409609398857235,
0.0025114651767607576,
0.010286056063597854,
0.21206175045908862,
0.03854285340895566,
0.0016913387201149014,
0.2298779388000646,
0.16098201665251746,
0.029433620004750503,
0.010486076914983693,
0.0008370744546492807,
0.0013847371498028522],
[0.0051101611415470555,
0.07725544313621165,
0.019239234137705227,
0.20523282098620949,
0.09610262697560319,
0.009738856873815375,
0.071560513472988,
0.12343057885105561,
0.08213940838079245,
0.23413751198914082,
0.000466307957630667,
0.01494642597957013,
0.032418356758069214,
0.00042506736764914485,
0.027796685992011953],
[0.0014885703744927819,
0.001227969574062455,
0.002946447357265609,
0.08422202997553724,
0.08232898236876773,
0.013076907482598436,
0.12263210488648499,
0.252493266256442,
0.00215023910310506,
0.3600802260680562,
0.0011674420013277232,
0.014809347057303246,
0.0020260420273922465,
0.05758997622561559,
0.001760449241548768],
[0.0005408461134097952,
0.00044616135246105626,
0.10375883400322977,
0.08399852008871195,
0.07098811897702147,
0.1115470919559602,
0.2745780168106358,
0.001373331203483586,
0.0007812519191189741,
0.29923767888235453,
0.03328442369273742,
0.017703313504118412,
0.0007361270752774289,
0.000386655858086068,
0.0006396285633934535],
[0.00055451217150247,
0.03414799927873927,
0.08532400153689242,
0.24615116893725467,
0.0011893920648208464,
0.0006600006164386703,
0.03725944149301786,
0.0014080324309528297,
0.0008009925326631522,
0.39523652502390955,
0.13519714502295746,
0.04762988328233219,
0.00917736856659493,
0.00039642584863876614,
0.004867111193284866],
[0.0006346999152863139,
0.029445490113557588,
0.0012563127145999331,
0.045551409911739074,
0.11222869483359423,
0.0007554429945288821,
0.2017179933460918,
0.06909609406761716,
0.0009168236853102299,
0.16799275284334217,
0.053521270351771975,
0.19912714696424907,
0.015324821133391531,
0.0004537528037781028,
0.10197729432114198],
[0.0004960226776960663,
0.0004091850588275365,
0.0009818176774741884,
0.020530308234493398,
0.0010639359551375721,
0.053329978485633825,
0.1953152423112062,
0.001259514312899105,
0.004483618361731313,
0.489163396507213,
0.00038901600990866677,
0.21212604605991536,
0.0006751194359180377,
0.0003546111718647106,
0.01942218774008114],
[0.022769651125365855,
0.0005986067080208719,
0.034502336150215765,
0.14576532363166497,
0.16137551247160056,
0.00086368743659881,
0.18102235063671748,
0.0073535758085241315,
0.0010481917289768346,
0.4069928444661491,
0.022613108485895976,
0.0017082115783723594,
0.0009876485329493368,
0.01154077303373803,
0.0008581782052099534],
[0.0012978318696772403,
0.0010706232473256631,
0.02228203597705254,
0.3395575013459443,
0.0027837638316994756,
0.0015447268392681534,
0.01820949840779362,
0.0032954900836140812,
0.11029694739873143,
0.25480181754405623,
0.0010178513970756477,
0.012911746001009916,
0.22846746068358728,
0.0009278319336673835,
0.0015348734394970294],
[0.040132552004472986,
0.00043025575008421457,
0.0683710535657086,
0.23152573281003916,
0.0011187225744042138,
0.0006207857026874088,
0.15387857759818271,
0.01716876689732362,
0.15523624965437735,
0.30441428385434044,
0.00040904811045060067,
0.0012277975574064453,
0.0007098842272727663,
0.004333970319395752,
0.020422319373853654],
[0.09165953344387553,
0.00044985695591751723,
0.0010794077206047338,
0.27106425882015917,
0.009452798102283696,
0.18701903667057648,
0.08634085201062287,
0.09664046898309117,
0.0007877231145211238,
0.17332809602652596,
0.0004276831576454293,
0.07997327524953621,
0.0007422244966447967,
0.00038985857100085026,
0.0006449266769944838],
[0.0008155314678010957,
0.019253762853253902,
0.026388920143421903,
0.16382176473925927,
0.01413659773263108,
0.0009706753054950015,
0.06099182880619812,
0.0020708197477706603,
0.0011780347653876301,
0.22824269269772096,
0.32271034581236663,
0.07624383236685876,
0.032078333076830064,
0.000583030942925349,
0.05051382954207958],
[0.014074602107076275,
0.0007171777453621543,
0.028131278185742573,
0.04258603785263916,
0.001864758189611456,
0.0010347652309568767,
0.38854687705482055,
0.06823366924192172,
0.0012558158317007256,
0.388570139267486,
0.0006818274980408546,
0.00204657133300267,
0.0011832803384591332,
0.0006215262146835991,
0.06045167390849625],
[0.27485918649641666,
0.0008262913743883605,
0.0019826419870840606,
0.10992243954486564,
0.002148468238676207,
0.001192197597298404,
0.18141120956614912,
0.13947218049472565,
0.0014468795166401338,
0.28033101109580266,
0.0007855628316624075,
0.0023579429931650433,
0.0013633082502558604,
0.0007160871255004884,
0.001184592887369288],
[0.0007216664533755098,
0.0005953258660469093,
0.034313235842576964,
0.36419830176966456,
0.0015479269837603761,
0.00633975094882707,
0.16358781221901889,
0.012794069558683835,
0.2750823071986396,
0.08139516395331806,
0.0005659817923669624,
0.001698849217123081,
0.0009822354316275424,
0.05532389806047177,
0.0008534747044989415],
[0.0028967373926660034,
0.002389611833740083,
0.00573374586889886,
0.2958929804087412,
0.028212989662386802,
0.003447802524587132,
0.3266390980621237,
0.007355474599910213,
0.004184335601371489,
0.08472013957139239,
0.002271826013040976,
0.20481621569712105,
0.0039426498071075365,
0.0020709041896407715,
0.02542548876727176],
[0.0011504221147629714,
0.0009490201998268809,
0.0022771232437957586,
0.39709749922499843,
0.046152791017199914,
0.07126561260359668,
0.12098574270941805,
0.002921183213134189,
0.0016617841243031894,
0.07733131351539671,
0.0009022422581049878,
0.2386083091373886,
0.03651396915746989,
0.0008224473448472961,
0.0013605401357564256],
[0.016857129260276535,
0.000858962680734347,
0.04950866529573465,
0.24870369132533646,
0.14457630877696823,
0.01705521347474636,
0.12532064588871283,
0.15289480591314175,
0.0015040886869148132,
0.11744056862908879,
0.0008166237439783096,
0.04989880558795813,
0.07258865842146868,
0.000744400989801639,
0.0012314313251384913],
[0.0010752650267627977,
0.0008870207008977187,
0.0021283587600249817,
0.16699883518029263,
0.06763638239376524,
0.001279819663066185,
0.05591798440476043,
0.34571289359184965,
0.0015532197512223753,
0.24377053370099022,
0.0008432987625656629,
0.10869250886740757,
0.0014635063092323021,
0.0007687168517709064,
0.001271656035391333],
[0.032182528473617626,
0.2578066942172616,
0.03926261585581455,
0.15333382595620224,
0.014313908938984479,
0.01352555741500811,
0.00531464899721474,
0.0020967934335585857,
0.03882093215060819,
0.39416066773945835,
0.00691897325400761,
0.039572014792989406,
0.0011239141831126734,
0.0005903437293387891,
0.000976580862823113],
[0.0009052395200287691,
0.0007467611924044905,
0.0017918135663488504,
0.1337172961912278,
0.09819124004251484,
0.001077449102018444,
0.17770039757339992,
0.16042288754911346,
0.0013076179984471096,
0.267099211638692,
0.0007099527540331673,
0.12588042749485281,
0.028731964980752842,
0.000647164054084529,
0.0010705763420809937],
[0.0011210384341754434,
0.0009247806567365862,
0.010732845546902545,
0.04639962169318012,
0.010918437354592742,
0.0013343008424909545,
0.1519511022125183,
0.0028465713699064804,
0.0016193394135423829,
0.41591149571070274,
0.000879197500894119,
0.011152880321118096,
0.001525806922454279,
0.3413567923268558,
0.0013257896939294137],
[0.03163232216073293,
0.00048178219431382934,
0.014462423074533203,
0.2282044291351979,
0.058913818850558845,
0.005130600395058955,
0.09690372009800799,
0.001482975872034382,
0.018585509148460817,
0.2832091213861032,
0.00045803477627955654,
0.06790689803784768,
0.0007948983381565695,
0.19114277100044164,
0.0006906955322724833],
[0.0012486040740239627,
0.001030013655534622,
0.26798703342929653,
0.10857573914766071,
0.08802246404202212,
0.010968833075137909,
0.027001496595963834,
0.00317048952213619,
0.0018036079115019551,
0.47272175672990707,
0.0009792434835613154,
0.012421993199807082,
0.0016994321349487495,
0.0008926385300391289,
0.001476654468458821],
[0.0005321035839537637,
0.05297370929808347,
0.0010532355242299824,
0.18366910702184597,
0.045593816300715136,
0.012756735237864998,
0.035753737020472354,
0.03772135035375977,
0.2917303706963176,
0.23782474367849693,
0.0004173132044068154,
0.005293741418098062,
0.09367034165741776,
0.0003804057434141644,
0.0006292892609232341],
[0.0011920832414871007,
0.0009833878030756174,
0.002359586470822459,
0.26662280151771744,
0.0025569399931996894,
0.06479296585269918,
0.03483265752475608,
0.02113385739352262,
0.16468394759880614,
0.24309375624276078,
0.0009349158555336334,
0.10239411968363243,
0.037836277792490984,
0.055172892600443016,
0.0014098104290528048],
[0.0016571827731110384,
0.0013670633642256917,
0.1920658042543131,
0.16927621780949806,
0.29302581463725386,
0.0019724393945065254,
0.17427993258926644,
0.004207963699397883,
0.002393799621969243,
0.1365671525861952,
0.0012996797506908586,
0.003901114765084746,
0.014841241826074374,
0.0011847351977866565,
0.0019598577306263313],
[0.0073268607188783745,
0.0007032479255123578,
0.03405925025889548,
0.18419500027959426,
0.0018285387923801554,
0.007489035765626104,
0.173820285131214,
0.002164670504434877,
0.001231423986843242,
0.3680741622233088,
0.20137401988381182,
0.014955558339951025,
0.0011602973582242701,
0.0006094542447173579,
0.001008194586607899],
[0.030946021289092105,
0.0004713293562888973,
0.27884216155726116,
0.11911155049767885,
0.14008113543136647,
0.005019285914089844,
0.12083670657157984,
0.10125327493126318,
0.000825322413307757,
0.17292285049479025,
0.026483525162968022,
0.001345007085372342,
0.0007776520727837444,
0.0004084671513831869,
0.0006757100707743243],
[0.0005321035839537637,
0.11763187537725739,
0.005094370904178352,
0.14729888860231066,
0.005182462501231442,
0.02083900599776174,
0.10041190309964626,
0.017515673454017926,
0.0007686233400349571,
0.5409098971746246,
0.0004173132044068154,
0.04166395983763339,
0.0007242279186052627,
0.0003804057434141644,
0.0006292892609232341],
[0.029416294584561214,
0.06485071198738636,
0.0018555995084737437,
0.273751878193993,
0.009130507515875259,
0.0011158047140947096,
0.0202730001047489,
0.1518942927688091,
0.001354167286574431,
0.3691637499447422,
0.0007352260336481023,
0.0022068522141817496,
0.04399419583944578,
0.0006702021478206216,
0.029587517155644807],
[0.001170882209499363,
0.0009658983899677301,
0.26909050179781385,
0.16406422991689504,
0.05586604122454563,
0.036963344087490295,
0.18538446243519618,
0.011865565385063228,
0.01947619740270986,
0.16763093341098226,
0.0009182885091628505,
0.04721847868734546,
0.03716336493887613,
0.0008370744546492807,
0.0013847371498028522],
[0.0015408282903797686,
0.0012710788094823797,
0.026453959255323758,
0.27441132659200157,
0.003304975140335589,
0.0018339500442728512,
0.13863927789366207,
0.003912513223119854,
0.00222572563441888,
0.5365497573760482,
0.0012084263490977152,
0.0036272088339274824,
0.0020971684824562476,
0.001101552308518981,
0.0018222517669547384],
[0.0009243031862811307,
0.00778223775157378,
0.19136280666839045,
0.2558690378650325,
0.16343682696108378,
0.0011001393730796691,
0.06912662960104411,
0.1778407740570766,
0.001335155454066794,
0.06915136146803444,
0.0007249038272666946,
0.04429437107783673,
0.015297537986940656,
0.0006607928443269177,
0.0010931218779657477],
[0.009717657229685147,
0.035280691529021885,
0.002238015963605295,
0.1240809869166848,
0.0024252014467463405,
0.0013457584737473736,
0.08455996560398017,
0.0028710148567595507,
0.001633244668855508,
0.5139398414993369,
0.0524087018448499,
0.028422634914082607,
0.13034379571911348,
0.0008083226465708725,
0.009924166686960126],
[0.0009308374326541273,
0.0007678777337532055,
0.13616061758597184,
0.1304291117648091,
0.0019965849496814815,
0.0011079166714975826,
0.034268433811556795,
0.002363607798852515,
0.0013445941694283886,
0.35948461716677327,
0.2905744271973795,
0.030468753464368104,
0.0012669308697412253,
0.0006654642371236012,
0.008170225146409197],
[0.0008636753341790245,
0.0007124735588006343,
0.0017095422222106157,
0.17349277494891926,
0.0149711338592546,
0.020705888668762946,
0.1629819579878539,
0.008752371558834522,
0.027484792923874825,
0.17612367480133945,
0.0006773551844835802,
0.39559136279236096,
0.007734822435851882,
0.007176753027918455,
0.0010214206953553845],
[0.0005892536771319013,
0.0004860943085535691,
0.0011663573113470907,
0.14074354052978777,
0.07286662689562697,
0.0007013512238911706,
0.02169315492119244,
0.001496249009668905,
0.0008511766188072837,
0.41552386301363475,
0.2242206236891265,
0.11774155549082649,
0.0008020129482097539,
0.00042126287036692566,
0.000696877491828458],
[0.10280928534800372,
0.0007902232742212089,
0.009171195664616579,
0.3233771756616421,
0.11845624084399653,
0.00114015747720127,
0.03526565855301199,
0.12610904135673473,
0.0013837223823004396,
0.15169284455003665,
0.0007512725561273831,
0.009530114563005608,
0.11770535354676886,
0.0006848295050393313,
0.0011328847172936201],
[0.0009115060958508604,
0.11843546816335031,
0.1887133652532,
0.1761783285008133,
0.02272280358931884,
0.0010849078091814146,
0.019711635782989564,
0.0023145211411531533,
0.0013166700638422876,
0.17895492887152278,
0.20146913724880153,
0.08521647627013802,
0.0012406196509502168,
0.0006516440867438675,
0.0010779874721438688],
[0.014963803841868718,
0.0007624874237799859,
0.029908549129133197,
0.171632033931507,
0.00900231974962028,
0.0011001393730796691,
0.44117339697411523,
0.0655247688123759,
0.001335155454066794,
0.19550686736832273,
0.0007249038272666946,
0.023235120094455344,
0.0012580373313530672,
0.0006607928443269177,
0.043211623844728514],
[0.0014885703744927819,
0.001227969574062455,
0.002946447357265609,
0.17466328370599107,
0.025803198787234076,
0.0017717507662917045,
0.07741147802125806,
0.003779818497693877,
0.18303274656401278,
0.2583338156212956,
0.0011674420013277232,
0.014809347057303246,
0.0020260420273922465,
0.24977764040283004,
0.001760449241548768],
[0.0007724715739221011,
0.0006372366438689166,
0.1188618918534261,
0.13757207774419147,
0.01339018827792492,
0.3411847659515699,
0.10470462341608917,
0.10169442598978214,
0.0011158347719034678,
0.1692583748004684,
0.000605826755443578,
0.0018184477364427945,
0.001051384536834743,
0.0005522470289726152,
0.006780202919159708],
[0.0005154398851062486,
0.0043397835170078634,
0.0010202517216010602,
0.2601233990328879,
0.0011055845854139384,
0.000613495355720404,
0.018975727626505012,
0.0013088190155857845,
0.04380493900871038,
0.22254770953671776,
0.0004042443550830512,
0.020786281516655358,
0.4078179277809723,
0.016026815029266388,
0.0006095820327664861],
[0.0008470079865913857,
0.0006987241277568265,
0.20752362578562286,
0.17657740413741832,
0.0018167763123431975,
0.007440860887381067,
0.13410582123346143,
0.002150745782847831,
0.0012235025798035693,
0.11483032164617184,
0.0006642834735601595,
0.3493608496105071,
0.0011528334889479269,
0.0006055337955495302,
0.0010017091520369934],
[0.0005945753282217145,
0.04113075662960892,
0.005692476697729206,
0.1374990337863294,
0.0012753248957710344,
0.0007076852471646933,
0.030920241153059934,
0.001509761891271431,
0.0008588637409363245,
0.5276505898552879,
0.000466307957630667,
0.13686724293935432,
0.0008092560647918323,
0.018487410620950506,
0.09553047319189206],
[0.0009441870585218019,
0.0007788902694529082,
0.4177730544374911,
0.3115686934740227,
0.0020252190174104502,
0.0011238058832312363,
0.17817511906942798,
0.0023975055329849195,
0.0013638776967942196,
0.06346820397726226,
0.0007404981639541379,
0.009393438211766225,
0.0012851005871568951,
0.0006750080073699129,
0.008287398613153264],
[0.0021780059282270284,
0.001796707134455614,
0.3351345393625431,
0.04052384077328604,
0.004671679182707445,
0.0025923421145869546,
0.3944647428263339,
0.005530452061028415,
0.0031461283886320547,
0.19602895842929227,
0.0017081460462488448,
0.005127165948688784,
0.002964409088150114,
0.001557076458931826,
0.002575806256887578],
[0.0019519886683892953,
0.0016102582281428877,
0.03351303258307705,
0.0659678819422056,
0.004186887055178187,
0.00232332812628351,
0.29423151694104394,
0.13837841102246684,
0.002819646578697238,
0.4425302670766701,
0.0015308873511404725,
0.004595106791531532,
0.002656784755976155,
0.0013954946422596866,
0.002308508236937499],
[0.0011920832414871007,
0.0009833878030756174,
0.17437501402576533,
0.19419525307353097,
0.16551892399261928,
0.001418860964036008,
0.27927563352388546,
0.0030269702824759995,
0.010775407154909876,
0.15255932068752767,
0.0009349158555336334,
0.011859684128399342,
0.0016225035703977446,
0.0008522312673031561,
0.0014098104290528048],
[0.0011404578859955405,
0.0009408003870689034,
0.0022574002424368264,
0.3503512541100147,
0.054414412275618135,
0.001357414582514798,
0.02466279773323702,
0.12415502739646081,
0.07093833115825178,
0.0939842536755882,
0.0008944276062932449,
0.00268471116777439,
0.27005463195505675,
0.0008153238261074697,
0.0013487559975814203],
[0.0013373777266104862,
0.001103245896497221,
0.06358859709932628,
0.2280212240004469,
0.002868587088699405,
0.0015917957609165602,
0.11017648104036028,
0.06433732354203042,
0.001931841404783755,
0.3133503241370058,
0.0010488660505669882,
0.0031482731298137015,
0.0018202589054846744,
0.17362345332647475,
0.032052350890982816],
[0.0007635130556094519,
0.07021313172421162,
0.00151128294871837,
0.2461501598689901,
0.0016376851878082803,
0.0009087610935497137,
0.09769173436554278,
0.0019387331766606874,
0.0011028941970325586,
0.5268090896307298,
0.0005988008527875387,
0.001797358808516547,
0.0010391914051457055,
0.0005458425018816853,
0.04729182118281514],
[0.0013940131516301526,
0.0011499662799805638,
0.045107397065173756,
0.1212201781722675,
0.11944738106054353,
0.022833262531337124,
0.17836441893245877,
0.003539716218224213,
0.012600679856362656,
0.4748385200738027,
0.0010932835501114991,
0.0032815965606120415,
0.001897343437929548,
0.0009965928162608828,
0.012235650293305072],
[0.03706376842752363,
0.0007845158452267454,
0.15355600393752836,
0.37159943376999915,
0.0020398462678562173,
0.03724468455860399,
0.09279136957231218,
0.13242076458492702,
0.07359925223192651,
0.09281681594812226,
0.0007458464507348127,
0.0022387304256306097,
0.0012943822934685322,
0.0006798832880639103,
0.0011247023980760057],
[0.0009649452340179333,
0.000796014355998688,
0.0019099938997825133,
0.09123783075195065,
0.1266527490544675,
0.0011485130210141044,
0.19674916527827038,
0.01710703939001309,
0.0013938628701026083,
0.2627306932093396,
0.044727250585795165,
0.15616819648556676,
0.06726906241930708,
0.0006898482178470777,
0.03045483522652683],
[0.0012727421350499791,
0.02038196361403501,
0.002519241121923043,
0.13000676712538461,
0.002729948004369178,
0.020846901817466408,
0.008191452850312385,
0.0032317815450918995,
0.30148505961074545,
0.30787209228941914,
0.0009981742554987761,
0.0029961167927590286,
0.19505266257788806,
0.0009098950517504658,
0.0015052012083065678],
[0.07869396880532947,
0.007837253313087328,
0.18564624664131069,
0.12335973618547497,
0.051482214005020344,
0.008177292250831705,
0.25341907677091463,
0.10133486590953023,
0.0013445941694283886,
0.07670959399340839,
0.0007300284446805396,
0.0021912511470316134,
0.10730756455975306,
0.0006654642371236012,
0.0011008495670750747],
[0.0005114357720247591,
0.023726924497983255,
0.07481157088396206,
0.27363935474259005,
0.001096996026730249,
0.000608729514251293,
0.06932253783719088,
0.0012986516624316354,
0.1599897706902559,
0.3334598355449772,
0.0004011040468587998,
0.040045660483692874,
0.000696097669407827,
0.0003656301346817859,
0.02002570049296152],
[0.04682270602455746,
0.0003873000102148621,
0.10433262666859092,
0.23337016095663238,
0.06875403530851937,
0.004124439651085027,
0.19200017236467906,
0.001192149849384385,
0.06842518603375555,
0.14565952187882691,
0.0003682096923164398,
0.0011052170864241178,
0.018467169818722606,
0.11443586124991015,
0.0005552434063809356],
[0.0010330829521623256,
0.024389903950982946,
0.002044864378865548,
0.050605050308595446,
0.010061788384248035,
0.0012296130189747858,
0.09295381931022081,
0.002623232410873358,
0.009338181300542842,
0.3518959484310386,
0.0008102166010262662,
0.12012034667184689,
0.024943774335785075,
0.19688589883214574,
0.11106427911269132],
[0.0007256435585652389,
0.11081864454202395,
0.012458328583415147,
0.17883133498186587,
0.007067459503996247,
0.02841869689509958,
0.00467029010231254,
0.0018425739168239773,
0.0010481917289768346,
0.3463718236574474,
0.006080102810795519,
0.0017082115783723594,
0.2985817506847576,
0.0005187692503377225,
0.0008581782052099534],
[0.0011605619939717737,
0.17723840522092596,
0.08162365306312441,
0.1449900573937356,
0.02011743102543362,
0.0013813432252735521,
0.03391160706347725,
0.011760981686432335,
0.001676431174351433,
0.27192203799731923,
0.000910194667396204,
0.24071141494683154,
0.001579601082671125,
0.000829696437702287,
0.010186583021353684],
[0.0014556575021193287,
0.0012008186871698063,
0.19081962011459677,
0.29240855842890884,
0.0031222894123793853,
0.0017325766648533384,
0.05358948943580726,
0.10319300280693705,
0.002102696476712929,
0.26367714934587405,
0.0011416294027085945,
0.003426711323919607,
0.06831241716186345,
0.012095858214913026,
0.0017215250212365236],
[0.0008751561641214331,
0.0007219444646414595,
0.2675921218491831,
0.3552544150647209,
0.0018771522981447242,
0.0276276282637688,
0.11862300139971803,
0.028808205872475248,
0.001264162607064205,
0.05882795091830309,
0.0006863592620297532,
0.021999662964030494,
0.03442362684375939,
0.08038361359480159,
0.0010349984332378482],
[0.0006139827714690162,
0.0005064941337552794,
0.13177869985031104,
0.18861690258587377,
0.0013169525825614925,
0.14994609240703297,
0.15782991994479095,
0.07150371729094465,
0.0008868977822397863,
0.288409742720984,
0.0004815286450590355,
0.006108333274459948,
0.0008356708694507394,
0.00043894192722531095,
0.0007261232138419676],
[0.0005738453450292592,
0.00047338347987303634,
0.17981996921521617,
0.17192844493153794,
0.01866345662921252,
0.0006830116648901827,
0.21724260994587855,
0.04503861420565377,
0.0008289192913953907,
0.13881128168807266,
0.22271565135201757,
0.0013508688267173986,
0.0007810411964223336,
0.00041024731210902973,
0.0006786549159741787],
[0.01707049813859755,
0.10415959519797427,
0.012322509833410312,
0.21503819640936733,
0.03969594203094644,
0.15348008235580088,
0.12999057698013464,
0.007273408274998879,
0.03374229537192776,
0.25538098322690944,
0.0005628966698703897,
0.012591432544627355,
0.000976881343089867,
0.0005131137090947655,
0.017201587913250077],
[0.0005664394622246906,
0.15533581915532357,
0.001121199296399799,
0.38050288331891224,
0.0012149753173964976,
0.12112750971126258,
0.04236277822882169,
0.0014383185328877709,
0.10836582221042004,
0.04237793462517058,
0.00044424182473463476,
0.061560091301709724,
0.05669571367423997,
0.00040495277841508844,
0.026481320562081155],
[0.13341397290153212,
0.002031987291037902,
0.004875644893927496,
0.12065947145156387,
0.09881969299492893,
0.002931811272881181,
0.20292593877402598,
0.21203442750324936,
0.022265367056674888,
0.053333841616770454,
0.0019318290614268384,
0.005798572202995431,
0.0033526006977110355,
0.0017609767975248908,
0.13386386548374965],
[0.012969990652417373,
0.00044985695591751723,
0.04249495655821429,
0.31662136254152967,
0.02601901763732752,
0.000649066901333483,
0.19402127898840774,
0.0013847066565892037,
0.0670526012546964,
0.2934331876555936,
0.0004276831576454293,
0.013708397109360924,
0.0007422244966447967,
0.021097632989805628,
0.008928036444516394],
[0.08048702113286117,
0.0009573849122828307,
0.002297193924235001,
0.2683867716097858,
0.002489328994569307,
0.15122021048762022,
0.19256452534125607,
0.14397174691791467,
0.001676431174351433,
0.08682696667324395,
0.044980449744556984,
0.020360139561027633,
0.001579601082671125,
0.000829696437702287,
0.0013725320059215257],
[0.0009243031862811307,
0.13413774365186207,
0.08606655175148355,
0.30500729015958905,
0.0019825694218264865,
0.0011001393730796691,
0.04104762828986893,
0.016386516517819333,
0.1136511606987675,
0.2867636216296421,
0.0007249038272666946,
0.00217586911107396,
0.008277787659146862,
0.0006607928443269177,
0.0010931218779657477],
[0.0022525247252984113,
0.0018581800866703001,
0.004458603797144915,
0.2471957163920341,
0.07325997828681909,
0.002681037105483402,
0.01449739862719342,
0.19389794051016346,
0.0032537707508104876,
0.4080213220026358,
0.01887370424778872,
0.005302587986769581,
0.003065834064277816,
0.0016103506319558732,
0.019771050784954645],
[0.0013239306911037624,
0.011146930528530191,
0.01267534028204141,
0.34638585161127394,
0.0330040766559439,
0.22278089613836202,
0.0185756833184244,
0.03352609349509097,
0.0019124171692468548,
0.31019965634071095,
0.0010383199357759801,
0.0031166179439083962,
0.0018019566071538068,
0.0009464902210129021,
0.0015657390614205432],
[0.0010841182084065403,
0.0008943239751522991,
0.010379370528870833,
0.18484079398312647,
0.1011272175439094,
0.0012903570428435876,
0.015210944692511247,
0.019219798430857297,
0.0015660081673318615,
0.3692799347429695,
0.0008502420527677204,
0.002552083945437319,
0.0014755560707979734,
0.2889471244149794,
0.001282126200038625],
[0.0014397408854694624,
0.0011876885582199328,
0.0028497952127955074,
0.34388284287465753,
0.0030881493185905477,
0.12199108949157983,
0.020200582197223652,
0.0036558293272751304,
0.00207970493254671,
0.17331950607271374,
0.31822426133814685,
0.003389242585268396,
0.001959581886419037,
0.0010292839935247802,
0.001702701325568909],
[0.0010752650267627977,
0.3602020744506454,
0.0021283587600249817,
0.1098350766291964,
0.002306372621083846,
0.06660982943574759,
0.023252979518419738,
0.00273034228527234,
0.0015532197512223753,
0.4070955581326937,
0.0008432987625656629,
0.0025312429868003033,
0.0014635063092323021,
0.017101219294941253,
0.001271656035391333],
[0.011154398229946239,
0.08977972048974663,
0.002568903256514549,
0.5366888285513243,
0.0027837638316994756,
0.0015447268392681534,
0.09706202928994559,
0.0032954900836140812,
0.001874717435772456,
0.12666645486055927,
0.0010178513970756477,
0.04248144508181691,
0.0017664343974003334,
0.07978036281581936,
0.0015348734394970294],
[0.0034240303727716844,
0.0028245927706723566,
0.006777459377080481,
0.063707292909917,
0.00734431950167223,
0.004075405866404819,
0.178062963288687,
0.008694391317627845,
0.004946010026742455,
0.36018453712261067,
0.0026853664022149786,
0.19009034357210614,
0.1086774478873653,
0.0024478707881435196,
0.05605796879598358],
[0.015572862094219599,
0.13111684249114675,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.07814155679201286,
0.0007624874237799859,
0.0018295478179580185,
0.3681850431097332,
0.05112082171638305,
0.02917914068425485,
0.12528463222339448,
0.009366766190025539,
0.015374656109654383,
0.30782287261302343,
0.0007249038272666946,
0.00217586911107396,
0.008277787659146862,
0.0006607928443269177,
0.0010931218779657477],
[0.029696700936632313,
0.001030013655534622,
0.0024714627116185547,
0.2887470192775136,
0.002678173454197059,
0.001486134120935125,
0.27355166940523623,
0.02213588743054176,
0.0018036079115019551,
0.09341379856179571,
0.0009792434835613154,
0.012421993199807082,
0.011182131089151535,
0.0008926385300391289,
0.257509526231934],
[0.0006377741894516056,
0.0005261204395766464,
0.0012623978733166783,
0.1038960332751736,
0.1660526150399309,
0.005602767738199599,
0.22691337600950795,
0.001619453618014072,
0.0009212644726839059,
0.3528657475217369,
0.0005001875550440489,
0.12743666782604404,
0.0008680525516002357,
0.00045595063063846153,
0.010441591259081335],
[0.0008470079865913857,
0.0006987241277568265,
0.008109272278220147,
0.11868291440946269,
0.008249497393227157,
0.0010081398064971086,
0.03761500502020206,
0.07934339875345534,
0.0012235025798035693,
0.48792814433744136,
0.02639516779709599,
0.22713914907371188,
0.0011528334889479269,
0.0006055337955495302,
0.0010017091520369934],
[0.0006736674057042582,
0.11311348732841268,
0.17017008034075828,
0.07904561532476771,
0.0014449721897033625,
0.0008018235232505978,
0.16805614256267148,
0.001710594651759105,
0.0009731122042021137,
0.23970183190335742,
0.010760860951644018,
0.016934641476501158,
0.19533485021115427,
0.0004816110208780096,
0.0007967089052355302],
[0.13504368658372062,
0.0009092973527507742,
0.1947225978132726,
0.20467820089080244,
0.03584964923295049,
0.0013119610742417826,
0.21637774263010967,
0.0027989121444279732,
0.00159222733651802,
0.17455054347332585,
0.00086447738097082,
0.002594812663038266,
0.0015002608297328079,
0.0007880224188936876,
0.026417608175244193],
[0.0007724715739221011,
0.0006372366438689166,
0.0015290152662893917,
0.1258387900854778,
0.025123475936638596,
0.0009194238488733797,
0.38043688339586046,
0.01369476854942961,
0.0011158347719034678,
0.22792481309403678,
0.2176716484416465,
0.0018184477364427945,
0.001051384536834743,
0.0005522470289726152,
0.0009135590898028718],
[0.02861144601963772,
0.0009923721886718289,
0.01151730126324451,
0.4335095396652775,
0.12135034471128968,
0.0014318239009042941,
0.03515089416096858,
0.021326939637745,
0.020010010208400382,
0.30013164246014,
0.0009434573937954013,
0.01196803606740607,
0.001637327018138394,
0.0008600173861655749,
0.01055884791821503],
[0.0007958154014945261,
0.0006564937179665155,
0.0015752215862846236,
0.20216877708049946,
0.0017069715909664015,
0.0009472085240881596,
0.1562202158784648,
0.11685546047923148,
0.061588872973404145,
0.19250510059104992,
0.0006241346334228689,
0.05626878685664394,
0.06152247507420739,
0.0005689357458871624,
0.14599552986638858],
[0.2095834691809988,
0.0008390570464820244,
0.0020132725349401117,
0.42060785107087745,
0.002181660695827165,
0.0012106162859955127,
0.1224164604132823,
0.08755417973234993,
0.001469232877743702,
0.11471899636762935,
0.0007976992738773531,
0.0023943717010026125,
0.0013843704888617462,
0.0007271502125881397,
0.03210161211754381],
[0.000547593891289293,
0.00045172781144112184,
0.001083896738435293,
0.09336407124366507,
0.05939745452528734,
0.0006517662269337108,
0.3487029822265856,
0.0013904653451300272,
0.025743671244455268,
0.38614664261189185,
0.00042946179724921555,
0.06367075162684528,
0.0173804260199534,
0.00039147990651959075,
0.0006476087843179422],
[0.0005498807235265207,
0.004629760655384375,
0.05120217959969686,
0.3401466086568898,
0.13063999515121746,
0.0006544880980915116,
0.011891361555979139,
0.005572418494409926,
0.12190254692067326,
0.3209409057090352,
0.0004312552925716248,
0.009646747292321815,
0.00074842377290842,
0.0003931147839071892,
0.000650313293386886],
[0.02752227642972545,
0.0005825544155892195,
0.012124244900504564,
0.14721968569165408,
0.2643124190973762,
0.0008405267150164102,
0.10644619999687997,
0.0017931632855305469,
0.006383301682440867,
0.37462601355250863,
0.0005538398566080356,
0.028478495815318163,
0.0009611636592281382,
0.0005048578865000159,
0.027651257015119664],
[0.027170557965839344,
0.001384488126479637,
0.016068131523710047,
0.1969260863873732,
0.0035998545534468886,
0.05298208541979383,
0.18924745288159497,
0.01700772520196111,
0.0024243112942061943,
0.21478461235240667,
0.014062371898826692,
0.2588733641850235,
0.002284283902404435,
0.001199835982209335,
0.0019848383247241504],
[0.0006877420795511567,
0.0005673405590777166,
0.07970861016166016,
0.2530611599377411,
0.048483545526470384,
0.00081857571354059,
0.020095815030907144,
0.05397787132405724,
0.000993443062982884,
0.28649506032946204,
0.2512507577103731,
0.001618989060615425,
0.0009360621311918643,
0.000491673134589513,
0.0008133542377796912],
[0.2727557232817991,
0.0004107323343014856,
0.004766888962332883,
0.2172385920569507,
0.0010679590785965614,
0.0005926167418236258,
0.18849108193794456,
0.001264276988278848,
0.1065972568926085,
0.19228576303768663,
0.0003904870189744179,
0.0011720846419937558,
0.0006776723046571248,
0.004137310763959227,
0.008151553958092663],
[0.014655176795525911,
0.007621729830153062,
0.056791562668337424,
0.38121616715017637,
0.0019416791140348373,
0.001077449102018444,
0.005826181629685645,
0.07104829525838204,
0.23505655168189854,
0.060850152506234816,
0.0007099527540331673,
0.0021309920153785218,
0.0012320904297585596,
0.15877144272230168,
0.0010705763420809937],
[0.0011210384341754434,
0.0009247806567365862,
0.0022189617557684563,
0.1570801109779233,
0.002404553563458652,
0.0013343008424909545,
0.28817324287066376,
0.02838822274330875,
0.0016193394135423829,
0.36482819296389823,
0.000879197500894119,
0.14737502097926353,
0.001525806922454279,
0.0008014406814922261,
0.0013257896939294137],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.15781332392994726,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0008309717579563114,
0.000685495327033656,
0.14048530659688263,
0.17954523202131514,
0.0017823796587286694,
0.007299984593035818,
0.13787775489289203,
0.008420957844587452,
0.02644406501978823,
0.4282028472433002,
0.0006517067306850642,
0.039821751075219236,
0.026374733892347728,
0.0005940693482887972,
0.0009827439979390295],
[0.02165118357321862,
0.001103245896497221,
0.002647179559501883,
0.20770741815383875,
0.002868587088699405,
0.0015917957609165602,
0.03907816057723181,
0.2674753820081118,
0.001931841404783755,
0.4453900621399587,
0.0010488660505669882,
0.0031482731298137015,
0.0018202589054846744,
0.0009561036303055868,
0.001581642121070617],
[0.06468910432000895,
0.00507481744397184,
0.06985701410991194,
0.2767152019062678,
0.0012928386676101713,
0.13804532619663307,
0.08169843396782672,
0.0061080925528308955,
0.014603450583757182,
0.2877064453649969,
0.0004727116679352321,
0.03346207180302615,
0.005397966825369491,
0.009586099559832336,
0.005290425030021329],
[0.0011404578859955405,
0.0009408003870689034,
0.07154834059946584,
0.09917159531578458,
0.15835082281116167,
0.001357414582514798,
0.09395373809026603,
0.06352545458406042,
0.0016473908012227707,
0.3105184422913039,
0.0008944276062932449,
0.1066211217033179,
0.0015522380715693093,
0.08742899927239373,
0.0013487559975814203],
[0.0010171224827424186,
0.0008390570464820244,
0.0020132725349401117,
0.21204150437262112,
0.10260249429128393,
0.0012106162859955127,
0.02199562681782554,
0.0025827051515788157,
0.4186019262742564,
0.22286450947042893,
0.0007976992738773531,
0.0023943717010026125,
0.0013843704888617462,
0.008451829719930967,
0.0012028940881724994],
[0.001025040592803845,
0.0008455889501166258,
0.07987709254828224,
0.28375554054787167,
0.13454049452159397,
0.001220040709462018,
0.3102050028178525,
0.010387625738594513,
0.0014806705834568565,
0.05333354484984225,
0.0008039092149155755,
0.04133708497088463,
0.07924329461630694,
0.0007328109422565454,
0.0012122583957598296],
[0.0007679661898134771,
0.04729293646483998,
0.013184951510583132,
0.09011028217843307,
0.00164723686720875,
0.11756260251937667,
0.016607535480190942,
0.036944603039407775,
0.0011093267469885072,
0.4657249675903755,
0.0006022933150831824,
0.10679152880139554,
0.10019651238479563,
0.0005490260884586035,
0.0009082308230491986],
[0.0012486040740239627,
0.001030013655534622,
0.0024714627116185547,
0.49736639626997486,
0.002678173454197059,
0.001486134120935125,
0.1028630882295861,
0.00317048952213619,
0.1630094901329493,
0.13134459437860685,
0.0009792434835613154,
0.08828358483342935,
0.0016994321349487495,
0.0008926385300391289,
0.001476654468458821],
[0.07701178084968874,
0.0004882794419598024,
0.1809830787943617,
0.26724425799267987,
0.08218475721188957,
0.005199790958206898,
0.05325768059275139,
0.05544641858999086,
0.014340863779877103,
0.1926274140510662,
0.0004642117695497097,
0.06882268101743685,
0.0008056182265570351,
0.0004231565678545255,
0.0007000101561297077],
[0.05008697804810574,
0.0010601735761192402,
0.11966818220066885,
0.1312756803256759,
0.05155840677523462,
0.001529649745048118,
0.037552492494070305,
0.003263324905131534,
0.1385014972805956,
0.2718355847840873,
0.18645480805341688,
0.003025359979340845,
0.00174919335700012,
0.0009187759574723377,
0.001519892518032565],
[0.0019234738380761104,
0.00158673542758596,
0.003807286846549004,
0.28412559906125945,
0.3839361201183757,
0.002289388734956259,
0.05620388168191379,
0.048708413638283904,
0.002778456921689112,
0.2023362700121386,
0.0015085240076675864,
0.004527981048153317,
0.0026179741994795187,
0.0013751091279524605,
0.0022747853359192365],
[0.0008006545027086768,
0.0006604856481826538,
0.05631094736168906,
0.03921965927767019,
0.06860486457466122,
0.0009529681988951073,
0.09028262806503375,
0.002033043752218531,
0.013317911075606934,
0.522032547204492,
0.14048363969842492,
0.06269162255365164,
0.0010897433535591867,
0.0005723952638275365,
0.0009468894693785477],
[0.0021780059282270284,
0.018337878965879205,
0.004311102734071291,
0.18939438725609836,
0.004671679182707445,
0.20108640409167006,
0.03055896253501491,
0.13785982671241714,
0.0031461283886320547,
0.3945230204063754,
0.0017081460462488448,
0.005127165948688784,
0.002964409088150114,
0.001557076458931826,
0.002575806256887578],
[0.0012253645477922296,
0.0010108426229672714,
0.002425462843676183,
0.20892311758659693,
0.011934529311164087,
0.0014584735889811961,
0.00788652755437396,
0.06825490084777878,
0.011076241537614275,
0.4546170642561355,
0.000961017406058512,
0.0028845869071095873,
0.2157104731257721,
0.010182227488331795,
0.0014491703756475908],
[0.0007256435585652389,
0.0005986067080208719,
0.0014363248000148399,
0.19536434065696634,
0.0015564576122960934,
0.2157927612129048,
0.04324730334421362,
0.11757361364252719,
0.0010481917289768346,
0.3463718236574474,
0.0005691009190953646,
0.0017082115783723594,
0.0009876485329493368,
0.0005187692503377225,
0.07250120279731195],
[0.0006503749853043746,
0.000536515241314821,
0.0012873396444791508,
0.1504030319347579,
0.001395011482945514,
0.0007741000347280319,
0.1523667808821657,
0.08562064034645128,
0.0009394663154970818,
0.3252619281989019,
0.03014625485149656,
0.23862050341938376,
0.0008852030621933268,
0.01034368734294178,
0.0007691622574387928],
[0.08338711522855208,
0.010899785621284856,
0.0013472297702650166,
0.16773845187475436,
0.1565345668359107,
0.005979268226230122,
0.18530102473747173,
0.06892729694282569,
0.000983172540228061,
0.25251825425123664,
0.0005337996673750508,
0.0016022514491644635,
0.0009263848303212256,
0.0004865900651063213,
0.06283480795927372],
[0.027848170233391797,
0.0009658983899677301,
0.011210050900186997,
0.19963394728208494,
0.13589790529622292,
0.010286056063597854,
0.17649203309389871,
0.23417629891750016,
0.0016913387201149014,
0.10538392802189991,
0.0009182885091628505,
0.06500333736994042,
0.010486076914983693,
0.009729503795946759,
0.010277166491100331],
[0.0022525247252984113,
0.0018581800866703001,
0.004458603797144915,
0.04191033280620522,
0.02193863239036186,
0.002681037105483402,
0.01449739862719342,
0.005719672223153624,
0.0032537707508104876,
0.459342667899093,
0.01887370424778872,
0.1250523950785031,
0.020172949363096892,
0.2753241954130611,
0.002663935486135569],
[0.0006083097015130162,
0.000501814235903712,
0.005823969876486693,
0.20535369636293613,
0.0013047842213683427,
0.0007240323993184447,
0.1563716051213076,
0.006164530026258732,
0.0008787030357480741,
0.25802553752608437,
0.3238696202021643,
0.038391147654731755,
0.0008279494487156878,
0.0004348861973653139,
0.0007194139900978795],
[0.0010752650267627977,
0.0008870207008977187,
0.04295961486795085,
0.183331337623463,
0.05130387995059489,
0.00944607088465136,
0.08858298929110113,
0.010896593506857514,
0.0015532197512223753,
0.48875807034854546,
0.0008432987625656629,
0.010697494208385477,
0.0014635063092323021,
0.0007687168517709064,
0.10743292191599861],
[0.04298163291455237,
0.0005112621423737953,
0.0012267461833631772,
0.1903924947540582,
0.015449973464687622,
0.005444538591505161,
0.2157981848720964,
0.006280592702350864,
0.00089524681530382,
0.4229172448969078,
0.0004860616348346152,
0.04852770585380762,
0.04791228240234587,
0.00044307401633070914,
0.0007329587554819737],
[0.001025040592803845,
0.0008455889501166258,
0.04095301901842974,
0.10470480231055021,
0.0021986445200954784,
0.001220040709462018,
0.20900241164023597,
0.127159846328152,
0.12603770587898486,
0.36472613308866225,
0.0008039092149155755,
0.010197826147002636,
0.0013951475566019486,
0.0007328109422565454,
0.00899707310173033],
[0.0018688725143226695,
0.001541693039653441,
0.358534577912144,
0.3754140771471835,
0.004008608382164729,
0.044804644391587024,
0.02622160224990559,
0.01893890677912398,
0.002699585338976099,
0.13981892596905307,
0.0014657017939717237,
0.004399446022479689,
0.0025436582124282407,
0.0013360741396904192,
0.016403626107315803],
[0.006306151880147071,
0.0006052780853678051,
0.018169595829086345,
0.437155754378771,
0.001573804086896194,
0.012018155374000847,
0.14403286821319622,
0.20247027010111002,
0.045639242769050056,
0.1050455529041799,
0.01729270687479505,
0.0017272493269893112,
0.0009986557200743103,
0.0005245508518109689,
0.006440163604524898],
[0.0007724715739221011,
0.006503880473225752,
0.03672887824243041,
0.190371872208403,
0.11898977720634797,
0.14758551958279428,
0.25723736297936695,
0.001961480890715939,
0.030449053918687647,
0.20445823777660943,
0.000605826755443578,
0.0018184477364427945,
0.001051384536834743,
0.0005522470289726152,
0.0009135590898028718],
[0.015572862094219599,
0.13111684249114675,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.02305626504844475,
0.0011748424614861645,
0.0028189716903142697,
0.015681942411389427,
0.013870795981523196,
0.0016950973992978402,
0.16059071877975406,
0.13340886651996256,
0.0020572107436818855,
0.3769497903984429,
0.0011169335653363982,
0.18722540436761193,
0.0019383869542152546,
0.001018151208203386,
0.07739662247033599],
[0.0006841685619354881,
0.016152435268592766,
0.0792944430908899,
0.12704190960266434,
0.0014674964777799016,
0.000814322382504041,
0.08234356736699737,
0.006933273680468054,
0.01138030953146098,
0.3525546126087871,
0.29670938306130756,
0.022394633595949662,
0.0009311983390601434,
0.0004891183939972939,
0.000809128037605374],
[0.019475288780233076,
0.0009923721886718289,
0.2947381756847885,
0.19596945144075673,
0.15789497366890828,
0.01056798114030894,
0.07169552311858716,
0.012190782398340351,
0.001737695729591091,
0.08086386871442852,
0.0009434573937954013,
0.01196803606740607,
0.13867968560920807,
0.0008600173861655749,
0.0014226906788103839],
[0.0006316551368978868,
0.01491265351619483,
0.13077451385796834,
0.400325219348241,
0.0013548586417752339,
0.0007518189882102542,
0.21034470032727576,
0.0016039159528641873,
0.0009124255045703262,
0.20556440809303675,
0.0004953885619417059,
0.0014869567925618686,
0.0008597241192640215,
0.029234737823434408,
0.0007470233357634649],
[0.013560229700145844,
0.0013015401509581946,
0.2907023888684394,
0.2690051050717518,
0.015366654243041782,
0.025842851637707928,
0.034119481588199836,
0.06391865408697055,
0.0022790650403437715,
0.26182873321407474,
0.0012373862274259741,
0.003714134716154527,
0.014129902557703909,
0.001127950955694079,
0.0018659219413876833],
[0.001093118385464064,
0.1918440929135755,
0.043672902657301574,
0.26109188242533327,
0.002344666898939191,
0.05941395680890222,
0.048544587781722943,
0.011077517081657955,
0.0015790089182372733,
0.23121434003127672,
0.00915914168814989,
0.03578063518471352,
0.10110989863473491,
0.00078148038201953,
0.0012927702079714852],
[0.0019519886683892953,
0.0016102582281428877,
0.003863728549177803,
0.11044183799305447,
0.004186887055178187,
0.00232332812628351,
0.10151104072069884,
0.004956542869920239,
0.1807154707820927,
0.5611274832122671,
0.0015308873511404725,
0.004595106791531532,
0.002656784755976155,
0.0013954946422596866,
0.017133160253887125],
[0.0007378423096581462,
0.006212316869784698,
0.01266776481092501,
0.187441305033432,
0.16969203371980968,
0.0008782068351889,
0.11121809544782826,
0.0242881373970476,
0.0010658128183512303,
0.23451807255622995,
0.19110266671014625,
0.052169751399632446,
0.0010042518342237886,
0.0005274902496283888,
0.006476252008113631],
[0.1338431498235277,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.0010093257646916717,
0.0008326252830209767,
0.15530716460999033,
0.3560599658902373,
0.009830403486806548,
0.22349985723188434,
0.0754852837447761,
0.010228373749822944,
0.009123436760109177,
0.14450148672372534,
0.00079158453702586,
0.010041483962953502,
0.0013737586436193448,
0.0007215762671840382,
0.0011936733441524634],
[0.0014241685786064394,
0.0011748424614861645,
0.08934735756966752,
0.09139428005582352,
0.00305474774660404,
0.12067162798340857,
0.009166043490885878,
0.0036162877009326905,
0.012873258978601042,
0.5067423692174727,
0.0011169335653363982,
0.0033525843739862908,
0.0019383869542152546,
0.15244282649707158,
0.0016842848259018947],
[0.0010665552684985334,
0.10618118435505614,
0.07501205248901949,
0.12514560859331555,
0.0022876907635910613,
0.001269452991027462,
0.031164731711594725,
0.0027082262295172158,
0.009640742249634484,
0.622500842847155,
0.00893657169463276,
0.010610843395544562,
0.0014516517563040677,
0.0007624901655252392,
0.0012613554895836673],
[0.0008470079865913857,
0.0006987241277568265,
0.0016765511973361894,
0.20874100954183808,
0.14333664009179028,
0.0010081398064971086,
0.05048044718196998,
0.002150745782847831,
0.0012235025798035693,
0.35927372271976227,
0.0006642834735601595,
0.02772479556630915,
0.0011528334889479269,
0.20001988730295225,
0.0010017091520369934],
[0.0006771317891435346,
0.0005585877892955247,
0.027053163780495413,
0.21830156920763066,
0.06830584487988424,
0.016233664300965286,
0.40033614358337344,
0.15599656493024744,
0.0009781165042116581,
0.076372197623357,
0.005673627011298636,
0.027306873991935467,
0.0009216208292171398,
0.000484087740444334,
0.0008008060385001812],
[0.07332299706768969,
0.0006564937179665155,
0.025750948808349678,
0.1840369816639507,
0.07423415325716155,
0.04325473116270201,
0.19248380671156237,
0.09267973325716644,
0.0011495549182415094,
0.08371432809175718,
0.0006241346334228689,
0.22549887741109934,
0.0010831570190447603,
0.0005689357458871624,
0.0009411665339982622],
[0.0013107513776124918,
0.0010812809649103981,
0.0025944758801978367,
0.3429376894393405,
0.0028114753248087115,
0.001560104108946917,
0.10798293663089371,
0.0033282956505604248,
0.1512136603173041,
0.21751954796212464,
0.0010279837874943667,
0.1524058736521228,
0.0017840186960660692,
0.010891753585935854,
0.0015501526216811748],
[0.0011404578859955405,
0.0009408003870689034,
0.13217791341186622,
0.2637375786637285,
0.02843030964173225,
0.1572620303858301,
0.059308267911751526,
0.011557249316288662,
0.036292860979737274,
0.2585502370235321,
0.0008944276062932449,
0.00268471116777439,
0.010213605616197937,
0.03546079400462197,
0.0013487559975814203],
[0.022644855285052654,
0.2253080113907376,
0.0014284525950612533,
0.2874671408587945,
0.0015479269837603761,
0.0008589537409077842,
0.10329904293190675,
0.0018324751428452637,
0.0010424468026752943,
0.23485748577505805,
0.022489170624044104,
0.08939160454383165,
0.006463032639546828,
0.0005159259812789171,
0.0008534747044989415],
[0.0015968888465021569,
0.001317324965124682,
0.01528864740278541,
0.13886178209146183,
0.015553017899520998,
0.0019006753633914887,
0.09517225852348171,
0.004054863716353747,
0.0023067050775018106,
0.2407485457027291,
0.001252392995859288,
0.47674324485619857,
0.002173470580582876,
0.0011416305154152563,
0.0018885514630910994],
[0.005252468988236323,
0.0005041433243405023,
0.061547031881738046,
0.2387961632689647,
0.04772419933380878,
0.005368728793955494,
0.05962932401036837,
0.08045451635379305,
0.005524117308765298,
0.1663964023797825,
0.0004792937088636312,
0.0014386465307828639,
0.3257253064342181,
0.0004369046502929779,
0.000722753032089354],
[0.000702417416305115,
0.0005794467163221277,
0.0013903514240465487,
0.23178804951448082,
0.0015066390677802167,
0.0008360428347361696,
0.14855521282481968,
0.0017835974628724474,
0.06502993389703646,
0.4579812538292536,
0.0005508853382481907,
0.006988143439776046,
0.05963672085303578,
0.021840595440410573,
0.0008307099408762157],
[0.0014241685786064394,
0.0011748424614861645,
0.013635019925233426,
0.3185312929891258,
0.035502892451361505,
0.0016950973992978402,
0.03079813996072419,
0.014432335935851847,
0.0020572107436818855,
0.5283744656873111,
0.0011169335653363982,
0.0033525843739862908,
0.0019383869542152546,
0.04428234414788001,
0.0016842848259018947],
[0.0010579854763849024,
0.0008727662440839018,
0.01012917520286211,
0.284840446220264,
0.002269309124267439,
0.0012592529118075194,
0.21571976524358297,
0.05893160032999892,
0.0015282594500211073,
0.30413328583964616,
0.0008297469189841413,
0.11498083531528804,
0.0014399877064977917,
0.000756363542367268,
0.0012512204739437028],
[0.02559020442530258,
0.0006727581351277902,
0.044969924861548015,
0.26911412480864055,
0.0017492612538803401,
0.0009706753054950015,
0.1910588618330809,
0.09497584333840121,
0.0011780347653876301,
0.14772500558584115,
0.14928763510985626,
0.0700501641274834,
0.0011099918799532165,
0.000583030942925349,
0.0009644836270766143],
[0.0006877420795511567,
0.0005673405590777166,
0.0013613033468198221,
0.1590443917599327,
0.011921469012878232,
0.00081857571354059,
0.04621158396918725,
0.001746333447497024,
0.000993443062982884,
0.772248362581472,
0.0005393759028840499,
0.001618989060615425,
0.0009360621311918643,
0.000491673134589513,
0.0008133542377796912],
[0.01769680050745749,
0.14203304654759522,
0.002163697352317997,
0.32750661091330696,
0.03555203114292605,
0.009602910442921928,
0.05684642884271966,
0.0027756760206612387,
0.0015790089182372733,
0.36404379700722417,
0.0008573006271531735,
0.002573270940726661,
0.034695170146761184,
0.00078148038201953,
0.0012927702079714852],
[0.019042703620115953,
0.0006604856481826538,
0.001584800009467225,
0.12434922182557082,
0.05644349849638971,
0.12256662898161029,
0.011233748556268882,
0.01419440983049005,
0.0011565449973354157,
0.4794677659305417,
0.0006279297983024605,
0.0018847921622940497,
0.0436545246275095,
0.12218605604654273,
0.0009468894693785477],
[0.0009720689558830165,
0.0008018909432627509,
0.0019240944568155803,
0.1066764236739401,
0.1349702789409835,
0.0011569919346681604,
0.19081915460748122,
0.0024683040072811927,
0.0014041530824945925,
0.4344681322251548,
0.0007623651167122712,
0.017053341146377386,
0.0013230496803440454,
0.0006949410320916781,
0.10450481019650971],
[0.0007337307415748742,
0.0006052780853678051,
0.3692321275591347,
0.2421210145287441,
0.023863488641184982,
0.0008733130968564548,
0.010294760887463518,
0.07987700505252171,
0.0010598736604724888,
0.07161102607274672,
0.17889291989338874,
0.0017272493269893112,
0.0009986557200743103,
0.0005245508518109689,
0.01758500588166929],
[0.0013794093058433455,
0.0220901544046519,
0.07606319962655893,
0.25613979081743943,
0.002958742056219769,
0.0016418232799309998,
0.24982867586806165,
0.11873992792161052,
0.0019925559983161893,
0.26034170280398466,
0.001081830183012049,
0.0032472181689522117,
0.0018774666448449697,
0.0009861523926652416,
0.0016313505279081486],
[0.0053016827695546755,
0.005193690566534555,
0.034014764273663324,
0.1520219507250445,
0.07159547622974148,
0.0007342082915266417,
0.04144873535033667,
0.13274140635541593,
0.2070232910147234,
0.23354300832400368,
0.098865080061121,
0.015506596932542473,
0.0008395858400593594,
0.00044099829272373686,
0.0007295249730085836],
[0.0005430768186838288,
0.008696947836652726,
0.14955598919853821,
0.08434496972961181,
0.0011648639866290444,
0.0006463898423251876,
0.20146998973600722,
0.0013789954711609328,
0.009033420475356256,
0.374712393387971,
0.1612803721115493,
0.0012784377380205727,
0.0007391632116357502,
0.004512723761627772,
0.0006422666942303172],
[0.0008693778474167097,
0.0007171777453621543,
0.0017208296664234395,
0.20765134109838373,
0.04148043096859016,
0.02744521375027601,
0.11783977973179943,
0.02861799646294301,
0.0012558158317007256,
0.32914663009901796,
0.0072844396278706385,
0.2331379958770451,
0.0011832803384591332,
0.0006215262146835991,
0.0010281647400281972],
[0.0007177327059646266,
0.0005920807912990945,
0.006871588022532671,
0.3349584762486754,
0.0015394893548029507,
0.000854271651226935,
0.0046193753299488965,
0.01272433008587652,
0.0664478262371936,
0.2444791396051542,
0.0005628966698703897,
0.05619880703164848,
0.19721006653468495,
0.0005131137090947655,
0.0717108060220265],
[0.0021082597544981705,
0.0017391712727228455,
0.004173048509169224,
0.19934089509009195,
0.036545027680654936,
0.178635549617402,
0.07761479858904038,
0.053387774840626866,
0.0030453800782978178,
0.23778595717025752,
0.0016534461717664481,
0.18108920091601433,
0.018880954687905762,
0.0015072142782066437,
0.002493321343345123],
[0.053572675789126147,
0.0009490201998268809,
0.0022771232437957586,
0.23983073820190895,
0.08983800241250255,
0.0188433589292335,
0.016141235360691703,
0.02039526777125525,
0.01913586868242425,
0.3656537087243942,
0.0009022422581049878,
0.002708167602754301,
0.0015658000412277777,
0.16682625064699735,
0.0013605401357564256],
[0.0015777541649665441,
0.28888095299526134,
0.015105451559315551,
0.2450401540013932,
0.003384178707862483,
0.001877900567349332,
0.09403185926409632,
0.004006276411074052,
0.0022790650403437715,
0.29777615981961264,
0.013219861762605273,
0.027679085786513125,
0.0021474270225246097,
0.001127950955694079,
0.0018659219413876833],
[0.03180808649652268,
0.001103245896497221,
0.06358859709932628,
0.2280212240004469,
0.002868587088699405,
0.0015917957609165602,
0.028921257653927744,
0.04402351769542229,
0.19491299694756103,
0.14068297444083666,
0.0010488660505669882,
0.013305176053117767,
0.2455859290647823,
0.0009561036303055868,
0.001581642121070617],
[0.04454359101549043,
0.0004775459123974538,
0.0011458458916873034,
0.2525766612170995,
0.2254616527322048,
0.009481957322020151,
0.23234222114031927,
0.023452286095598154,
0.0008362079289700408,
0.20597889078020462,
0.0004540073039845546,
0.0013627469352269271,
0.0007879088447816937,
0.0004138545920999484,
0.0006846222879151352],
[0.0011022692495805055,
0.18507874618719342,
0.05240984189574876,
0.38884764972524505,
0.10282035790002053,
0.0013119610742417826,
0.007094278045515762,
0.0027989121444279732,
0.09367695175373934,
0.11595117338963956,
0.00086447738097082,
0.03608016699657329,
0.009871599413116565,
0.0007880224188936876,
0.001303592425092926],
[0.0011306647827424405,
0.0009327217412510619,
0.002238015963605295,
0.3129948207494243,
0.0024252014467463405,
0.0013457584737473736,
0.04162500336926665,
0.011458007303702257,
0.018807229562740918,
0.15328615872774332,
0.0008867471631936678,
0.20016248385293672,
0.010125901461915592,
0.0008083226465708725,
0.24177296275441318],
[0.015986026436554203,
0.0004202674255671795,
0.03196154818879657,
0.04817034762689574,
0.0359150329281987,
0.000606374253095477,
0.0032788987688429543,
0.08641475914715638,
0.28705244571896266,
0.3283004895957952,
0.042960118194644334,
0.02441414863961249,
0.07420710940955895,
0.00036421545963572546,
0.019948218206683535],
[0.0004886593362404497,
0.0004031108016571216,
0.0009672428220664024,
0.1501172585035537,
0.15320701164448688,
0.0302711556955924,
0.07736888273467685,
0.004952009056198583,
0.008128252029181889,
0.5487033344217037,
0.0003832411575854546,
0.01228391130891363,
0.004376289385448231,
0.0003493470513721871,
0.008000294051322588],
[0.0005840264421545153,
0.040401019546771784,
0.17413937248436503,
0.20602707505049905,
0.02786552313198044,
0.000695129578119182,
0.08803277846412844,
0.028095800773673022,
0.058504746500918775,
0.29651553383692253,
0.04037727212873751,
0.0013748357837510734,
0.005230369155096343,
0.031465821590609795,
0.0006906955322724833],
[0.0009441870585218019,
0.0007788902694529082,
0.00186890556940843,
0.08927509666521995,
0.09524511445336001,
0.008294567070611972,
0.006076850572290329,
0.10995892334369595,
0.022876161258936427,
0.52956768115701,
0.0007404981639541379,
0.09544257246033504,
0.0012851005871568951,
0.0006750080073699129,
0.0369704433626762],
[0.0009178600379786612,
0.0007571722635348408,
0.008787611308389223,
0.21226052004311938,
0.09956018636010305,
0.0010924705029087703,
0.41021479025458457,
0.009301472177657463,
0.0013258483298189427,
0.23596892638673309,
0.0007198506553925402,
0.002160701525829752,
0.00822008471676188,
0.0006561865783782531,
0.008056318858809603],
[0.0007816428139040031,
0.0006448022946753411,
0.0787190179295066,
0.09171504612819104,
0.0016765723245761393,
0.006866635892403433,
0.0050307050262217564,
0.055411433645471506,
0.09610982020895173,
0.18314050265883966,
0.012485611687683843,
0.061202998416001894,
0.15540756577367548,
0.0005588036327402911,
0.25024884156715727],
[0.0011813876153939375,
0.0009745646371420428,
0.0023384157557682643,
0.255258390531444,
0.011506212701492194,
0.22571148367483523,
0.21396441389116796,
0.029916454021183734,
0.001706513773263599,
0.08838502694625319,
0.00989874171169937,
0.13736427420268624,
0.0016079461209513887,
0.0008445848658919542,
0.019341589550826915],
[0.0006568639659458149,
0.010519159446821167,
0.0013001838070326572,
0.21675603982574265,
0.0014089299187893894,
0.0007818234562208459,
0.13393240615644247,
0.016633863754224026,
0.08575581515640586,
0.3434730980397862,
0.0005151591057729774,
0.0015462999964649302,
0.0008940349909484401,
0.015435534942368996,
0.17039078743703354],
[0.007374606642702827,
0.0007078306824623708,
0.014731520889057147,
0.28314371046197856,
0.0018404545742191755,
0.007537838512792141,
0.18798611436207385,
0.13902652621336045,
0.0012394486345235138,
0.3053071443996549,
0.0006729411591211052,
0.015053017125732444,
0.03375065600613639,
0.0006134257895657249,
0.001014764546619337],
[0.0004886593362404497,
0.026381454387038265,
0.004678434762835137,
0.3096985119566093,
0.004759334013737505,
0.0005816201694425235,
0.021701003623145837,
0.001240817115429849,
0.0007058681476444186,
0.3408765857386546,
0.0003832411575854546,
0.00486152742737616,
0.0006650974446794964,
0.2749775506682585,
0.008000294051322588],
[0.001025040592803845,
0.0008455889501166258,
0.048737833724400235,
0.17476813466428473,
0.03333790334397748,
0.05571374365125552,
0.061090932226796485,
0.18165354926994548,
0.0014806705834568565,
0.38808057720657374,
0.04751279745073857,
0.002413011441032137,
0.0013951475566019486,
0.0007328109422565454,
0.0012122583957598296],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.1294034699905887,
0.01841715630801397],
[0.0010752650267627977,
0.0008870207008977187,
0.3042796539586764,
0.47731638160052925,
0.002306372621083846,
0.001279819663066185,
0.129414245399027,
0.01906284472844269,
0.0015532197512223753,
0.03961425316136085,
0.0008432987625656629,
0.0025312429868003033,
0.0014635063092323021,
0.017101219294941253,
0.001271656035391333],
[0.0017684700017416548,
0.0014588677780994353,
0.13780941261221938,
0.3149528096323294,
0.0037932516093307496,
0.3378772409040744,
0.05167467150752527,
0.044783228472650906,
0.002554553963704199,
0.07858377847898326,
0.0013869590538000667,
0.004163092054385936,
0.0024070037998249884,
0.001264295460518152,
0.015522364670812234],
[0.08639070578140319,
0.0004904843096808544,
0.0011768908844038651,
0.3226380521326684,
0.005790910709096375,
0.0007076852471646933,
0.09865402835294004,
0.001509761891271431,
0.2627628409138061,
0.13479462409598333,
0.000466307957630667,
0.0013996685395941068,
0.032418356758069214,
0.018487410620950506,
0.03231227180533729],
[0.0013373777266104862,
0.001103245896497221,
0.23625594679549541,
0.2889626415402713,
0.002868587088699405,
0.0015917957609165602,
0.00860745180731961,
0.12527874108185483,
0.001931841404783755,
0.24225200367387734,
0.0010488660505669882,
0.0031482731298137015,
0.04244787059870094,
0.0009561036303055868,
0.04220925381428688],
[0.08062466738863991,
0.001227969574062455,
0.01425160407357234,
0.11813750012445742,
0.03710835550354081,
0.0017717507662917045,
0.1339372616027917,
0.01508497521400061,
0.00215023910310506,
0.1905028753234552,
0.2046602628948489,
0.19569185451821094,
0.0020260420273922465,
0.0010641926440819313,
0.001760449241548768],
[0.27728168995720914,
0.0010706232473256631,
0.1208476995797425,
0.08328677597895041,
0.032353462912506466,
0.01140129319953715,
0.15620142745155957,
0.0032954900836140812,
0.001874717435772456,
0.2745149502645942,
0.0010178513970756477,
0.032624878721547915,
0.0017664343974003334,
0.0009278319336673835,
0.0015348734394970294],
[0.0009374647228724892,
0.0007733447985867396,
0.3436015578487384,
0.3805474901753257,
0.002010800050453078,
0.0011158047140947096,
0.0985897822243929,
0.009500143460365517,
0.001354167286574431,
0.1057345737241215,
0.029214055895336825,
0.0022068522141817496,
0.015515365977757057,
0.007789909613242802,
0.001108687293956086],
[0.0005840264421545153,
0.00048178219431382934,
0.15196201839966617,
0.09070483381006494,
0.0500428772166793,
0.000695129578119182,
0.19448407807068302,
0.0635795673091912,
0.0008436258807017214,
0.24328988403364527,
0.18231233827081025,
0.005810306600690846,
0.01410131078897589,
0.0004175258720313801,
0.0006906955322724833],
[0.001214066201136415,
0.0010015222534581858,
0.011623495344382275,
0.5112698392234251,
0.011824488222106584,
0.001445025884601019,
0.007813810645450414,
0.24281309197149406,
0.06629649157689095,
0.04472788070794303,
0.0009521564447915292,
0.09506195216450758,
0.0016524238220023454,
0.0008679470872299913,
0.0014358084505805538],
[0.0009178600379786612,
0.0007571722635348408,
0.1412331330418679,
0.3935017603099849,
0.0437936508933752,
0.02200492130293172,
0.04076149278751243,
0.0023306552443164794,
0.12680055312995664,
0.22202729252005113,
0.0007198506553925402,
0.002160701525829752,
0.0012492677834208962,
0.0006561865783782531,
0.0010855019254686186],
[0.05264140146347434,
0.0004354300948312387,
0.0010447912385524337,
0.15413539536319829,
0.0011321765637468612,
0.008645722785449617,
0.4483668588025478,
0.05345386327738294,
0.0007624609244001142,
0.2639791404500534,
0.0004139674077317548,
0.0012425633050817096,
0.000718421441991373,
0.0003773558512515183,
0.012650451030306589],
[0.0015055913203197073,
0.035545284737465446,
0.002980138287643687,
0.10805390979216074,
0.003229394161717557,
0.0017920097169793,
0.021124510339669608,
0.25538038149147324,
0.02504367520870522,
0.23841886481959154,
0.0011807910289593172,
0.0035442587415124537,
0.1278278801242846,
0.012510785753981561,
0.16186252447553598],
[0.0009243031862811307,
0.0007624874237799859,
0.03692829945692699,
0.09441478032577524,
0.02304182040520787,
0.0853371433066052,
0.01998837730648755,
0.10062352045134487,
0.001335155454066794,
0.4482178791688993,
0.0007249038272666946,
0.00217586911107396,
0.0012580373313530672,
0.18317430136696558,
0.0010931218779657477],
[0.0006806319884541681,
0.000561475216272618,
0.0013472297702650166,
0.16256929667224826,
0.05315146278578831,
0.000810113023724003,
0.19047017993997786,
0.06892729694282569,
0.026828948552758657,
0.16981177101113873,
0.041887041287424,
0.19803014914439698,
0.0009263848303212256,
0.0004865900651063213,
0.0835114287692982],
[0.000547593891289293,
0.00045172781144112184,
0.07594191322992146,
0.1432694155713225,
0.005333331503658434,
0.10046245488224861,
0.3487029822265856,
0.0013904653451300272,
0.000790999080626543,
0.2114779374650908,
0.00042946179724921555,
0.09694098117861691,
0.0007453112440675867,
0.00039147990651959075,
0.013123944866232303],
[0.0013373777266104862,
0.001103245896497221,
0.002647179559501883,
0.095981485997494,
0.002868587088699405,
0.011748698684220628,
0.05939196642383995,
0.00339590600220602,
0.2355406086407773,
0.48601767383317496,
0.0010488660505669882,
0.08440349651624623,
0.0018202589054846744,
0.011113006553609653,
0.001581642121070617],
[0.0008990585208941215,
0.02122574080331444,
0.00177957901642255,
0.25570874208844585,
0.001928421278226662,
0.0010700922513509085,
0.14234692374820815,
0.002282913919298463,
0.0012986895485307885,
0.12188690161998224,
0.0007051051780477748,
0.0977088079535461,
0.0012236776840579484,
0.3488720799708758,
0.001063266418798185],
[0.0005713552935023467,
0.0004713293562888973,
0.0011309295975834089,
0.1494862164932686,
0.00556475745089755,
0.013697761912829775,
0.14687213456779966,
0.00145080094575398,
0.005164560412677722,
0.4115809404601383,
0.01780504916422809,
0.10982595706962148,
0.02247384206963357,
0.0004084671513831869,
0.11349589805439342],
[0.0012727421350499791,
0.0010499259183504872,
0.03151729766544983,
0.20733491790812272,
0.002729948004369178,
0.011180882969624146,
0.2981720182855802,
0.13855604541488356,
0.06950060726253117,
0.14354977187610074,
0.05899428734255234,
0.0029961167927590286,
0.0017322856210428364,
0.029907951595277246,
0.0015052012083065678],
[0.024765067636172197,
0.000858962680734347,
0.0020610350403606806,
0.3198751367083974,
0.025957233138533292,
0.0012393367229550374,
0.2123079680235651,
0.13707892916135042,
0.0015040886869148132,
0.25187552101931504,
0.0008166237439783096,
0.018267052084375488,
0.0014172130384077278,
0.000744400989801639,
0.0012314313251384913],
[0.0021082597544981705,
0.0017391712727228455,
0.3083910682294019,
0.3274326928670321,
0.06856797712488996,
0.0025093276741093755,
0.07761479858904038,
0.005353350674274337,
0.0030453800782978178,
0.17374005828178749,
0.0016534461717664481,
0.004962978972721721,
0.0028694799657882518,
0.0015072142782066437,
0.018504796065462632],
[0.003821535478465839,
0.0031525075160489242,
0.007564273281375208,
0.41938150099186583,
0.1533128805845222,
0.004548530945129069,
0.256781158089973,
0.06775012265538857,
0.005520205937526132,
0.053721097741750094,
0.0029971179754569883,
0.008996140149556917,
0.005201360729203382,
0.0027320508421828666,
0.004519517081555029],
[0.0008206140791599731,
0.0006769509446935538,
0.1698955664228191,
0.18977182139969245,
0.0017601631202946288,
0.0009767248149600291,
0.2795013558549573,
0.15165817793268274,
0.0011853765947542586,
0.1985038182956164,
0.00064358350757764,
0.0019317782881833588,
0.0011169096477648295,
0.0005866645485066018,
0.000970494548337137],
[0.0009115060958508604,
0.0007519306923320339,
0.0018042175051121735,
0.16233320644539936,
0.02272280358931884,
0.14645868939102752,
0.12355005119859393,
0.030004765251980984,
0.0013166700638422876,
0.2897159053148341,
0.0007148674452997505,
0.2167451357965702,
0.0012406196509502168,
0.0006516440867438675,
0.0010779874721438688],
[0.0005945753282217145,
0.0004904843096808544,
0.010208062511054546,
0.09685876146640134,
0.046431183029024437,
0.0007076852471646933,
0.3470112480858338,
0.09633706397110357,
0.0008588637409363245,
0.32896481406897293,
0.0049818937709560085,
0.05107111248617285,
0.0008092560647918323,
0.00042506736764914485,
0.014249928552035931],
[0.0009441870585218019,
0.0007788902694529082,
0.0162104279441699,
0.25420260397497685,
0.0020252190174104502,
0.4743940442503597,
0.09929674600823989,
0.0023975055329849195,
0.0013638776967942196,
0.11366353228892741,
0.0007404981639541379,
0.03090572177390843,
0.0012851005871568951,
0.0006750080073699129,
0.0011166374257725283],
[0.0008105114289062284,
0.0006686169435999072,
0.0016043106181983938,
0.31054638122601563,
0.032516207846950605,
0.0009647002720594302,
0.2822159335593791,
0.2913685866374053,
0.0011707833219115164,
0.04217144954878114,
0.0006356602958618589,
0.03268571024580034,
0.001103159277373973,
0.0005794420709738006,
0.0009585467067828556],
[0.0020750353779958896,
0.0017117634160564127,
0.11442131753067795,
0.2592360426348523,
0.0044508141383508715,
0.0024697828090924708,
0.12366909811698572,
0.16286046172923932,
0.0029973874843596026,
0.2813160957801022,
0.0016273892696129944,
0.036403061714507225,
0.0028242594076735837,
0.0014834618660373611,
0.002454028724456143],
[0.07207054962016747,
0.0007032479255123578,
0.0016874058082509311,
0.6179777159182313,
0.0018285387923801554,
0.16934825801884887,
0.01843543176812017,
0.002164670504434877,
0.014180161767101062,
0.08967629994776567,
0.0006685842898156042,
0.0020068205596932035,
0.007634666248353181,
0.0006094542447173579,
0.001008194586607899],
[0.0172586492336657,
0.05570862562502241,
0.2439204080348216,
0.25047435957396785,
0.034622468962497016,
0.006374689328298964,
0.12591233171971591,
0.0018425739168239773,
0.028603201187477605,
0.20859677636494356,
0.0005691009190953646,
0.0017082115783723594,
0.006498650424649491,
0.01154077303373803,
0.006369180096910108],
[0.0010579854763849024,
0.0008727662440839018,
0.18689959870402295,
0.21252527296978915,
0.002269309124267439,
0.0012592529118075194,
0.006809264742211033,
0.002686465579629547,
0.009563278700073875,
0.25592317033932954,
0.0008297469189841413,
0.10694581606523526,
0.0014399877064977917,
0.000756363542367268,
0.21016172097531563],
[0.0011605619939717737,
0.009771435927714987,
0.002297193924235001,
0.18024626145546424,
0.002489328994569307,
0.10714995541045944,
0.05153970909434156,
0.0029469306710001777,
0.001676431174351433,
0.22785178292015845,
0.2477036230994966,
0.13494280276164566,
0.001579601082671125,
0.000829696437702287,
0.027814685052217993],
[0.08259260111304302,
0.001103245896497221,
0.012804082482805951,
0.23817812692375095,
0.002868587088699405,
0.011748698684220628,
0.2320593161200091,
0.08465112938863856,
0.04255945309800002,
0.24225200367387734,
0.0010488660505669882,
0.0031482731298137015,
0.0018202589054846744,
0.0009561036303055868,
0.04220925381428688],
[0.13413147645183202,
0.0006298464740085478,
0.00151128294871837,
0.05479612543093169,
0.06542236333382775,
0.0009087610935497137,
0.19046944803248023,
0.007737340280844277,
0.006901501301216148,
0.3238578409843042,
0.06438347899880702,
0.04238760853780167,
0.041629441134430824,
0.006344449606065274,
0.05888903539118232],
[0.0021082597544981705,
0.0017391712727228455,
0.3244025429515194,
0.3274326928670321,
0.00452207823641992,
0.0025093276741093755,
0.09362627331115789,
0.08541072428486189,
0.0030453800782978178,
0.07767120994908243,
0.0016534461717664481,
0.03698592841695674,
0.034892429410023275,
0.0015072142782066437,
0.002493321343345123],
[0.0019519886683892953,
0.0016102582281428877,
0.003863728549177803,
0.22903905412865144,
0.10795945117382555,
0.09127124022798126,
0.10151104072069884,
0.18285236707331573,
0.12141686271429422,
0.131212574720728,
0.0015308873511404725,
0.004595106791531532,
0.01748143677292578,
0.0013954946422596866,
0.002308508236937499],
[0.0009243031862811307,
0.0007624874237799859,
0.008849298145751814,
0.22779003655385732,
0.00900231974962028,
0.14149514592895557,
0.09018588058442549,
0.0023470158622317444,
0.232986916271262,
0.20252661769611655,
0.00774465415506049,
0.009195619438867753,
0.008277787659146862,
0.0076805431721207115,
0.050231374172522304],
[0.030502125642813124,
0.008184405114995276,
0.0019240944568155803,
0.49056716060403155,
0.11282273642578593,
0.10451219033892353,
0.0431688711728307,
0.03938087486594383,
0.0014041530824945925,
0.1613151078710513,
0.0007623651167122712,
0.0022883128029123297,
0.0013230496803440454,
0.0006949410320916781,
0.0011496117922543388],
[0.025600092057672875,
0.035462387538548225,
0.0013001838070326572,
0.21176739420739724,
0.0014089299187893894,
0.0007818234562208459,
0.11896646930140625,
0.0016679268991877892,
0.3252108048369856,
0.19381372948942385,
0.0005151591057729774,
0.0015462999964649302,
0.08071236488447504,
0.0004695980873327599,
0.0007768364132895302],
[0.008543807466726531,
0.07631727270240853,
0.00951739555115118,
0.07134394589007997,
0.017231691820465626,
0.0011831968369176412,
0.02149744421740621,
0.1912672580295695,
0.0014359559785801486,
0.38391069734273187,
0.000779632050701391,
0.1986329121343715,
0.0013530156520209062,
0.015810124786434877,
0.001175649540434075],
[0.007727084692852751,
0.12364613338269388,
0.00177957901642255,
0.22839663740061134,
0.01558447362214392,
0.0010700922513509085,
0.012614426480994196,
0.07056317563888476,
0.0012986895485307885,
0.4154920270142033,
0.0007051051780477748,
0.008944467718083911,
0.11047209643539603,
0.000642745200985689,
0.001063266418798185],
[0.06410144142491556,
0.0016852060059899094,
0.004043561736118847,
0.1310968872101933,
0.004381761315342018,
0.0024314649935456363,
0.5871899115666545,
0.03621653969869298,
0.002950884067004339,
0.07526112669384913,
0.001602140894886942,
0.06686758070482292,
0.018295091885382524,
0.001460446474584997,
0.0024159553280165198],
[0.03930265680046632,
0.022642614274821487,
0.08410135317551715,
0.23394135389886742,
0.007067459503996247,
0.02841869689509958,
0.08182431658611469,
0.0018425739168239773,
0.0010481917289768346,
0.2526847914985448,
0.0005691009190953646,
0.062329232387074045,
0.0009876485329493368,
0.1823818316764428,
0.0008581782052099534],
[0.0014397408854694624,
0.0011876885582199328,
0.0028497952127955074,
0.1251965567573139,
0.0030881493185905477,
0.0017136321270408209,
0.09674078233829393,
0.036458772244876675,
0.14422579090882007,
0.5669548210839322,
0.0011291464679985593,
0.014323556891135578,
0.001959581886419037,
0.0010292839935247802,
0.001702701325568909],
[0.0011920832414871007,
0.0009833878030756174,
0.02951991713739239,
0.10366081751829787,
0.0025569399931996894,
0.010472304519559317,
0.0529395446358027,
0.0030269702824759995,
0.010775407154909876,
0.4965901757974135,
0.0009349158555336334,
0.002806240572876033,
0.0016225035703977446,
0.28150898148852577,
0.0014098104290528048],
[0.0009579251636902121,
0.0007902232742212089,
0.03099648713268376,
0.12694955244903747,
0.0020546863476382207,
0.00114015747720127,
0.006165269928922413,
0.0024323897043540526,
0.06685959678650198,
0.4645220222589996,
0.1171528270524857,
0.06045579465516236,
0.11770535354676886,
0.0006848295050393313,
0.0011328847172936201],
[0.003821535478465839,
0.0031525075160489242,
0.007564273281375208,
0.3613351254609761,
0.06624331728818769,
0.004548530945129069,
0.024595655966414304,
0.12579649818627825,
0.005520205937526132,
0.28590659986530875,
0.0029971179754569883,
0.06704251568044658,
0.005201360729203382,
0.0027320508421828666,
0.033542704846999866],
[0.0009115060958508604,
0.01459705274774595,
0.0018042175051121735,
0.19694601158393416,
0.0019551205061979675,
0.01493002986459533,
0.1581628563371287,
0.0023145211411531533,
0.0013166700638422876,
0.3866317597027315,
0.06994047772236932,
0.09906159832555193,
0.0012406196509502168,
0.04910957128069257,
0.0010779874721438688],
[0.022521419949475194,
0.09870867338709663,
0.0014206662116550293,
0.13327436924620273,
0.0015394893548029507,
0.0063051934621045766,
0.015521218951704179,
0.08903723543816348,
0.001036764506661912,
0.5170252301490362,
0.0005628966698703897,
0.012591432544627355,
0.09364255212800977,
0.0005131137090947655,
0.006299744291494797],
[0.03845495547108083,
0.0005856956291402618,
0.028366033207841826,
0.12105282663846346,
0.0015228870780247333,
0.011629334091293785,
0.24182361180857992,
0.23366474779151977,
0.017201996463863806,
0.1717448208836252,
0.04369392679970076,
0.08255343148554202,
0.0063584839562055165,
0.0005075801496774745,
0.0008396685454406551],
[0.0010016476683084978,
0.0008262913743883605,
0.0019826419870840606,
0.19360113196456535,
0.002148468238676207,
0.001192197597298404,
0.40962582525623925,
0.0025434110806715907,
0.03948264879832182,
0.28033101109580266,
0.008392716687998746,
0.0023579429931650433,
0.008970462106592199,
0.0007160871255004884,
0.04682751602538731],
[0.0007337307415748742,
0.0006052780853678051,
0.17419738770910784,
0.31456248933018266,
0.12416706913548452,
0.0008733130968564548,
0.14403286821319622,
0.0018631091125109608,
0.0010598736604724888,
0.0994731317656077,
0.0061478645976506575,
0.040734197296994686,
0.09015739393722946,
0.0005245508518109689,
0.0008677424659527011],
[0.0010665552684985334,
0.0008798357412013391,
0.002111118833273848,
0.05224467493756993,
0.018487898242645646,
0.001269452991027462,
0.023064627972067434,
0.22141102719675412,
0.0015406385101071913,
0.39569793814039084,
0.000836467955105466,
0.00251073965601727,
0.0014516517563040677,
0.2761660173094532,
0.0012613554895836673],
[0.0026624456000032067,
0.022416653937136214,
0.005269993234055819,
0.3932826826620932,
0.0057107703534815665,
0.0031689398854407554,
0.1384575782100135,
0.06742150530318453,
0.20604907061267044,
0.1385288180925819,
0.0020880778449947623,
0.006267568073923903,
0.0036237632924073886,
0.001903406833390164,
0.0031487260646226355],
[0.0005364392310654656,
0.20821974206907967,
0.07439495251267775,
0.18516566607046409,
0.07855782495187169,
0.0006384895433869057,
0.15826695479996108,
0.03802870867391156,
0.0007748861799494103,
0.21124412825989317,
0.0004207135250284459,
0.0012628124301289577,
0.0373966965653071,
0.00038350533738880626,
0.004708479849885899],
[0.0006408783900829178,
0.0005286811945079246,
0.0012685422679004203,
0.20661377957652613,
0.05004705109154869,
0.0007627968406371838,
0.03819542227794114,
0.10870663608386269,
0.03986367583243408,
0.2864418572752965,
0.1903250178884894,
0.001508668923393818,
0.0738808913417717,
0.00045816985220447086,
0.0007579311634029426],
[0.029627231808044746,
0.0007788902694529082,
0.009039666756789164,
0.11078738022736215,
0.14544044276502516,
0.0011238058832312363,
0.07778446244609769,
0.2462033859039299,
0.01570540007155569,
0.314444845535588,
0.0007404981639541379,
0.03807648296128917,
0.00845586177453763,
0.0006750080073699129,
0.0011166374257725283],
[0.0006055122992142174,
0.0004995065687835009,
0.0011985393128756002,
0.14462692068473035,
0.3829865825165979,
0.0007207028290465576,
0.07287683997177409,
0.015333477871835446,
0.010071958539968945,
0.35800922871245366,
0.0004748855025843623,
0.010622711222628603,
0.0008241420004942215,
0.0004328863087473949,
0.0007161056582651267],
[0.0007958154014945261,
0.049007948162096616,
0.10432206228006109,
0.17194911805291815,
0.044014494229580245,
0.0009472085240881596,
0.04742944337917206,
0.002020756174422491,
0.0011495549182415094,
0.30129587309034267,
0.10941490713271562,
0.04418092324561142,
0.01921495243559355,
0.10331577643966365,
0.0009411665339982622],
[0.0008470079865913857,
0.0006987241277568265,
0.2975817209179983,
0.26663549926979374,
0.0018167763123431975,
0.013873581968265025,
0.12124037907169352,
0.06647795659168741,
0.0012235025798035693,
0.15985936921235955,
0.0006642834735601595,
0.0019939112427733137,
0.0011528334889479269,
0.0006055337955495302,
0.06532891996087659],
[0.014801761953547345,
0.0014207050996532951,
0.2519204233606608,
0.2413161576150994,
0.003694023534240365,
0.002049835274548289,
0.25959576500904735,
0.0697708462988867,
0.0024877291129852663,
0.11576675764567848,
0.001350677443374007,
0.030213296379439404,
0.0023440387296449973,
0.0012312226201655284,
0.002036759923028764],
[0.0005386336585570413,
0.004535065175698704,
0.001066161027375328,
0.3659152023504119,
0.0011553337009669454,
0.0947278672441951,
0.08528126094237531,
0.0013677132777737578,
0.0007780560292774427,
0.15483806545297552,
0.2458661714538933,
0.013540165098686736,
0.02118676052092998,
0.008566532050569963,
0.0006370120163129578],
[0.0009115060958508604,
0.0007519306923320339,
0.008726778532819132,
0.28693930494412456,
0.01580024256161188,
0.0010849078091814146,
0.019711635782989564,
0.16153342477841315,
0.11900020753486057,
0.2412579781208854,
0.014559989500713666,
0.002145743937654536,
0.12584671814967546,
0.0006516440867438675,
0.0010779874721438688],
[0.0007863105863705676,
0.0006486528902976949,
0.007528154091895221,
0.23558465276215565,
0.0076583305414098975,
0.0009358955463694727,
0.03491947788213185,
0.0019966212886029745,
0.09668376365800249,
0.3872735404361329,
0.0006166802862312924,
0.049624994881304285,
0.0010702203415226814,
0.12596880986879067,
0.04870389493878231],
[0.0005114357720247591,
0.0004218998160214908,
0.001012326057749818,
0.2969443794245518,
0.07101207007261554,
0.000608729514251293,
0.06155419627653695,
0.02071950556406644,
0.03569630571979319,
0.24412390759745714,
0.0004011040468587998,
0.03616148970336591,
0.2298621737086985,
0.0003656301346817859,
0.0006048465913267184],
[0.029584996803883153,
0.00062621529753551,
0.0015025701348598377,
0.20437482085159867,
0.03045412917891763,
0.000903521924897219,
0.06253746157149516,
0.0019275560365335323,
0.0010965358166561048,
0.3162255660662046,
0.0005953486598192447,
0.32463691472280565,
0.024093908711657095,
0.0005426956231856716,
0.0008977585999509097],
[0.0007138416111568596,
0.022274352282131815,
0.07731214908425282,
0.1271304691880011,
0.0015311432128683495,
0.0008496403282303828,
0.18892092371617308,
0.0018126060900504517,
0.17993636520794204,
0.38953072696323954,
0.0005598449985570444,
0.0016804290355903408,
0.0009715853075458372,
0.0005103319296486564,
0.006265591044611684],
[0.0005408461134097952,
0.00044616135246105626,
0.0010705403176431377,
0.03470813911963035,
0.3297626190646998,
0.004751266522950091,
0.21707257234670735,
0.001373331203483586,
0.0007812519191189741,
0.2253021074287322,
0.12365012213605368,
0.05877863097835307,
0.0007361270752774289,
0.000386655858086068,
0.0006396285633934535],
[0.0009374647228724892,
0.0007733447985867396,
0.0018555995084737437,
0.15271685128181595,
0.009130507515875259,
0.2004676137459158,
0.055871537431859804,
0.002380435994943336,
0.001354167286574431,
0.5471564365802967,
0.0007352260336481023,
0.0022068522141817496,
0.015515365977757057,
0.0006702021478206216,
0.008228394759378267],
[0.19695588639978534,
0.012461637172857155,
0.0010447912385524337,
0.25435378768008093,
0.005140912256422167,
0.08481170094628042,
0.07956317507641973,
0.0013402992726039699,
0.024814875080451947,
0.32010144014750763,
0.00442270310040706,
0.013268770383107626,
0.000718421441991373,
0.0003773558512515183,
0.0006242439522806736],
[0.015572862094219599,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.2184982617047336,
0.03954303620063915,
0.022494990895988647,
0.33718523733438593,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.01841715630801397],
[0.04444649599401836,
0.0006769509446935538,
0.02655338298828441,
0.32688173598924736,
0.0017601631202946288,
0.0009767248149600291,
0.1299269035754428,
0.00208372565316826,
0.007417645439734029,
0.19227154945063663,
0.00064358350757764,
0.13280942403275853,
0.0011169096477648295,
0.10030296606818294,
0.032131838773235985],
[0.002417983623875596,
0.001994672453233688,
0.004786109935116351,
0.10007999903290916,
0.005186415525040604,
0.0028779723229021936,
0.2542906128452453,
0.20814068746555855,
0.0034927760405661598,
0.30944645999036885,
0.0018963535009198682,
0.09751066954816712,
0.0032910344901815177,
0.001728638719493378,
0.0028596145064216953],
[0.05870144593925908,
0.0010398744721037425,
0.0024951232139097035,
0.32980525907969027,
0.1271590710488859,
0.020647324390747925,
0.00811303212884185,
0.03192128633327389,
0.0018208747182888068,
0.2474837961641078,
0.1254438764037828,
0.04126135910929009,
0.0017157016168768315,
0.0009011841884000138,
0.0014907911925412668],
[0.016977952646214253,
0.0005888709020552902,
0.23453188908980763,
0.1271304691880011,
0.0015311432128683495,
0.0008496403282303828,
0.20518503475123046,
0.0018126060900504517,
0.0010311438223107267,
0.3841093566182204,
0.0005598449985570444,
0.012523169725628603,
0.006392955652564969,
0.005931702274667787,
0.0008442206995925524],
[0.0011306647827424405,
0.0009327217412510619,
0.002238015963605295,
0.10690700202279939,
0.0024252014467463405,
0.0013457584737473736,
0.10173395049786559,
0.020044999750644964,
0.001633244668855508,
0.10176420404608709,
0.19838757344287591,
0.4577722572612179,
0.0015389090149728866,
0.0008083226465708725,
0.00133717424001742],
[0.0005195071906247365,
0.00042855818879991667,
0.09966506002174784,
0.17143020037440146,
0.06029636322035661,
0.0006183364111334399,
0.09803487000965824,
0.10390137468528615,
0.13489641813066275,
0.3268860481508933,
0.00040743422327865644,
0.0012229533186066135,
0.0007070834001360781,
0.00037140046603365684,
0.0006143922083805271],
[0.01814880601644362,
0.009438664447870674,
0.0022189617557684563,
0.26776060026266646,
0.002404553563458652,
0.0013343008424909545,
0.007215077763238792,
0.0028465713699064804,
0.010133223204676472,
0.5095642174131777,
0.000879197500894119,
0.045208415485654456,
0.12072017999833153,
0.0008014406814922261,
0.0013257896939294137],
[0.009625746954872396,
0.0004904843096808544,
0.0011768908844038651,
0.10588993309305202,
0.09610262697560319,
0.03683237175376742,
0.030920241153059934,
0.05118120583785018,
0.15890436720732323,
0.4012141870821784,
0.0049818937709560085,
0.0013996685395941068,
0.09563655814462399,
0.00042506736764914485,
0.005218756925385252],
[0.0007296647423405847,
0.0006019239118376687,
0.27297980974120206,
0.21861312557234663,
0.007106624122981601,
0.14494854834493093,
0.049028501399875704,
0.001852784616349593,
0.0010540003267715252,
0.1765034760048973,
0.0005722546153631652,
0.04050846705886471,
0.012076204301097552,
0.0005216440315825682,
0.0729029712095584],
[0.11947780609442984,
0.00046132045994059205,
0.0011069137857770575,
0.12507634632249415,
0.035176233207445076,
0.0006656067834822551,
0.10977649879773528,
0.06937346897211387,
0.0008077963110641547,
0.3178989837220989,
0.00043858161666396377,
0.16270595181718706,
0.055973337890953295,
0.00039979316296016165,
0.0006613610556543307],
[0.17722057945117886,
0.08923528823413734,
0.003807286846549004,
0.4886388889432126,
0.004125724623319712,
0.002289388734956259,
0.026987697413063335,
0.004884137235008215,
0.017386549056114343,
0.17312008574328816,
0.0015085240076675864,
0.004527981048153317,
0.0026179741994795187,
0.0013751091279524605,
0.0022747853359192365],
[0.0014397408854694624,
0.3948230035694385,
0.0028497952127955074,
0.026787728004509254,
0.0030881493185905477,
0.0017136321270408209,
0.009266267891356471,
0.0036558293272751304,
0.00207970493254671,
0.46854599233112765,
0.012063460773865742,
0.025257871197002763,
0.01289389619228622,
0.0010292839935247802,
0.03450564424317045],
[0.001408929528862659,
0.0011622712792675454,
0.00278880781051779,
0.17601883769639814,
0.045823313516997005,
0.0016769593263361905,
0.0946704690109938,
0.0035775922901574724,
0.22674177371560506,
0.23381223889073718,
0.09740780021403642,
0.11031984199093522,
0.0019176456068342547,
0.0010072566714599313,
0.0016662624508613439],
[0.0005763571955909842,
0.000475455586182045,
0.031781409795287686,
0.08513650158837048,
0.06689463290821553,
0.018194903949424197,
0.00808669880948792,
0.058367435324132015,
0.022718675893623547,
0.32326236716813356,
0.2805944614252847,
0.08452406917908824,
0.0007844599899787468,
0.01354371999843314,
0.005058851188767203],
[0.016857129260276535,
0.032490716184316995,
0.0020610350403606806,
0.0747290470556319,
0.07340486339390725,
0.0012393367229550374,
0.06996507725744319,
0.0026439767711241963,
0.0015040886869148132,
0.33095490477827166,
0.3250420971557004,
0.06571468233974945,
0.0014172130384077278,
0.000744400989801639,
0.0012314313251384913],
[0.0006316551368978868,
0.010115459889575722,
0.10199135209825368,
0.3283673149489544,
0.0013548586417752339,
0.3125694047184523,
0.0760232787819407,
0.05437304584567438,
0.005709619131189435,
0.018473856654891513,
0.0004953885619417059,
0.06864766756522939,
0.01045411137250224,
0.00045157606371975796,
0.010341410589001681],
[0.0004886593362404497,
0.08204933349856929,
0.0009672428220664024,
0.24660824896354083,
0.0010481420729687694,
0.0005816201694425235,
0.05881292303083318,
0.001240817115429849,
0.08977447472609405,
0.25551917110097366,
0.0003832411575854546,
0.008572719368144896,
0.004376289385448231,
0.24528801514210866,
0.0042891021105538555],
[0.0008006545027086768,
0.0006604856481826538,
0.001584800009467225,
0.27636629780396477,
0.007798034183303637,
0.043517749472845424,
0.2544610701216993,
0.050678508065304606,
0.0011565449973354157,
0.20583702916943256,
0.1161609075418819,
0.038368890397108606,
0.0010897433535591867,
0.0005723952638275365,
0.0009468894693785477],
[0.057339716460094024,
0.00044073041000757305,
0.4839038667746026,
0.18847188279548466,
0.0011459580930769442,
0.024981093357908546,
0.06024400347403121,
0.12308258672855987,
0.012944339307425359,
0.027798039562350347,
0.0004190064662620541,
0.017487818198784384,
0.0007271664968628483,
0.0003819492520499683,
0.0006318426224995765],
[0.0005055449037943752,
0.0004170402493690324,
0.001000665787313945,
0.2436114735780288,
0.0010843605025917266,
0.13498182939288614,
0.28353223862584703,
0.08191176025208886,
0.058321735657261294,
0.16068394950597506,
0.00039648401201578426,
0.005029516952393296,
0.0006880798109195077,
0.0003614186988333688,
0.02747390207068192],
[0.0013511007255191903,
0.02163681477029677,
0.00267434259764987,
0.18931648143090582,
0.09524813950501375,
0.0016081293748651795,
0.060001394765738396,
0.02395300014601916,
0.0019516642693059771,
0.3781323957122358,
0.001059628595344661,
0.003180577951305109,
0.19680029594949852,
0.0009659143283710803,
0.022120119877930743],
[0.13667933700312798,
0.0004927091802653558,
0.0011822293424507326,
0.5146164510379435,
0.0012811098572678243,
0.0007108953561496817,
0.01291622220952968,
0.03326909202451694,
0.0099348972486599,
0.23519957585718165,
0.00046842316261841974,
0.0014060175324167748,
0.05070968394077174,
0.0004269955024825086,
0.0007063607446173949],
[0.0008309717579563114,
0.000685495327033656,
0.0016448093970881346,
0.11012498342141788,
0.17217753531302193,
0.0009890529021360681,
0.2199198668745888,
0.008420957844587452,
0.02644406501978823,
0.4218919155524004,
0.0006517067306850642,
0.0019561609298207354,
0.001131007128748731,
0.025837796111887797,
0.00729367568883878],
[0.006449918529572095,
0.023416918363205984,
0.0014854424571634779,
0.41862466031088597,
0.0016096834226186313,
0.000893222750195016,
0.06182460201113595,
0.001905583978278495,
0.01248295711399249,
0.4437085230937485,
0.0005885623277035096,
0.018865007816039617,
0.006720883225672969,
0.0005365094788550334,
0.0008875251209322889],
[0.0020116321619637278,
0.0016594600641159762,
0.003981785724728106,
0.11381641550027059,
0.0501476871282906,
0.002394317988271609,
0.10461273552200878,
0.0051079912556618555,
0.0181834244763228,
0.5782728713906854,
0.01685528689378245,
0.00473551140905459,
0.0027379634672503703,
0.0014381343240757961,
0.09404478269351732],
[0.00088101180588888,
0.12785516432985944,
0.001743857698407849,
0.37101334666027425,
0.0018897122638417147,
0.001048612392764794,
0.01905218617543611,
0.008928057069256227,
0.0012726210784390836,
0.012384791820588447,
0.0006909516697930582,
0.002073958419031186,
0.40265718657675575,
0.04746661846517238,
0.001041923574490774],
[0.0006702382912044729,
0.0005529011211519951,
0.10822125168439516,
0.2822520082222358,
0.10833221196216596,
0.0007977420660707404,
0.03485501098076045,
0.006792106160072329,
0.0009681588501566421,
0.40645891783965865,
0.0005256481960531033,
0.0066680028271576,
0.041633988800935856,
0.0004791595154007293,
0.000792653482580496],
[0.0014397408854694624,
0.0011876885582199328,
0.0028497952127955074,
0.07052498522797798,
0.0030881493185905477,
0.3953489471382594,
0.19514961109109857,
0.0036558293272751304,
0.11142284799121853,
0.18425382037858093,
0.0011291464679985593,
0.014323556891135578,
0.01289389619228622,
0.0010292839935247802,
0.001702701325568909],
[0.025222612038584346,
0.0005338782223661319,
0.02094135922065929,
0.12508835428401036,
0.21273688472412375,
0.0007702952659114814,
0.3727967882624712,
0.05079420028852183,
0.0009348487570665894,
0.17129554865137692,
0.0005075629507028033,
0.016268759622284298,
0.0008808522123596467,
0.00046267374133545015,
0.0007653817582259245],
[0.0007504582185372428,
0.0006190771190665769,
0.0014854424571634779,
0.2704386922239798,
0.0016096834226186313,
0.000893222750195016,
0.06182460201113595,
0.15009155206518462,
0.13787108395675923,
0.2784241740737378,
0.0005885623277035096,
0.007466087193969912,
0.001021422914638116,
0.0005365094788550334,
0.08637942978645508],
[0.0007910344432636261,
0.048713526927341586,
0.0015657582501965115,
0.3331219106907193,
0.0016967167506323026,
0.024972006640968304,
0.017106394469975944,
0.002008616234875451,
0.0011426488266555502,
0.4316534885632112,
0.02465087365616367,
0.049923123099595325,
0.049137626996522986,
0.000565517794899981,
0.012950756654978263],
[0.00161649334898042,
0.0013334973496961148,
0.0031996556143767917,
0.2756100873290907,
0.10168075736433003,
0.0019240093574597675,
0.010403858477190038,
0.09004144395604008,
0.002335023770758114,
0.02272379719848317,
0.0012677682310516828,
0.052912072075184814,
0.002200153595795113,
0.43083964571571737,
0.0019117366158458418],
[0.0009308374326541273,
0.0007678777337532055,
0.001842481578623515,
0.1304291117648091,
0.0019965849496814815,
0.0011079166714975826,
0.04840718497022504,
0.002363607798852515,
0.0013445941694283886,
0.38776211948410977,
0.08556253539669001,
0.3344519033757354,
0.0012669308697412253,
0.0006654642371236012,
0.0011008495670750747],
[0.0006536033703561519,
0.07996129951718332,
0.0012937298472986084,
0.0866191415478146,
0.09571570493531979,
0.0007779425763957847,
0.20772581993021164,
0.12575671168907784,
0.010871894850615049,
0.267309899406192,
0.06007919272437971,
0.016430272046846443,
0.005853479673568232,
0.040178327596180924,
0.0007729802885599384],
[0.01859053111124916,
0.0006448022946753411,
0.01341976083924102,
0.4360202198768641,
0.0016765723245761393,
0.0009303397932883805,
0.010967001125336807,
0.0019847687534360306,
0.0011290826231108828,
0.19501309485706977,
0.0006130194894537365,
0.03152151792042664,
0.18508904626925074,
0.0005588036327402911,
0.10184143908928098],
[0.0008580471424749677,
0.0007078306824623708,
0.034281199389740724,
0.4330245789672194,
0.021390133074902753,
0.2682002185219065,
0.05765492435751667,
0.0021787767085754152,
0.007756008134751373,
0.116326918893047,
0.052805417160943974,
0.0020198981252767248,
0.0011678585049970957,
0.0006134257895657249,
0.001014764546619337],
[0.0026624456000032067,
0.10329792168891092,
0.06593094404788684,
0.0697576116549944,
0.04615140422936891,
0.06382989069927178,
0.11823726127206984,
0.006760554489353497,
0.0038459012332336786,
0.28007103665818767,
0.0020880778449947623,
0.006267568073923903,
0.023844080230351065,
0.001903406833390164,
0.2053518954440594],
[0.0014241685786064394,
0.19586371069003097,
0.05689921286491005,
0.3617954859288024,
0.00305474774660404,
0.0882234832786511,
0.03079813996072419,
0.09014467358028594,
0.023689307213520198,
0.09573253629054482,
0.03356507827009387,
0.014168632608905446,
0.0019383869542152546,
0.001018151208203386,
0.0016842848259018947],
[0.0005664394622246906,
0.00046727412897574913,
0.005423103324909461,
0.13099244966535184,
0.0012149753173964976,
0.0006741969129920521,
0.22734465145473715,
0.0014383185328877709,
0.0008182214976785011,
0.46396452941911737,
0.1165956505944955,
0.0013334349025744675,
0.0007709613036143699,
0.00040495277841508844,
0.047990840704629466],
[0.012554044536721288,
0.0004354300948312387,
0.0010447912385524337,
0.32250229445556117,
0.0011321765637468612,
0.0006282514000990055,
0.06753696799839382,
0.0013402992726039699,
0.0007624609244001142,
0.364197532766936,
0.0004139674077317548,
0.0012425633050817096,
0.1650765848416789,
0.0605083912413811,
0.0006242439522806736],
[0.0005074933900312224,
0.0235440264587387,
0.05881796968424284,
0.12892351397870616,
0.035776608137776965,
0.0006040371473751598,
0.2422285091570442,
0.0012886410585398154,
0.03542114218880209,
0.3732859017440493,
0.062065689060272634,
0.001194672059809699,
0.008399191442106147,
0.00036281168955512377,
0.02757979280295006],
[0.0009374647228724892,
0.029252174660275465,
0.0018555995084737437,
0.24527304833230426,
0.002010800050453078,
0.0011158047140947096,
0.1270686120860816,
0.023739558391209878,
0.008473874751996612,
0.2908469678250982,
0.16448849773835827,
0.0022068522141817496,
0.10095185556282323,
0.0006702021478206216,
0.001108687293956086],
[0.001699973511002808,
0.014313050339282981,
0.029186269668058814,
0.01871891228390916,
0.10693183130452738,
0.053666120568765845,
0.24333351768647224,
0.0043166191082282465,
0.054098360896688166,
0.24337900440488375,
0.001333239268963335,
0.22348353473938287,
0.002313775578073582,
0.001215326689649966,
0.0020104639521108525],
[0.0014719299754449471,
0.0012142423737357916,
0.09234374062814826,
0.10563808826510267,
0.03669352940316363,
0.00175194475626383,
0.5013397092596261,
0.1378829111740514,
0.002126202055655343,
0.1101218337212028,
0.0011543914253521563,
0.003465017772058605,
0.002003393351588136,
0.0010522962698394118,
0.0017407695687668807],
[0.0010412525084852137,
0.22228123720581286,
0.0020610350403606806,
0.21707193782175382,
0.00223341801084631,
0.0012393367229550374,
0.022517447002069226,
0.018459853522915518,
0.0015040886869148132,
0.24396758264341936,
0.0008166237439783096,
0.02617499046027115,
0.0014172130384077278,
0.2379825522666715,
0.0012314313251384913],
[0.008608801524644836,
0.0008262913743883605,
0.0019826419870840606,
0.140351054970211,
0.10864862222738493,
0.07726373616066179,
0.01405382472674969,
0.05579348807502595,
0.1155541873616852,
0.25750954952679367,
0.0007855628316624075,
0.13167955855088279,
0.0013633082502558604,
0.0235375486945095,
0.06204182373805999],
[0.0007099924789860977,
0.0005856956291402618,
0.03915030834844032,
0.22350344047414913,
0.0015228870780247333,
0.0008450589506952904,
0.15554941068379197,
0.1581748218073303,
0.13582902301044725,
0.16635268331332595,
0.0005568262373067808,
0.05559274363404578,
0.01714275909680401,
0.04364468071207145,
0.0008396685454406551],
[0.0011022692495805055,
0.0009092973527507742,
0.010553148978829977,
0.22142087805756996,
0.0023642948994154634,
0.0013119610742417826,
0.2666057741304122,
0.044655605061346755,
0.00159222733651802,
0.2582639293071634,
0.00086447738097082,
0.002594812663038266,
0.0015002608297328079,
0.0007880224188936876,
0.18547304125953556],
[0.0006055122992142174,
0.009696802919116228,
0.0011985393128756002,
0.3377701440417176,
0.11626498835694885,
0.0007207028290465576,
0.21543493340193137,
0.05212266327316635,
0.000874662189636218,
0.15566870900513372,
0.0004748855025843623,
0.07040513749979133,
0.033014679226658766,
0.005031534483913758,
0.0007161056582651267],
[0.21499198335621764,
0.0007267749718517647,
0.001743857698407849,
0.25057592516321436,
0.0018897122638417147,
0.001048612392764794,
0.012361218314488335,
0.015619024930204001,
0.0012726210784390836,
0.28671447411944717,
0.0006909516697930582,
0.002073958419031186,
0.20861911860927035,
0.000629843438537968,
0.001041923574490774],
[0.08203439678644382,
0.0005888709020552902,
0.012255704944023255,
0.20302965401826892,
0.12080129080328925,
0.0008496403282303828,
0.1618140719910774,
0.023498087470126976,
0.011873884512348989,
0.35700250489312474,
0.011402585688595307,
0.012523169725628603,
0.0009715853075458372,
0.0005103319296486564,
0.0008442206995925524],
[0.0012727421350499791,
0.23303437826656473,
0.002519241121923043,
0.09134269173401557,
0.002729948004369178,
0.020846901817466408,
0.046855528241681425,
0.0998919700235145,
0.011504494175477607,
0.375534224224315,
0.0009981742554987761,
0.0029961167927590286,
0.03073034216456962,
0.07823804583448855,
0.0015052012083065678],
[0.03816640383171737,
0.0008078549438343119,
0.0019384047574076964,
0.19671887360698123,
0.0021005310500431377,
0.06066496527439425,
0.036052515205529594,
0.15123508258235913,
0.031164280511956063,
0.355887823234674,
0.0007680351470699035,
0.06180470025226456,
0.0013328897578708844,
0.0007001096011438889,
0.060657530242753985],
[0.03797754090405332,
0.019253762853253902,
0.044969924861548015,
0.15143442826050854,
0.0017492612538803401,
0.013358011784245742,
0.11673484296057644,
0.10736317981715195,
0.07550205363789207,
0.20966168797959484,
0.0006395973648473705,
0.0019198134943543225,
0.007303660119328587,
0.000583030942925349,
0.2115492037658392],
[0.0007910344432636261,
0.0006525497517671285,
0.0015657582501965115,
0.28506093351514483,
0.1398720261304089,
0.0009415180531810709,
0.23338079176006105,
0.08010770414518395,
0.0011426488266555502,
0.15530286980365807,
0.03065849580311048,
0.0018621459240208706,
0.06716049343736341,
0.000565517794899981,
0.000935512361084649],
[0.0026096771018971517,
0.00215280656807988,
0.005165544291328178,
0.2665706382428116,
0.084875822082382,
0.003106132893875226,
0.4131872384255467,
0.006626563279630038,
0.003769677166179794,
0.0366854421358677,
0.0020466930625944128,
0.08542158431745832,
0.003551941901415603,
0.0018656821490312875,
0.08236455638190214],
[0.0013239306911037624,
0.001092153004453591,
0.0026205627579648084,
0.41676929427981013,
0.0330040766559439,
0.011630568132753377,
0.0185756833184244,
0.013416538446937763,
0.25328185527116187,
0.1593779934795619,
0.0010383199357759801,
0.013171395467984997,
0.0018019566071538068,
0.031110822793242707,
0.04178484915772695],
[0.0005278374586953719,
0.128714972260441,
0.0010447912385524337,
0.24633631629473032,
0.0011321765637468612,
0.0006282514000990055,
0.1316767390811987,
0.07750627743343476,
0.024814875080451947,
0.3241101758401829,
0.012440174485757672,
0.04934739161718537,
0.000718421441991373,
0.0003773558512515183,
0.0006242439522806736],
[0.37282610677660105,
0.001439533555881558,
0.0034540838234210654,
0.13849113140035238,
0.0037429800421304197,
0.0020770015272430957,
0.2100245785385244,
0.004431034512175474,
0.042279385240140945,
0.2100712709183878,
0.001368577830391183,
0.004107919030413992,
0.0023751040299872317,
0.0012475398848932268,
0.002063752889456199],
[0.006037025109474075,
0.3259905159996287,
0.0013903514240465487,
0.13576511103743955,
0.022845069840456058,
0.04884751207325681,
0.09520913589313008,
0.060464282087731004,
0.0010146415790089393,
0.16457783070496082,
0.01655470841775507,
0.006988143439776046,
0.11298279778472539,
0.0005021646677347359,
0.0008307099408762157],
[0.009413216031034857,
0.00047965469989025815,
0.03647798014284756,
0.09913606034139387,
0.04540600937026403,
0.0006920599663349312,
0.056732845863361143,
0.0014764272221770803,
0.0008399005264276192,
0.43209856857111684,
0.00045601214770623553,
0.001368764668010853,
0.0007913881590721335,
0.00041568212609197563,
0.3142154301642706],
[0.0008206140791599731,
0.0006769509446935538,
0.0016243076083653299,
0.21470089677961152,
0.05785058272511256,
0.0009767248149600291,
0.24210774278507868,
0.16412271562264227,
0.0011853765947542586,
0.16734247407071756,
0.11905669156219328,
0.008164047133163129,
0.01981371618270414,
0.0005866645485066018,
0.000970494548337137],
[0.0012253645477922296,
0.02892945195062723,
0.039650275280556126,
0.2926789455695768,
0.08638415418492397,
0.0014584735889811961,
0.00788652755437396,
0.08686730706621876,
0.29956853792343385,
0.128899955433436,
0.000961017406058512,
0.012190790016329573,
0.0109740047229324,
0.0008760243791118095,
0.0014491703756475908],
[0.11300145328879584,
0.0006769509446935538,
0.0016243076083653299,
0.1399136706398543,
0.10147646463997095,
0.0009767248149600291,
0.260804549320018,
0.02701280103308734,
0.0011853765947542586,
0.26082650674541413,
0.00064358350757764,
0.0019317782881833588,
0.01358144733772437,
0.0005866645485066018,
0.07575772068809439],
[0.0005763571955909842,
0.1230377737046824,
0.0011408302656625978,
0.054495922058745386,
0.0012362482018760514,
0.0006860013610670012,
0.3101152684586495,
0.0014635019119711315,
0.08837706059996303,
0.2444723055205262,
0.0004520200115695875,
0.14142800259124913,
0.005161685637068046,
0.0004120430571652428,
0.026944979424213696],
[0.0008006545027086768,
0.0006604856481826538,
0.03806889824428178,
0.12434922182557082,
0.019959400261575156,
0.061759798590252706,
0.05987921286935496,
0.06892055718271188,
0.0011565449973354157,
0.2666438595607902,
0.2864200326376831,
0.0018847921622940497,
0.007170426392694947,
0.0005723952638275365,
0.06175371986073615],
[0.00061976265113767,
0.0005112621423737953,
0.0012267461833631772,
0.23746123949118564,
0.00132935004354939,
0.0007376641177924166,
0.09812632302927778,
0.001573718228638119,
0.00089524681530382,
0.4040897470020568,
0.02872730847711108,
0.15207894427548801,
0.0008435376652184255,
0.07104619112202187,
0.0007329587554819737],
[0.004199851277009185,
0.0004031108016571216,
0.09374704134128475,
0.5323700284027333,
0.0010481420729687694,
0.02284877181405493,
0.05510173109006444,
0.12742134310156683,
0.05637374725917544,
0.08851553376638065,
0.0003832411575854546,
0.0011503354866074257,
0.0006650974446794964,
0.015194114814447124,
0.0005779101697851205],
[0.0008206140791599731,
0.03807056401457218,
0.09510834028306188,
0.20846862793463175,
0.014224700810254167,
0.0009767248149600291,
0.055139677435685555,
0.05194187641300642,
0.10713394695941035,
0.36054280826509044,
0.00064358350757764,
0.06425446673798106,
0.0011169096477648295,
0.0005866645485066018,
0.000970494548337137],
[0.0008580471424749677,
0.07890654468519667,
0.0016984018886014283,
0.21146155595947214,
0.0018404545742191755,
0.0010212790125642818,
0.012039007855921656,
0.028245014709486854,
0.007756008134751373,
0.4486714534046678,
0.0006729411591211052,
0.028086136126188162,
0.17711496501114932,
0.0006134257895657249,
0.001014764546619337],
[0.0008415942455465081,
0.0070858638230499775,
0.07197349765145228,
0.2137984249872468,
0.0018051641945743026,
0.01378490750317134,
0.011808162083505169,
0.0021369990639195265,
0.26966312033799467,
0.3953270207448774,
0.0006600376355477944,
0.008372772612765686,
0.0011454650318898736,
0.0006016634623119188,
0.0009953066221467665],
[0.0009308374326541273,
0.0007678777337532055,
0.001842481578623515,
0.13749848734414322,
0.0019965849496814815,
0.0011079166714975826,
0.2463497011915805,
0.03771048569552313,
0.022552720907430757,
0.19688897884208847,
0.0007300284446805396,
0.0021912511470316134,
0.0012669308697412253,
0.311717989727825,
0.03644772746374569],
[0.0007462052513234392,
0.0006155687096372843,
0.0014770242162644412,
0.21223446719124117,
0.18861685938272485,
0.000888160713458837,
0.05580707151094936,
0.16057528025053314,
0.012412214191834067,
0.22017469403325252,
0.0005852268504961548,
0.0017566151247497854,
0.13136032709770182,
0.0005334689934994158,
0.012216816482333734],
[0.00161649334898042,
0.0013334973496961148,
0.0031996556143767917,
0.11601317313204744,
0.0034672717046111273,
0.0019240093574597675,
0.20683082979662787,
0.04093470112618062,
0.002335023770758114,
0.3296409398851048,
0.0012677682310516828,
0.2861691005170172,
0.002200153595795113,
0.0011556459544470605,
0.0019117366158458418],
[0.0012253645477922296,
0.05684806127828719,
0.021037869062116157,
0.06933007094829713,
0.002628326201944101,
0.0014584735889811961,
0.03580513688203392,
0.18923554126763859,
0.0017700384283942886,
0.43600465803769556,
0.000961017406058512,
0.040109399343989535,
0.1412608482520122,
0.0008760243791118095,
0.0014491703756475908],
[0.020821924700280537,
0.00044073041000757305,
0.025402703568055407,
0.31831292016371043,
0.0011459580930769442,
0.008750963686880324,
0.2347178974375846,
0.1474277812351022,
0.0048292744719112485,
0.21038699836141783,
0.0004190064662620541,
0.025602883034298494,
0.0007271664968628483,
0.0003819492520499683,
0.0006318426224995765],
[0.0010171224827424186,
0.0008390570464820244,
0.0020132725349401117,
0.10389599126982152,
0.009906340203169994,
0.00893529579333834,
0.045169665339854025,
0.0025827051515788157,
0.11733942548788612,
0.3464593815879142,
0.0007976992738773531,
0.3577296290387727,
0.0013843704888617462,
0.0007271502125881397,
0.0012028940881724994],
[0.0021082597544981705,
0.0017391712727228455,
0.004173048509169224,
0.3594556423112671,
0.00452207823641992,
0.16262407489528447,
0.09362627331115789,
0.005353350674274337,
0.0030453800782978178,
0.20576300772602252,
0.0016534461717664481,
0.1490662514717793,
0.0028694799657882518,
0.0015072142782066437,
0.002493321343345123],
[0.19898136984151144,
0.0006942581586640128,
0.0016658353432066622,
0.009267043726895912,
0.15520370013983747,
0.0010016961743994086,
0.414479318939821,
0.03409502738584935,
0.02678210509132797,
0.08213834318996507,
0.013443248964319726,
0.008372772612765686,
0.00753707069627584,
0.0006016634623119188,
0.045736546272848524],
[0.006881337541844437,
0.0006604856481826538,
0.062391630400824814,
0.10610717270816354,
0.09292759673120427,
0.061759798590252706,
0.029475797673676158,
0.002033043752218531,
0.0011565449973354157,
0.3335313729912835,
0.0006279297983024605,
0.014046158240565569,
0.2868818461929399,
0.0005723952638275365,
0.0009468894693785477],
[0.0009510064990138774,
0.0007845158452267454,
0.08855303246621082,
0.18381307174174846,
0.06704281773917377,
0.001131922630094234,
0.2589100744434571,
0.03130503118509972,
0.0013737283749070063,
0.17948744457654567,
0.0007458464507348127,
0.08890935905405402,
0.0012943822934685322,
0.09457306430218927,
0.0011247023980760057],
[0.0008751561641214331,
0.0007219444646414595,
0.0017322671545501782,
0.23561748045213607,
0.0018771522981447242,
0.03427412463113462,
0.23161343964493702,
0.1019196659134993,
0.09431511175018571,
0.23163685646981447,
0.04721183383359051,
0.0020601738619330273,
0.014484137741661922,
0.0006256571864117162,
0.0010349984332378482],
[0.0013651082709869133,
0.19810874400143502,
0.002702068861743506,
0.1705442083982888,
0.0029280672789089683,
0.001624801666509275,
0.02952094030366572,
0.003466320156874962,
0.0019718981611792337,
0.3405826600138568,
0.0010706143016268376,
0.0032135526136859785,
0.0018580019973208604,
0.21869356361078332,
0.022349450363133856],
[0.1338431498235277,
0.012846554761838653,
0.030824621495110654,
0.17147740106794468,
0.03340276292083453,
0.018535388599407066,
0.10022797397542552,
0.03954303620063915,
0.022494990895988647,
0.21891494960507782,
0.01221333811367245,
0.03665951833203013,
0.021195687909900382,
0.011133182261280586,
0.13668744403732208],
...])
When the topic model finishes, it will output your results to your output_directory_path
.
Display Topics and Top Words#
To examine the 15 topics that the topic model extracted from the Reddit posts, run the cell below. This code uses the little_mallet_wrapper.load_topic_keys()
function to read and process the MALLET topic model output from your computer, specifically the file “mallet.topic_keys.15”.
topics = little_mallet_wrapper.load_topic_keys(path_to_topic_keys)
for topic_number, topic in enumerate(topics):
print(f"✨Topic {topic_number}✨\n\n{topic}\n")
✨Topic 0✨
['baby', 'pregnant', 'child', 'husband', 'pregnancy', 'pain', 'birth', 'hospital', 'doctor', 'could', 'sil', 'think', 'abortion', 'would', 'medical', 'surgery', 'help', 'weeks', 'control', 'children']
✨Topic 1✨
['table', 'store', 'manager', 'drink', 'order', 'restaurant', 'bar', 'drinking', 'drinks', 'pay', 'tip', 'place', 'wine', 'people', 'coffee', 'bottle', 'food', 'alcohol', 'beer', 'ordered']
✨Topic 2✨
['money', 'pay', 'buy', 'would', 'paying', 'college', 'job', 'bought', 'house', 'gift', 'paid', 'car', 'give', 'afford', 'since', 'year', 'got', 'rent', 'working', 'much']
✨Topic 3✨
['time', 'get', 'like', 'want', 'work', 'one', 'doesn', 'would', 'going', 'take', 'even', 'home', 'really', 'think', 'day', 'every', 'make', 'feel', 'something', 'week']
✨Topic 4✨
['sister', 'mom', 'dad', 'family', 'parents', 'brother', 'mother', 'cousin', 'aunt', 'year', 'father', 'also', 'house', 'siblings', 'older', 'nephew', 'always', 'close', 'birthday', 'years']
✨Topic 5✨
['school', 'work', 'office', 'class', 'name', 'people', 'company', 'high', 'teacher', 'boss', 'job', 'coworkers', 'students', 'team', 'college', 'year', 'one', 'student', 'english', 'coworker']
✨Topic 6✨
['years', 'told', 'would', 'never', 'wife', 'life', 'family', 'ago', 'relationship', 'time', 'know', 'want', 'together', 'love', 'months', 'married', 'feel', 'even', 'things', 'much']
✨Topic 7✨
['kids', 'daughter', 'son', 'husband', 'wife', 'old', 'child', 'year', 'kid', 'name', 'mother', 'children', 'mom', 'two', 'told', 'school', 'think', 'house', 'baby', 'parents']
✨Topic 8✨
['room', 'house', 'dog', 'door', 'sleep', 'bed', 'bathroom', 'apartment', 'roommate', 'living', 'live', 'cat', 'use', 'stuff', 'home', 'dogs', 'girlfriend', 'bedroom', 'moved', 'place']
✨Topic 9✨
['said', 'told', 'didn', 'got', 'asked', 'like', 'really', 'went', 'back', 'one', 'friend', 'could', 'friends', 'get', 'know', 'started', 'wasn', 'would', 'left', 'aita']
✨Topic 10✨
['wedding', 'dress', 'hair', 'wear', 'ring', 'married', 'fiancé', 'party', 'wearing', 'one', 'would', 'think', 'tattoo', 'family', 'husband', 'getting', 'makeup', 'engaged', 'fiance', 'ceremony']
✨Topic 11✨
['people', 'like', 'girls', 'gay', 'think', 'girl', 'women', 'said', 'always', 'group', 'girlfriend', 'friends', 'woman', 'one', 'white', 'doesn', 'really', 'look', 'know', 'thing']
✨Topic 12✨
['car', 'seat', 'back', 'get', 'minutes', 'around', 'front', 'woman', 'guy', 'next', 'move', 'people', 'away', 'flight', 'door', 'phone', 'lady', 'sit', 'park', 'drive']
✨Topic 13✨
['food', 'eat', 'eating', 'vegan', 'dinner', 'make', 'meat', 'cook', 'weight', 'cooking', 'meal', 'ate', 'chicken', 'lunch', 'fridge', 'eats', 'diet', 'cake', 'family', 'hungry']
✨Topic 14✨
['phone', 'facebook', 'post', 'pictures', 'social', 'account', 'game', 'sent', 'picture', 'video', 'posted', 'media', 'call', 'also', 'message', 'messages', 'number', 'instagram', 'photos', 'sarah']
Load Topic Distributions#
MALLET also calculates the likely mixture of these topics for every single Reddit post in the corpus. This mixture is really a probability distribution, that is, the probability that each topic exists in the document. We can use these probability distributions to examine which of the above topics are strongly associated with which specific posts.
To get the topic distributions, we’re going to use the little_mallet_wrapper.load_topic_distributions()
function, which will read and process the MALLET topic model output, specifically the file “mallet.topic_distributions.15”.
topic_distributions = little_mallet_wrapper.load_topic_distributions(path_to_topic_distributions)
topic_distributions[0]
[0.011076196974682066,
0.000564392649472897,
0.0013542299952905628,
0.1530219806345308,
0.1521519084626053,
0.000814322382504041,
0.08234356736699737,
0.006933273680468054,
0.000988281118714402,
0.33177055578329395,
0.2551412694103212,
0.0016105767704565042,
0.0009311983390601434,
0.0004891183939972939,
0.000809128037605374]
It’s a bit easier to understand if we pair these probabilities with the topics themselves. As you can see below, Topic 8 “‘room’, ‘house’, ‘dog’, ‘door’, ‘sleep’, ‘bed’” has a relatively high probability of existing in the Reddit post “AITA for walking my cat on a trail frequented by dogs?” while Topic 10 “‘wedding’, ‘dress’, ‘hair’, ‘wear’, ‘ring’, ‘married’” has a relatively low probability.
reddit_post_to_check = "AITA for walking my cat on a trail frequented by dogs?"
reddit_post_number = reddit_titles.index(reddit_post_to_check)
print(f"Topic Distributions for {reddit_titles[reddit_post_number]}\n")
for topic_number, (topic, topic_distribution) in enumerate(zip(topics, topic_distributions[reddit_post_number])):
print(f"✨Topic {topic_number} {topic[:6]} ✨\nProbability: {round(topic_distribution, 3)}\n")
Topic Distributions for AITA for walking my cat on a trail frequented by dogs?
✨Topic 0 ['baby', 'pregnant', 'child', 'husband', 'pregnancy', 'pain'] ✨
Probability: 0.002
✨Topic 1 ['table', 'store', 'manager', 'drink', 'order', 'restaurant'] ✨
Probability: 0.002
✨Topic 2 ['money', 'pay', 'buy', 'would', 'paying', 'college'] ✨
Probability: 0.024
✨Topic 3 ['time', 'get', 'like', 'want', 'work', 'one'] ✨
Probability: 0.177
✨Topic 4 ['sister', 'mom', 'dad', 'family', 'parents', 'brother'] ✨
Probability: 0.024
✨Topic 5 ['school', 'work', 'office', 'class', 'name', 'people'] ✨
Probability: 0.003
✨Topic 6 ['years', 'told', 'would', 'never', 'wife', 'life'] ✨
Probability: 0.035
✨Topic 7 ['kids', 'daughter', 'son', 'husband', 'wife', 'old'] ✨
Probability: 0.006
✨Topic 8 ['room', 'house', 'dog', 'door', 'sleep', 'bed'] ✨
Probability: 0.509
✨Topic 9 ['said', 'told', 'didn', 'got', 'asked', 'like'] ✨
Probability: 0.091
✨Topic 10 ['wedding', 'dress', 'hair', 'wear', 'ring', 'married'] ✨
Probability: 0.002
✨Topic 11 ['people', 'like', 'girls', 'gay', 'think', 'girl'] ✨
Probability: 0.006
✨Topic 12 ['car', 'seat', 'back', 'get', 'minutes', 'around'] ✨
Probability: 0.022
✨Topic 13 ['food', 'eat', 'eating', 'vegan', 'dinner', 'make'] ✨
Probability: 0.02
✨Topic 14 ['phone', 'facebook', 'post', 'pictures', 'social', 'account'] ✨
Probability: 0.078
Explore Heatmap of Topics and Texts#
We can visualize and compare these topic probability distributions with a heatmap by using the little_mallet_wrapper.plot_categories_by_topics_heatmap()
function.
We have everything we need for the heatmap except for our list of target_labels
, the sample of texts that we’d like to visualize and compare with the heatmap. Below we make our list of desired target labels by randomly selecting 6 Reddit post titles.
target_labels = random.sample(reddit_titles, 6)
little_mallet_wrapper.plot_categories_by_topics_heatmap(reddit_titles,
topic_distributions,
topics,
output_directory_path + '/categories_by_topics.pdf',
target_labels=target_labels,
dim= (18, 8)
)
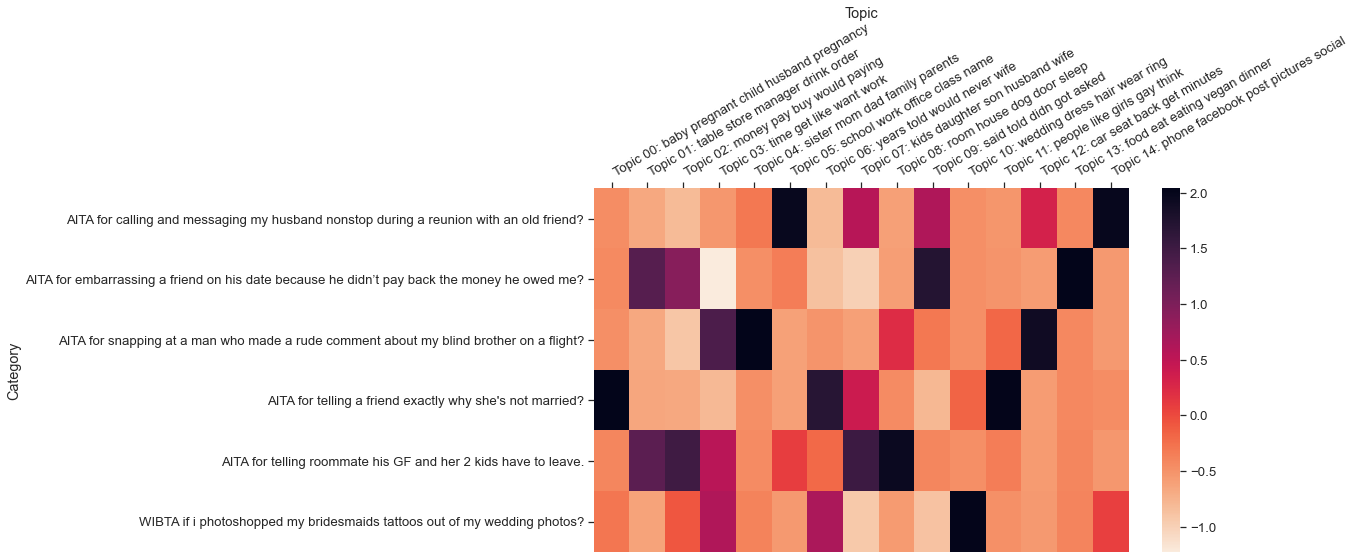
The plot_categories_by_topics_heatmap()
function also helpfully outputs a PDF of the heatmap to output_directory_path + '/categories_by_topics.pdf'
. We can download this PDF and explore it in more detail or embed it in an article or blog post!
Show code cell source
from IPython.display import IFrame
IFrame("topic-model-output/reddit/categories_by_topics.pdf", width=1000, height=600)
Display Top Titles Per Topic#
We can also display the Reddit posts and titles that have the highest probability for every topic with the little_mallet_wrapper.get_top_docs()
function.
training_data_reddit_titles = dict(zip(training_data, reddit_titles))
training_data_original_text = dict(zip(training_data, original_texts))
We’ll make our own function display_top_titles_per_topic()
that will display the top Reddit post titles for every topic. This function accepts a given topic_number
as well as a desired number_of_documents
to display.
def display_top_titles_per_topic(topic_number=0, number_of_documents=5):
print(f"✨Topic {topic_number}✨\n\n{topics[topic_number]}\n")
for probability, document in little_mallet_wrapper.get_top_docs(training_data, topic_distributions, topic_number, n=number_of_documents):
print(round(probability, 4), training_data_reddit_titles[document] + "\n")
return
Topic 0
To display the top 5 Reddit post titles with the highest probability of containing Topic 0, we will run:
display_top_titles_per_topic(topic_number=0, number_of_documents=5)
✨Topic 0✨
['baby', 'pregnant', 'child', 'husband', 'pregnancy', 'pain', 'birth', 'hospital', 'doctor', 'could', 'sil', 'think', 'abortion', 'would', 'medical', 'surgery', 'help', 'weeks', 'control', 'children']
0.4107 AITA for offering my DNA because my brother won't do a paternity test
0.378 AITA for causing a scene in the hospital over an IV?
0.3728 AITA for lying about my birth control use?
0.363 AITA for asking my immediate family to get the flu shot if they want to see our newborn in the hospital
0.3542 AITA for feeling jealous that my sister is getting more attention for her illness than I am for mine?
What would you label this topic?
To display the top 5 Reddit post titles with the highest probability of containing Topic 9, we will run:
display_top_titles_per_topic(topic_number=10, number_of_documents=5)
✨Topic 10✨
['wedding', 'dress', 'hair', 'wear', 'ring', 'married', 'fiancé', 'party', 'wearing', 'one', 'would', 'think', 'tattoo', 'family', 'husband', 'getting', 'makeup', 'engaged', 'fiance', 'ceremony']
0.4522 AITA for showing up at my cousin's wedding in a hoodie and jean shorts?
0.438 AITA? I wore an accessory ring to work knowing that my co-worker has the same ring for her engagement ring.
0.4347 AITA for not including a bridesmaid in photographs because she wore a suit?
0.4049 AITA for dressing nicer than the couple getting married?
0.3982 AITA for getting a tattoo before my brother's wedding?
What would you label this topic?
To display the top 7 Reddit posts with the highest probability of containing Topic 8, we will run:
display_top_titles_per_topic(topic_number=8, number_of_documents=7)
✨Topic 8✨
['room', 'house', 'dog', 'door', 'sleep', 'bed', 'bathroom', 'apartment', 'roommate', 'living', 'live', 'cat', 'use', 'stuff', 'home', 'dogs', 'girlfriend', 'bedroom', 'moved', 'place']
0.5773 AITA for refusing to eat my GF cooking because her cats litter is disgusting?
0.5279 AITA for peeing in places that are not the toilet?
0.5087 AITA for walking my cat on a trail frequented by dogs?
0.5039 Aita for not wanting all 3 of my triplet children to have to share a room
0.4808 WIBTA if I told my roommate his girlfriend is getting too comfortable in our home?
0.4544 AITA for turning off the dryer on someone who removed my laundry before it was done?
0.4374 AITA for not allowing my roommate's girlfriend to use my bathroom causing her to piss herself
What would you label this topic?
Display Topic Words in Context of Original Text#
Often it’s useful to actually look at the document that has ranked highly for a given topic and puzzle out why it ranks so highly.
To display the original Reddit post texts that rank highly for a given topic, with the relevant topic words bolded for emphasis, we are going to make the function display_bolded_topic_words_in_context()
.
In the cell below, we’re importing two special Jupyter notebook display modules, which will allow us to make the relevant topic words bolded, as well as the regular expressions library re
, which will allow us to find and replace the correct words.
from IPython.display import Markdown, display
import re
def display_bolded_topic_words_in_context(topic_number=3, number_of_documents=3, custom_words=None):
for probability, document in little_mallet_wrapper.get_top_docs(training_data, topic_distributions, topic_number, n=number_of_documents):
print(f"✨Topic {topic_number}✨\n\n{topics[topic_number]}\n")
probability = f"✨✨✨\n\n**{probability}**"
reddit_title = f"**{training_data_reddit_titles[document]}**"
original_text = training_data_original_text[document]
topic_words = topics[topic_number]
topic_words = custom_words if custom_words != None else topic_words
for word in topic_words:
if word in original_text:
original_text = re.sub(f"\\b{word}\\b", f"**{word}**", original_text)
display(Markdown(probability)), display(Markdown(reddit_title)), display(Markdown(original_text))
return
Topic 3
To display the top 3 original Reddit posts with the highest probability of containing Topic 0 and with relevant topic words bolded, we will run:
display_bolded_topic_words_in_context(topic_number=3, number_of_documents=3)
✨Topic 3✨
['time', 'get', 'like', 'want', 'work', 'one', 'doesn', 'would', 'going', 'take', 'even', 'home', 'really', 'think', 'day', 'every', 'make', 'feel', 'something', 'week']
✨✨✨
0.7187352724737381
AITA for setting my boyfriend up to fail?
My boyfriend has always been extremely shitty about getting out of bed in the morning. If we have fights, it’s usually stemming from his behavior and general inability to wake himself up. When we first met in college he would sleep until 6pm or later every single day unless someone woke him up.
So now we live together and for about two years, I’ve woken up early to get him ready for work. He refuses to set his own alarms (we’ve tried but he sleeps through them), meaning I have to set several and try to wake him up several more times a morning. When he finally does get up, he’s usually a complete and total dick. I mean just nasty to me. He usually ends up with about 30 minutes before work to get ready, but likes to take his time and watch the news, meaning he’s usually a little late.
Here’s where I’m concerned I’m an asshole: Recently he’s started taking an additional 20 minutes to smoke weed while watching the news every morning. (Idc about the weed, he works at a pizza shop) But he refuses to get up any earlier, making him around 45 minutes late to work every day. All of this stresses me out so badly. I get stressed because I have to do all this shit to get him out of bed in plenty of time and then he’s still willfully 45 minutes late everyday. Not to mention the added stress of him acting like I am doing this just to hurt him. So, I’ve decided to stop waking him up. No more alarms, no more begging, I’m just going to let him get up when he decides is right.
He will definitely sleep until late afternoon unless I intervene. He will definitely lose his job. I feel the need to prevent that. At the same time, I’m so done being screamed at every morning. WIBTA if I set him up to fail like this?
✨Topic 3✨
['time', 'get', 'like', 'want', 'work', 'one', 'doesn', 'would', 'going', 'take', 'even', 'home', 'really', 'think', 'day', 'every', 'make', 'feel', 'something', 'week']
✨✨✨
0.674436146664204
AITA for complaining she is constantly making plans for both of us?
I (27M) am a “chill at home” type of person. I was diagnosed with depression and its largest effect is on my energy levels. My weight isn’t doing me any favors either. My wife (27F) isn’t incredibly active, but can’t go an entire day without making plans to do something, which is fine. I like doing things with my wife and I can deal deal with her plans most of the time, but sometimes I get home from work and just need to sit in my lazyboy and watch a Netflix.
For example, It’s common to go from having an entire weekend with no plans to her sister and 5 year old to come visit for a whole day. Then the next day we are going to her grandparents. The whole weekend gone in two sentences. I know it might seem normal but it just goes on and on and on. I can’t remember the last time i had a no-obligation, get stuff done (or not) kind of day.
There are two more layers to this.
She NEVER asks me about any of the plans. She is happy to commit my time and then tell me the night before. If I ever want to make my own plans I have to do so around her plans. Plus I always talk to her about it before making them.
I don’t know if she does it on purpose or not, but she makes me feel bad telling her I don’t want to do what she has planned. Sometimes i think it is on purpose because she is always saying “you never want to do anything”. I could do 8 or 9 of 10 things she wants and still be told this. It just seems manipulative and inconsiderate.
This has led to me becoming bitter at times. We have small arguments about it from time-to-time, she keeps making plans, and I keep piling on stress. It’s gotten to the point that I contest her plans every time.
However, today she laid out “our” weekend plans and i said “Nope, I’m staying home. You can do that on your own for once.” She comes back with “We haven’t seen them in forever.” I said “Well I could go a little longer.” She stormed off and I left for work.
From her perspective I’m sure it’s annoying to have to force me out the door. I could see her thinking that I’m just being lazy. We get along great but she just doesn’t understand that I need more time to relax than normal. AITA for being complaining often and saying what I did?
✨Topic 3✨
['time', 'get', 'like', 'want', 'work', 'one', 'doesn', 'would', 'going', 'take', 'even', 'home', 'really', 'think', 'day', 'every', 'make', 'feel', 'something', 'week']
✨✨✨
0.6179777159182313
AITA for reporting a pregnant coworker to management after discovering we’re all covering for her?
I am 5 months pregnant, coworker is 4 months pregnant, we work in a small team of 8 and often cover each other shifts, stay back when someone has appointments and generally help each other out. I have been really struggling with my pregnancy and have been careful to schedule appointments on my days off and to take as many precautions as I can to avoid impacting everyone else. Coworker, who is saying that she’s having a wonderful, enjoyable pregnancy, is showing up late, then going for a smoke and a coffee before starting. “I’m just running late, at OBGYN” quickly becomes and hour or more late, and isn’t being noted on her time sheets, so the person covering her isn’t getting paid for that time and it’s especially difficult for me when I’m already struggling physically to not know what time I’ll be able to go home. I recently learned that other coworkers have been covering a significant part of her tasks, because they feel badly for her having to do it whilst pregnant- tasks that I’m still doing and have been doing with ease.
Our manager is very accommodating and has made several offers to purchase alternative equipment and make changes in the workplace, that she has refused (as have I so far) because it’s easy to complete these tasks the way things are now. I know they are not aware that coworker simply isn’t doing those things, because our manager has asked me about it recently.
WIBTA if I discussed this with management? Other staff are complaining to me about the additional workload. I’m also being expected to put in additional effort at a time that I have no extra energy to give. Trying to bring it up with coworker hasn’t made a difference, and other staff have already told me they’re too tired to help me when I’m struggling because they’re already helping her. I’m worried that I’m just being petty about everyone fussing over her, when I’ve been working so hard to not impact the rest of the workplace.
Topic 8
To display the top 3 original Reddit posts with the highest probability of containing Topic 8 and with relevant topic words bolded, we will run:
display_bolded_topic_words_in_context(topic_number=14, number_of_documents=5)
✨Topic 14✨
['phone', 'facebook', 'post', 'pictures', 'social', 'account', 'game', 'sent', 'picture', 'video', 'posted', 'media', 'call', 'also', 'message', 'messages', 'number', 'instagram', 'photos', 'sarah']
✨✨✨
0.44218633047423606
AITA for suing over not winning a contest?
I entered a contest from a motion capture company who makes mocap suits. Since I am making a video game with their suit already, I figured i’d enter and try to win the cash prize + a brand new upgraded suit.
​
Only 8 people entered the contest total, all of which were publicly posted with videos. Anyone could go on the site and click Vote and ultimately, after 30 days, the winners would be chosen
​
There were 5 prizes:
$3000 + mocap suit
$2000 + mocap suit
$1000 + mocap suit
mocap suit
mocap suit
​
After the contest started, about four days in, they allowed an additional four people to enter. I complained about it that it wasn’t fair, everyone had three months to prepare their videos and post them. They told me too bad.
​
I have a fair sized community behind my game, so they all voted and we obviously stayed at #1 throughout the whole thing… Until the others figured out how click bots worked. I took video of two people using click bots to get 700 votes in minutes. I sent them over and asked them to place a captcha and a cookie drop on the voting mechanism to keep it fair. They declined. I asked to be removed from the contest, so their PR manager instead reached out to me and apologized and took my advice and implemented those methods. The cheaters votes stopped.
​
I continued promoting the contest on social platforms until the end. All in all, just to reach my own FB followers, my ads cost me $400 to run.
​
I finished the contest with several thousand votes, winners were to be announced on July 15th (today). When they were announced, I was nowhere on there. Not even for honorable mention.
​
Instead someone with 7 votes got 1st place. Someone with 14 votes got 2nd, and so on.
​
My community erupted on their facebook page wondering why we won nothing, the company said it was because “cheating was evident in some of the votes, so they made an administrative decision to give prizes instead to people of their choosing”.
​
I messaged them and asked them for an explanation and they old me “because you complained throughout the contest” and “we do not feel as though you are pitching a product at all”
​
Meanwhile, for the last year and a half, they have been promoting my game on their social channels and have it listed on their highlighted products section on their website.
​
I gave them an opportunity to fix it, but they said they stand by their decision.
​
So, I am suing them for the prize I believe I am owed.
AITA?
✨Topic 14✨
['phone', 'facebook', 'post', 'pictures', 'social', 'account', 'game', 'sent', 'picture', 'video', 'posted', 'media', 'call', 'also', 'message', 'messages', 'number', 'instagram', 'photos', 'sarah']
✨✨✨
0.41619810812974495
AITA for deleting a video off my friends phone.
so my friend runs a Instagram account with around 20,000 followers. she loves to update her fans with what she is doing and so sometimes when we are out she will be on her phone taking videos and such.
yesterday we all decided to have a girls movie night and hang out in pjs and watch scary movies. now my pjs are a crop with no bra and pj shorts. I thought that because I was around friends it would be ok.
I then noticed that she was updating her Instagram story and I saw that she was showing all of us in the video. me and another girl asked her to delete the video as we where both in reveling clothes and did not want that posted on social media. she refused so i grabbed her phone off her and deleted the video.
she got pissed and left, and told me that I have no right to take her phone and delete her video. I’m now thinking I overstepped.
AITA?
✨Topic 14✨
['phone', 'facebook', 'post', 'pictures', 'social', 'account', 'game', 'sent', 'picture', 'video', 'posted', 'media', 'call', 'also', 'message', 'messages', 'number', 'instagram', 'photos', 'sarah']
✨✨✨
0.39980537706581076
AITA for messing with my sister’s fake profile?
Going to try to make this quick. I’m 22 and my sister is 18. I went to my sister’s room to grab a top she had borrowed(we do this all the time, borrow clothes go into each other’s rooms to get them back) and I notice she has Facebook open and the profile pic is…well..me. I have to do a double take because it really caught me off guard. I know I shouldn’t have but I snooped at the profile and it was just pictures of myself- my sister literally has a fake Facebook profile of me.
I’m livid at this point for many reasons. 1. I don’t do social media. I’m a private person and I don’t like pictures of me to be on profiles like that. 2. Some of the pictures she had were ones that she took without me knowing 3. Other pictures that she had were ones I had sent to friends which means she has gone onto my computer and taken pictures off.
I delete all of the pictures and I check her computer and find a folder filled with pictures that she has taken of me and ones that were on my computer- not even all of me per se, ones of my friends, group photos, food I had taken pictures of. I go through even more and find out that she has even stolen nudes that I had taken for my boyfriend.
I deleted the folder completely and went back to the fake facebook profile. I uploaded a single picture of her with the caption “The real me”, changed her name(she was using my middle name and a fake last name) and then left her room. I’ve heard her sobbing from her room all day but I’m too angry and embarrassed to even confront her right now. AITA for messing with the profile and not just confronting her in the beginning?
✨Topic 14✨
['phone', 'facebook', 'post', 'pictures', 'social', 'account', 'game', 'sent', 'picture', 'video', 'posted', 'media', 'call', 'also', 'message', 'messages', 'number', 'instagram', 'photos', 'sarah']
✨✨✨
0.39082206926846264
[Update} WIBTAA if I take only one dog for a walk.
Original post
Update. Baxter couldn’t do stairs or lay down without hurting.. even when I gave him drugs.
​
I let him go this morning. He is with Lincoln, Indigo and Winston now. I have never cried so much.
✨Topic 14✨
['phone', 'facebook', 'post', 'pictures', 'social', 'account', 'game', 'sent', 'picture', 'video', 'posted', 'media', 'call', 'also', 'message', 'messages', 'number', 'instagram', 'photos', 'sarah']
✨✨✨
0.38733474788555244
AITA Changing someone’s instagram password for using my email
I got an email that “my” account name had been changed. Since I didn’t have an account under the supposed name I checked it out.
It wasn’t my account so I dm’ed the other person, “Hi it’s actually [name]. Do you want to change your email?” To which he responded, “No I’m also actually [name] bitch.”
Now this user appeared to be a kid so I decided to use this as a learning opportunity. I changed his password and deleted his recovery phone number. I then gave him a bio which said, “dm me @[my account] with your email so I can change it for you.”